Unlocking Java: Synchronizing Non-Final Fields Effectively
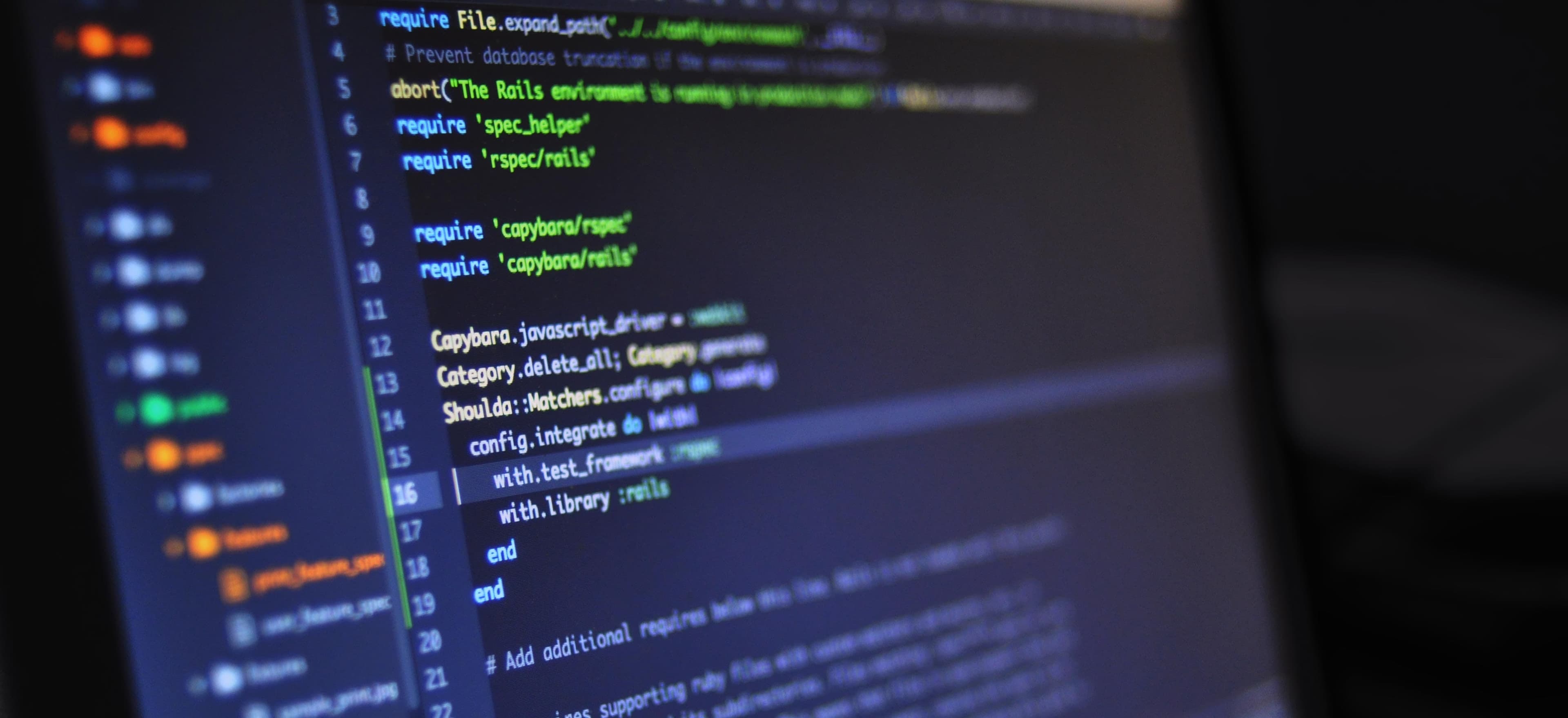
- Published on
Unlocking Java: Synchronizing Non-Final Fields Effectively
Java is a powerful object-oriented programming language widely used for building enterprise applications, web applications, and Android apps. One of the notable features of Java is its concurrency API, enabling developers to write multi-threaded programs. However, with great power comes great responsibility. Ensuring that shared resources are accessed properly in concurrent environments is critical to preventing issues such as race conditions and data inconsistency. This blog post explores synchronizing non-final fields effectively in Java, providing insights through code snippets and discussions.
