Navigating Edge Cases in Java Type Inference Simplified
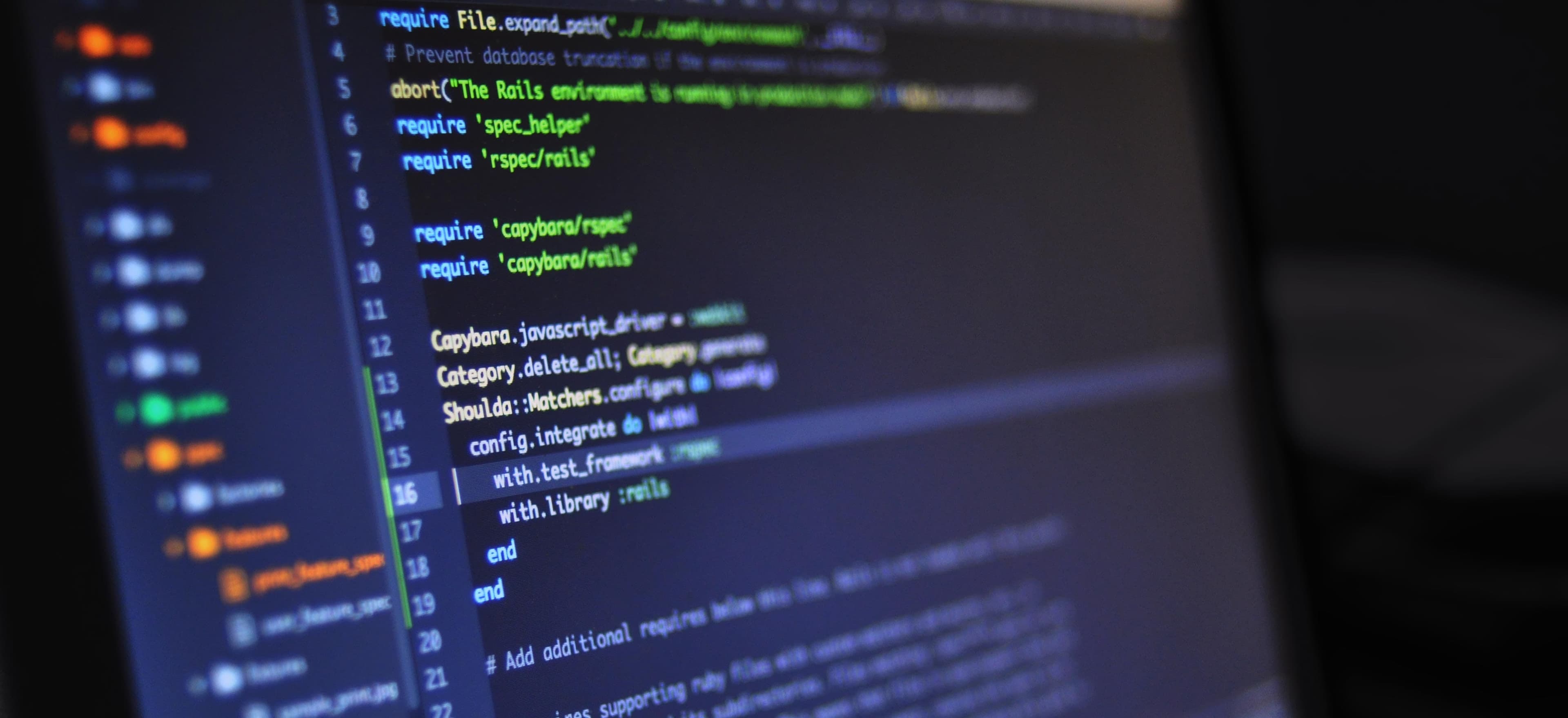
- Published on
Navigating Edge Cases in Java Type Inference Simplified
Understanding Java's type inference mechanism can be a daunting task, especially when corner cases arise. Type inference allows the Java compiler to deduce the types of variables, method arguments, and return types based on context, reducing the need for verbose type declarations. While this is an intuitive feature, it can often lead to confusion when dealing with edge cases.
In this blog post, we'll explore several edge cases in Java's type inference system, why they matter, and how to navigate them effectively. We'll back our discussions with relevant code snippets that illustrate these points clearly.
