Mastering Java Externalization: Avoiding Common Pitfalls
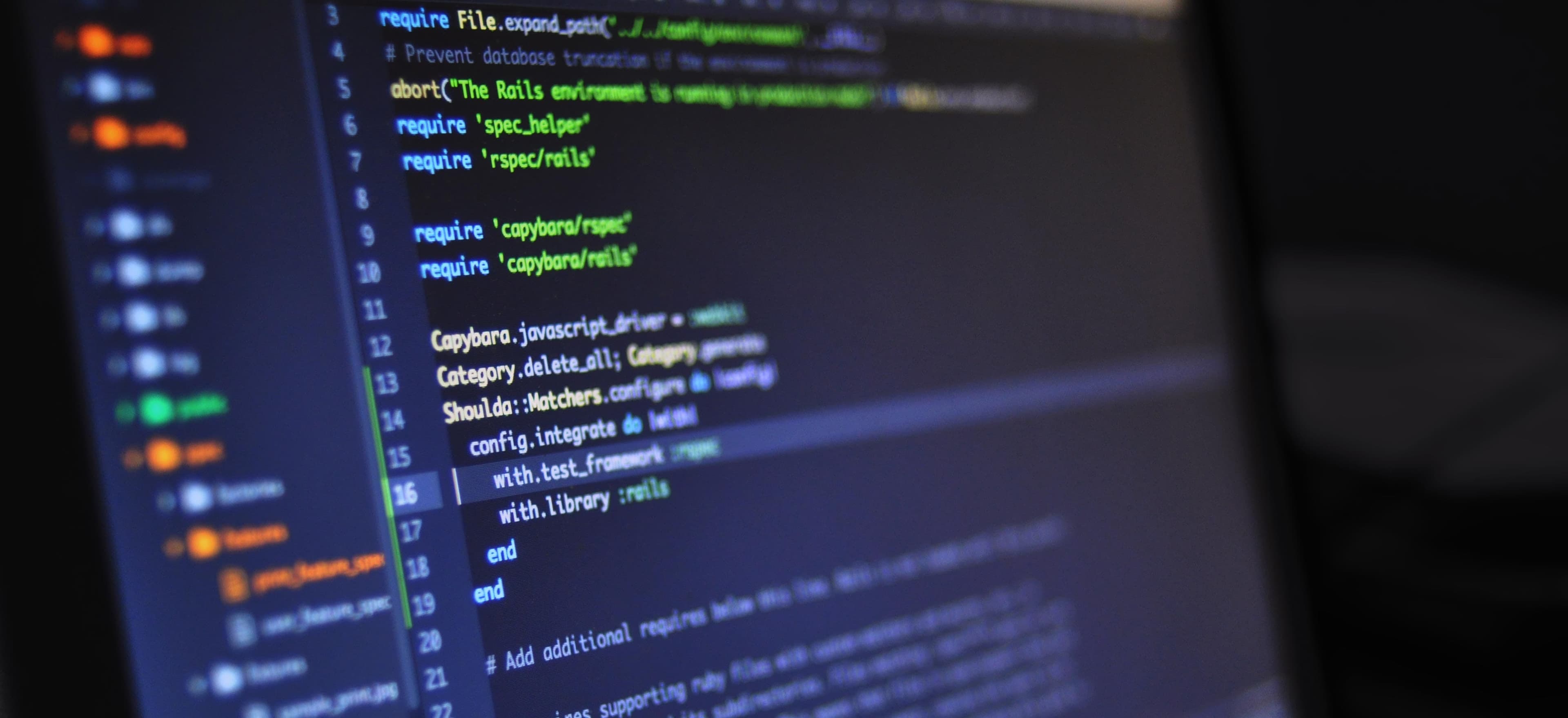
- Published on
Mastering Java Externalization: Avoiding Common Pitfalls
When it comes to Java serialization, developers often pursue its counterpart: externalization. Externalization is a powerful feature, allowing developers to control the serialization process manually. However, it is fraught with pitfalls that can lead to fewer maintainable and error-prone code. In this comprehensive guide, we will explore the concept of Java Externalization, delve into its advantages over serialization, and highlight common pitfalls while illustrating best practices through actionable code snippets.
What is Externalization in Java?
Java serialization allows for the conversion of an object into a byte stream for storage or transmission. This means we can persist the state of an object and later reconstruct it. Externalization, on the other hand, takes this a step further, allowing developers to dictate exactly how and what data is serialized.
