Scaling Akka in Java: Overcoming Event Processing Challenges
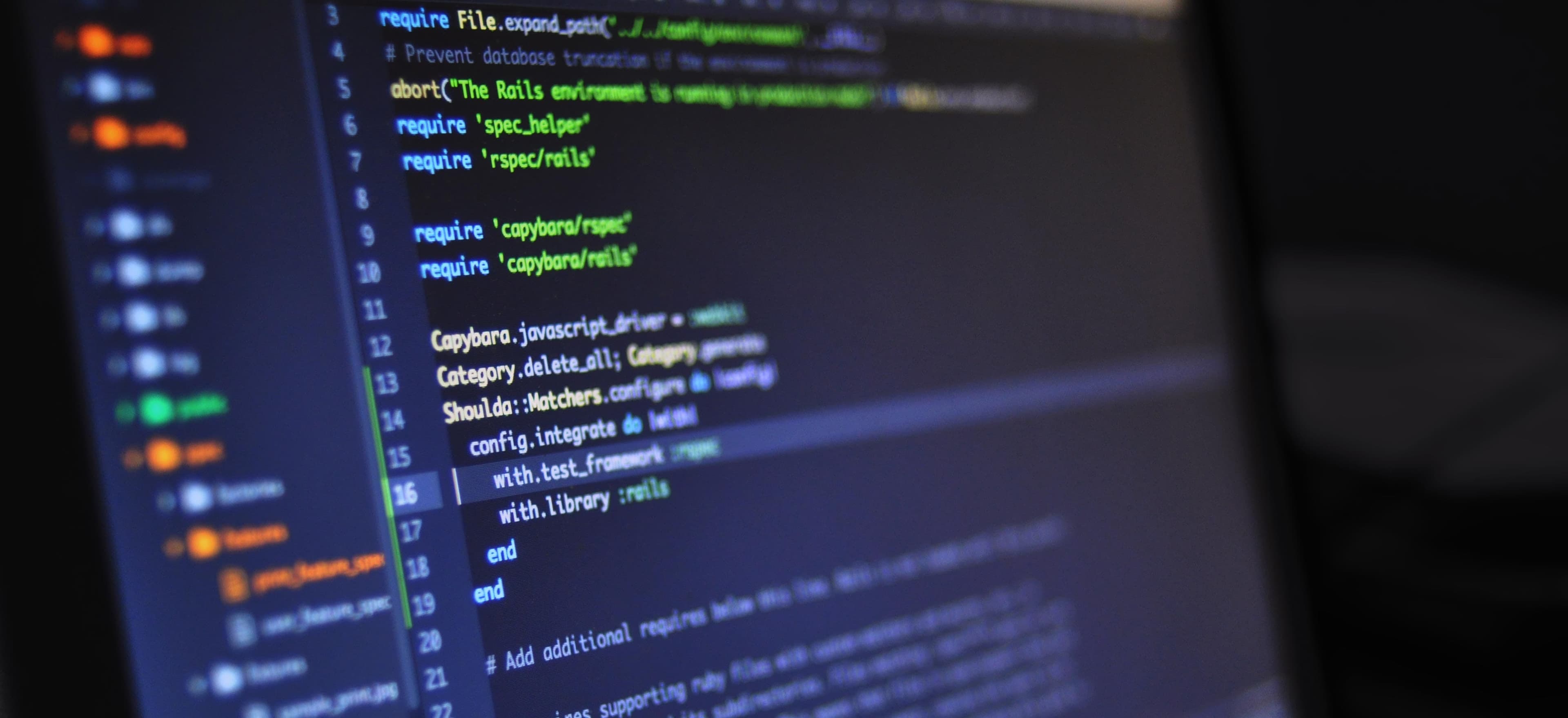
- Published on
Scaling Akka in Java: Overcoming Event Processing Challenges
When it comes to building distributed systems that scale efficiently, Akka is a powerful toolkit for Java developers. Originally developed for Scala, its support for Java continuously improves, enabling Java developers to build highly responsive and resilient applications. In this blog post, we will dive deep into the details of using Akka for event processing, explore common challenges in scaling your application, and provide strategies to overcome them.
What is Akka?
Akka is a framework that simplifies the construction of concurrent applications. It enables the development of message-driven, reactive applications by using the Actor model. In the Actor model, actors are lightweight units of computation that communicate through asynchronous messages. This model allows developers to easily manage concurrency without explicitly dealing with threads.
For a more extensive introduction, check out the Akka documentation.
Why Use Akka for Event Processing?
Event-driven architectures are crucial for modern applications, especially those that require real-time data processing. Here are a few reasons to choose Akka:
- Concurrency: With its actor model, Akka manages thousands of actors concurrently, reducing the complexity and overhead associated with traditional multi-threading.