What is Type Inference?
Type inference in Java, particularly since Java 7, allows you to omit explicit types when using generics. For example, instead of writing:
Map<String, List<String>> myMap = new HashMap<String, List<String>>();
You can simplify it to:
Map<String, List<String>> myMap = new HashMap<>();
In the second example, the Java compiler infers the type arguments from the left-hand side of the assignment. This not only improves readability but also maintains type safety.
The Importance of Edge Cases
Even though type inference reduces boilerplate code, it can sometimes lead to subtle bugs and misunderstandings. These edge cases often appear in methods overloading and type erasure scenarios. Let's breakdown some of these cases.
1. Type Inference with Collections
Example Scenario
Imagine a situation where we have a generic method that takes a list of objects and processes it.
public static <T> void processList(List<T> list) {
for (T item : list) {
System.out.println(item);
}
}
You might think you can call this method with a raw type, like this:
List<String> strings = new ArrayList<>();
strings.add("Hello");
processList(strings);
Discussion
While the above code works perfectly, let’s examine what happens when using a completely raw type:
List rawList = new ArrayList();
rawList.add("Hello");
processList(rawList); // Warning: unchecked call
This results in an unchecked call since the raw type List
is not compatible with the expected type List<T>
. Type safety is compromised because rawList
could contain any object, breaking the generic type contract.
Key Takeaway
Always use generics with collections to ensure type safety.
2. Method Overloading and Type Inference
Method overloading can introduce ambiguity if both methods have similar signatures that can be invoked by a caller.
Example
public class OverloadedMethod {
public void display(String s) {
System.out.println("String: " + s);
}
public void display(Object o) {
System.out.println("Object: " + o);
}
}
If you try to call display(null)
, you might expect it to resolve to the String
method.
OverloadedMethod om = new OverloadedMethod();
om.display(null); // Ambiguous call
Discussion
The Java compiler cannot infer which display()
method to use since null
can represent both a String
and an Object
. This results in a compiler error: "Ambiguous method call".
Key Takeaway
Avoid overloading with methods that can accept null
as an argument unless necessary. It’s better to use distinct method names or refine your method signatures.
3. Varargs and Type Inference
Variadic arguments (varargs) can lead to similar issues with type inference.
Example
public void printNumbers(Integer... numbers) {
for (Integer num : numbers) {
System.out.println(num);
}
}
public void printNumbers(Object... objects) {
for (Object obj : objects) {
System.out.println(obj);
}
}
If you invoke it like this:
printNumbers(1, 2, 3); // Ambiguous call
Discussion
Here, just like before with null
, the compiler sees both printNumbers(Integer...)
and printNumbers(Object...)
as valid candidates, resulting in an ambiguous call error.
Key Takeaway
When using varargs, ensure that the signatures are distinct enough to avoid ambiguity.
4. Captured Wildcards
Wildcards can also complicate type inference in Java.
Example
When you have methods that operate on collections of a generic type:
public static void processList(List<?> list) {
for (Object item : list) {
System.out.println(item);
}
}
Let’s consider calling the method with different types of lists:
List<String> stringList = new ArrayList<>();
stringList.add("Test");
processList(stringList);
List<Integer> intList = new ArrayList<>();
intList.add(1);
processList(intList);
Discussion
In this case, type inference works because the method can accept any type due to the wildcard. However, if you try to add an element to these lists inside the processList
method, you will be restricted:
public static void addToList(List<?> list) {
list.add("new item"); // Compilation error
}
The reason is simple: Java cannot guarantee which type of items the list can accept.
Key Takeaway
When using wildcards, remember that they are immutable in terms of adding elements. Always declare the specific type you want if you need to modify the list.
In Conclusion, Here is What Matters
Understanding the edge cases in Java's type inference is crucial for writing clean, safe, and maintainable code. Whether you are dealing with collections, method overloads, varargs, or wildcards, knowing the nuances helps you communicate your intentions clearly both to the compiler and your fellow developers.
Java's type inference is there to simplify our lives, but with power comes responsibility. Avoiding ambiguous calls, embracing generic collections, and respecting wildcard constraints will empower you to write more robust Java applications.
For more advanced insights into type inference and generics, consider checking out the Java Documentation on Generics.
Happy coding!
Checkout our other articles