Understanding the Dangers of Java Unsafe Class Usage
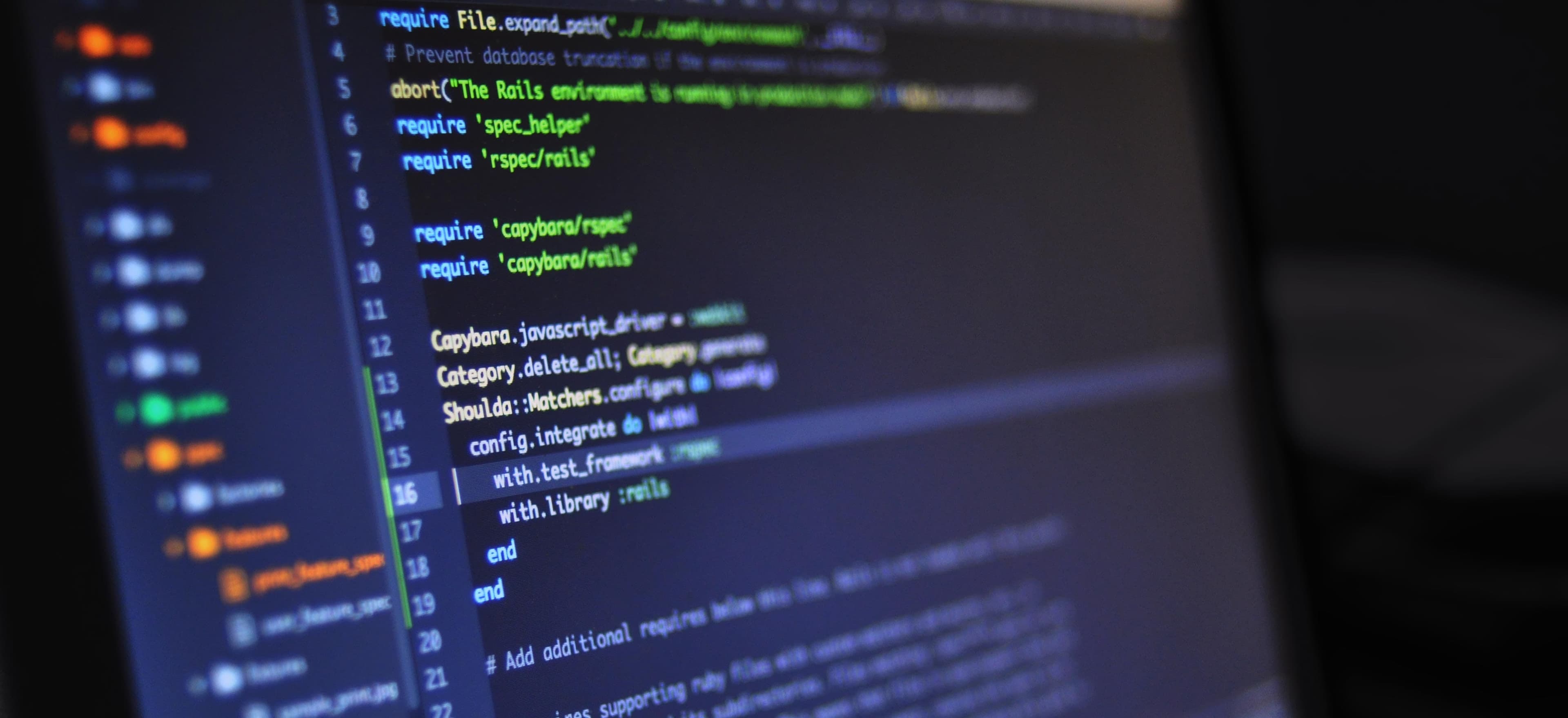
- Published on
Understanding the Dangers of Java Unsafe Class Usage
Java is renowned for its robustness and safety features, but there exist some classes that can negate these advantages. Chief among them is the Unsafe
class. While it offers powerful capabilities that allow for low-level programming, its misuse can lead to severe security vulnerabilities. This article aims to outline the dangers of using the Unsafe
class and provide some best practices.
What is the Unsafe Class?
The Unsafe
class is a part of the sun.misc
package. It provides low-level operations that are not ordinarily available through standard Java APIs. Functions such as memory allocation, memory deallocation, and atomic operations are some of its features.
Java designed this class for the following purposes:
- Performance Tuning: Low-level access can enhance performance in specific scenarios.
- Memory Management: It allows direct manipulation of memory, bypassing the Java Memory Model.
