Overcoming Common Pitfalls in XML to Avro Conversion
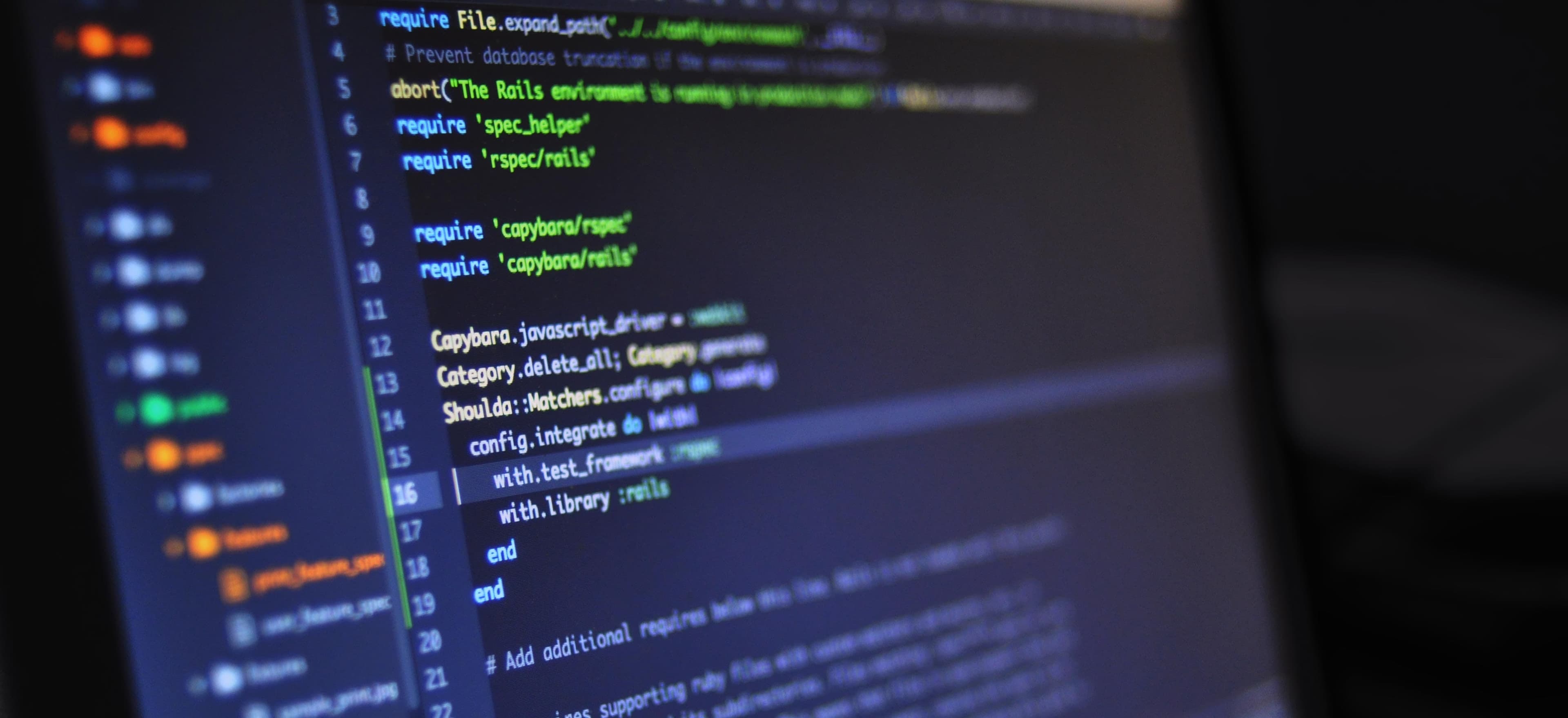
- Published on
Overcoming Common Pitfalls in XML to Avro Conversion
In today’s data-driven world, converting data from one format to another is a common task, and XML to Avro conversion is gaining traction due to the advantages of Avro. This blog post will explore common pitfalls in this conversion process and offer solutions to navigate them effectively.
