Mastering Java's Serial Annotation: Common Pitfalls Explained
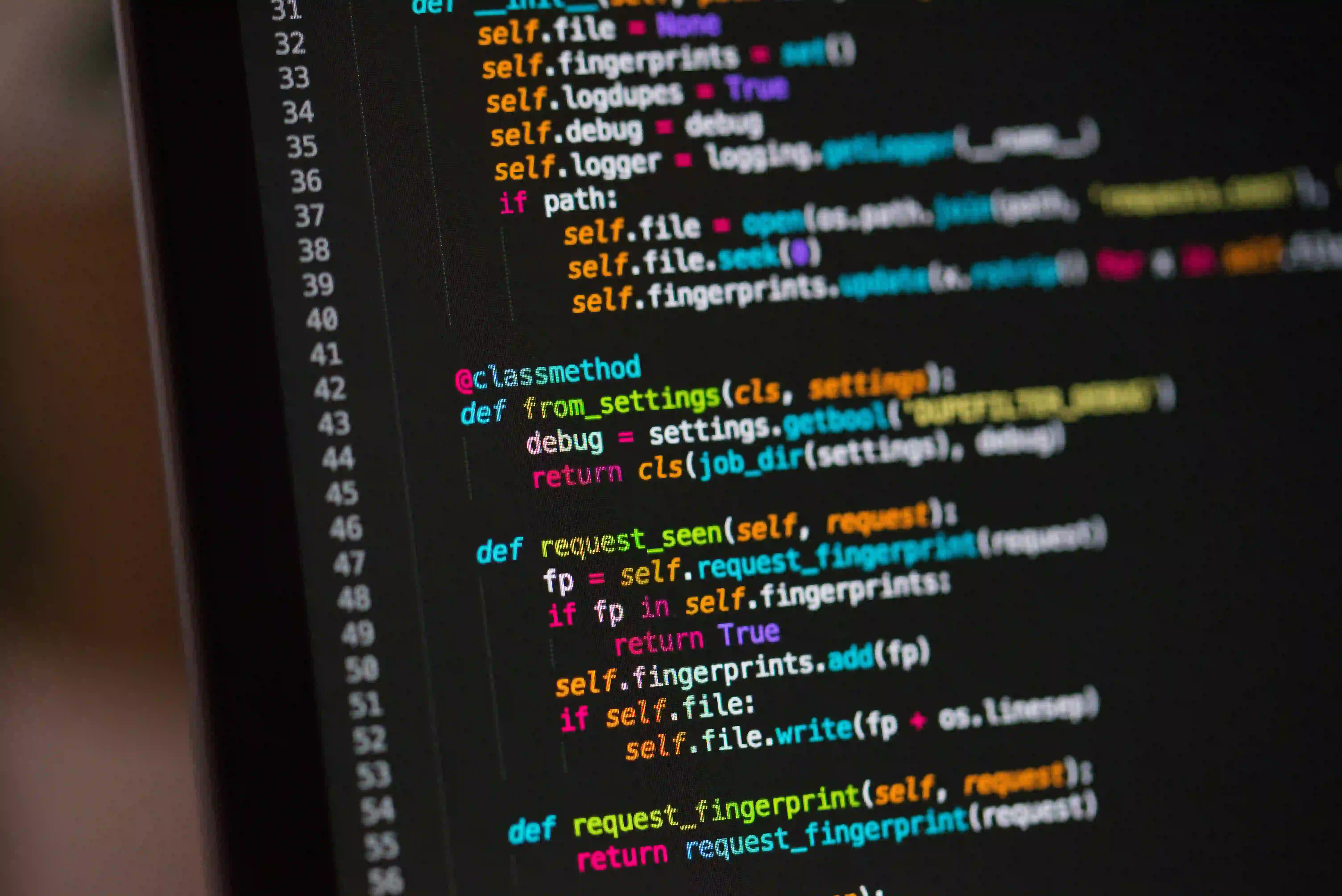
Mastering Java's @Serial
Annotation: Common Pitfalls Explained
Java is a powerful language, and with its robust set of features, it strives to make the developer's experience as seamless as possible. One such feature is the serialization mechanism, which allows the conversion of an object into a byte stream for easy storage or transmission. An integral part of this mechanism is the @Serial
annotation, introduced in Java 14 to enhance the clarity and correctness of the serialization process. In this article, we will explore the @Serial
annotation in detail, discuss its significance, and highlight common pitfalls developers face.
