Maximizing Efficiency: Avoiding Thread Pool Exhaustion
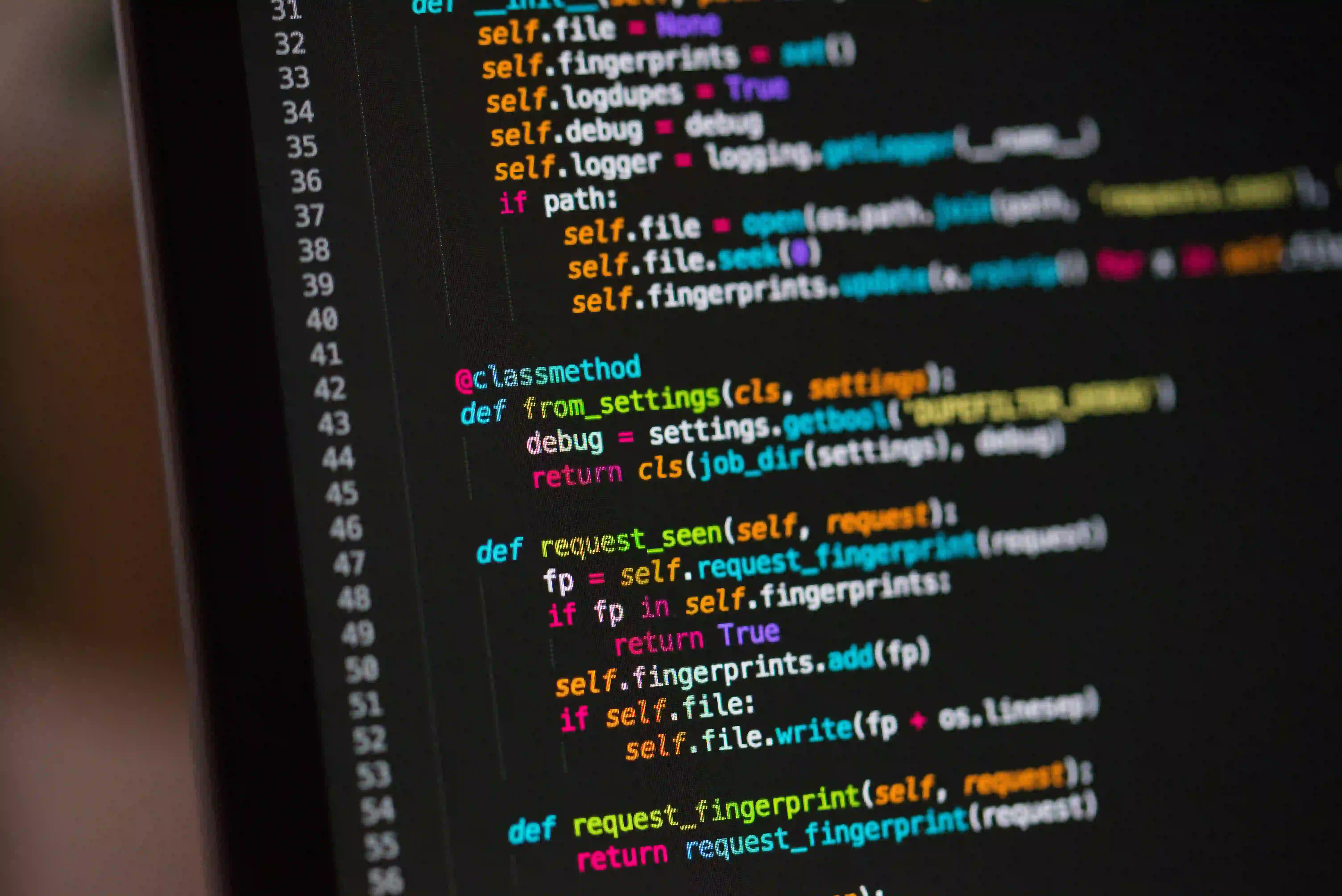
Maximizing Efficiency: Avoiding Thread Pool Exhaustion in Java
In the world of concurrent programming, threading is a powerful tool. However, with great power comes great responsibility. One of the most common pitfalls in multithreaded applications is thread pool exhaustion, which can lead to performance bottlenecks and system instability. This blog post delves into the concept of thread pools in Java, the implications of exhaustion, and best practices for avoiding it.
Understanding Thread Pools
In Java, a thread pool is a collection of worker threads that efficiently manage the task of executing asynchronous tasks. Through the use of thread pools, you can reduce the overhead associated with thread creation, minimize resource consumption, and improve overall application responsiveness.
