Choosing the Right Error Tracking Tool: A Comparison Guide
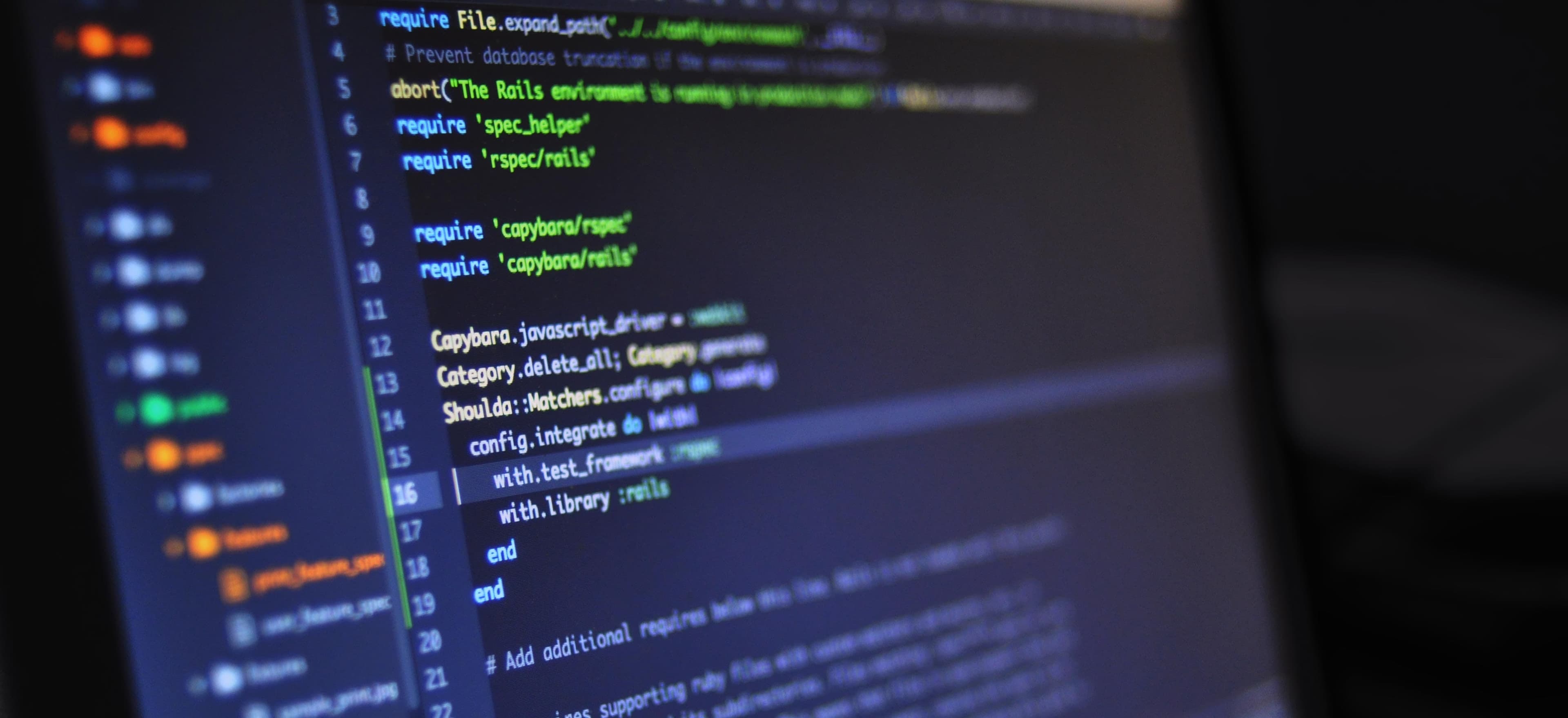
- Published on
Choosing the Right Error Tracking Tool: A Comparison Guide
Error tracking is a fundamental aspect of software development, especially for Java applications. Every developer knows that bugs occur, and how swiftly they are identified and resolved can significantly impact the overall user experience. With so many options available, selecting the right error tracking tool can be daunting. This guide compares some of the leading error tracking tools to help you make an informed decision.
Why Is Error Tracking Important?
Error tracking allows you to:
- Identify Issues Early: Catch bugs in real-time for quicker resolutions.
- Enhance User Experience: Ensure your application runs smoothly for users.
- Boost Developer Productivity: Save developers time, allowing them to focus on innovation rather than troubleshooting.
- Improve Code Quality: Understand recurring issues and refine the coding process.
Common Features of Error Tracking Tools
Before we dive into specific tools, here are common features you should look for:
- Real-Time Alerts: Provides immediate notifications when an error occurs.
- Stack Trace Information: Offers detailed error logs to help identify the source of the problem.
- Integration Capabilities: Works seamlessly with other tools in your stack, like CI/CD pipelines and project management software.
