Debugging API Gateway Issues with Couchbase Integration
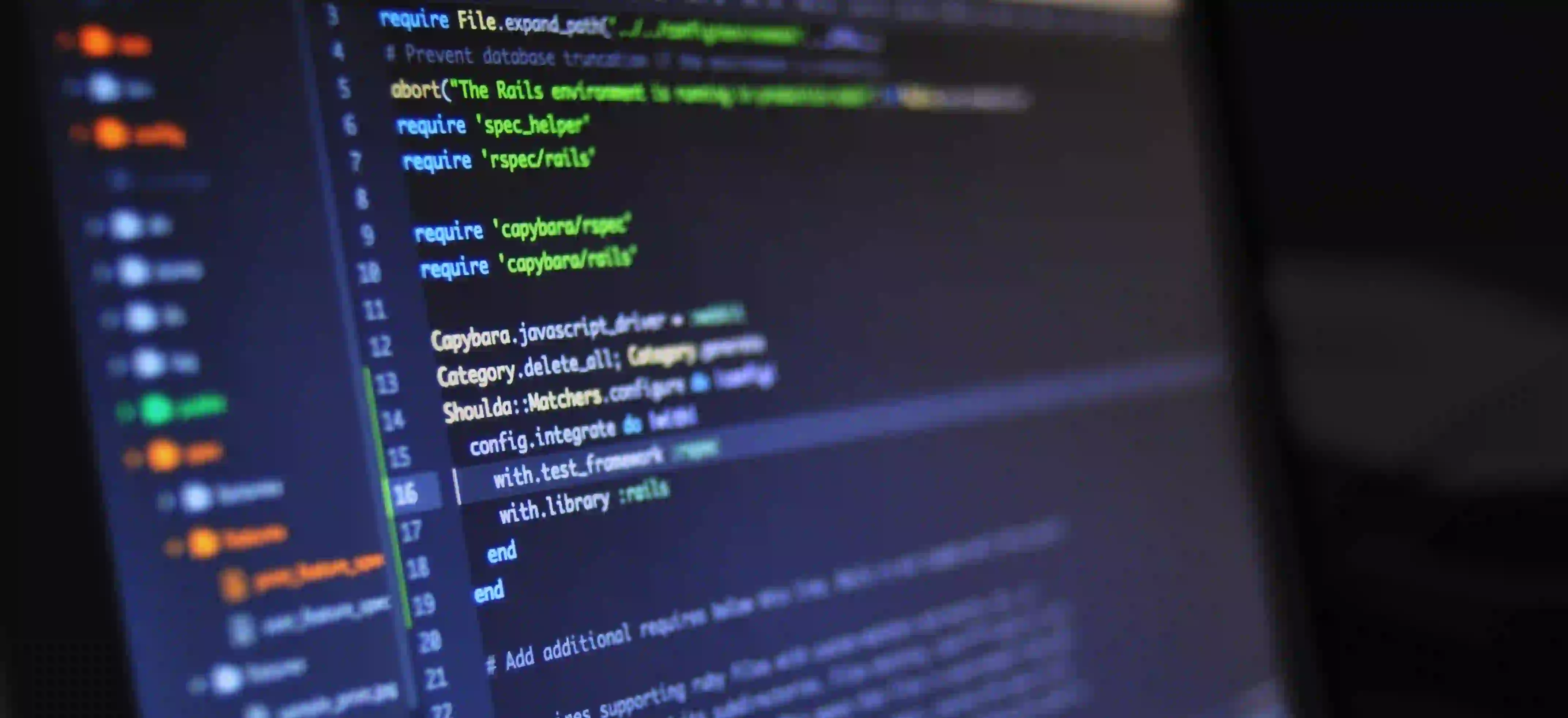
Debugging API Gateway Issues with Couchbase Integration
When building modern applications, integrating an API Gateway with a database like Couchbase can significantly enhance scalability, flexibility, and performance. However, like any architecture comprising multiple components, challenges can arise. Debugging these issues effectively is crucial to maintaining a robust application. In this post, we will explore strategies for diagnosing problems when integrating an API Gateway with Couchbase.
Understanding the Components
Before delving into debugging techniques, it's essential to understand the components involved in this architecture:
-
API Gateway: This serves as the entry point for client requests. It handles tasks such as request routing, composition, and protocol translation.