The Java Executor
framework provides a robust API, making it easy to create and manage thread pools. Here’s a simple example:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
public static void main(String[] args) {
ExecutorService executorService = Executors.newFixedThreadPool(4);
for (int i = 0; i < 10; i++) {
final int taskId = i;
executorService.submit(() -> {
System.out.println("Executing task " + taskId);
// Simulate some work with sleep
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
}
executorService.shutdown();
}
}
Why Use ExecutorService?
The ExecutorService
takes care of the threading mechanics for you, allowing you to focus on the core logic of your application. By specifying the pool size with newFixedThreadPool
, we tell the executor that it can use up to four threads concurrently.
The Problem: Thread Pool Exhaustion
Thread pool exhaustion occurs when all the threads in the pool are busy executing tasks, and new tasks cannot be submitted until existing ones complete. This can lead to delays, performance degradation, or even failure if the application cannot process requests in a timely manner.
Consequences of Exhaustion:
- Task Queue Backlog: Incoming tasks are queued, causing increased waiting times.
- Increased Latency: Response times may significantly increase, negatively impacting user experience.
- Resource Wastage: System resources are tied up waiting on threads, leading to inefficient usage.
Signs of Thread Pool Exhaustion
Detecting thread pool exhaustion early is crucial for diagnosing performance issues. Here are a few signs to look out for:
- Slow Application Response: A noticeable lag in application responsiveness.
- High Queue Sizes: Unusually large task queues can indicate that tasks are piling up faster than they are being processed.
- Increased CPU Utilization: Periods of high CPU usage may be indicative of a stalled thread pool.
Best Practices to Avoid Thread Pool Exhaustion
1. Configure the Thread Pool Size Appropriately
Choosing the right size for your thread pool is paramount. A thread pool that is too small can lead to increased queuing, while one that is excessively large can lead to context-switching overhead.
A good starting point for calculating the optimal pool size is:
idealPoolSize = availableProcessors * (1 + averageWaitTime / averageExecutionTime)
Where:
availableProcessors
is the number of CPU cores.averageWaitTime
is the time tasks typically wait to execute.averageExecutionTime
is how long tasks take to complete.
2. Monitor and Tune System Resources
Use monitoring tools like Java VisualVM or Prometheus to track thread utilization and performance metrics. Monitoring allows you to understand task execution and adjust the thread pool size dynamically.
Example of tuning parameters for a ThreadPoolExecutor
:
import java.util.concurrent.LinkedBlockingQueue;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
ThreadPoolExecutor executor = new ThreadPoolExecutor(
4, // core thread pool size
8, // maximum thread pool size
60, // time to wait before resizing
TimeUnit.SECONDS,
new LinkedBlockingQueue<>()
);
3. Use an Appropriate Blocking Queue
Blocking queues manage runnable tasks until they can be executed. Different types of queues impact behavior during contention and workloads.
Example: Using a Synchronous Queue
import java.util.concurrent.Executors;
import java.util.concurrent.Executors;
ExecutorService executor = Executors.newCachedThreadPool();
- SynchronousQueue: If you want to reject tasks when all threads are busy, use a
SynchronousQueue
. It doesn't hold tasks for future execution. Instead, tasks are handed off directly to threads.
4. Implement a Rejection Policy
When a thread pool cannot accept new tasks, it can choose to reject tasks. Java's ThreadPoolExecutor
provides several built-in rejection policies that define how tasks should be handled when the pool is full.
executor.setRejectedExecutionHandler(new ThreadPoolExecutor.AbortPolicy());
Rejection Policies:
- AbortPolicy: Throws a
RejectedExecutionException
. - CallerRunsPolicy: Executes the task on the caller's thread if the pool is saturated.
- DiscardPolicy: Discards the incoming task silently.
5. Handle Timeouts Gracefully
Implementing a timeout for tasks can also mitigate thread pool exhaustion. By setting time limits for tasks, you can ensure that long-running operations do not clog up your resources:
executor.submit(() -> {
try {
// Task logic
} catch (TimeoutException ex) {
// Handle timeout
}
});
6. Optimize Task Design
Efficient task design can minimize the resource contention that leads to exhaustion.
- Break down long-running tasks into smaller, manageable pieces.
- Use asynchronous patterns when possible to allow tasks to complete more smoothly.
7. Regularly Review Application Performance
Regular performance assessments can help you identify trends over time. Analyzing logs and usage patterns ensures that you can adjust thread pool configurations proactively.
Closing Remarks
Thread pool exhaustion can be a significant bottleneck in your Java applications, but with careful planning and design, it's possible to manage and mitigate these risks. By configuring thread pools appropriately, monitoring system resources, and implementing effective task management strategies, you can maximize application efficiency and ensure a smooth user experience.
For more detailed insights into Java threading, consider exploring the Java Concurrency documentation or referring to Java Concurrency in Practice by Brian Goetz.
By understanding the intricacies of thread pooling and applying best practices, you can leverage the full potential of Java's concurrency features while avoiding the pitfalls of exhaustion.