Mastering Java Reflection: Overcoming Access Control Pitfalls
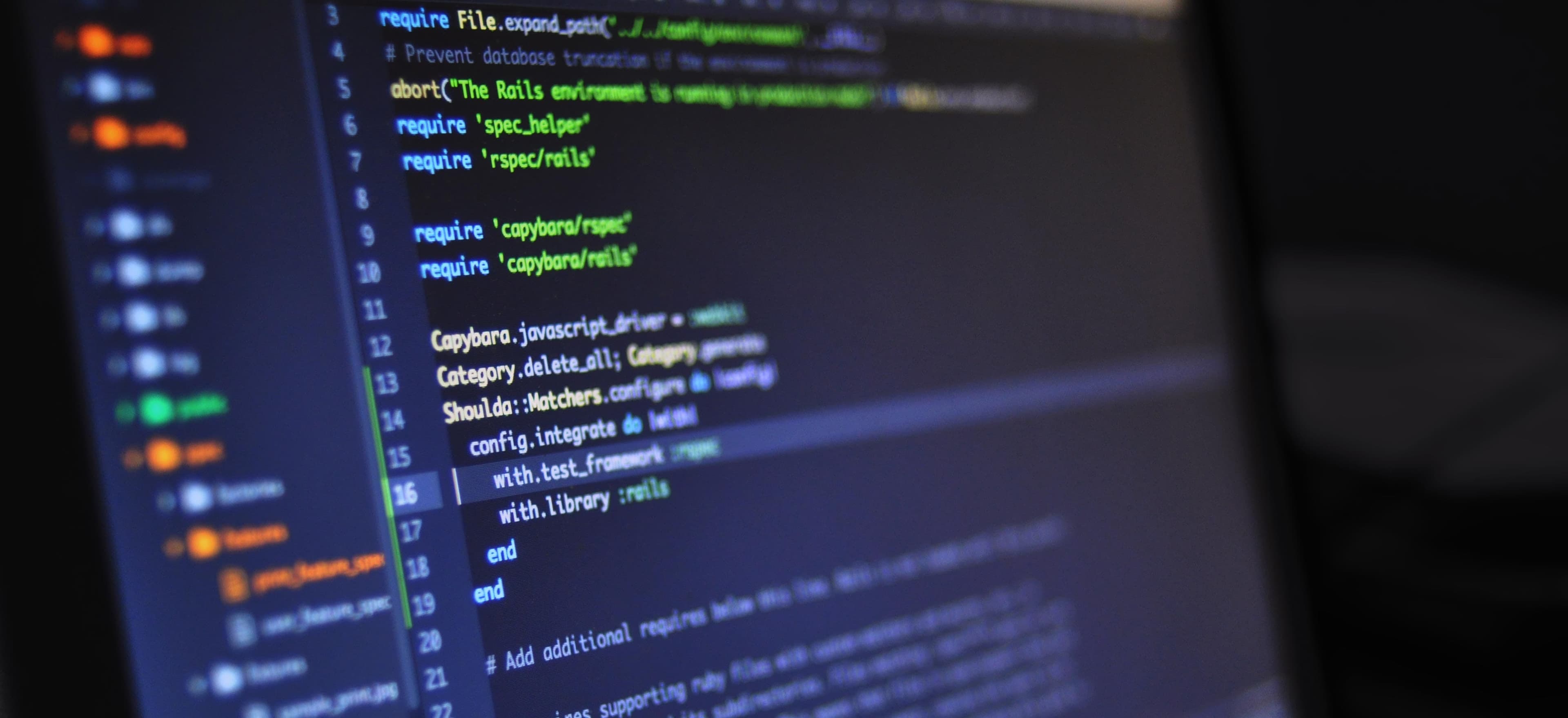
- Published on
Mastering Java Reflection: Overcoming Access Control Pitfalls
Java reflection is a powerful feature that allows developers to inspect and manipulate the properties of classes, methods, and fields at runtime. While it provides great flexibility, it also comes with its own set of challenges, particularly regarding access control. In this blog post, we will delve into the intricacies of Java reflection, discuss access control, and present solutions to overcome common pitfalls.
Table of Contents
- Understanding Java Reflection
- Access Control in Java
- Using Reflection to Access Private Members
- Overcoming Access Control Pitfalls
- Best Practices for Using Reflection
- Conclusion
Understanding Java Reflection
Reflection is a feature in Java that allows the program to examine or manipulate the underlying properties of classes and objects. Java provides classes within the java.lang.reflect
package to facilitate this functionality.