To implement externalization, a class must:
- Implement the
java.io.Externalizable
interface. - Provide implementations for two methods:
writeExternal(ObjectOutput out)
andreadExternal(ObjectInput in)
.
When to Use Externalization
Externalization should be used when:
- You need control over the serialization format.
- You want to minimize the serialized object size.
- You want to manage backward compatibility manually.
Basic Example of Externalization
Let's start with a simple example that highlights how externalization works.
Code Snippet: Implementing Externalizable
import java.io.*;
public class UserProfile implements Externalizable {
private String username;
private String email;
// Default constructor is mandatory for Externalizable
public UserProfile() {
}
public UserProfile(String username, String email) {
this.username = username;
this.email = email;
}
@Override
public void writeExternal(ObjectOutput out) throws IOException {
out.writeUTF(username);
out.writeUTF(email);
}
@Override
public void readExternal(ObjectInput in) throws IOException {
username = in.readUTF();
email = in.readUTF();
}
@Override
public String toString() {
return "UserProfile{" + "username='" + username + '\'' + ", email='" + email + '\'' + '}';
}
}
Why Use This Approach?
This code snippet provides a clear structure for the serialization process. Implementing the writeExternal
and readExternal
methods allows the class to dictate what fields get serialized. Additionally, the presence of a no-argument constructor is crucial, as Java requires it for externalization.
Advantages of Externalization Over Serialization
- Performance: Externalization can lead to better performance because you can serialize only relevant fields.
- Control: Greater control over the serialization process allows for finer adjustments, especially in cases involving large and complex objects.
- Flexibility: Externalizable classes can be changed more easily without breaking existing serialized data formats, as you manage your serialization format.
Common Pitfalls in Externalization
While externalization offers many benefits, developers often encounter pitfalls. Here are some common ones with solutions.
1. Forgetting the No-Argument Constructor
Pitfall: Failing to provide a default constructor can lead to java.io.InvalidClassException
when deserializing.
Solution: Always define a public no-argument constructor.
2. Missing or Incorrectly Implemented Methods
Pitfall: If you forget to implement writeExternal
or readExternal
, the object may not serialize or deserialize correctly.
Solution: Always ensure that both methods are implemented correctly. Notify users with clear error messages if serialization fails.
3. Handling of Transient Fields
Pitfall: Transient fields are not serialized automatically, which may lead to loss of important data if not handled properly.
Solution: If you need a transient field in your object, ensure you explicitly manage the serialization logic in writeExternal
and readExternal
.
@Override
public void writeExternal(ObjectOutput out) throws IOException {
out.writeUTF(username);
out.writeUTF(email);
// Handle transient field
// out.writeBoolean(isActive);
}
@Override
public void readExternal(ObjectInput in) throws IOException {
username = in.readUTF();
email = in.readUTF();
// Reinitialize transient field
// isActive = true; // or some default logic
}
4. Ignoring Backward Compatibility
Pitfall: Changing class definitions without managing versioning can lead to Stream Corruption
.
Solution: Use explicit versioning strategies in writeExternal
and readExternal
. For instance, maintain a static final field to represent the current version of the class.
Code Snippet: Adding Version Control
private static final long serialVersionUID = 1L;
@Override
public void writeExternal(ObjectOutput out) throws IOException {
out.writeInt(1); // Write version
out.writeUTF(username);
out.writeUTF(email);
}
@Override
public void readExternal(ObjectInput in) throws IOException {
int version = in.readInt();
if (version == 1) { // Handle version-specific logic
username = in.readUTF();
email = in.readUTF();
}
// Handle future versions
}
5. Not Handling Exceptions Properly
Pitfall: Exception handling can get neglected, leading to runtime failures during serialization or deserialization.
Solution: Always wrap your Serialization logic with try-catch blocks to handle I/O exceptions gracefully.
@Override
public void writeExternal(ObjectOutput out) {
try {
out.writeUTF(username);
out.writeUTF(email);
} catch (IOException e) {
System.err.println("Error during serialization: " + e.getMessage());
}
}
Closing Remarks: Mastering Externalization
Java externalization provides developers with significant power over serialization. However, understanding its challenges is crucial to mastering its use. By avoiding the common pitfalls described and adhering to best practices, you can implement externalization effectively and maintain a codebase that is both robust and easy to manage.
For more deep dives into Java serialization techniques, consider checking resources such as Java's Official Documentation or the comprehensive guide on Java's I/O streams.
By grasping these concepts, your journey to mastering Java externalization will not only be successful but also enriching. Happy coding!
Checkout our other articles