Overcoming Common JavaCV Issues in Scala with SBT
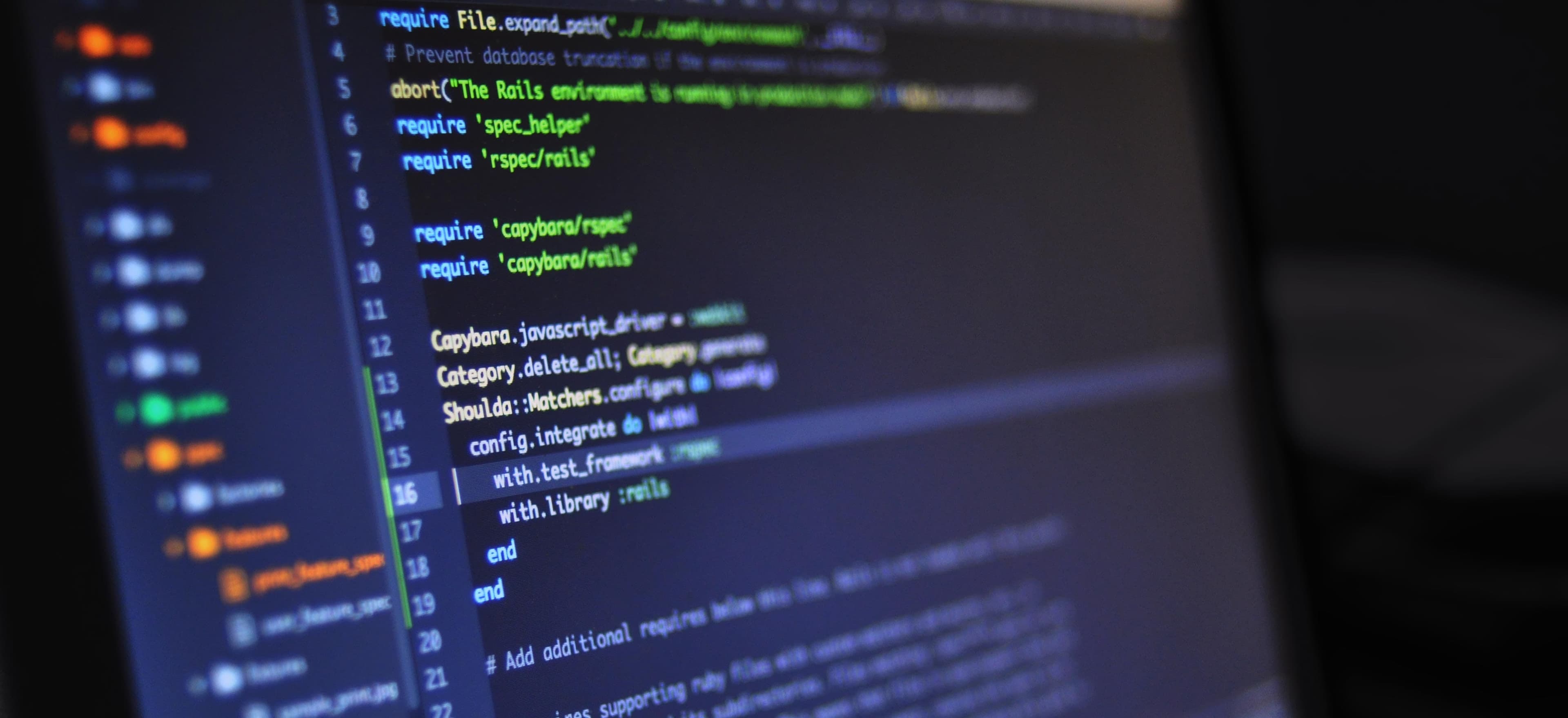
- Published on
Overcoming Common JavaCV Issues in Scala with SBT
JavaCV, an open-source framework for building computer vision applications, is gaining traction among developers, especially those using Scala. Whether you're working with image processing, video analysis, or machine learning in your projects, JavaCV provides a robust set of tools, libraries, and wrappers around popular native libraries like OpenCV.
However, integrating JavaCV with Scala can present challenges, particularly when using SBT (Simple Build Tool) for project management. In this blog post, we'll navigate common issues you may encounter when using JavaCV in Scala projects with SBT, while providing best practices and crucial tips to help you overcome them.
Understanding JavaCV and Scala Integration
JavaCV offers a lot of flexibility when utilizing Java libraries, especially OpenCV. Scala, being interoperable with Java, allows developers to create sophisticated applications leveraging the powerful features of JavaCV. However, the integration does come with its set of pitfalls, such as dependency management, classpath issues, and performance concerns.
