Resolving Java Classpath and Modulepath Conflicts Easily
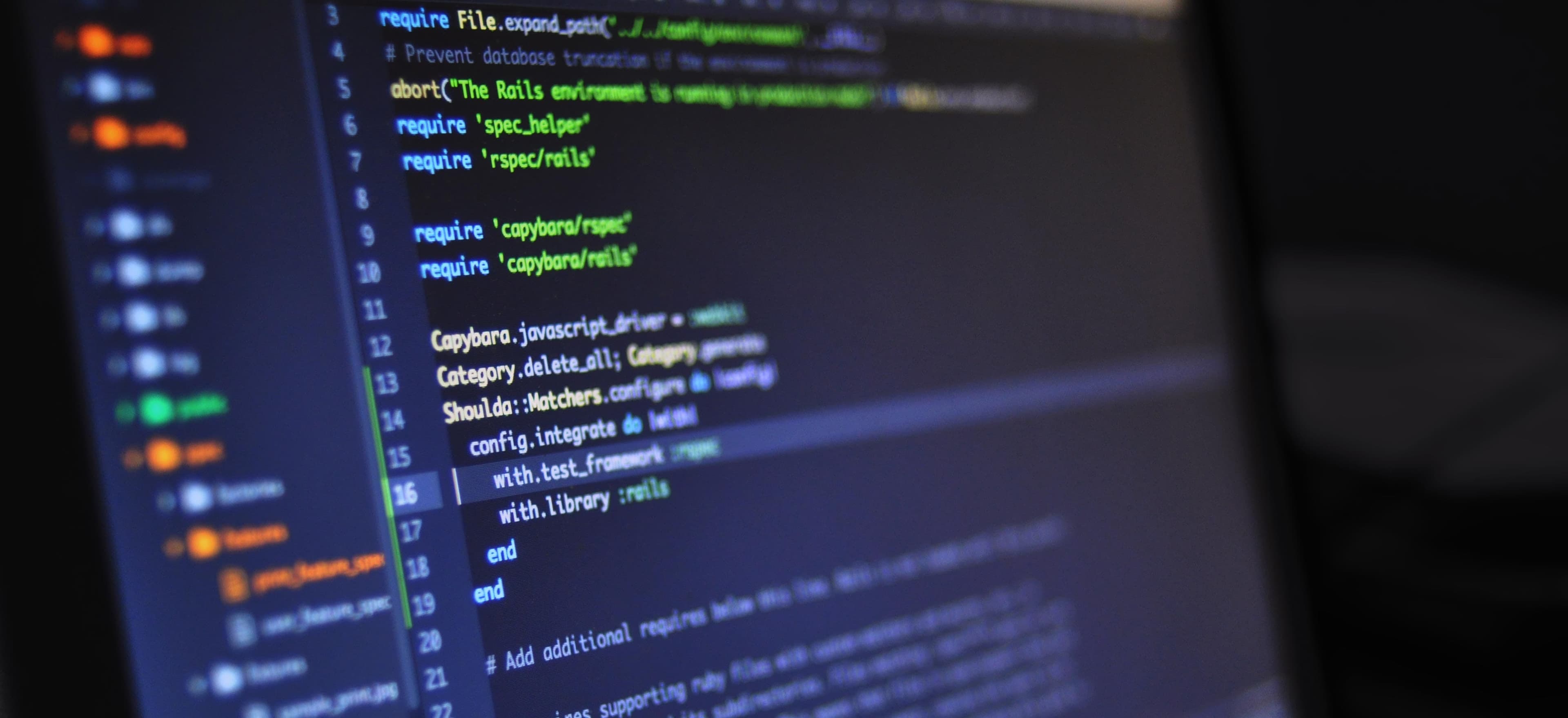
- Published on
Resolving Java Classpath and Modulepath Conflicts Easily
Java, one of the most widely-used programming languages, has continually evolved over the years. With the introduction of modules in Java 9, developers have been given powerful tools to manage dependencies and encapsulation more effectively. However, this has led to potential conflicts arising between the traditional classpath and the newer modulepath. In this blog post, we will explore how to resolve these conflicts seamlessly.
