Choosing the Right Dynamic Proxy: Common Pitfalls Explained
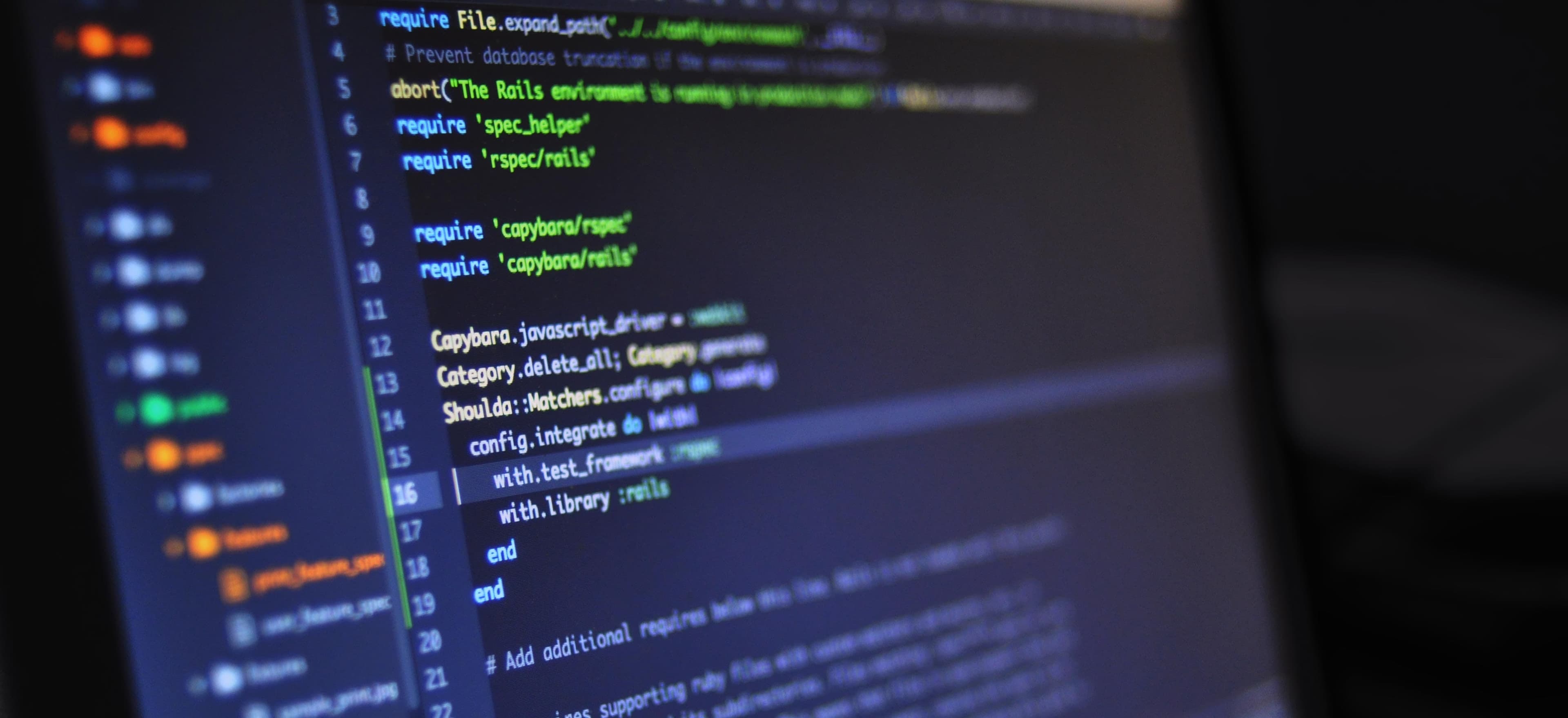
- Published on
Choosing the Right Dynamic Proxy: Common Pitfalls Explained
In the realm of Java programming, dynamic proxies offer an invaluable mechanism for creating flexible and reusable components. With dynamic proxies, developers can intercept method calls, allowing for functionality such as logging, security checks, or even remote procedure calls. Despite their advantages, new developers, and even seasoned programmers, can stumble upon common pitfalls when implementing dynamic proxies. In this blog post, we will explore these pitfalls, provide insights on how to avoid them, and demonstrate the functionality with code examples.
What is a Dynamic Proxy?
A dynamic proxy in Java is an instance of a class that implements a specified interface — created at runtime. It acts as a mediator for method calls directed at interface methods. A dynamic proxy can be created using java.lang.reflect.Proxy
along with an InvocationHandler
that defines how to handle these calls.