-
Couchbase: A NoSQL database that provides high performance, scalability, and flexibility. It features a document-based data model, which is ideal for modern applications.
-
Client Application: The front-end application interacting with the API Gateway.
Understanding these components helps in pinpointing where issues may arise.
Common Issues in API Gateway and Couchbase Integration
1. Authentication Failures
Problem: If the API Gateway requires authentication (e.g., using JWT tokens) and the token is absent, expired, or malformed, requests to Couchbase will fail.
Solution:
- Ensure that the token is included in the request headers.
- Validate the token's structure and expiration.
Code Snippet:
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jwts;
public Claims decodeJWT(String jwt) {
Claims claims = Jwts.parser()
.setSigningKey(YOUR_SECRET_KEY)
.parseClaimsJws(jwt)
.getBody();
return claims;
}
In the snippet above, we decode a JWT token to obtain claims. It's crucial for validating that the token is issued properly and has not expired.
2. Connection Issues with Couchbase
Problem: The API Gateway might be unable to connect to Couchbase due to various reasons, such as incorrect connection strings or network issues.
Solution:
- Check your connection string for accuracy.
- Verify that the network security groups allow access between the API Gateway and the Couchbase cluster.
Code Snippet:
String clusterAddress = "couchbase://localhost";
Cluster cluster = Cluster.connect(clusterAddress, "username", "password");
In this example, we've created a cluster connection to Couchbase. If the parameters are incorrect, this connection will throw an exception, leading to debugging efforts.
3. Query Errors in Couchbase
Problem: Queries sent from the API Gateway to Couchbase can fail due to syntax errors or incorrect N1QL queries.
Solution:
- Inspect the query syntax and ensure that it follows the N1QL guidelines.
- Use Couchbase's Query Workbench for debugging complex queries.
Code Snippet:
String query = "SELECT name FROM `bucket-name` WHERE type = $type";
N1qlQuery n1qlQuery = N1qlQuery.parameterized(query, JsonObject.create().put("type", "user"));
Here, we parameterize our N1QL query to avoid SQL injection attacks. Always ensure that your queries are properly structured and utilize parameters effectively.
Logging and Monitoring
Implementing Logs
Effective logging provides insight into the interactions between your API Gateway and Couchbase. Consider:
- Logging all incoming and outgoing requests.
- Incorporating logging at various levels (INFO, DEBUG, ERROR).
Code Snippet:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ApiService {
private static final Logger logger = LoggerFactory.getLogger(ApiService.class);
public void processRequest(Request request) {
logger.info("Processing request: {}", request);
// Process request...
}
}
Using a logging framework like SLF4J allows you to maintain a clear record of operations, which is invaluable in tracing issues.
Monitoring Tools
Leverage tools to monitor API performance, such as:
- Couchbase Dashboard: Provides real-time information on queries, operations, and server health.
- API Gateway Metrics: Most API Gateway implementations offer built-in metrics for request processing time, failure rates, etc.
Debugging Steps
To systematically address issues, follow these steps:
- Check Connection: Validate connections between the API Gateway and Couchbase, ensuring network accessibility.
- Review Logs: Analyze log files for unusual patterns or error messages.
- Test Individual Components: Isolate the API Gateway from Couchbase. Test each component independently to narrow down which is causing the issue.
- Use Monitoring Tools: Implementing monitoring tools can provide near real-time insights into how queries are performing.
- Debug Incrementally: Introduce logging at code breakpoints to pinpoint where things go awry.
Best Practices
- Use Version Control: Maintain your Couchbase database version and API code. It simplifies rollbacks and tracking.
- Maintain Query Optimization: Keep your N1QL queries optimized for speed.
- Implement Circuit Breaker Patterns: These prevent cascading failures between your API Gateway and Couchbase. If Couchbase is unresponsive, it will stop requests until it's healthy again.
- Regularly Update Dependencies: Ensure that both your API Gateway and Couchbase SDKs are up-to-date to benefit from bug fixes and performance improvements.
In Conclusion, Here is What Matters
Debugging API Gateway and Couchbase integration issues requires a structured approach to diagnosing problems. Grasping the relationship between components, utilizing effective logging, and leveraging advanced monitoring techniques can dramatically improve your response to issues as they arise.
For a deeper dive into debugging techniques, check out the official Couchbase documentation and AWS API Gateway documentation. By refining your debugging strategies, you can ensure seamless integration and a better user experience in your applications.