Common Errors When Generating QR Codes in Java
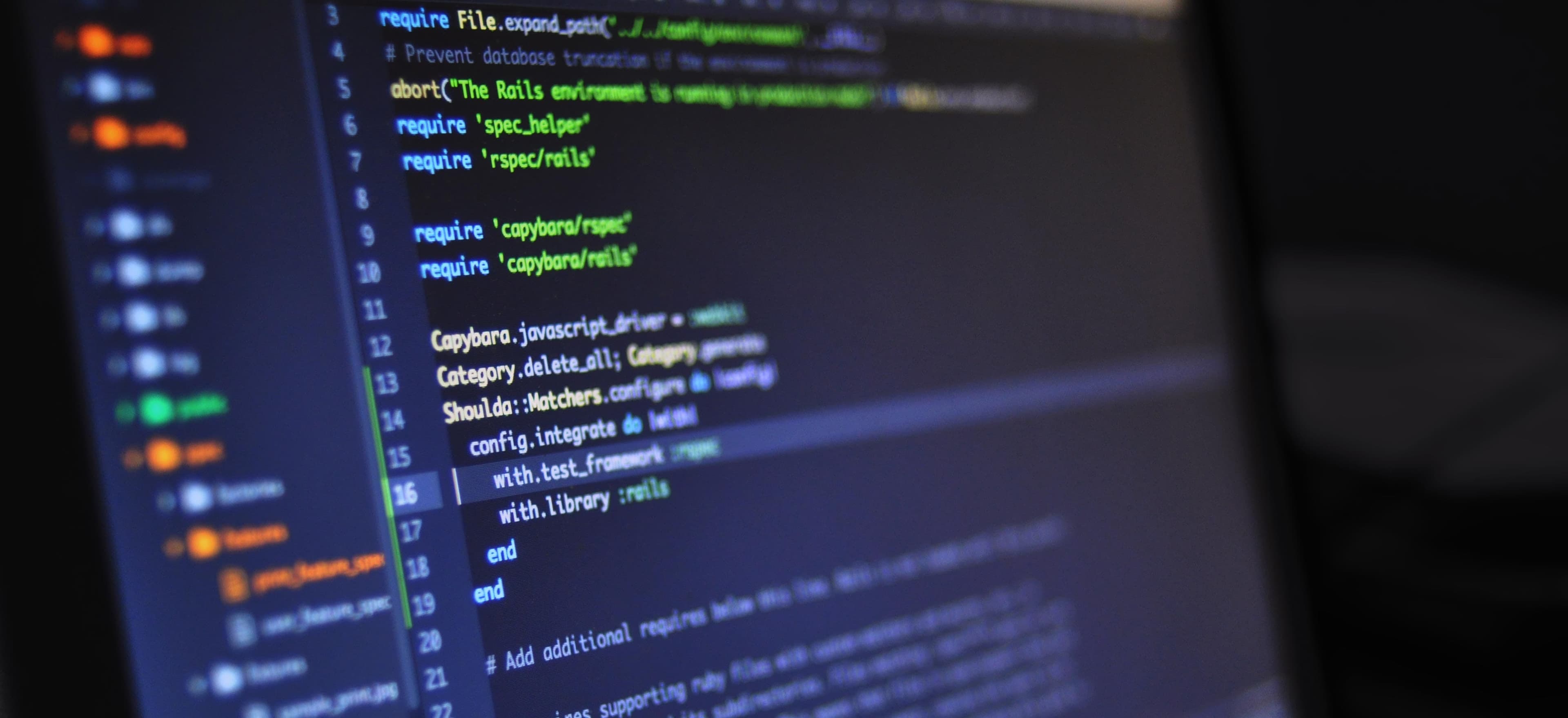
- Published on
Common Errors When Generating QR Codes in Java
In today’s digital age, QR (Quick Response) codes are everywhere. You see them on product packaging, posters, and even business cards. These tiny squares can hold a wealth of information, making them incredibly useful for both personal and professional purposes. If you’re working with Java and want to generate QR codes, you might encounter a few common errors along the way. This blog post dives into those challenges and provides solutions, ensuring you can create functional QR codes efficiently.
Understanding QR Code Generation in Java
Before addressing common errors, it’s essential to understand how QR code generation works in Java. The most popular libraries used are ZXing (Zebra Crossing) and QRGen. ZXing is an open-source library that makes it easy to create QR codes and barcodes in Java.
