Mastering APT: Tackling Annotation Processing Errors in Java
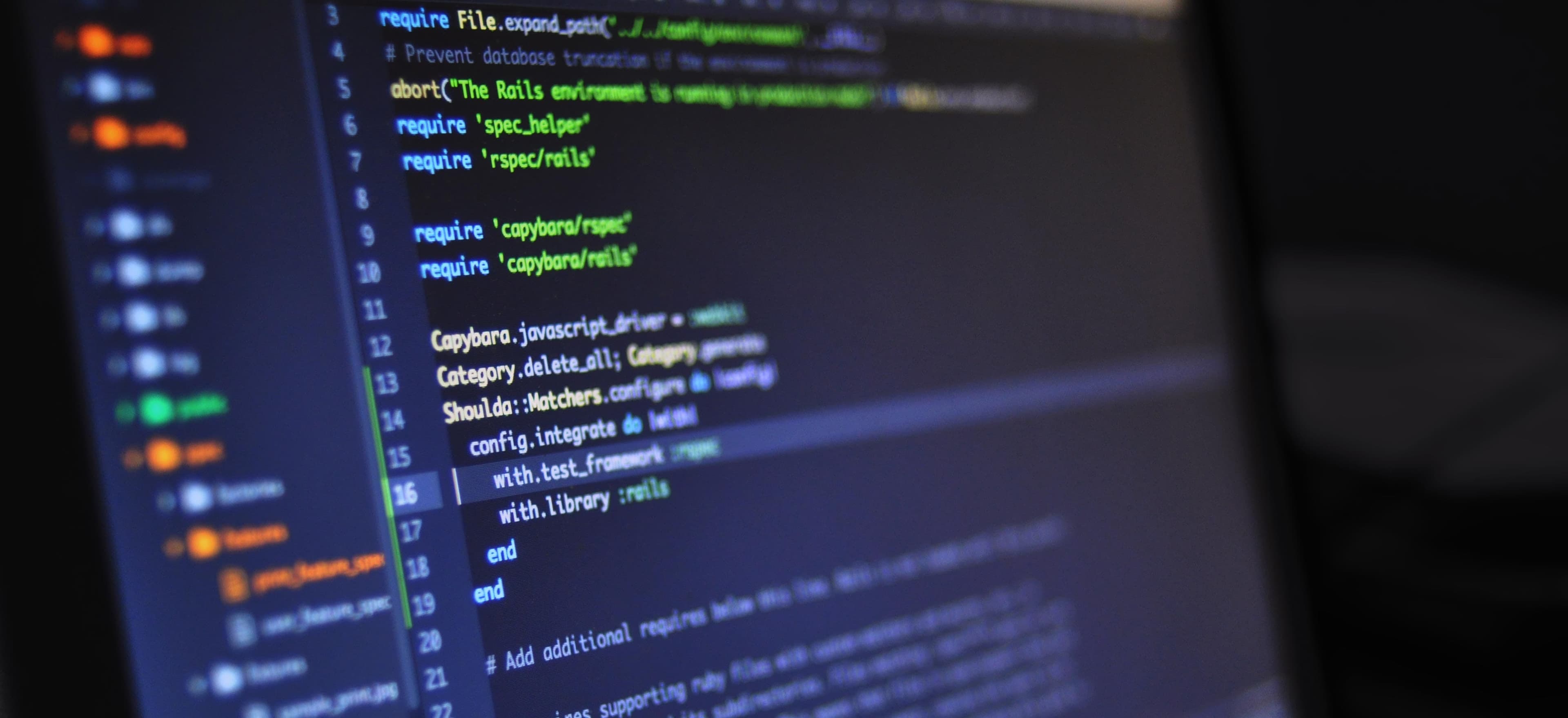
- Published on
Mastering APT: Tackling Annotation Processing Errors in Java
Java has always been a language that thrives on its ability to create robust and extendable applications. One of its powerful features is annotation processing, which allows developers to generate code or perform checks during the compilation process. Nevertheless, like any other tool, annotation processing is prone to errors, and understanding how to tackle these can significantly improve your development workflow.
In this blog post, we will delve deep into the world of annotation processing tools (APT), addressing common errors and presenting solutions. Along the way, we will provide exemplary code snippets and highlight best practices.
What is Annotation Processing?
Java annotations are metadata that provide data about a program but are not part of the program itself. Annotation processing refers to the process where tools analyse these annotations in source code to generate additional files or perform validations.
