Common Pitfalls in Java Method Overloading Explained
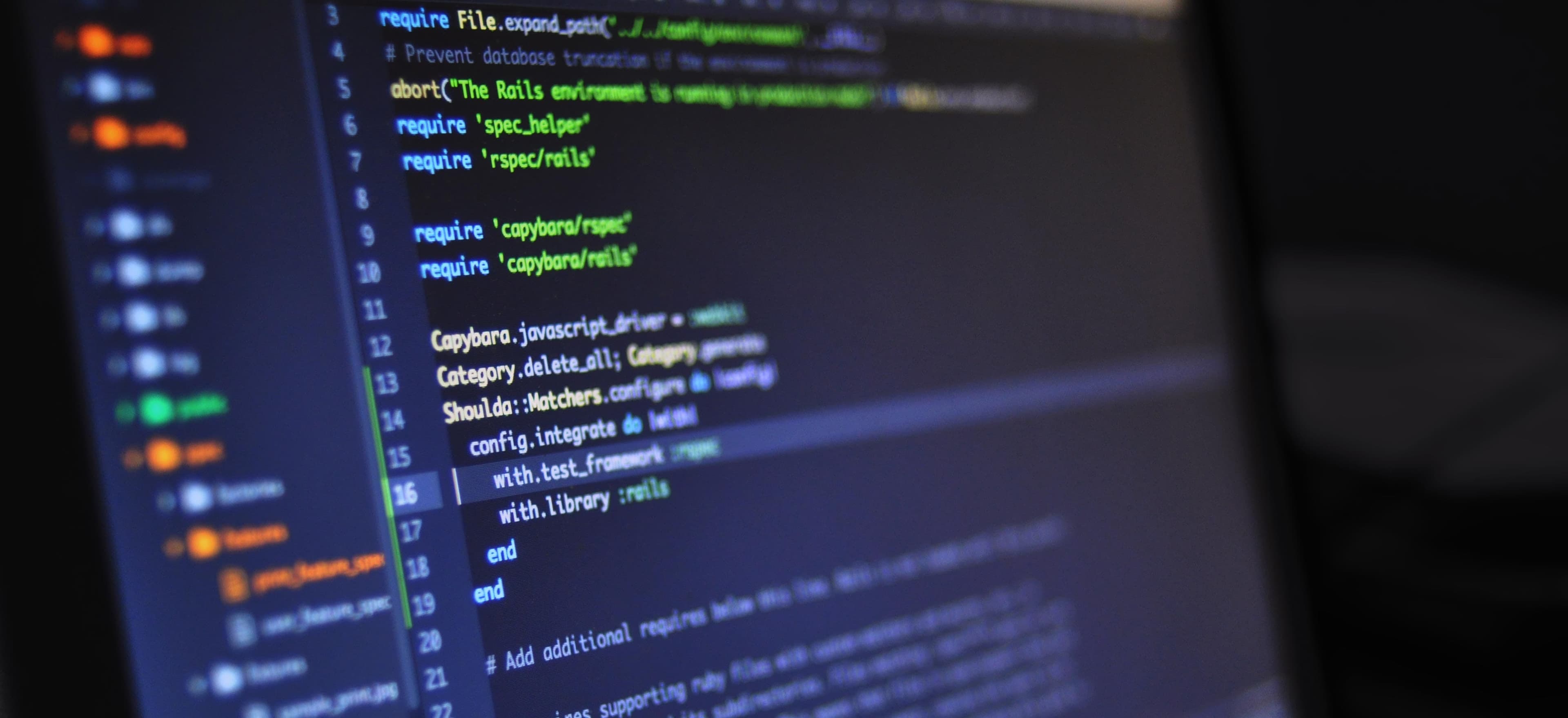
- Published on
Common Pitfalls in Java Method Overloading Explained
Method overloading is one of the fundamental features of Java that allows developers to define multiple methods with the same name but different parameter lists. This can enhance code readability and usability, yet it can also introduce confusion if not implemented carefully. Understanding common pitfalls in Java method overloading is crucial for writing clear and maintainable code. In this post, we'll explore these pitfalls, provide exemplary code snippets, and discuss ways to avoid them.
What is Method Overloading?