Here’s a simple example of generating a QR code using the ZXing library:
import java.awt.image.BufferedImage;
import java.io.File;
import javax.imageio.ImageIO;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.WriterException;
import com.google.zxing.client.j2se.MatrixToImageWriter;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.QRCodeWriter;
public class QRCodeGenerator {
public static void main(String[] args) {
try {
String qrCodeText = "https://example.com";
String filePath = "QRCode.png";
int size = 250;
QRCodeWriter qrCodeWriter = new QRCodeWriter();
BitMatrix bitMatrix = qrCodeWriter.encode(qrCodeText, BarcodeFormat.QR_CODE, size, size);
// Create a BufferedImage from the BitMatrix
BufferedImage qrImage = MatrixToImageWriter.toBufferedImage(bitMatrix);
// Write the BufferedImage to a file
ImageIO.write(qrImage, "PNG", new File(filePath));
System.out.println("QR Code generated at: " + filePath);
} catch (WriterException e) {
System.err.println("Error in generating QR code: " + e.getMessage());
} catch (Exception e) {
System.err.println("Error saving the QR code image: " + e.getMessage());
}
}
}
In this snippet:
- QRCodeWriter is used to generate the QR code.
- The BitMatrix holds the QR code data.
- We convert the BitMatrix into a BufferedImage and save it as a PNG file.
Now, let’s explore common errors you might encounter while using this code.
Common Errors When Generating QR Codes
1. Java Developer Environment Setup Issues
Symptoms:
- Your code compiles but throws exceptions when executed.
Cause:
- The ZXing library is not included in your project's build path or dependencies.
Solution:
Make sure to add ZXing to your project. If you’re using Maven, include the following dependency in your pom.xml
:
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>core</artifactId>
<version>3.3.3</version>
</dependency>
Why this matters: Proper library inclusion ensures that your project can compile with the required classes.
Additional Resources:
For more information on ZXing, visit the ZXing GitHub page.
2. Incorrect Input Data for QR Codes
Symptoms:
- You receive a
WriterException
or a blank QR code.
Cause:
- The input string (URL or text) is formatted incorrectly, or it exceeds the QR code's data capacity.
Solution:
Make sure the data is encoded properly. URLs should start with "http://" or "https://", and keep text within reasonable limits.
String qrCodeText = "https://example.com"; // Valid URL input
Why this matters: Inaccurate data leads to unreadable QR codes. Keeping your data concise allows for more effective QR code designs.
3. Output File Not Found or Access Denied
Symptoms:
- The application throws an IOException stating that the file cannot be created.
Cause:
- You might be trying to write to an unauthorized directory or running in an environment with limited file access.
Solution:
- Specify an accessible file path, like your Desktop or use a relative path to your project:
String filePath = System.getProperty("user.home") + "/Desktop/QRCode.png"; // Valid path
Why this matters: Writing permissions ensure that the application can output files without running into security issues.
4. QR Code Appears Blurry or Pixelated
Symptoms:
- The generated QR code is unclear when scanned.
Cause:
- Insufficient resolution or improper image size.
Solution:
Increase the size parameter when generating the QR code:
int size = 500; // Higher resolution for clearer QR code
Why this matters: Higher resolutions result in clearer QR codes, reducing scanning errors.
5. Missing Image Type in ImageIO.write()
Symptoms:
- The code executes without errors, but no image file is created.
Cause:
- A wrong or unsupported image format is specified.
Solution:
- Ensure you're using a valid type like "PNG" or "JPG":
ImageIO.write(qrImage, "PNG", new File(filePath)); // Correct format
Why this matters: Using a recognizable format is crucial for image generation and storage.
Advanced Considerations
Customizing QR Code Properties
Beyond the basic QR code creation process, you might want to customize the appearance of your QR code. ZXing allows for some level of customization regarding the error correction level:
QRCodeWriter qrCodeWriter = new QRCodeWriter();
HashMap<EncodeHintType, Object> hintMap = new HashMap<>();
hintMap.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.L); // Low error correction
BitMatrix bitMatrix = qrCodeWriter.encode(qrCodeText, BarcodeFormat.QR_CODE, size, size, hintMap);
Why this matters: The error correction level defines how much of the QR code can be damaged while remaining functional. Adjusting this helps in scenarios where the QR code may become scratched or dirty.
Troubleshooting Scanning Issues
If your QR code is generated correctly but fails to scan, consider the following troubleshooting tips:
- Ensure good lighting conditions. Poorly lit areas can hinder scanning.
- Avoid reflections. Ensure the QR code does not reflect light from nearby sources.
- Test with different scanning applications. Some apps handle QR codes better than others, so it might not be an issue with your code.
To Wrap Things Up
Generating QR codes in Java can be straightforward, yet several common pitfalls can complicate the process. By being aware of these potential issues and knowing how to address them, you can optimize your QR code generation workflows effectively. Whether you are building a marketing campaign, enhancing user engagement, or simply experimenting with QR codes, these insights will boost your development experience.
For more information on QR code generation and advanced techniques, consider exploring resources like Java Code Geeks or Stack Overflow.
By following the tips outlined in this blog post, you are well on your way to mastering QR code generation in Java! Happy coding!
Checkout our other articles