Common Pitfalls in Building RESTful Web Services with Java
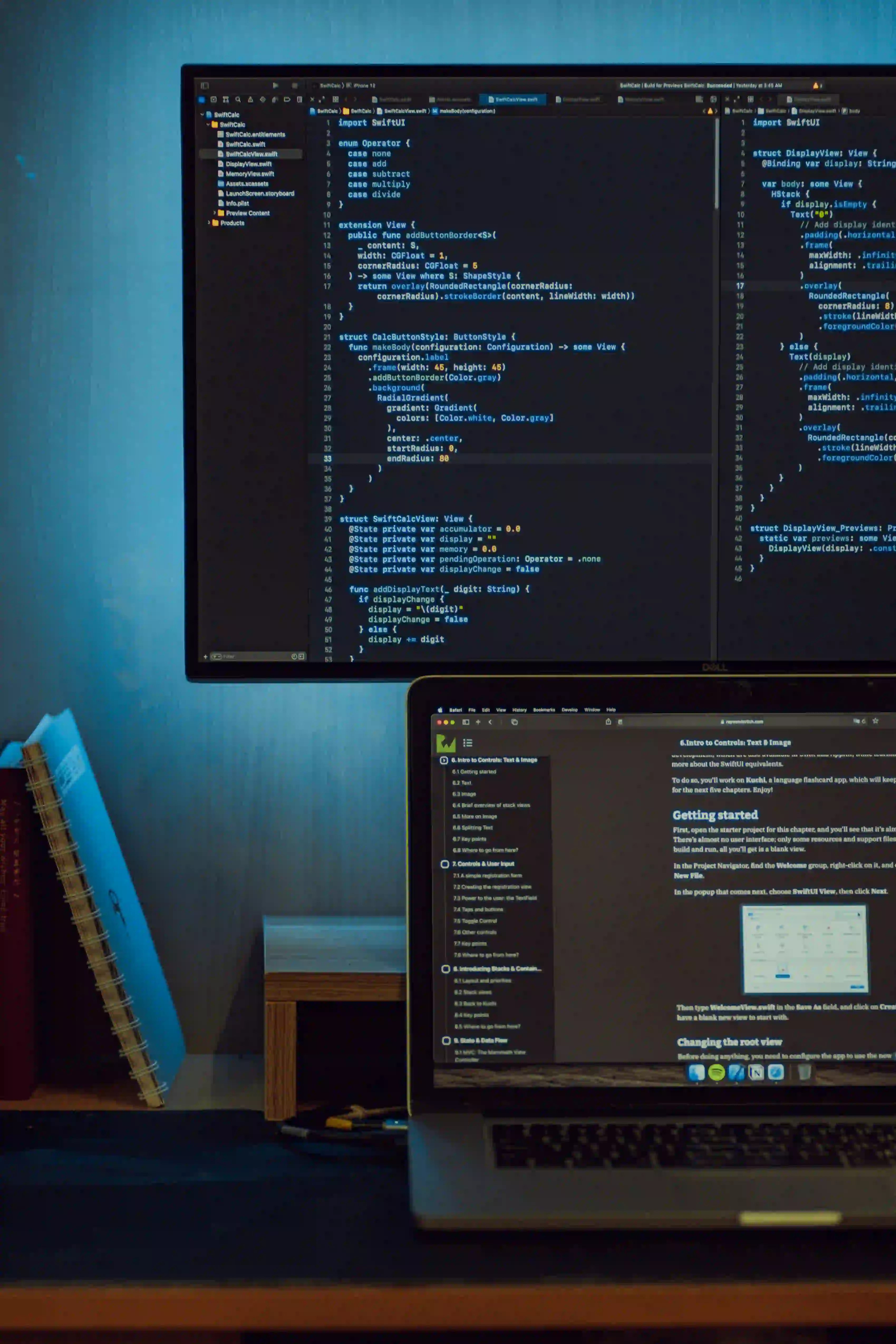
Common Pitfalls in Building RESTful Web Services with Java
Creating RESTful web services in Java can be a rewarding but challenging endeavor. As developers, we often encounter common pitfalls that can hinder our development process and result in inefficient, unmaintainable, and even insecure applications. In this post, we will explore these pitfalls, offering insights and code examples to guide you in building efficient RESTful services.
Understanding REST
Before diving into the common pitfalls, let’s briefly discuss what REST (Representational State Transfer) is. REST is an architectural style that allows for interaction between clients and servers through stateless communication, often using HTTP. It emphasizes a resource-based approach, where each resource is represented by a URL. For more detailed exploration of REST, consider checking out REST API Tutorial.
Pitfall 1: Ignoring HTTP Status Codes
Why It Matters
One of the cornerstones of RESTful web services is the proper use of HTTP status codes. Many developers unknowingly overlook this aspect, leading to confusion for the clients consuming the API.
Common Mistake
Returning the same status code for every response. For instance, an application may always return 200 OK, regardless of whether the request was successful or resulted in an error.