For instance, with the help of @Entity
in JPA (Java Persistence API), you can declare a class as a database entity. Annotation processors can generate boilerplate code that interfaces with the database seamlessly.
Setting Up Annotation Processing
To use annotation processing, you need to define annotations and create a processor. The following steps outline a simple setup:
// Defining a custom annotation
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
@Retention(RetentionPolicy.SOURCE)
public @interface MyCustomAnnotation {
String value();
}
By using @Retention(RetentionPolicy.SOURCE)
, we specify that the annotation information will be discarded by the compiler. This is ideal for annotation processing.
Next, we create an annotation processor:
import javax.annotation.processing.*;
import javax.lang.model.SourceVersion;
import javax.lang.model.element.TypeElement;
import java.util.Set;
@SupportedAnnotationTypes("MyCustomAnnotation")
@SupportedSourceVersion(SourceVersion.RELEASE_8)
public class MyAnnotationProcessor extends AbstractProcessor {
@Override
public boolean process(Set<? extends TypeElement> annotations, RoundEnvironment roundEnv) {
// Processing logic goes here
return true;
}
}
Common Axis of Errors
Even with a solid foundation, various issues can arise while using annotation processing:
-
ClassNotFoundException: This typically occurs when the processor cannot locate the specified annotation class. Ensure all dependencies are included in the compilation classpath.
-
NullPointerException: When accessing elements from the annotation processor, if the elements are not correctly formatted or expected structures are missing, a
NullPointerException
can easily surface. -
Annotation Processing Failures: Often arise from invalid configurations, missing annotations, or faulty logic in the processing method.
-
Unexpected Behavior in Generated Code: If your generated code isn't functioning correctly, it often requires debugging the processor's output.
Debugging Annotation Processing Errors
Let's dive into the common problems mentioned and explore strategies to resolve them.
1. ClassNotFoundException
ClassNotFoundExceptions often arise in environments where dependencies are improperly managed. Use Maven or Gradle to ensure all dependencies are listed in your pom.xml
or build.gradle
.
Example Setup with Maven
<dependency>
<groupId>javax.annotation</groupId>
<artifactId>javax.annotation-api</artifactId>
<version>1.3.2</version>
</dependency>
2. Handling NullPointerExceptions
Properly check for nulls, especially when working with the RoundEnvironment
and Element
objects. Always ensure the elements are non-null before processing them.
if (roundEnv.getElementsAnnotatedWith(MyCustomAnnotation.class).isEmpty()) {
return false;
}
3. Streamlining Annotation Processing Logic
Ensure your logic for processing annotations is robust. Always validate the input and output. Here’s a more refined implementation:
@Override
public boolean process(Set<? extends TypeElement> annotations, RoundEnvironment roundEnv) {
if (roundEnv.processingOver()) {
return false;
}
for (Element element : roundEnv.getElementsAnnotatedWith(MyCustomAnnotation.class)) {
String value = element.getAnnotation(MyCustomAnnotation.class).value();
// Validation logic here
}
return true;
}
4. Addressing Unexpected Code Generation
When encountering unexpected behaviors in generated code, step back and analyze your annotation processing logic. You can add debugging information using processingEnv.getMessager().printMessage(...)
.
processingEnv.getMessager().printMessage(Diagnostic.Kind.NOTE, "Processing: " + element.getSimpleName());
This can provide insights into the flow of your processing logic.
Best Practices for Annotation Processing
To excel in Annotation Processing, here are some best practices:
-
Use a Robust Annotation Naming Scheme: Meaningful names lead to better comprehension of your created annotations and their intended use.
-
Document Your Annotations: Documentation is crucial. Well-documented annotations will save you time and effort in the future.
-
Keep Processors Lightweight: Focus on making processors only do one thing well, which fosters better maintainability and performance.
-
Test Your Processor: Unit tests are a must. They can help catch errors before you compile your code. Tools exist like JUnit specifically designed for testing annotation processors.
-
Utilize Dependency Injection: For complex scenarios, considering a DI framework can help simplify configuration and manage state within your processor.
Lessons Learned
Annotation processing is a powerful feature in the Java ecosystem, enabling developers to write cleaner and more efficient code. By mastering APT and understanding common errors, you can streamline your development process, ensuring your applications are not only robust but also maintainable.
For further reading and a deeper understanding of annotation processing, consider the following resources:
These resources provide foundational and advanced knowledge that can help solidify your expertise in Java and annotation processing. Happy coding!