- Implementing Data Structures: Advanced data structures can be built that exploit these capabilities.
Why Use Unsafe?
While you might wonder why JNI (Java Native Interface) or other libraries aren't sufficient for low-level tasks, the Unsafe
class is faster. With JNI
, there's a context switch between Java and native code, which incurs overhead. Unsafe
operates entirely within the Java Virtual Machine (JVM) context, avoiding additional overhead.
import sun.misc.Unsafe;
public class UnsafeExample {
private static final Unsafe unsafe = UnsafeRetriever.getUnsafe();
private volatile long value; // Example variable
public void incrementValue() {
long offset = unsafe.objectFieldOffset(UnsafeExample.class.getDeclaredField("value"));
unsafe.getAndAddLong(this, offset, 1); // Atomically increment the value
}
}
In the snippet above, we retrieve the offset for the value
field and perform an atomic increment using Unsafe.getAndAddLong()
. This is an illustration of a feature that can optimize performance; however, the risks involved in using Unsafe
can outweigh the benefits if not handled correctly.
The Dangers of Using Unsafe
1. Bypassing Java's Safety Guarantees
Java was designed with certain safety mechanisms that prevent developers from performing operations that could compromise stability. When you use Unsafe
, you can:
- Directly manipulate memory addresses.
- Create memory leaks or null pointer dereferences.
For example:
public void unsafeMemoryManipulation() {
long baseAddress = unsafe.allocateMemory(8);
unsafe.putLong(baseAddress, 42L); // Unsafe memory writing
// Note: Potential memory leak if not deallocated
}
Failing to properly free memory allocated with Unsafe.allocateMemory()
can lead to memory leaks, negating Java's garbage collection benefits.
2. Security Risks
Operations that manipulate memory directly can expose sensitive data or lead to arbitrary code execution vulnerabilities. If an attacker can exploit code using Unsafe
, they can:
- Execute harmful instructions.
- Manipulate application state unexpectedly.
Example of Possible Exploitation
Consider an application that uses Unsafe
to serialize objects directly into memory. If not sufficiently secured, it could lead to exposure of sensitive data:
public byte[] serialize(Object obj) {
long address = unsafe.allocateMemory(1024); // Assume specific size
unsafe.putObject(address, 0, obj);
// Serialize object without proper safety checks
return unsafe.getByteArray(address, 0, 1024);
}
In this example, an attacker could manipulate the serialization process to output sensitive information from memory.
3. Portability Issues
Java was designed to be platform-independent. However, using class Unsafe
makes your code less portable. This could lead to inconsistent behavior across different systems, as low-level memory operations rely on underlying hardware architecture and JVM implementation.
4. Unhandled Exceptions
Unlike standard Java methods, exceptions thrown from Unsafe
methods often go unchecked. This means you can face:
- Crashes from the JVM without any diagnostic information.
For example, if you try to access a memory address using Unsafe
methods that are not allocated or authorized, it may lead to a segmentation fault without clear indications of what went wrong.
Best Practices for Using Unsafe
If you find yourself needing Unsafe
, ensure you adhere to the following practices:
1. Limit Usage
Only use Unsafe
when absolutely required. Consider alternative libraries before proceeding, such as Apache Commons Lang or other established standards that abstract away the complexities and dangers of low-level programming.
2. Wrap Unsafe Calls
Encapsulate dangerous calls in a separate class or method. This limits their exposure throughout your codebase and allows for improved troubleshooting.
3. Testing
Thoroughly test any code utilizing Unsafe
. Ensure you account for edge cases and improper usage, and run extensive integrations tests.
4. Documentation
Provide sufficient commentary documenting why you are using Unsafe
and its intended purpose. This can aid other developers (or your future self) in understanding the reasoning and risks when revisiting the project.
/**
* Handles low-level memory operations for custom data structure.
* Caution: This code manipulates memory directly.
*/
public class LowLevelOperations {
// Unsafe usage code...
}
Closing the Chapter
The Unsafe
class provides powerful capabilities that can enhance performance and memory management in Java applications. However, the risks associated with its use - from bypassing Java's safety mechanisms to creating security vulnerabilities - cannot be ignored.
Before employing Unsafe
, seriously consider if it's the right tool for the job. Often, the safer alternatives in Java's extensive libraries will provide adequate functionality without the complexities and dangers of low-level manipulation.
For further reading on memory management in Java, check out Java Memory Model and understand how it interacts with the Unsafe
class.
Stay safe, and happy coding!