Mastering Cache Synchronization: Common Pitfalls to Avoid
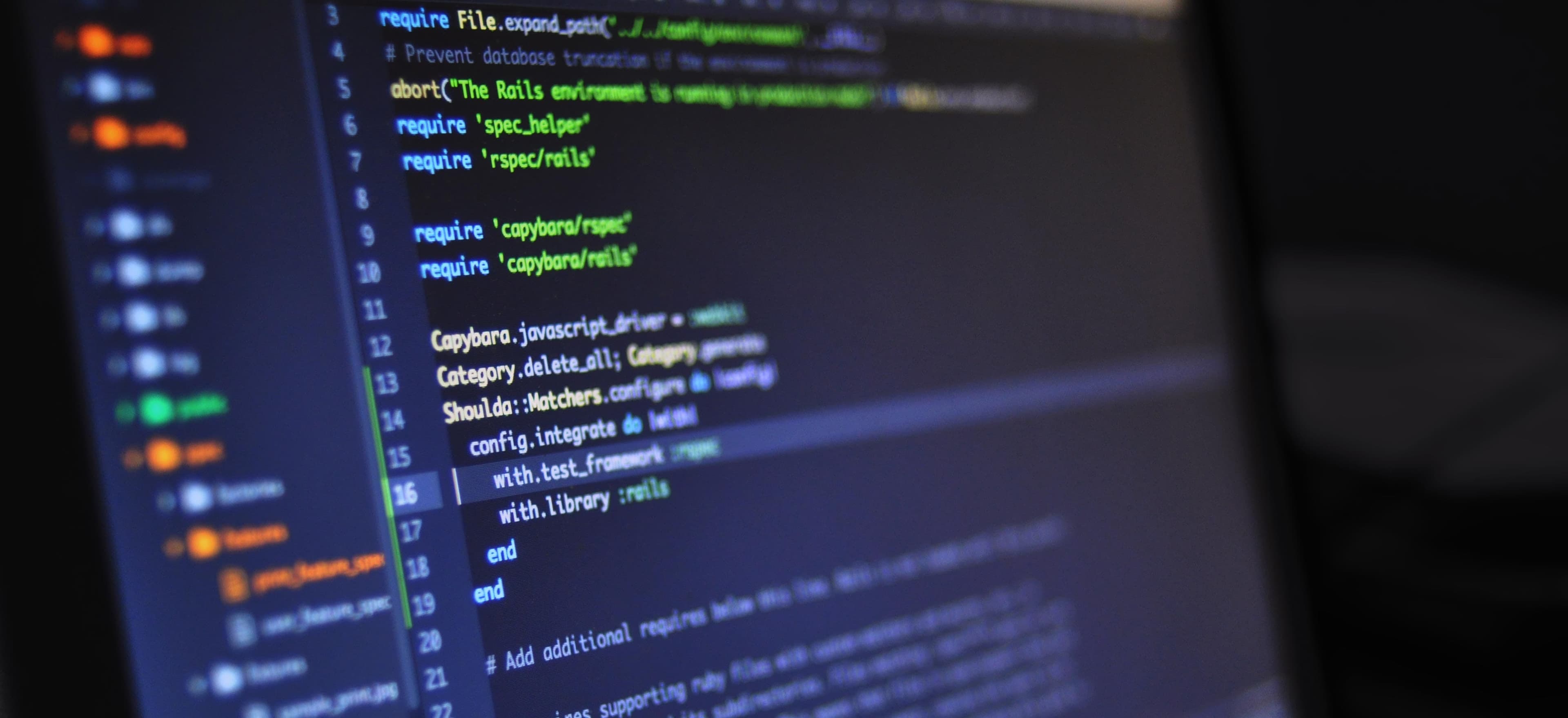
- Published on
Mastering Cache Synchronization: Common Pitfalls to Avoid
Cache synchronization is a crucial aspect of modern application performance. As systems become more distributed, managing how data is cached across multiple servers can significantly impact both efficiency and user experience. Whether you're working with web applications, microservices, or real-time data feeds, understanding the nuances of cache synchronization can make or break your application's performance. In this blog post, we will explore common pitfalls in cache synchronization, particularly in the context of Java applications, and how to avoid them.
What is Cache Synchronization?
Cache synchronization is the process of ensuring that cached data is in line with the source data. In many cases, caching is employed to improve performance by storing frequently accessed data in memory rather than retrieving it from a slower data source like a database. However, the challenge arises when multiple threads or services might alter the source data simultaneously. Thus, failing to synchronize the cache can lead to stale data, inconsistencies, or even application errors.