Before diving into common pitfalls, let's briefly define method overloading. In Java, a method is overloaded when two or more methods in the same class share the same name but differ in:
- Number of parameters
- Type of parameters
- Order of parameters
Example of Method Overloading
Here's a simple example to illustrate method overloading:
public class Calculator {
// Method to add two integers
public int add(int a, int b) {
return a + b;
}
// Method to add three integers
public int add(int a, int b, int c) {
return a + b + c;
}
// Method to add two doubles
public double add(double a, double b) {
return a + b;
}
}
In this example, the add
method is overloaded three different ways: by the number and type of parameters. This allows users to call the method with different arguments, depending on their needs.
Common Pitfalls in Method Overloading
While method overloading is beneficial, developers can encounter several common pitfalls. Let’s discuss each of these in detail.
1. Ambiguity with Parameter Types
One of the significant pitfalls occurs when overloaded methods have parameters that can be easily confused, leading to ambiguity. Java may not always be able to determine which method to invoke.
Example of Ambiguity
public class Ambiguous {
public void display(int number) {
System.out.println("Integer: " + number);
}
public void display(double number) {
System.out.println("Double: " + number);
}
}
// In main method
Ambiguous amb = new Ambiguous();
amb.display(10); // Ambiguous call
In the above example, you might expect this to call the display(int number)
method. However, if you were to call display(10.0)
, it would behave differently based on the context. Java considers this an ambiguous call, which leads to a compilation error.
Solution
To avoid ambiguity, ensure that the parameter types are distinct enough. If necessary, you can redefine method signatures to make it clear.
public void display(int number) {...}
public void display(float number) {...} // Use float instead of double
2. Varargs and Overloading Confusion
Using varargs (variable-length arguments) in conjunction with overloaded methods can introduce complexity and confusion.
Example of Varargs Issue
public class VarargsExample {
public void showNumbers(int... numbers) {
System.out.println("Varargs: " + Arrays.toString(numbers));
}
public void showNumbers(int number) {
System.out.println("Single: " + number);
}
}
// In main method
VarargsExample vExample = new VarargsExample();
vExample.showNumbers(10); // Calls the single parameter method
vExample.showNumbers(1, 2, 3); // Calls the varargs method
In the above case, when you call showNumbers(10)
, you might predict it will call the single parameter method, which it does. But if you call showNumbers()
with multiple parameters, it will still correctly call the varargs method.
Solution
Be cautious with the order of overloaded methods. Place more specific methods before more general ones (like varargs).
3. Mixed Parameter Orders
Another pitfall arises when methods are overloaded with the same types but in different orders. This can yield confusing results, especially when using automatic type conversion.
Example of Mixed Parameter Issues
public class MixedParameters {
public void process(String text, int number) {
System.out.println("Text: " + text + ", Number: " + number);
}
public void process(int number, String text) {
System.out.println("Number: " + number + ", Text: " + text);
}
}
// In main method
MixedParameters mp = new MixedParameters();
mp.process(5, "Hello"); // Calls the second method
Here, because both process
methods contain one int
and one String
, the order becomes crucial. If a user calls mp.process(5, "Hello")
, they are calling the second method.
Solution
To avoid confusion, ensure method names and signatures clearly express their purpose. When feasible, stick to a consistent order for parameters across methods.
4. Shadowing Inherited Methods
When you override a method in a subclass, it can lead to confusion with overloaded methods defined in the parent class.
Example of Shadowing
class Parent {
public void show(int number) {
System.out.println("Parent: " + number);
}
}
class Child extends Parent {
public void show(double number) {
System.out.println("Child: " + number);
}
}
// In main method
Child child = new Child();
child.show(5); // Calls Parent's method, not Child's
In this scenario, calling child.show(5)
runs the show(int number)
method from the Parent
class instead of the Child
class.
Solution
Clearly differentiate between overloaded and overridden methods. Prefix new methods with a different name for increased clarity.
5. Overloading Based on Return Type Only
Java does not support method overloading based solely on differing return types. This leads to compile-time errors or unintended behavior.
Example of Incorrect Overloading
public class ReturnTypeOverload {
public int process() {
return 1;
}
public double process() { // Compilation error
return 1.0;
}
}
Here, attempting to overload process()
based solely on return type will result in a compilation error.
Solution
Always include parameters when overloading methods. This avoids confusion and complies with Java's rules on method overloading.
Closing Remarks
Method overloading enhances Java functionality and maintainability when used correctly. However, it’s crucial to be aware of common pitfalls like ambiguity with parameter types, mixed parameter orders, and the impact of varargs.
By following the guidelines discussed in this article, you can prevent confusion and write cleaner, more understandable code. For further reading on Java method overloading and best practices, refer to the Java documentation here.
Start implementing these best practices today to enhance your Java programming experience! Happy coding!
Checkout our other articles