Why Use Dynamic Proxies?
Dynamic proxies promote cleaner, more modular code. Here are some of the benefits:
- Separation of Concerns: Business logic stays decoupled from cross-cutting concerns such as logging and transaction management.
- Flexibility: You can easily change behavior or add new capabilities without modifying existing code.
Common Pitfalls When Using Dynamic Proxies
While the advantages are clear, developers often encounter the following pitfalls.
1. Not Understanding Interfaces
Dynamic proxies work only with interfaces. You cannot create a proxy for classes. If you try to do so, you will face IllegalArgumentException
.
Example:
import java.lang.reflect.Proxy;
public class ProxyExample {
public static void main(String[] args) {
SomeClass obj = new SomeClass();
// This will fail because SomeClass is not an interface.
SomeClass proxy = (SomeClass) Proxy.newProxyInstance(
SomeClass.class.getClassLoader(),
new Class[]{ SomeClass.class },
new MyInvocationHandler(obj));
}
}
Solution
Always ensure that you have an interface. If your design does not follow interfaces, consider refactoring your code to adopt an interface-based approach.
2. Overusing Proxies
While dynamic proxies can make your code flexible, overusing them can lead to complexity that is hard to debug. It’s essential to ask when a proxy is truly necessary.
Example:
public interface Service {
void execute();
}
public class ServiceImpl implements Service {
public void execute() {
System.out.println("Service executed");
}
}
public class MyInvocationHandler implements InvocationHandler {
private final Service service;
public MyInvocationHandler(Service service) {
this.service = service;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) {
System.out.println("Before execution");
Object result = method.invoke(service, args);
System.out.println("After execution");
return result;
}
}
// Usage
Service service = new ServiceImpl();
Service proxy = (Service) Proxy.newProxyInstance(Service.class.getClassLoader(),
new Class[]{Service.class}, new MyInvocationHandler(service));
proxy.execute();
Solution
Use dynamic proxies judiciously. Focus on scenarios where behavior needs to be modified at runtime or when adhering to cross-cutting concerns.
3. Ignoring Performance Overhead
Dynamic proxies come with a performance cost. The framework has to handle reflection and method invocation, adding overhead.
Impact:
In scenarios involving high-frequency method calls, using dynamic proxies can slow down an application.
Solution
Evaluate whether the performance cost is justifiable. Profiling your application can help assess the impact that proxies have on your system.
4. Poor Exception Handling
When using dynamic proxies, exception handling can become convoluted. Failing to properly handle exceptions in the InvocationHandler
may lead to unhandled exceptions or swallowed exceptions.
Example:
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
try {
return method.invoke(service, args);
} catch (InvocationTargetException e) {
throw e.getCause(); // Bad practice: Ignoring the original exception context
}
}
Solution
Always maintain a clear strategy for exception handling. Specificity enhances maintainability.
5. Not Implementing Cloneable or Serializable
If your proxy needs to be serialized or cloned, you should be mindful of the InvocationHandler
. The default implementation of these interfaces may not suffice, leading to erroneous behavior.
Example:
public class SerializableInvocationHandler implements InvocationHandler, Serializable {
private final Service service;
// Constructor and invoke method...
}
// Usage
SerializableInvocationHandler handler = new SerializableInvocationHandler(service);
Service proxy = (Service) Proxy.newProxyInstance(Service.class.getClassLoader(),
new Class[]{Service.class}, handler);
Solution
Always implement Serializable or Cloneable interfaces if your proxy needs to be serialized. You also need to be cautious about serialization-related issues in the InvocationHandler
.
6. Failing to Respect Method Contracts
When using a proxy, ensure that you respect the methods' contracts. Method contracts involve expected behavior, preconditions, postconditions, and invariants.
Example:
@Override
public Object invoke(Object proxy, Method method, Object[] args) {
// Potentially violating contract by failing to validate input
return method.invoke(service, args);
}
Solution
Always validate inputs as per the method's contracts. Failing to do so could lead to unexpected behavior, violating the expected contract and resulting in security vulnerabilities.
My Closing Thoughts on the Matter
Dynamic proxies are a powerful feature in Java that promote clean, maintainable code. However, it’s crucial to be aware of potential pitfalls. By keeping the above considerations in mind, you can harness the power of dynamic proxies effectively while avoiding common traps.
Additional Resources
Dynamic proxies can make your application robust when used wisely. Happy coding!