Overcoming Latency Issues When Streaming from MongoDB GridFS
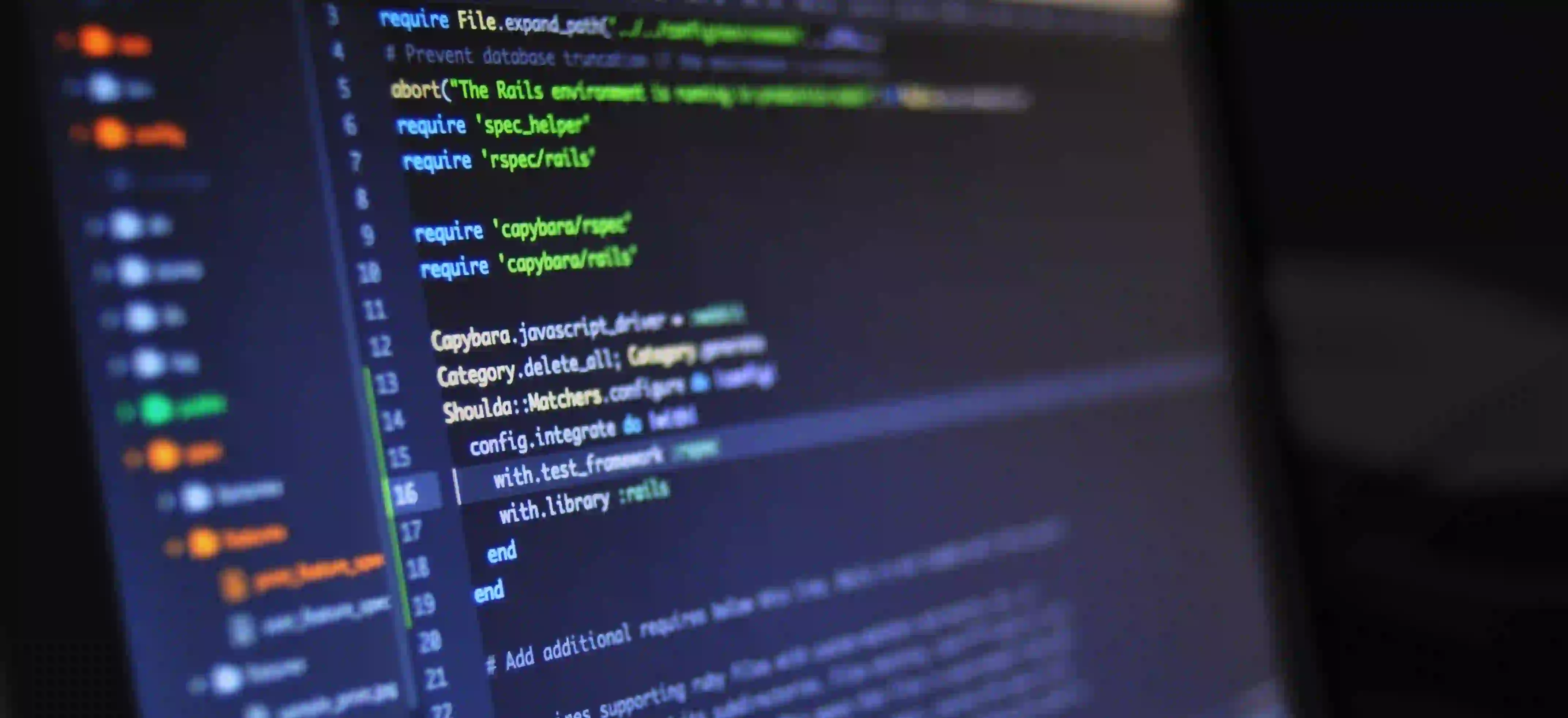
Overcoming Latency Issues When Streaming from MongoDB GridFS
In the digital age, user experience hinges on speed. In web applications, especially those focused on content delivery, latency can cripple performance. This article dives into how to streamline the process of streaming large files from MongoDB's GridFS, making it not just feasible but efficient.
GridFS is a specification for storing and retrieving large files such as images, audio files or video content in MongoDB. While GridFS offers a robust solution for handling large files, it can introduce latency challenges that developers must address.
Understanding GridFS
Before delving into solutions, it's vital to grasp what GridFS is at its core. Unlike conventional file storage, GridFS divides files into smaller chunks (default size is 255 KB). These chunks are stored as separate documents in a MongoDB collection, allowing for efficient handling of large files. This design provides many benefits:
- Scalability: Easily store files larger than the MongoDB BSON document size limit (16 MB).
- Streamability: Helps in streaming files instead of loading complete files into memory.
But, with these advantages come some inherent latency drawbacks, particularly when dealing with large files or high concurrency levels.
Identifying Latency Issues
Streaming files from GridFS can produce latency for several reasons:
- Chunk Retrieval Time: Since files are broken into smaller chunks, retrieving many chunks can add up and lead to delays.
- Network Overhead: Overheads caused by the unoptimized network requests can slow down the data transfer.