Common Pitfalls of Cache Synchronization
1. Stale Cache Data
One of the most common pitfalls is stale cache data. When the cached version of an object becomes outdated with respect to the original data source, it can lead to incorrect data being served to users or downstream services.
Example Code
// Assuming a simple User entity
public class User {
private String name;
private String email;
// Getters and Setters...
}
// A simple cache management class
public class UserCache {
private Map<String, User> userCache = new HashMap<>();
public User getUser(String userId) {
return userCache.get(userId); // Potential stale data here
}
public void updateUser(String userId, User user) {
userCache.put(userId, user); // Updates cache but does not check source
}
}
In the above example, if the updateUser
method is called and the data from the database changes elsewhere, a stale user might still linger in the cache.
Solution: Use Cache Expiry
To combat stale data, implement a cache expiry mechanism. This ensures that cached data has a lifespan, after which it must be refreshed. Popular libraries like Ehcache or Caffeine in Java can help with this.
public class UserCache {
private Cache<String, User> userCache = Caffeine.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES) // Sets expiry time
.build();
}
2. Race Conditions
Race conditions occur when multiple threads access shared data concurrently, and the final result depends on the timing of their execution. Without proper synchronization, two threads could read a cache simultaneously, and one might overwrite the changes of another.
Example Code
public void updateUserInCache(String userId, User user) {
userCache.put(userId, user);
// Assume another thread might read here, causing inconsistency
}
Solution: Use Synchronized Blocks or Atomic Data Structures
Using synchronized blocks can help control access to shared resources. However, this might lead to overhead. Java provides atomic structures that can help simplify the task of handling race conditions:
public void updateUserInCache(String userId, User user) {
synchronized (userCache) {
userCache.put(userId, user);
}
}
// Or using an AtomicReference
AtomicReference<User> atomicUser = new AtomicReference<>();
atomicUser.set(user); // thread-safe update
3. Not Invalidating Cache on Updates
When changes are made to the source of truth, it is critical to invalidate the cache. Failing to do so means the cache may serve outdated data regardless of whether the data is accessed simultaneously or not.
Example Code
// Assuming some external process updates the user
public void updateUser(String userId, User updatedUser) {
// Update database and forget to invalidate cache
database.updateUser(userId, updatedUser);
}
Solution: Cache Invalidation
Implement a strategy that invalidates the cache whenever an update is made. You might also consider a pub-sub model where changes trigger notifications to clear relevant cache entries.
public void updateUser(String userId, User updatedUser) {
database.updateUser(userId, updatedUser);
userCache.invalidate(userId); // Ensure cache stays relevant
}
4. Not Using a Distributed Cache
In a microservices architecture, some applications poorly manage cache synchronization due to the absence of a centralized cache approach. Using local caches in each service can become a debilitating flaw.
Solution: Implement Distributed Caching Solutions
Distributed caching solutions like Redis or Apache Ignite can unify cache management. This minimizes the chances of cache inconsistency across different services.
// Example using Redis
Jedis jedis = new Jedis("localhost");
// Set value with expiry
jedis.setex(userId, 3600, user.toString());
5. Ignoring Performance Metrics and Monitoring
Caching strategies should always be backed by solid metrics and monitoring. Not paying attention to performance data can lead to over- or under-caching, impacting application performance.
Solution: Implement Monitoring Tools
Use tools like Java Management Extensions (JMX), Micrometer, or Prometheus to track cache usage, eviction rates, stale data, and other critical metrics. This data will help in fine-tuning your caching strategy.
// Monitor cache hits and misses
private void monitorCacheUsage() {
long hits = cache.getCacheHitCount();
long misses = cache.getCacheMissCount();
System.out.println("Cache Hits: " + hits + ", Cache Misses: " + misses);
}
A Final Look
Cache synchronization is not just a technical hurdle but a design principle that can greatly enhance the performance and reliability of your application. By recognizing common pitfalls like stale data, race conditions, and improper cache management, you can proactively implement solutions to create a robust caching layer.
To further your understanding, consider exploring additional resources: Java Caching and Configuration and Microservices Patterns.
By mastering these strategies, you not only ensure that your data remains consistent across the board, but you also deliver a better user experience and maximize the efficiency of your applications.
Checkout our other articles