Understanding Concurrency in Java
Concurrency in Java refers to the ability of multiple threads to execute simultaneously. This enables improved performance and resource utilization but introduces complexity. Whenever multiple threads interact with shared data, you risk encountering concurrency issues. To mitigate those risks, Java provides constructs like synchronized
blocks, ReentrantLock
, and atomic variables.
Why Synchronization Matters
Synchronization is the process of controlling access to shared resources. The following problems can arise without proper synchronization:
- Race Conditions: Occur when multiple threads read and write data at the same time, leading to unpredictable results.
- Data Corruption: If one thread modifies a field while another thread is reading it, the reading thread might not always get the expected value.
- Visibility Issues: Changes made by one thread may not be visible to other threads promptly.
By synchronizing field access, you can ensure that only one thread can access a shared resource at a time, thereby preventing data inconsistencies.
Synchronizing Non-Final Fields
Non-final fields are mutable and can be changed after the object's construction. This flexibility is both a strength and a liability in multi-threaded contexts. Here, we’ll look at various strategies to synchronize non-final fields effectively.
Using the synchronized
Keyword
One of the simplest methods for synchronizing access to mutable fields is using the synchronized
keyword. This ensures that only one thread can execute a block of code at a time.
public class Counter {
private int count = 0;
// Synchronized method to increment count
public synchronized void increment() {
count++;
}
// Synchronized method to get the count
public synchronized int getCount() {
return count;
}
}
In this code snippet:
- The
increment
method is synchronized, which means if one thread is executing it, no other thread can enter this method until it's finished. - The
getCount
method is also synchronized, ensuring a consistent return value.
While this approach is straightforward, it can lead to performance bottlenecks if heavily contended. To solve this, you may consider using finer-grained locking or other concurrency utilities.
Using Locks
Java provides a more sophisticated locking mechanism through the java.util.concurrent.locks
package. For instance, using ReentrantLock
allows more control over synchronization.
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class LockingCounter {
private int count = 0;
private final Lock lock = new ReentrantLock();
public void increment() {
lock.lock(); // Acquire the lock
try {
count++;
} finally {
lock.unlock(); // Ensure the lock is released
}
}
public int getCount() {
lock.lock(); // Acquire the lock
try {
return count;
} finally {
lock.unlock(); // Ensure the lock is released
}
}
}
Why Use ReentrantLock
?
- Flexibility: Reentrant locks support multiple lock acquisition and allow threads to interrupt waiting threads.
- Fairness: You can create fair locks to prevent thread starvation by granting access to the longest-waiting thread first.
- Extensibility: You can extend
ReentrantLock
for more complex scenarios.
Atomic Variables
If you only need to perform simple operations on non-final fields, the java.util.concurrent.atomic
package can simplify your efforts without traditional locking.
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicCounter {
private AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet();
}
public int getCount() {
return count.get();
}
}
Benefits of Atomic Variables:
- Performance: They are generally more efficient than locks since they use optimized machine-level instructions.
- Simplicity: Code is cleaner, and you avoid potential deadlocks associated with traditional locking.
Best Practices for Synchronizing Non-Final Fields
- Minimize Scope: Keep synchronized code blocks as small as possible to reduce contention.
- Avoid Nested Locks: This can lead to deadlocks. Always try to acquire locks in a consistent order.
- Use Immutable Objects: Whenever possible, favor immutability to avoid synchronization altogether. Final fields can help since they cannot be changed once they are set.
- Measure Performance: Before and after applying synchronization strategies, profiling the application can help identify bottlenecks.
Wrapping Up
Synchronizing non-final fields in Java is essential for maintaining consistency and integrity in multi-threaded applications. As we've explored through code snippets and discussions, various approaches like synchronized
methods, ReentrantLock
, and atomic variables provide developers with the tools needed for effective synchronization.
Careful consideration should be given to the method of synchronization chosen; each has its pros and cons. Choose the right synchronization method based on performance requirements and use cases. For further reading on this subject, you might explore the Java Concurrency in Practice book, which offers valuable insights.
Now, it's your turn! How do you handle synchronization in your Java applications? Share your thoughts in the comments below!