- Database Load: Heavy load on databases due to multiple concurrent connections can delay responses.
Addressing these issues requires a comprehensive strategy. Let's outline some effective methods here.
Strategies to Reduce Latency
1. Connection Pooling
Connection pooling allows multiple requests to share a set of established database connections. This is significant because establishing a connection to a database can be resource-intensive and time-consuming.
Code Snippet for Connection Pooling
import com.mongodb.MongoClient;
import com.mongodb.MongoClientURI;
MongoClientURI uri = new MongoClientURI("mongodb://yourUsername:yourPassword@localhost:27017/?maxPoolSize=50");
MongoClient mongoClient = new MongoClient(uri);
Why it Works: By reusing existing connections, your application minimizes the duplicated overhead of establishing new connections, which leads to reduced latency.
2. Use of Asynchronous Processing
Implementing asynchronous processing ensures the application remains responsive while waiting for large file chunks to be processed.
Code Snippet for Asynchronous Streaming
import com.mongodb.client.gridfs.GridFSBucket;
import com.mongodb.client.gridfs.GridFSBuckets;
import com.mongodb.client.MongoDatabase;
import java.util.concurrent.CompletableFuture;
MongoDatabase database = mongoClient.getDatabase("yourDatabase");
GridFSBucket gridFSBucket = GridFSBuckets.create(database);
public CompletableFuture<Void> streamFile(String fileId) {
return CompletableFuture.runAsync(() -> {
try (OutputStream outputStream = new FileOutputStream("path/to/destination")) {
gridFSBucket.downloadToStream(new ObjectId(fileId), outputStream);
System.out.println("Streamed file: " + fileId);
} catch (IOException e) {
e.printStackTrace();
}
});
}
Why it Works: By offloading the file streaming to a separate thread, the main application remains responsive. Users can continue to interact with the application while files are processed.
3. Optimal Chunk Size
While GridFS defaults to a chunk size of 255 KB, experimenting with different chunk sizes can yield improved latency results. It’s essential to balance between the number of chunks and their size.
Code Snippet for Setting Chunk Size
GridFSBucket gridFSBucket = GridFSBuckets.create(database, "files", new GridFSBucketOptions().chunkSizeBytes(512 * 1024));
Why it Works: Increasing the chunk size reduces the total number of chunks and, subsequently, the number of database requests made. However, testing must be conducted to determine the optimal size for specific use-cases.
4. Efficient Indexing
GridFS relies on the underlying MongoDB collections, and ensuring that appropriate indexes exist can drastically improve performance. Particularly, indexing on fields frequently queried or filtered can help avoid full collection scans.
Code Snippet for Indexing
import com.mongodb.client.MongoCollection;
import org.bson.Document;
MongoCollection<Document> filesCollection = database.getCollection("fs.files");
filesCollection.createIndex(Indexes.ascending("filename"));
Why it Works: Indexes allow MongoDB to locate data faster, significantly reducing the latency associated with retrieving large files when users request a specific file.
5. Caching Frequently Accessed Files
Caching can minimize redundant network trips. By storing frequently accessed files in-memory or using an external caching service like Redis, the time to retrieve data can be significantly decreased.
Code Snippet for Caching Example
import redis.clients.jedis.Jedis;
// Initialize Redis cache
Jedis jedis = new Jedis("localhost");
public byte[] getFromCacheOrDB(String fileId) {
String cacheKey = "file_" + fileId;
byte[] cachedData = jedis.get(cacheKey.getBytes());
if (cachedData != null) {
return cachedData;
} else {
// Retrieve from MongoDB and cache it
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
gridFSBucket.downloadToStream(new ObjectId(fileId), outputStream);
byte[] data = outputStream.toByteArray();
jedis.set(cacheKey.getBytes(), data);
return data;
}
}
Why it Works: By retrieving data from cache when available, latency in file retrieval can be massively reduced.
Monitoring and Ongoing Optimization
Finally, leveraging monitoring tools to analyze the performance of MongoDB can uncover bottlenecks and areas for improvement. Keep an eye on:
- Connection pool usage
- Query execution times
- Index usage statistics
Tools like MongoDB Atlas offer built-in performance monitoring, which can give insights into potential concerns.
Lessons Learned
Streaming files efficiently from MongoDB's GridFS requires a combination of solid practices around connection management, processing, chunk handling, and caching. By implementing these strategies, you can significantly reduce latency, delivering a smooth and responsive user experience.
When tackling latency issues, remember that there is no one-size-fits-all solution. Experimentation, monitoring, and tailoring your approach to specific application needs are key to overcoming the challenges associated with streaming from MongoDB GridFS.
For further reading, check out the official MongoDB GridFS documentation and explore how different configurations can fit your use case.
By focusing on these diverse strategies, developers can enhance their applications' performance and provide users with a more engaging and uninterrupted experience.
This post encapsulates techniques necessary for optimizing streaming from MongoDB GridFS. Stay tuned for more deep dives into performance optimization and advanced MongoDB techniques.