- Resilience: Akka's built-in supervision strategies enable the system to recover from failures, making it an excellent choice for systems requiring high uptime.
- Scalability: Akka's distributed capabilities allow systems to gracefully scale up or down depending on the load, making it suitable for varying workloads.
Getting Started with Akka in Java
To illustrate how to get started, let’s set up a basic Akka project.
1. Setting Up Your Project
You can use Maven to manage your dependencies. Add the following dependencies to your pom.xml
:
<dependency>
<groupId>com.typesafe.akka</groupId>
<artifactId>akka-actor_2.12</artifactId>
<version>2.6.18</version>
</dependency>
<dependency>
<groupId>com.typesafe.akka</groupId>
<artifactId>akka-stream_2.12</artifactId>
<version>2.6.18</version>
</dependency>
2. Creating an Actor
Let’s create a simple actor that processes events:
import akka.actor.AbstractActor;
import akka.actor.Props;
public class EventProcessor extends AbstractActor {
public static Props props() {
return Props.create(EventProcessor.class);
}
@Override
public Receive createReceive() {
return receiveBuilder()
.match(String.class, this::processEvent)
.build();
}
private void processEvent(String event) {
System.out.println("Processing event: " + event);
// Add your event processing logic here
}
}
What This Code Does
- AbstractActor: This class simplifies the actor creation by providing the basic functionality required to make an actor work.
- Receive: The
createReceive
method defines what message types the actor can handle. - Event Processing Logic: The
processEvent
method will be called when a message of typeString
is received.
Challenges in Scaling Akka Applications
While Akka provides robust capabilities, there are challenges to consider when scaling applications. Here are some common challenges:
1. Managing State
Actors are designed to be stateless; however, often you need to maintain some state across messages. Here's how to handle state:
- Use Persistent Actors to store state across failures. This feature allows you to recover your actor’s state on failure.
Code Example for a Persistent Actor
import akka.persistence.AbstractPersistentActor;
import akka.persistence.SnapshotOffer;
public class PersistentEventProcessor extends AbstractPersistentActor {
private String state = "";
@Override
public String persistenceId() {
return "persistent-event-processor";
}
@Override
public Receive createReceiveRecover() {
return receiveBuilder()
.match(String.class, this::updateState)
.match(SnapshotOffer.class, snapshot -> state = (String) snapshot.snapshot())
.build();
}
@Override
public Receive createReceive() {
return receiveBuilder()
.match(String.class, this::processEvent)
.build();
}
private void processEvent(String event) {
persist(event, this::updateState);
}
private void updateState(String event) {
state += event;
System.out.println("Updated state: " + state);
}
}
What This Code Does
- AbstractPersistentActor: This allows the actor to persist its state.
- persist: The
persist
method is used to store changes to the actor's state, ensuring that it can recover from failures. - SnapshotOffer: A mechanism to restore the actor's state from a snapshot.
2. Message Backpressure and Flow Control
When designing your system, it is crucial to manage how messages flow through actors. High throughput can lead to backpressure. Utilizing Akka Streams can mitigate this.
Code Example for Stream Processing
import akka.stream.Materializer;
import akka.stream.javadsl.Sink;
import akka.stream.javadsl.Source;
public class EventStream {
private final Materializer materializer;
public EventStream(Materializer materializer) {
this.materializer = materializer;
}
public void startProcessing() {
Source<String, NotUsed> source = Source.range(1, 100)
.map(i -> "Event " + i);
source
.buffer(10, OverflowStrategy.backpressure())
.to(Sink.foreach(event -> {
System.out.println("Processing: " + event);
}))
.run(materializer);
}
}
What This Code Does
- Source: Represents a source of elements that will be processed.
- buffer: Implements backpressure by buffering events until the downstream is ready.
- Sink: The destination for the events after processing.
Best Practices to Scale Akka Applications
To optimize your Akka applications, consider the following best practices:
-
Supervision Strategies: Implement custom supervision strategies to define how your system should react to actor failures.
-
Cluster Sharding: Use Akka Cluster Sharding to distribute actors across the cluster based on identifiers.
-
Load Balancing: Distribute event processing evenly to prevent bottlenecks.
-
Monitoring and Logging: Utilize Akka's monitoring capabilities to analyze performance and failures.
Final Thoughts
Scaling Akka in Java involves understanding how to efficiently process events while managing state, concurrency, and performance. By leveraging Akka’s powerful features like persistent actors, stream processing, and proper supervision strategies, your application can achieve remarkable scalability and resilience.
For additional insights and detailed examples, consider visiting the Akka official documentation.
If you have any questions or insights about Akka and event processing in Java, feel free to leave a comment below!
Checkout our other articles