Enhancing Performance: Java Agent Bytecode Instrumentation Issues
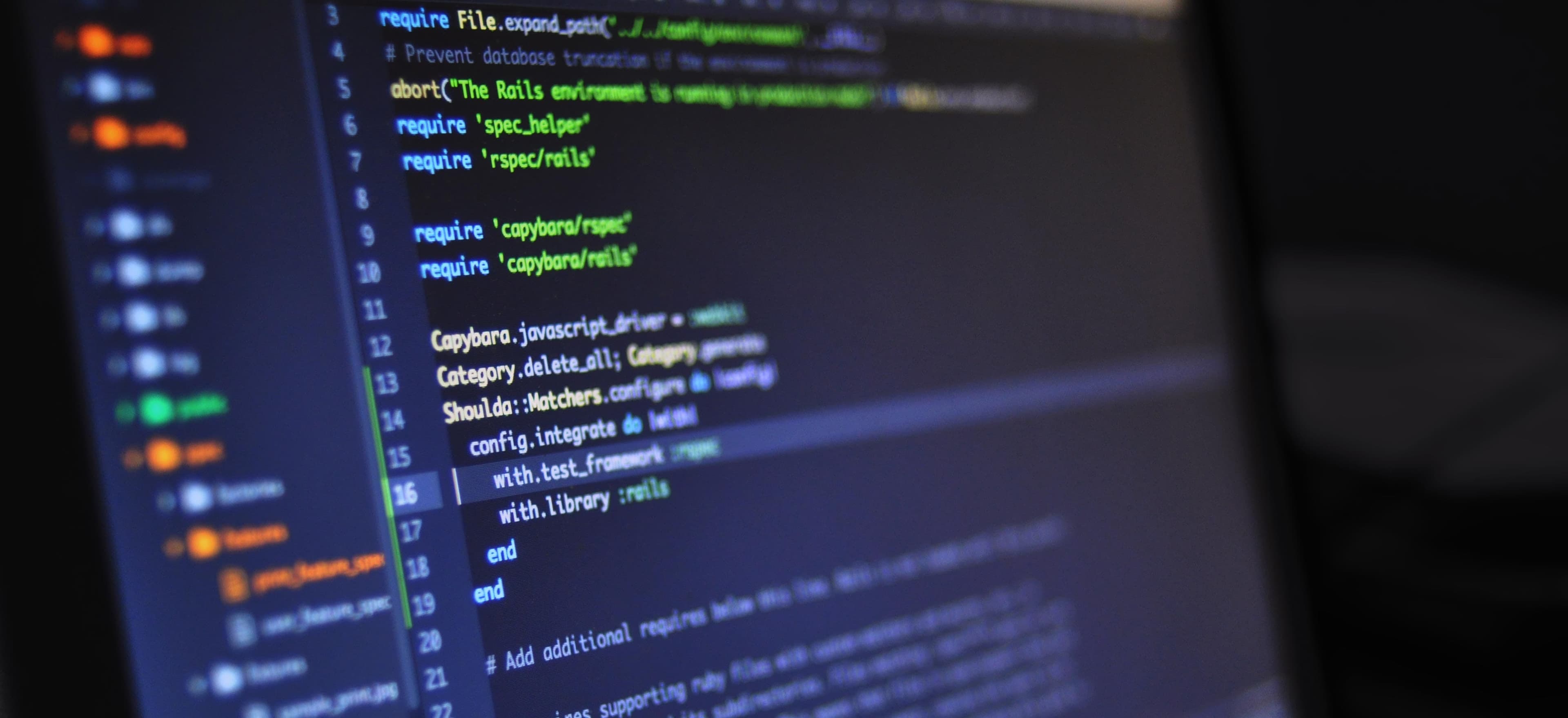
- Published on
Enhancing Performance: Java Agent Bytecode Instrumentation Issues
Java is a widely used programming language that thrives on its ability to run efficiently on multiple platforms. One of the lesser-known yet powerful features of Java is bytecode instrumentation, which allows developers to modify Java bytecode at runtime. However, while it holds the potential for significant performance enhancements, it also presents a set of intricacies that must be navigated carefully.
In this post, we will delve into Java agent bytecode instrumentation, examining its benefits, the challenges developers face, and the best practices to mitigate those issues.
What is Bytecode Instrumentation?