Here is a simple example of how to utilize reflection:
import java.lang.reflect.Method;
public class ReflectionExample {
public static void main(String[] args) {
try {
Class<?> cls = Class.forName("java.lang.String");
Method[] methods = cls.getDeclaredMethods();
for (Method method : methods) {
System.out.println("Method: " + method.getName());
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
In this example, we load the String
class and print out all its declared methods. The Class.forName
method dynamically loads the specified class, while getDeclaredMethods
retrieves all methods in that class.
Access Control in Java
Java enforces access control rules to protect the integrity of data and prevent unauthorized access to class members. There are four access modifiers in Java:
- Public: Accessible from any other class.
- Protected: Accessible within its own package and by subclasses.
- Default (package-private): Accessible only within its own package.
- Private: Accessible only within its own class.
These modifiers ensure that sensitive data members are well-guarded. However, reflection can bypass these rules, which raises ethical considerations on when and how to use it.
Using Reflection to Access Private Members
One common use case of reflection is accessing private variables or methods from outside their declared scope. Let's look at how to do this:
import java.lang.reflect.Field;
class PrivateFieldExample {
private String secret = "Top Secret";
public String getSecret() {
return secret;
}
}
public class AccessPrivateField {
public static void main(String[] args) {
try {
PrivateFieldExample example = new PrivateFieldExample();
Field field = example.getClass().getDeclaredField("secret");
field.setAccessible(true); // Bypass the access control
String secretValue = (String) field.get(example);
System.out.println("Accessed secret: " + secretValue);
} catch (NoSuchFieldException | IllegalAccessException e) {
e.printStackTrace();
}
}
}
In this example, we first get the private field secret
using getDeclaredField
. The crucial part is calling setAccessible(true)
, which allows us to bypass Java's access control checks. Finally, we retrieve the value of the private field.
Overcoming Access Control Pitfalls
Utilizing reflection comes with risks, especially concerning access control. The following best practices can help mitigate those risks:
1. Limit the Scope of Reflection
Only use reflection when absolutely necessary. It can lead to maintenance challenges, as the reliance on the specific structure of a class may break if the implementation changes.
2. Understand Security Risks
Exploiting reflection may expose sensitive data or methods. Be aware of the potential vulnerabilities it introduces and avoid using it in sensitive areas of your application.
3. Use Annotations
Consider using annotations to mark certain fields for reflection rather than relying on access modifiers. This way, you can only access specific fields without exposing all information unnecessarily.
import java.lang.reflect.Field;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
@interface ReflectiveAccess {
}
class AnnotatedClass {
@ReflectiveAccess
private String value = "Accessible via reflection";
}
public class TestReflectionWithAnnotations {
public static void main(String[] args) {
try {
AnnotatedClass instance = new AnnotatedClass();
for (Field field : instance.getClass().getDeclaredFields()) {
if (field.isAnnotationPresent(ReflectiveAccess.class)) {
field.setAccessible(true);
System.out.println("Annotated value: " + field.get(instance));
}
}
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
}
4. Use Reflection Libraries
There are libraries like Apache Commons Lang or Google Guava that offer reflection capabilities with additional safety nets and functionalities. They abstract away many complexities, making the code cleaner and easier to manage.
5. Regular Code Reviews
Regular reviews help ensure reflection is used appropriately and not excessively within codebases. It fosters a culture of accountability and proper design patterns.
Best Practices for Using Reflection
Even when necessary, it's crucial to follow best practices to maintain code quality:
- Performance Overhead: Reflection incurs performance overhead. Use it judiciously, particularly in performance-sensitive areas.
- Error Handling: Always include error handling to manage potential exceptions arising from reflection operations.
- Documentation: Comment on the purpose and usage of reflection in your code, as it can be unclear to future maintainers.
- Limitations: Be aware of the limitations of reflection, such as type erasure, which impacts generics.
Final Thoughts
Java reflection is a double-edged sword; it offers powerful capabilities at the cost of type safety and access control integrity. By understanding the underlying principles and following best practices, you can master its use effectively while mitigating potential risks. Use it wisely, and you can unlock a world of flexibility within your Java applications.
For more about advanced features of Java, check out Java Reflection Essentials and Java Reflection and Its Pitfalls. Happy coding!
Checkout our other articles