Overcoming Continuous Delivery Downtime: Key Strategies
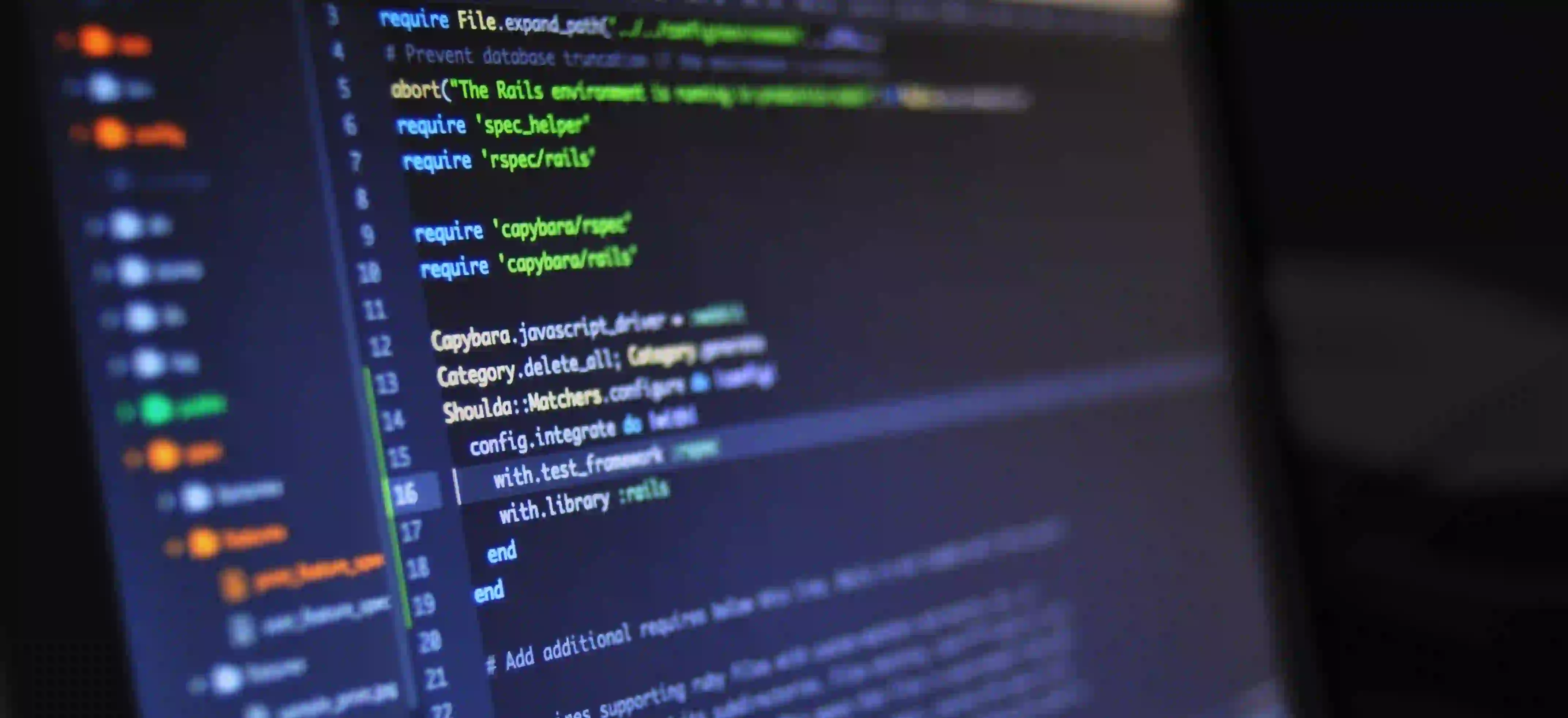
Overcoming Continuous Delivery Downtime: Key Strategies
Continuous Delivery (CD) is a software development practice that allows teams to release applications frequently and reliably. However, the prospect of downtime during deployments poses a significant challenge for development teams. In this blog post, we’ll explore effective strategies to overcome downtime in Continuous Delivery, ensuring a smoother operational flow while maintaining product quality.
Understanding Continuous Delivery Downtime
Downtime refers to periods during which an application is unavailable to its users. This can occur during deployment processes, where new features or updates are being integrated into the production environment. Downtime can lead to user dissatisfaction, loss of revenue, and damage to brand reputation. Therefore, minimizing or eliminating downtime is critical.
The Importance of Zero Downtime Deployments
Zero Downtime Deployment (ZDD) is an approach that effectively allows updates to be made without interruption. In a world increasingly leaning on 24/7 availability, ZDD is not merely a luxury; it's becoming a necessity. It enhances user experience and preserves the integrity of ongoing transactions.