Bytecode instrumentation refers to the process of modifying the compiled Java bytecode. Essentially, this means altering the .class files that the Java Virtual Machine (JVM) executes. In Java, this can be accomplished using a Java agent, which is a special type of program that can manipulate the bytecode as it is loaded into the JVM.
Why Use Bytecode Instrumentation?
-
Performance Monitoring: Instrumentation allows for the collection of runtime metrics, which helps in profiling applications. This can lead to insights into memory management, CPU usage, and method invocation times.
-
Dynamic Analysis: You can dynamically observe how your application behaves in production, making it easier to identify bottlenecks and resource leaks.
-
Adding Behavior: Developers can inject additional behavior into classes without modifying their source code, which can be particularly useful for cross-cutting concerns like logging and security.
Common Libraries for Bytecode Instrumentation
1. ASM
ASM is a Java bytecode manipulation framework that allows you to read, write, and transform bytecode. Its lightweight design makes it a popular choice for performance-sensitive applications.
Example Code Snippet Using ASM
Below is an example of how to insert a logging statement into an existing method using ASM.
import org.objectweb.asm.*;
public class MethodLogger extends ClassVisitor {
public MethodLogger() {
super(Opcodes.ASM9);
}
@Override
public MethodVisitor visitMethod(int access, String name, String descriptor, String signature, String[] exceptions) {
MethodVisitor mv = super.visitMethod(access, name, descriptor, signature, exceptions);
if (name.equals("targetMethod")) {
mv = new MethodVisitor(Opcodes.ASM9, mv) {
@Override
public void visitCode() {
mv.visitLdcInsn("Entering targetMethod");
mv.visitMethodInsn(Opcodes.INVOKESTATIC, "System", "out", "println(Ljava/lang/String;)V", false);
super.visitCode();
}
};
}
return mv;
}
}
In this snippet, we are using ASM to introduce a logging statement whenever the targetMethod
is invoked. This approach maintains performance since a simple logging mechanism usually has a minimal impact on execution time.
Challenges of Bytecode Instrumentation
1. Complexity of Code
While bytecode manipulation can offer significant benefits, the complexity of handling and inserting code can lead to maintenance headaches. Developers who are not familiar with the bytecode structure may inadvertently introduce bugs or performance regressions.
2. Performance Overhead
Instrumenting code can add overhead. Depending on how instrumentation is applied, performance can degrade due to added method calls or increased execution time.
3. Ecosystem Compatibility
Different Java libraries and frameworks may have varying bytecode manipulation implementations. For instance, Spring AOP uses a proxy mechanism that can conflict with direct bytecode manipulation, causing confusion and unexpected behavior.
Best Practices for Effective Bytecode Instrumentation
1. Limit Scope of Instrumentation
Keep the scope of your instrumentation limited. Focus on specific methods that have the most significant impact on performance. This reduces overhead and maintains the clarity of your codebase.
2. Measure Impact
Always measure the performance impact of your instrumentation. Use profiling tools like Java VisualVM or Java Mission Control to gather metrics before and after implementing instrumentation.
3. Maintain Readability
Code readability should not be compromised for performance. Commenting on the reasons for instrumentation will help future maintainers understand why certain changes were made.
4. Use Established Libraries
Leverage established libraries like ASM and Byte Buddy instead of implementing your bytecode manipulation from scratch. Not only do these libraries offer a wealth of functionality, but they are also actively maintained to handle various edge cases.
My Closing Thoughts on the Matter
Java agent bytecode instrumentation can be a powerful ally in enhancing the performance of Java applications. When wielded skillfully, it enables developers to gather crucial insights into application dynamics while allowing them to introduce new behaviors seamlessly.
However, the challenges accompanying bytecode modification cannot be understated. The complexity, potential for performance overhead, and the intricacies of the Java ecosystem mandate vigilance and best practices.
As you explore the world of bytecode instrumentation, remember the key is to find the right balance between performance enhancement and code maintainability. By choosing established tools, limiting the scope of instrumentation, and carefully measuring the impact, you can leverage Java’s capabilities while avoiding common pitfalls.
Be sure to stay updated with the latest in Java instrumentation techniques and practices as both the Java community and technology continue to evolve. Happy coding!
For further reading, you might find the following resources helpful:
By learning and applying these concepts, you will empower yourself to enhance your Java applications more dynamically and efficiently.
Checkout our other articles