Understanding XML and Avro
Before diving into the problems you may face, it’s crucial to understand the two formats:
-
XML (Extensible Markup Language) is a markup language that defines rules for encoding documents in a format that is both human-readable and machine-readable. It is widely used for data interchange on the web, owing to its flexibility and compatibility across different systems.
-
Avro, on the other hand, is a data serialization system. It provides a compact, fast, and efficient way to store data. Avro is particularly popular in big data contexts, especially with Apache Hadoop, because it supports both schema evolution and dynamic typification.
Common Pitfalls in XML to Avro Conversion
1. Ignoring Schema Definition
One of the most frequent oversight is neglecting the schema definition during conversion. Avro relies heavily on a defined schema for data serialization and deserialization.
Solution:
Always start by defining your Avro schema according to the XML structure. Here is an example:
{
"type": "record",
"name": "Person",
"fields": [
{"name": "name", "type": "string"},
{"name": "age", "type": "int"},
{"name": "email", "type": "string"}
]
}
Commentary:
This schema outlines a Person
record that includes name
, age
, and email
. This explicit definition allows Avro to accurately serialize and deserialize the data while ensuring compatibility with data structures over time. For more on Avro schemas, check Apache Avro Documentation.
2. Nested Structures Handling
XML often contains nested structures which can complicate conversion to Avro's flat file format.
Solution:
Flatten these structures appropriately. For instance, consider the following XML example:
<employees>
<employee>
<name>John Doe</name>
<age>30</age>
<address>
<city>New York</city>
<state>NY</state>
</address>
</employee>
</employees>
You might convert this to an Avro schema like this:
{
"type": "record",
"name": "Employee",
"fields": [
{"name": "name", "type": "string"},
{"name": "age", "type": "int"},
{"name": "city", "type": "string"},
{"name": "state", "type": "string"}
]
}
Commentary:
This flattened structure maintains the essential details while ensuring that it fits into Avro's schema-required format. Nested elements are flattened to attributes at the root level to meet Avro's requirements.
3. Data Type Mismatches
Another pitfall arises when the data types between XML and Avro are not correctly aligned. XML does not enforce data types, while Avro does.
Solution:
Convert XML data types to the appropriate Avro types. For example, consider an XML element like this:
<age>30</age>
This should map directly to int
in Avro. However, if you encounter:
<age>30 years</age>
You need to strip the string to use it effectively.
String ageStr = xmlElement.getAge();
int age = Integer.parseInt(ageStr.replaceAll("[^0-9]", ""));
Commentary:
This code extracts the numeric part of the string by removing non-numeric characters before parsing it into an integer. Matching data types accurately is essential for preventing errors during serialization.
4. Special Characters and Encoding
XML documents may contain special characters or different encodings that can cause conversion errors.
Solution:
Normalize your XML data before conversion by encoding it to ensure compatibility with Avro’s specifications.
String normalizedData = xmlData.replace("&", "&")
.replace("<", "<")
.replace(">", ">");
Commentary:
The above code snippet safeguards against XML parsing errors by converting special characters into their encoded equivalents. Proper encoding allows the conversion process to handle diverse characters seamlessly.
5. Performance Issues
When dealing with large XML datasets, performance issues can manifest during the conversion to Avro, particularly due to memory management.
Solution:
Implement a streaming approach. Instead of loading the entire XML document into memory, process it in chunks. Utilize Java libraries like StAX
(Streaming API for XML):
XMLInputFactory factory = XMLInputFactory.newInstance();
XMLStreamReader reader = factory.createXMLStreamReader(inputStream);
while (reader.hasNext()) {
int event = reader.next();
// Process each XML element here
}
Commentary:
The code above reads the XML document in a streaming manner, thereby reducing memory overhead and allowing for handling larger documents efficiently. This method is particularly beneficial when dealing with substantial datasets and resource constraints.
The Closing Argument
Converting XML to Avro can enhance data serialization and parsing efficiency. However, it is crucial to be mindful of the common pitfalls we’ve discussed. By taking preemptive measures—defining schemas, flattening nested structures, ensuring data type compatibility, handling special characters, and optimizing performance—you can ensure a smooth conversion process.
For further reading on efficient data handling practices with Avro, feel free to explore Apache Avro Best Practices.
Happy coding!