Resolving Java Jar Hell: Your Ultimate Guide
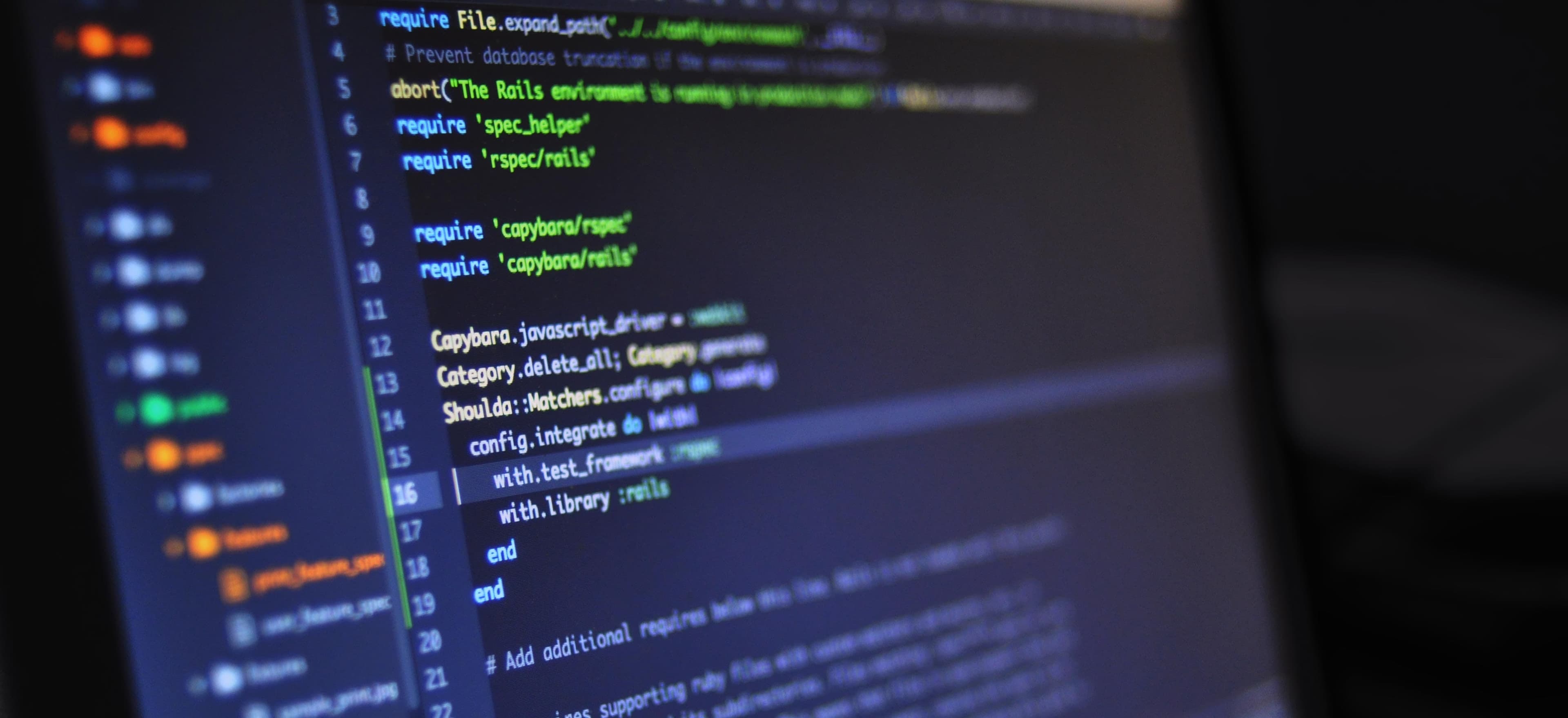
- Published on
Resolving Java Jar Hell: Your Ultimate Guide
Java developers often face challenges stemming from library dependencies in complex applications. One prominent issue that arises is known as "Jar Hell." This blog post will delve into what Jar Hell is, how it manifest, and provide practical solutions to resolve it effectively.
What is Jar Hell?
Jar Hell refers to conflicts that arise from multiple versions of Java Archive (JAR) files being present in the same classpath. These conflicts can lead to unpredictable behavior, runtime errors, and even application crashes. The root causes can often be traced back to:
- Different versions of the same library being included.
- Class files in JARs that have the same name but different implementations.
- Overlapping libraries that contain classes with the same fully qualified name.
To visualize, consider a scenario where you're building a Java application that relies on several libraries. If two of these libraries depend on different versions of a common third library, you may find yourself in the infamous Jar Hell.
