Common Pitfalls in Spring Cloud AWS Proxy Settings
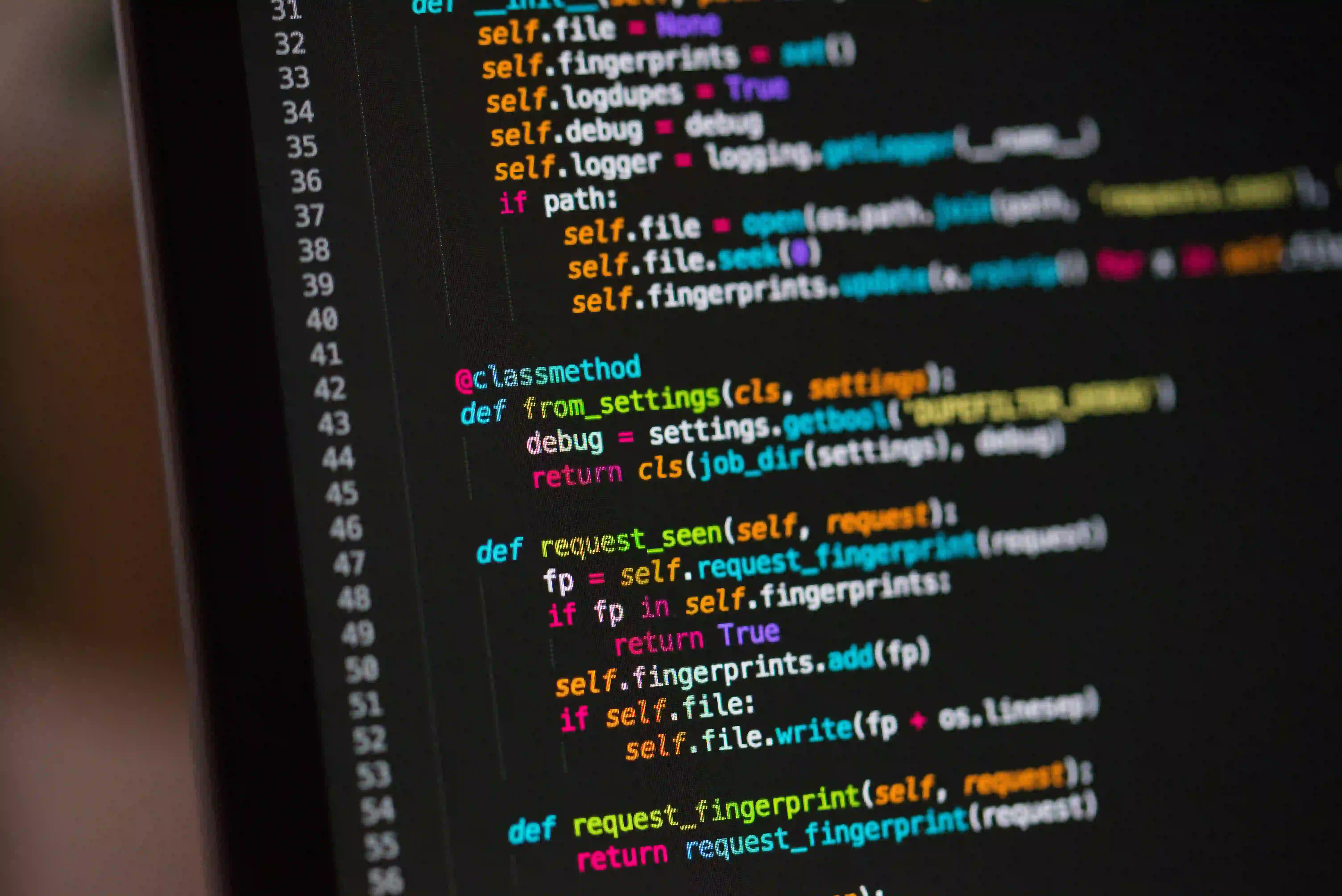
Common Pitfalls in Spring Cloud AWS Proxy Settings
Spring Cloud AWS simplifies the integration of AWS services within Spring applications. However, working with proxies—especially in cloud environments—can lead to several pitfalls. This blog post aims to elucidate some common mistakes developers make when configuring proxy settings in Spring Cloud AWS, offering clarity, code examples, and recommended solutions.
Understanding Spring Cloud AWS
Spring Cloud AWS allows for seamless integration with various AWS services such as S3, DynamoDB, and more, making it easier for developers to access AWS resources. It provides robust abstractions over these resources, allowing Spring developers to leverage AWS’s capabilities with familiar Spring patterns.
When using these integrations, especially in environments with proxy servers, careful configuration is essential. Neglecting proper proxy settings can lead to connectivity issues, latency, and security vulnerabilities.