Correct Approach
By using appropriate status codes, clients can programmatically ascertain the outcome of their requests. Here's an example:
import javax.ws.rs.*;
import javax.ws.rs.core.*;
@Path("/users")
public class UserService {
@GET
@Path("/{id}")
@Produces(MediaType.APPLICATION_JSON)
public Response getUser(@PathParam("id") String id) {
User user = findUserById(id);
if (user == null) {
return Response.status(Response.Status.NOT_FOUND).entity("User not found").build();
}
return Response.ok(user).build();
}
}
In this code, we return a 404 NOT FOUND
status if the user is not found. This is crucial for letting the client know the outcome of their operation.
Pitfall 2: Overusing HTTP Verbs
Why It Matters
HTTP defines specific methods (verbs) for actions like creating, reading, updating, and deleting resources. Overusing or misusing these verbs can lead to unexpected behavior and poor API design.
Common Mistake
Using POST for all operations, including read operations. This goes against REST principles.
Correct Approach
Each HTTP method should correspond to its intended action. Here is how to correctly use these methods:
@POST
@Path("/users")
@Consumes(MediaType.APPLICATION_JSON)
@Produces(MediaType.APPLICATION_JSON)
public Response createUser(User user) {
// Code to save user
return Response.status(Response.Status.CREATED).entity(user).build();
}
@GET
@Path("/users/{id}")
@Produces(MediaType.APPLICATION_JSON)
public Response readUser(@PathParam("id") String id) {
User user = findUserById(id);
// Rely on the implementation from previous examples for responses
}
By using POST
for creation and GET
for reading, your API adheres to RESTful principles.
Pitfall 3: Neglecting Proper Versioning
Why It Matters
As your API grows and evolves, changes will be necessary. Neglecting API versioning can leave clients with broken functionalities when you introduce breaking changes.
Common Mistake
Not versioning the API. For instance, an endpoint like /api/users
could change its response format without warning.
Correct Approach
Implement versioning as part of your URL. Here's a way to structure your endpoints:
@Path("/v1/users")
public class UserServiceV1 {
// Implementation
}
@Path("/v2/users")
public class UserServiceV2 {
// Implementation with updates
}
This separation allows consumers to continue using the older version until they transition to the new one smoothly. For more on API versioning strategies, refer to this Comprehensive Guide to API Versioning.
Pitfall 4: Ignoring CORS Configuration
Why It Matters
Cross-Origin Resource Sharing (CORS) is crucial when dealing with web applications that interact with RESTful APIs, particularly if they are hosted on different domains.
Common Mistake
Neglecting to configure CORS can result in blocked requests from web applications hosted on different domains.
Correct Approach
Incorporate CORS in your application, usually via configuration. For example, using a filter:
import javax.ws.rs.container.*;
import javax.ws.rs.core.*;
public class CORSFilter implements ContainerResponseFilter {
@Override
public void filter(ContainerRequestContext requestContext, ContainerResponseContext responseContext) throws IOException {
responseContext.getHeaders().add("Access-Control-Allow-Origin", "*");
responseContext.getHeaders().add("Access-Control-Allow-Headers", "origin, content-type, accept, authorization");
responseContext.getHeaders().add("Access-Control-Allow-Credentials", "true");
responseContext.getHeaders().add("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE, OPTIONS, HEAD");
}
}
Proper CORS configuration ensures that your API can be accessed from various domains, enhancing the usability of your web services.
Pitfall 5: Not Applying Proper Security Measures
Why It Matters
Security should never be an afterthought. APIs are gateways into your data and can be vulnerable to attacks if not secured properly.
Common Mistake
Exposing endpoints without authentication or authorization will put your applications at risk.
Correct Approach
Require authentication (e.g., using OAuth2) and implement role-based access control where necessary. Below is a simplistic illustration:
import javax.ws.rs.*;
import javax.ws.rs.core.*;
@Path("/secure/users")
@Consumes(MediaType.APPLICATION_JSON)
@Produces(MediaType.APPLICATION_JSON)
public class SecureUserService {
@GET
@Path("/{id}")
@Secured // Custom annotation for security context
public Response getSecureUser(@PathParam("id") String id) {
// User retrieval logic
}
}
This sample indicates a secured service. Use libraries such as Spring Security or Apache Shiro for better authentication and authorization management.
To Wrap Things Up
Constructing RESTful web services entails meticulous attention to detail. Awareness of common pitfalls—such as neglecting proper HTTP status codes, overusing HTTP verbs, failing to version your API, ignoring CORS configurations, and inadequately securing your service—can significantly enhance the quality, robustness, and security of your APIs.
Every pitfall discussed has potential solutions that can lead to a better development experience and ensure that your RESTful web services are resilient, maintainable, and user-friendly. By adhering to these practices, you'll create APIs that bring value to your users while minimizing frustration and confusion.
If you found this article helpful, consider sharing it with your development peers. For further reading, explore Building RESTful Web Services with Spring Boot.
Happy coding!