Preventing Bugs: Immutability in Java Multithreading
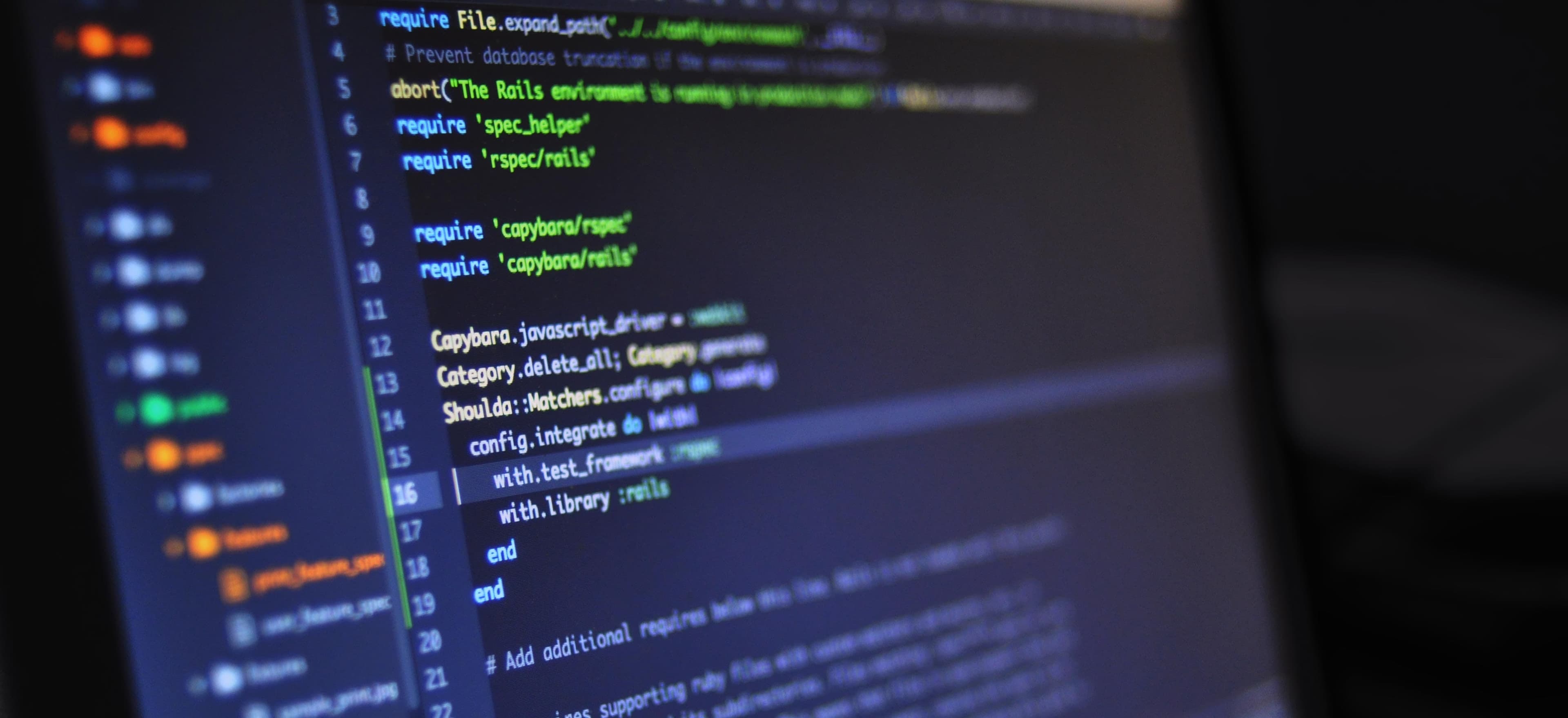
- Published on
Preventing Bugs: Immutability in Java Multithreading
In the realm of Java programming, particularly when dealing with multithreading, one common source of bugs arises from shared mutable state. When multiple threads interact with the same variables, unexpected results can occur. This is where immutability comes into play as a powerful concept to ensure data consistency and prevent bugs. In this blog post, we’ll explore the importance of immutability in Java multithreading, how to implement it, and its benefits.
What is Immutability?