Understanding Classpath vs. Modulepath
Before diving into conflict resolution, it's essential to establish a clear understanding of what classpath and modulepath are:
-
Classpath: This is a fundamental part of Java that tells the Java Virtual Machine (JVM) where to find user-defined classes and packages in Java programs. It's a way to organize libraries and ensures that the required classes are available at runtime.
-
Modulepath: With the introduction of the Java Platform Module System (JPMS) in Java 9, the modulepath takes center stage. Unlike the classpath, which is a flat structure, the modulepath supports encapsulation, allowing developers to define explicit dependencies between modules.
While they serve similar purposes in managing dependencies, the two systems are fundamentally different. Mixing the two can lead to conflicts, primarily when trying to access classes or packages that may not be correctly resolved.
Common Conflicts
1. Class Visibility
One of the primary issues that arise when transitioning to modules is class visibility. In traditional Java applications, classes are accessible as long as they are in the classpath. However, with the module system, classes that are not exported from a module are not visible to other modules.
Example: Consider two modules, moduleA
and moduleB
:
moduleA
exports a packagecom.example.utils
.moduleB
tries to access a classUtils
fromcom.example.utils
but does not declare a dependency onmoduleA
.
Here's the module declaration for moduleA
:
module moduleA {
exports com.example.utils;
}
Resolution: To resolve the visibility issue in moduleB
, you must declare a dependency in module-info.java
:
module moduleB {
requires moduleA;
}
2. Duplicate Classes
Another conflict is when two different libraries provide the same class. This can happen often in large projects or when using multiple dependencies.
Example: If your project uses both libA
and libB
, which both contain a class com.example.MyClass
, it can lead to runtime errors.
Resolution: To avoid such conflicts, identify which library to use and exclude the other, or, if possible, update to different versions of the libraries that do not have these conflicts. Apache Maven and Gradle provide mechanisms to manage such dependency conflicts.
Here's how to exclude dependencies in Maven:
<dependency>
<groupId>com.example</groupId>
<artifactId>libA</artifactId>
<version>1.0</version>
<exclusions>
<exclusion>
<groupId>com.example</groupId>
<artifactId>libB</artifactId>
</exclusion>
</exclusions>
</dependency>
3. Runtime Exceptions
Runtime exceptions can often arise due to classpath/modulepath conflicts. An attempt to load a class at runtime that could not be resolved leads to ClassNotFoundException
or NoClassDefFoundError
.
Example: If you run a module but forget to include the necessary libraries or modules in the modulepath:
java --module-path mods -m moduleB/com.example.Application
Resolution: Make sure that all dependencies are included in the modulepath by correctly structuring your command. The modulepath should point to directories containing your modules or jar files.
Best Practices for Managing Classpath and Modulepath
Adopting best practices is crucial in preventing and resolving conflicts between classpath and modulepath. Here are some strategies:
-
Use a Build Tool: Using a build tool like Maven or Gradle can help manage dependencies effectively, as they can resolve the correct versions automatically and avoid conflicts.
-
Encapsulate Modules: Make sure to design your modules with encapsulation in mind. Explicitly export only those packages that must be accessible from other modules.
-
Keep Dependencies Updated: Regularly update your dependencies to ensure that you're using the most stable versions and to benefit from fixed bugs and enhancements.
-
Migration Plan: If you are transitioning from a classpath to a modulepath, create a clear migration plan. Identify core modules and their dependencies to minimize conflicts.
-
Testing: Comprehensive testing is necessary. Run unit and integration tests to ensure that changes in dependencies do not break existing functionality.
The Last Word
Managing classpath and modulepath effectively is crucial to the success of your Java applications. By understanding the differences, anticipating conflicts, and following best practices, you can maintain a harmonious development environment.
For more in-depth insights on Java modules, consider reading the Official Java Documentation on Modules.
To sum up, while the transition from classpath to modulepath might seem daunting at first, with proper understanding and conflict resolution strategies, it can lead to cleaner, more maintainable code. Happy coding!
Feel free to reach out if you have any questions or need further clarification.
Checkout our other articles