Overcoming Issues with Java's Dynamic Class Unloading
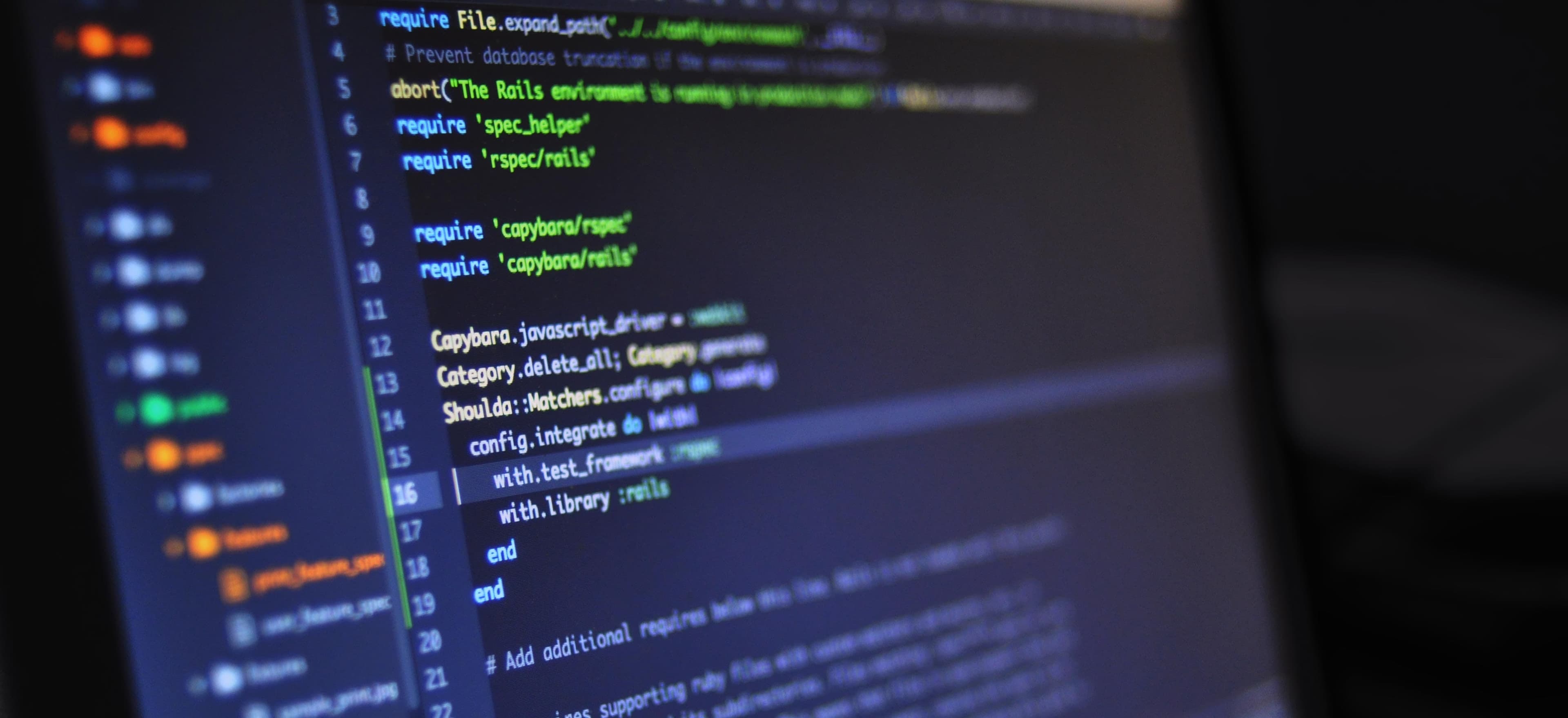
- Published on
Overcoming Issues with Java's Dynamic Class Unloading
Java is renowned for its robustness, portability, and vast ecosystem. However, one of the complexities that developers often encounter is dynamic class unloading, particularly when dealing with long-running applications or classloader hierarchies. This blog post dives into the challenges of class unloading in Java and presents techniques to effectively manage dynamic classes.
Understanding Java Class Loading
Before delving into dynamic class unloading, it's essential to understand how Java's class loading mechanism works. Java uses the ClassLoader to load classes into memory. Each class is loaded when needed, and if the class has been loaded once, it won't be reloaded unless it's explicitly unloaded.
Why Dynamic Class Unloading Matters
Dynamic class unloading allows Java applications to reclaim memory by unloading classes that are no longer in use. This is critical in many scenarios, such as:
- Hot-Reloading: Updating classes in a running application without restarting the entire service.
- Plugin Systems: Loading and unloading plugins dynamically.