Immutability refers to the property of an object whose state cannot be modified after it is created. In Java, an object is considered immutable if its fields are fixed once assigned. Any alteration leads to the creation of a new instance rather than changing the existing one.
Example of an Immutable Class
Here’s a straightforward example of an immutable class in Java:
public final class ImmutablePoint {
private final int x;
private final int y;
public ImmutablePoint(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public ImmutablePoint move(int deltaX, int deltaY) {
return new ImmutablePoint(this.x + deltaX, this.y + deltaY);
}
}
Explanation:
-
Final Class: We declare the class as
final
to prevent subclassing. Subclassing can lead to mutable behavior which defeats the purpose of immutability. -
Final Fields: The fields
x
andy
are declared asfinal
so that they cannot be reassigned after construction. -
Constructor: The values of
x
andy
are assigned in the constructor and cannot be altered afterwards. -
Creating New Instances: The
move
method demonstrates how to create a new instance rather than modifying the current one. This method returns a newImmutablePoint
, thus maintaining immutability.
Why Use Immutability in Multithreading?
When you utilize immutable objects in a multithreaded environment, you largely eliminate the risks associated with shared mutable state. Here are some reasons supporting immutability in Java multithreading:
1. Thread Safety
Immutable objects are inherently thread-safe. Since their state cannot change, you don’t have to worry about one thread modifying an object while another is reading it. This reduces the complexity of managing synchronization in your code.
2. Easier to Understand
Immutability leads to more predictable code. When developers know that an object cannot change, reasoning about code becomes simpler. You can rely on the fact that once an object is created, it will remain the same throughout its lifecycle.
3. Reduced Bugs
Immutable objects reduce the likelihood of introduced bugs. Concurrency issues, such as race conditions, are significantly minimized. This allows programmers to focus on functionality without getting bogged down in thread conflicts.
To read more on common multithreading issues and their fixes, refer to the Oracle Java Tutorials on Concurrency.
Working with Immutable Collections
Java provides collections that can be made immutable, ensuring that the data within the collections cannot be modified after creation. The Collections framework introduced in Java 9 added methods to create immutable lists, sets, and maps.
Example of an Immutable List:
List<String> items = List.of("Apple", "Banana", "Cherry");
Explanation:
-
List.of: The
List.of
method creates an immutable list. Any attempt to modify this list (e.g., adding or removing items) will throw anUnsupportedOperationException
. -
Concurrency-Friendly: Since
items
cannot change, it provides a thread-safe mechanism to share data across multiple threads.
Using the Builder Pattern for Immutable Objects
While immutability can make the construction of objects somewhat restrictive, using the Builder Pattern can offer flexibility. Here’s how you can leverage the Builder Pattern to create immutable objects.
Example of an Immutable Class with Builder Pattern
public final class Person {
private final String name;
private final int age;
private Person(Builder builder) {
this.name = builder.name;
this.age = builder.age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public static class Builder {
private String name;
private int age;
public Builder withName(String name) {
this.name = name;
return this;
}
public Builder withAge(int age) {
this.age = age;
return this;
}
public Person build() {
return new Person(this);
}
}
}
Explanation:
-
Private Constructor: The constructor for
Person
is private. This prevents direct instantiation and ensures that the builder is the only way to createPerson
objects. -
Builder Inner Class: The
Builder
inner class contains methods to set each property. It returns the builder itself after setting a property, allowing method chaining. -
Create Instances: The
build
method constructs an instance ofPerson
, ensuring that any createdPerson
object is immutable.
Usage Example:
Person person = new Person.Builder()
.withName("Alice")
.withAge(30)
.build();
To Wrap Things Up
Immutability is a cornerstone of safe multithreading in Java. By using immutable objects, you can enhance thread safety, simplify your code, and significantly reduce bugs. It makes your applications not only more robust but also easier to understand and maintain.
Java ecosystems continue to evolve, providing tools and libraries that embrace immutability, including the Java Standard Library and various third-party libraries. Familiarizing yourself with immutable concepts can yield immediate benefits in concurrent programming scenarios.
By incorporating immutability into your Java applications, particularly in multithreaded environments, you appreciate greater stability and reliability in your software solutions.
For further reading, check out the following resources:
- Effective Java by Joshua Bloch
- Java Concurrency in Practice by Brian Goetz
- Oracle's Java Documentation
Happy coding!