Understanding Serialization in Java
Before diving into the @Serial
annotation, it's essential to grasp what serialization in Java entails. Serialization is fundamentally a mechanism for object persistence. It enables developers to save an object's state to a file and then reconstruct it later. The two primary interfaces associated with serialization are:
- Serializable: A marker interface that must be implemented by classes intending to be serialized.
- Externalizable: An interface allowing full control over the serialization process by overriding
readExternal
andwriteExternal
methods.
Example of a Serializable Class
import java.io.*;
public class User implements Serializable {
private static final long serialVersionUID = 1L; // Unique version identifier
private String username;
public User(String username) {
this.username = username;
}
public String getUsername() {
return username;
}
}
Why it's Important: The serialVersionUID
field ensures that during deserialization, the JVM can verify that the sender and receiver of a serialized object maintain compatibility in terms of serialization. A mismatch can lead to InvalidClassException
.
The @Serial
Annotation
With the introduction of the @Serial
annotation, Java brought more clarity to how developers should indicate serialization methods and fields. The @Serial
annotation is used to mark fields and methods that are relevant to serialization in the context of a Serializable
or Externalizable
class, improving the readability and maintainability of the code.
Example Usage of @Serial
Here is an example demonstrating the use of the @Serial
annotation:
import java.io.*;
public class Product implements Serializable {
private static final long serialVersionUID = 1L;
@Serial
private transient String sensitiveData; // Indicates this field will not be serialized
@Serial
private void writeObject(ObjectOutputStream oos) throws IOException {
oos.defaultWriteObject(); // Perform default serialization
oos.writeObject(sensitiveData); // Serializing sensitive data explicitly
}
@Serial
private void readObject(ObjectInputStream ois) throws IOException, ClassNotFoundException {
ois.defaultReadObject(); // Perform default deserialization
sensitiveData = (String) ois.readObject(); // Deserializing sensitive data explicitly
}
}
Why it's Important: The @Serial
annotation explicitly indicates that these methods are related to serialization, making the intent clear to anyone reading the code. This helps avoid bugs and misunderstandings, particularly in large codebases.
Common Pitfalls with the @Serial
Annotation
While the @Serial
annotation offers clarity and structure, developers still face various pitfalls. Below are the most common issues encountered.
1. Forgetting serialVersionUID
As noted earlier, the serialVersionUID
is pivotal in ensuring version compatibility. Many developers often overlook this or fail to declare it entirely. Without this ID, changes in class structure can lead to deserialization errors.
Solution: Always define a unique serialVersionUID
for your classes implementing Serializable
.
2. Misusing the @Serial
Annotation
The @Serial
annotation is part of the serialization process. Still, if it's misused on non-serializable fields or methods, it can lead to confusion and compile-time errors. For instance, marking a transient field without understanding its purpose can lead to loss of critical data.
Solution: Use @Serial
judiciously. Ensure that methods and fields marked have clear roles in serialization.
3. Ignoring transient
Fields marked as transient
are not serialized, which means they won't retain their state across sessions. Developers sometimes assume that everything must be serialized, forgetting to mark sensitive or irrelevant fields as transient
, leading to potential memory leaks or exposure of sensitive information.
Solution: Understand the implications of transient
and apply it to fields where necessary.
More Examples to Avoid Pitfalls
Consider a class where a developer incorrectly marks a method.
Incorrect Usage
import java.io.*;
public class Order implements Serializable {
private static final long serialVersionUID = 1L;
private String orderId;
@Serial // This should not be here; using wrong annotation purposefully
private void finalize() {
// Incorrect use
}
}
Why It's Wrong: Using @Serial
on the finalize
method will lead to misunderstandings about its purpose. Finalization is unrelated to serialization, and this misuse can confuse other developers.
Best Practices for Using @Serial
- Always Define
serialVersionUID
: This cannot be stressed enough. It should reflect changes in the class structure. - Proper Annotation Usage: Use the
@Serial
annotation exclusively for serialization-related methods and fields. - Avoid Default Serialization When Possible: Consider implementing custom serialization logic to address intricate needs instead of relying solely on default serialization.
Further Reading
- Java Serialization - Oracle
- Effective Java by Joshua Bloch: A must-read book for Java developers.
The Last Word
Understanding and correctly using the @Serial
annotation is crucial for Java developers who work with serialization. By avoiding common pitfalls and adhering to best practices, developers can create efficient and maintainable serialized objects within their applications. It’s not just about making your code functional; it's also about writing code that's clear and comprehensible to current and future team members.
By navigating through the challenges and leveraging the potential of the @Serial
annotation, you can significantly improve your Java serialization process. Keep it simple, keep it clear, and happy coding!