Signs You Are in Jar Hell
Identifying Jar Hell can sometimes be non-trivial. Below are some clear signs that your application may already be suffering from this issue:
- ClassNotFoundException: When your Java application cannot find a class it needs, it's a likely sign that the JAR containing that class is either missing or conflicts with another version.
- NoSuchMethodError or NoClassDefFoundError: These errors typically indicate that you're using a method or class that is not present in the loaded version of the JAR.
- Unexpected Behavior: If your application behaves differently under various deployment environments, conflicting JARs might be the reason.
Solutions to Jar Hell
1. Dependency Management Tools
Using dependency management tools like Maven or Gradle can significantly simplify the task of managing dependencies and resolving conflicts.
Using Maven
Maven uses a central repository and a local repository to manage dependencies. You can specify which version of a library you want to use, and Maven will take care of fetching and configuring it.
For example, in your pom.xml
, you can declare dependencies like so:
<dependencies>
<dependency>
<groupId>org.example</groupId>
<artifactId>some-library</artifactId>
<version>1.0.0</version>
</dependency>
<dependency>
<groupId>org.example</groupId>
<artifactId>another-library</artifactId>
<version>1.5.0</version>
</dependency>
</dependencies>
Why this works: Maven automatically handles transitive dependencies, so if some-library
depends on another library, Maven includes it for you—all without causing version conflicts.
Using Gradle
Similar to Maven, Gradle offers dependency management with a flexible Groovy-based DSL. Here’s an example of how you can declare dependencies:
dependencies {
implementation 'org.example:some-library:1.0.0'
implementation 'org.example:another-library:1.5.0'
}
Why this works: Gradle handles version conflicts with its built-in resolution strategies. You can also enforce specific versions or ranges, reducing the likelihood of encountering version-related issues.
2. ClassLoader Isolation
For larger applications, particularly those using OSGi or Java EE (now Jakarta EE), utilizing ClassLoader isolation can provide a solution.
Class loading in Java allows applications to load different versions of a class, isolated from one another. This can be critical in applications that dynamically load modules or plugins.
Here’s how you can define a simple ClassLoader:
URL[] urls = {new File("path/to/jars/some-library.jar").toURI().toURL()};
URLClassLoader classLoader = new URLClassLoader(urls);
Class<?> loadedClass = Class.forName("com.example.SomeClass", true, classLoader);
Why this works: By isolating different libraries in separate ClassLoaders, you can prevent class conflicts between different versions of JARs, allowing simultaneous usage.
3. Shade Your JARs
If you have control over the libraries you are using, you might consider shading them, allowing you to create a "fat JAR" that contains all dependencies bundled uniquely. This prevents conflicts because it renames the classes in the JAR.
Maven Shade Plugin
To do this using Maven, you can add the following plugin configuration to your pom.xml
:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-shade-plugin</artifactId>
<version>3.2.4</version>
<executions>
<execution>
<phase>package</phase>
<goals>
<goal>shade</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
Why this works: The Shade Plugin packages your application and its dependencies into a single JAR, renaming classes when necessary to avoid conflicts.
4. Diagnosing Dependency Conflicts
Sometimes, you will need to troubleshoot and diagnose which conflicting dependencies are causing issues. Tools like Apache Maven's dependency tree can help.
You can use the following command to display your dependency tree:
mvn dependency:tree
Why this works: This command outputs the hierarchy of dependencies, which lets you see where potential conflicts originate.
5. Utilizing Environment-Specific Classpaths
Another effective way to manage library versions is through environment-specific classpaths. By creating a specific structure for your deployment environments (Staging, QA, Production), you can ensure that each environment uses only the appropriate versions of libraries.
For instance, you can have a lib
folder structure:
/lib/
/staging/
/production/
You would then configure your application to load these paths based on the environment.
Why this works: This approach encapsulates library management to each environment, limiting version conflicts across deployments.
Closing Remarks
Resolving Jar Hell requires a combination of good practices in dependency management, careful use of ClassLoaders, and sometimes creative solutions such as shading. Utilizing tools like Maven and Gradle will empower you to better control your application’s dependencies and minimize conflicts.
For more details about dependency management in Gradle, visit the official Gradle documentation. Additionally, if you are interested in diving deeper into OSGi and ClassLoader isolation, check out the OSGi Alliance.
With these strategies, you’ll navigate the complexities of Java dependencies more effectively, ensuring your applications run smoothly and efficiently.