- Memory Management: Reducing memory footprint by unloading unused classes in long-running applications.
Common Issues with Dynamic Class Unloading
1. ClassLoader Leaks
One of the most common issues with dynamic class unloading is ClassLoader leaks. This typically happens when classes loaded by a specific ClassLoader are still referenced somewhere in the application. These references prevent the ClassLoader (and the classes it loaded) from being garbage collected.
2. Static References
Sometimes, classes hold static references or singletons that keep them alive even after they are no longer needed. This can be problematic in environments such as application servers where redeployments are frequent.
3. System Resources
If classes are not unloaded correctly, they may still occupy system resources such as file handles or network connections, leading to resource exhaustion.
Solutions to Overcome Class Unloading Issues
1. Understanding ClassLoader Hierarchies
Java employs a hierarchical class loading mechanism where different ClassLoaders can have parent-child relationships. Understanding this hierarchy is pivotal in managing dynamic class unloading.
When a child ClassLoader loads a class, if the parent ClassLoader has already loaded that class, the child ClassLoader doesn't reload it. This means if a parent retains a static reference, it can hinder the unloading of the child's classes.
Code Example: ClassLoader Hierarchy
class Parent {
// Parent class implementation
}
class Child extends Parent {
// Child class implementation
}
In this example, if Parent
is loaded by the system ClassLoader and holds static references, even if Child
is no longer used, it could still prevent its unloading.
2. Remove Static References
To enable proper class unloading, avoid static variables in classes that will be frequently loaded and unloaded. If you must use static members, make sure to nullify them when they are no longer needed.
Code Example: Managing Static References
public class Cache {
private static Map<String, Object> cacheMap = new HashMap<>();
public static void put(String key, Object value) {
cacheMap.put(key, value);
}
public static Object get(String key) {
return cacheMap.get(key);
}
public static void clear() {
cacheMap.clear(); // Clear references before unloading
}
}
In the above code snippet, observe the clear
method, which is crucial before unloading the class. It ensures that no lingering references remain.
3. Use Weak References
Using WeakReference
can help to break the strong reference cycles that prevent class unloading. This can be particularly useful in caching strategies.
Code Example: Using WeakReference
import java.lang.ref.WeakReference;
public class WeakCache {
private static WeakReference<Object> weakObjectRef;
public static void put(Object object) {
weakObjectRef = new WeakReference<>(object);
}
public static Object get() {
return weakObjectRef.get(); // Will return null if the object is no longer referenced
}
}
With WeakReference
, the garbage collector can reclaim memory if there are no strong references to the object, allowing potentially unused classes to be unloaded.
4. Leverage Java ClassLoader for Dynamic Loading/Unloading
Implement a custom ClassLoader for your application that can handle dynamic loading and unloading of classes based on your specific needs. This ensures more control over which classes stay in memory.
Code Example: Custom ClassLoader
import java.io.File;
import java.net.URL;
import java.net.URLClassLoader;
public class DynamicClassLoader extends URLClassLoader {
public DynamicClassLoader(URL[] urls) {
super(urls);
}
public Class<?> loadClass(String name) throws ClassNotFoundException {
return super.loadClass(name);
}
public void unload() {
// Implement logic to unload classes and cleanup resources if needed
}
}
// Example usage
public class Main {
public static void main(String[] args) throws Exception {
File file = new File("path/to/classes");
URL[] urls = { file.toURI().toURL() };
DynamicClassLoader dynamicClassLoader = new DynamicClassLoader(urls);
Class<?> clazz = dynamicClassLoader.loadClass("com.example.YourClass");
// Use the class ...
// When done, invoke unload to release resources
dynamicClassLoader.unload();
}
}
The custom ClassLoader above allows dynamic loading while also providing a framework for unloading specific classes when they are not in use anymore.
5. Implement Application Monitoring
To effectively manage dynamic unloading, consider implementing monitoring solutions that can detect memory usage patterns and alert you to potential leaks or misuse of resources. Tools like Java Flight Recorder and VisualVM can provide insightful data on memory usage and ClassLoader behavior.
Final Thoughts
Dynamic class unloading in Java can be a double-edged sword. While it presents numerous advantages, improper handling often leads to significant issues such as memory leaks and resource exhaustion. By leveraging proper techniques—such as nullifying static references, using weak references, employing custom ClassLoaders, and monitoring your application—you can efficiently manage classes, ensuring that your Java applications remain responsive and performant.
For more reading on Java performance and memory management techniques, check out the articles on Java Memory Management and Managing ClassLoader.
By understanding and actively managing dynamic class unloading, developers can build more maintainable, efficient Java applications. Embrace these practices and ensure your applications can adapt to evolving requirements while minimizing resource consumption.