Common Pitfalls to Avoid
Let's delve into some widespread issues when configuring Spring Cloud AWS proxy settings.
1. Incorrect Proxy Configuration
One of the most fundamental issues developers face relates to improper proxy configuration.
Example:
spring:
cloud:
aws:
java-sdk:
region: us-west-2
proxy:
host: your-proxy-host
port: 8080
username: your-username
password: your-password
Why is this a problem? Omitting key details or providing incorrect values can result in failure to establish a connection to AWS services via the proxy. Additionally, ensure the URI formats are valid and acceptable by your proxy server.
Solution: Double-check your configuration details. Utilize environment variables for sensitive information like usernames and passwords. This approach enhances security and simplifies configuration management.
spring:
cloud:
aws:
java-sdk:
proxy:
host: ${PROXY_HOST}
port: ${PROXY_PORT}
username: ${PROXY_USER}
password: ${PROXY_PASSWORD}
2. Missing Proxy Dependencies
Using Spring Cloud AWS effectively requires that you have the appropriate dependencies included in your project. Failing to include necessary libraries can lead to classes not being found and incompatible methods being invoked.
Example Dependency:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-aws</artifactId>
<version>2.3.2</version>
</dependency>
Why is this a problem? If the necessary libraries for proxy settings are absent, the application may not compile, or worse, it may run but fail to connect to AWS services as expected.
Solution: Ensure you have the square build dependencies. Refer to the official Spring Cloud AWS documentation for a complete list of dependencies you might need.
3. Failure to Handle Authentication
When using proxies that require authentication, developers often overlook implementation. Proxy servers frequently require credentials for secure access.
Example:
System.setProperty("http.proxyUser", "your-username");
System.setProperty("http.proxyPassword", "your-password");
Why is this a problem? If authentication details are omitted, the proxy will deny access, causing an inability to reach AWS services.
Solution: Integrate proper authentication management using Spring's properties. Leverage Spring Security to encapsulate your authentication credentials. For example:
spring:
security:
user:
name: your-username
password: your-password
4. Ignoring Thread Context
When making requests through a proxy, it’s essential to manage the thread context correctly. Failing to pass required context information can disrupt request handling and compromise service quality.
Example:
String proxyHost = System.getProperty("http.proxyHost");
String proxyPort = System.getProperty("http.proxyPort");
Why is this a problem? Lack of context passing can lead to confusing results, as different threads may not have access to the necessary proxy configuration.
Solution: Utilize proper context management libraries or frameworks when working in multi-threaded environments. This strategy ensures that proxy settings persist across thread boundaries.
5. Neglecting Health Checks
In a cloud environment, connectivity can be fickle. A common pitfall is the neglect of regular health checks against the AWS services through proxies.
Why is this a problem? Without periodic checks, your application could perform poorly, leading to hidden errors that affect user experience and service reliability.
Solution: Implement health check endpoints in your application to verify the status of proxy settings and connectivity to AWS services. Incorporate health monitoring tools such as Spring Actuator.
import org.springframework.boot.actuate.health.Health;
import org.springframework.boot.actuate.health.HealthIndicator;
import org.springframework.stereotype.Component;
@Component
public class AwsHealthIndicator implements HealthIndicator {
@Override
public Health health() {
// Check AWS service connectivity
boolean isUp = checkAwsService();
if (isUp) {
return Health.up().build();
}
return Health.down().withDetail("Error", "Cannot connect to AWS").build();
}
private boolean checkAwsService() {
// Implement your proxy service connectivity logic here
return true; // Placeholder
}
}
6. Overlooking Performance Tuning
Proxy connections inherently introduce a performance overhead. Failing to optimize these connections can lead to slower application responses.
Why is this a problem? At scale, these minor performance costs can lead to significant latency and degrade user experience.
Solution: Consider configuring connection pooling and optimization parameters like connection timeout settings. For example:
spring:
cloud:
aws:
java-sdk:
client:
config:
connectionTimeout: 1000 # 1 second timeout
requestTimeout: 5000 # 5 seconds request timeout
This setup ensures efficient proxy utilization, enhancing your application's overall performance and reliability.
Closing Remarks
Configuring Spring Cloud AWS with proxy settings can be fraught with challenges. As we've explored, these common pitfalls can lead to connectivity issues or application inefficiencies. Awareness and careful planning around proxy configuration can mitigate these challenges significantly.
For further insights into improving your AWS application management, explore the AWS Well-Architected Framework and consider integrating proper logging mechanisms for debugging purposes.
Avoid these pitfalls by staying informed, continually optimizing, and ensuring secure access through your proxy settings. By mastering these common issues, developers will pave the way for successfully deploying their Spring Cloud applications in AWS environments.