Strategies for Achieving Zero Downtime
1. Blue-Green Deployments
Blue-Green Deployment is a technique that uses two identical environments. One, the "blue" environment, is currently live, while the other, the "green", is idle.
How It Works:
- Deploy the new application version to the green environment while the blue environment is still serving all traffic.
- Once all tests succeed in the green environment, switch traffic to it.
- If issues arise, you can revert traffic back to the blue environment without any downtime.
Example Code Snippet
# Switch traffic from blue to green
aws elbv2 modify-listener --listener-arn <listener-arn> --default-actions Type=forward,TargetGroupArn=<green-target-group-arn>
Why This Works: The beauty of Blue-Green Deployments lies in its simplicity. Rollbacks become as effortless as flipping a switch, providing a high level of assurance against downtime.
2. Canary Releases
Canary Releases allow you to roll out the new version to a small subset of users before a full-scale deployment. This method lets you monitor the performance and catch any issues before impacting the entire user base.
How It Works:
- Release the new version to a small number of users.
- Monitor key metrics and feedback.
- Gradually increase the percentage of users receiving the new version as confidence grows.
Example Code Snippet
In a deployment pipeline, you might conditionally route traffic like this:
if (isCanaryVersion) {
routeTrafficToCanary();
} else {
routeTrafficToStable();
}
Why This Works: It's a powerful way to mitigate risk. By testing changes on a smaller scale, developers gain insights quickly and can make informed decisions before a wide rollout.
3. Feature Toggles (Feature Flags)
Feature Toggles are a strategic way to control the visibility of new features in production. This method allows developers to deploy code with new features hidden from users until they are fully ready.
How It Works:
- Deploy new code along with the feature toggle.
- The feature is hidden from users until toggled on.
- Once it is verified to work as expected, switch the toggle to enable the feature to all users.
Example Implementation
Using a Feature Toggle library, you might use:
if (FeatureToggle.isEnabled("newFeature")) {
showNewFeature();
} else {
showOldFeature();
}
Why This Works: This strategy provides a way to separate deployment from feature release. Developers can continuously push code while controlling user experience precisely.
4. Database Migration Strategies
Database changes often introduce downtime, especially in monolithic architectures. Using careful migration strategies can help alleviate this issue.
Techniques Include:
- Rolling Migrations: Update the database schema gradually rather than doing a big bang, which can lead to downtime.
- Backwards Compatible Changes: Always ensure that new changes don’t break existing functionality.
Example Code Snippet
To roll out migrations:
ALTER TABLE users ADD COLUMN new_col VARCHAR(50) DEFAULT NULL; -- Non-breaking change
Why This Works: Ensuring backwards compatibility ensures that existing users are not affected by changes, reducing the risk of downtime significantly.
5. Load Balancing
Utilizing Load Balancers can help manage traffic during deployments. By distributing user requests evenly, load balancing ensures that not all users experience the deployment simultaneously.
Implementation Note
Using tools like AWS Elastic Load Balancer (ELB), you can route traffic dynamically:
# Register new instance to load balancer
aws elbv2 register-targets --target-group-arn <target-group> --targets Id=<instance-id>
Why This Works: This method minimizes the risk of overwhelming servers during deployment, thus ensuring that user experience remains unaffected.
6. Infrastructure as Code (IaC)
Managing infrastructure with IaC allows for scalable and reproducible environments. Tools like Terraform or AWS CloudFormation can help automate infrastructure management.
Benefits:
- Consistency: All environments can be replicated with identical configurations.
- Simplicity: Infrastructure changes can be rolled back seamlessly.
Example Terraform Code
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "ExampleInstance"
}
}
Why This Works: Infrastructure as Code facilitates rapid deployment and rollback, ultimately reducing the risk of human error and downtime.
Bringing It All Together
Navigating the challenges of Continuous Delivery does not need to compromise your application's availability. By leveraging strategies like Blue-Green Deployments, Canary Releases, Feature Toggles, and effective Database Migration techniques, teams can minimize or even eliminate downtime during deployments.
Remember, the goal is to deliver value continuously while ensuring smooth user experiences. Adopting these tactics allows organizations to achieve operational excellence, providing significant business advantages in today’s fast-paced technology landscape.
For more insights on Continuous Delivery and avoiding downtime, consider checking out articles from Martin Fowler, a well-known voice in the software development community.
By implementing these best practices, you can ensure a robust deployment pipeline that meets today’s demands without sacrificing quality or user satisfaction. Happy Deploying!