This post will focus on resolving common issues to ensure smooth development with JavaCV in Scala projects.
Setting Up SBT for JavaCV
Before we delve into solving specific issues, let's outline how to properly set up SBT for using JavaCV in a Scala project. Here’s a slice of code showcasing an example configuration.
name := "JavaCV-Scala-Project"
version := "0.1"
scalaVersion := "2.13.6"
// JavaCV and OpenCV dependencies
libraryDependencies ++= Seq(
"org.bytedeco" % "javacv" % "1.5.7",
"org.bytedeco" % "opencv" % "4.5.4-1.5.7"
)
// Repositories for JavaCV dependencies
resolvers += "Bytedeco" at "https://repo.bytedeco.org"
Explanation
- Project Name and Version: The basic project metadata.
- Scala Version: Here, we're using Scala 2.13.6, a stable release.
- Dependencies: We include JavaCV and OpenCV libraries, ensuring that we always reference compatible versions.
- Resolving Dependencies: Adding Bytedeco’s repository helps SBT locate JavaCV libraries easily.
Common Issues and Solutions
Issue 1: Dependency Conflicts
Problem: When managing dependencies, conflicts can arise, mainly due to different versions of libraries or transitive dependencies.
Solution: Utilize SBT's dependency tree feature to detect conflicts.
sbt dependencyTree
This command will render the dependency hierarchy, showcasing any potential conflicts. You can force specific versions or exclude certain artifacts by modifying your build.sbt
.
Issue 2: Native Library Loading
Problem: A common problem is the failure to load the native libraries required by JavaCV, leading to exceptions such as "Unable to load native library".
Solution: Make sure native libraries are included in your classpath appropriately. Setting up the java.library.path
can be a solution. You can add the following lines in your build.sbt
file.
javaOptions in run += "-Djava.library.path=/path/to/native/libs"
Loading Native Libraries Example
Suppose you want to load and utilize the JavaCV features for image processing. Here’s how you can write the code with appropriate comments:
import org.bytedeco.javacv.OpenCVFrameConverter
import org.bytedeco.opencv.global.opencv_imgcodecs._
import org.bytedeco.opencv.opencv_core.Mat
object JavaCVExample {
def main(args: Array[String]): Unit = {
// Load an image from a file
val imagePath = "path/to/image.jpg"
val image: Mat = imread(imagePath)
// Check if the image is loaded successfully
if (image.empty()) {
println("Could not load image!")
// Exit if image loading fails
sys.exit(1)
}
// Process the image (e.g. display or manipulate it)
println("Image successfully loaded!")
// More processing can be done here...
// Display the image (code for display would go here)
}
}
Explanation
- Imports: We import necessary classes from JavaCV.
- Image Loading: The
imread
method reads an image using its path. Error handling is incorporated to alert if the image isn’t loaded. - Continuing Processing: Incorporating checks ensures our subsequent processing only occurs if the image was loaded successfully.
Issue 3: ClassLoader Issues on IDE
Problem: ClassLoader conflicts may lead to failures in loading classes when you run your Scala project from an IDE.
Solution: Verify that your IDE is configured correctly to use SBT. If you are using IntelliJ IDEA, ensure that the SBT project settings point to the correct JDK and Scala version.
Another option is to run your application directly through SBT:
sbt run
Running through SBT alleviates any internal IDE classpath mismatches.
Issue 4: Performance Bottlenecks
Problem: JavaCV applications can hit performance bottlenecks due to improper processing or thread management.
Solution: Make use of multi-threading where necessary using Scala's Future
or Akka
actors. This can parallelize tasks like frame capture and processing in video applications.
Here's a simplistic use of Scala's Future
.
import scala.concurrent.ExecutionContext.Implicits.global
import scala.concurrent.Future
val futureResult: Future[Unit] = Future {
// Perform time-consuming processing here
}
Final Thoughts
The integration of JavaCV into Scala projects with SBT can come with its challenges, but understanding common issues and their solutions can help you smoothly navigate through the process. Always keep your dependencies in check, ensure native libraries are accessible, and consider performance management through multi-threading.
This post serves as a stepping stone into the world of JavaCV and Scala. As you deepen your understanding and experiment with the framework, you will uncover more sophisticated features and encounter unique challenges—a hallmark of growing as a developer.
Additional Resources
To further your knowledge, consider checking the following links:
- JavaCV Official Documentation
- SBT Documentation
- Scala Futures
By taking proactive measures to understand and handle common issues, you can unlock the fantastic potential that JavaCV offers within the Scala ecosystem. Go ahead, start building your next computer vision project with confidence!
Checkout our other articles