- User Feedback: Enables users to report issues directly, augmenting your tracking data.
- Analytics and Reporting: Gives insights into trends and recurring problems.
Popular Error Tracking Tools
Let’s evaluate some of the most widely used error tracking tools in the Java ecosystem.
1. Sentry
Overview: Sentry is an open-source error tracking tool that provides real-time crash reporting for modern applications.
Key Features:
- Real-time error tracking
- Intelligent aggregation of similar errors
- Issue resolution workflow integration
Why Use Sentry? Sentry’s real-time alerts and comprehensive stack trace info help developers quickly locate and fix issues. Its deployment tracking feature shows you which version of your application an error occurred in, allowing for precise fixes.
Example Code Snippet: To integrate Sentry into your Java application, you would typically add the following code:
import io.sentry.Sentry;
public class SentryExample {
public static void main(String[] args) {
Sentry.init("your-sentry-dsn");
try {
// Code that might throw an exception
Integer.parseInt("not-an-integer");
} catch (Exception e) {
Sentry.captureException(e); // Captures the exception
}
}
}
In this snippet, we initialize Sentry with a Data Source Name (DSN) and capture any exceptions. This helps in tracking errors without impacting user experience.
More Information: Sentry Documentation.
2. Rollbar
Overview: Rollbar provides real-time error tracking for developers, allowing them to detect and resolve issues faster.
Key Features:
- Real-time error monitoring
- Automatic deployment tracking
- Rich API for customizing integrations
Why Use Rollbar? The deployment tracking feature automatically associates errors with specific code deployments. This allows you to quickly identify if a recent change introduced a bug.
Example Code Snippet: Setting up Rollbar in a Java app can be done as follows:
import com.rollbar.api.Rollbar;
public class RollbarExample {
public static void main(String[] args) {
Rollbar rollbar = Rollbar.init("access-token");
try {
// Potentially problematic code
throw new RuntimeException("Sample exception");
} catch (Exception e) {
rollbar.error(e); // Log the error to Rollbar
}
}
}
In this example, we initialize Rollbar with an access token and log errors. This integration is straightforward, enabling efficient error handling.
More Information: Rollbar Documentation.
3. Bugsnag
Overview: Bugsnag is designed for modern applications and provides advanced error monitoring and reporting.
Key Features:
- Automatic error monitoring
- API access for customized error reporting
- User feedback collection tools
Why Use Bugsnag? The standout feature of Bugsnag is its stability score, which helps you determine how many users are affected by a particular bug.
Example Code Snippet: To implement Bugsnag in a Java application, do the following:
import com.bugsnag.Bugsnag;
public class BugsnagExample {
public static void main(String[] args) {
Bugsnag bugsnag = new Bugsnag("YOUR_API_KEY");
try {
// Potential issue triggering code
String test = null;
System.out.println(test.length());
} catch (Exception e) {
bugsnag.notify(e); // Notify Bugsnag of the error
}
}
}
In this code snippet, we notify Bugsnag when an exception occurs, which helps in automated error reporting.
More Information: Bugsnag Documentation.
4. Loggly
Overview: Loggly is a cloud-based log management solution that provides error tracking among other logging features.
Key Features:
- Centralized logging
- Real-time error tracking
- Advanced search capabilities
Why Use Loggly? Loggly offers powerful log analytics, helping you gain insights into your application logs and track down issues quickly.
Example Code Snippet: To log an error in a Java application, you might set up Loggly like this:
import com.loggly.log4j.LogglyAppender;
public class LogglyExample {
public static void main(String[] args) {
LogglyAppender appender = new LogglyAppender();
appender.setLogglyKey("LOGGLY_KEY");
try {
// Code that triggers an error
System.out.println(1 / 0);
} catch (Exception e) {
appender.error("Error occurred:", e); // Log to Loggly
}
}
}
Here, we utilize LogglyAppender to log errors efficiently, ensuring you capture critical information.
More Information: Loggly Documentation.
Making the Right Choice
Choosing the right error tracking tool depends on your specific needs:
- For Real-Time Monitoring: Sentry or Rollbar take the lead with their robust real-time capabilities.
- For Detailed Analytics: Bugsnag stands out with its stability score.
- For Log Management: Loggly excels with its advanced log analytics features.
Before deciding, consider your project's scale, team size, existing tools, and budget. Many of these tools offer free tiers or trials, so be sure to test them out.
Lessons Learned
Error tracking is no longer a luxury; it’s a necessity for any serious developer. By leveraging the right tools, you not only reduce the time spent debugging but also enhance the overall quality of your Java applications. Each error tracking tool has its strengths and weaknesses; therefore, the best choice revolves around your specific use case and requirements.
With this guide, you’re now equipped to make an informed decision on which error tracking tool to implement in your development process.
Explore more about the tools mentioned in this post using the links provided, and take your error tracking capability to the next level. Happy coding!