How Static Fields Cause Java Memory Leaks: A Deep Dive
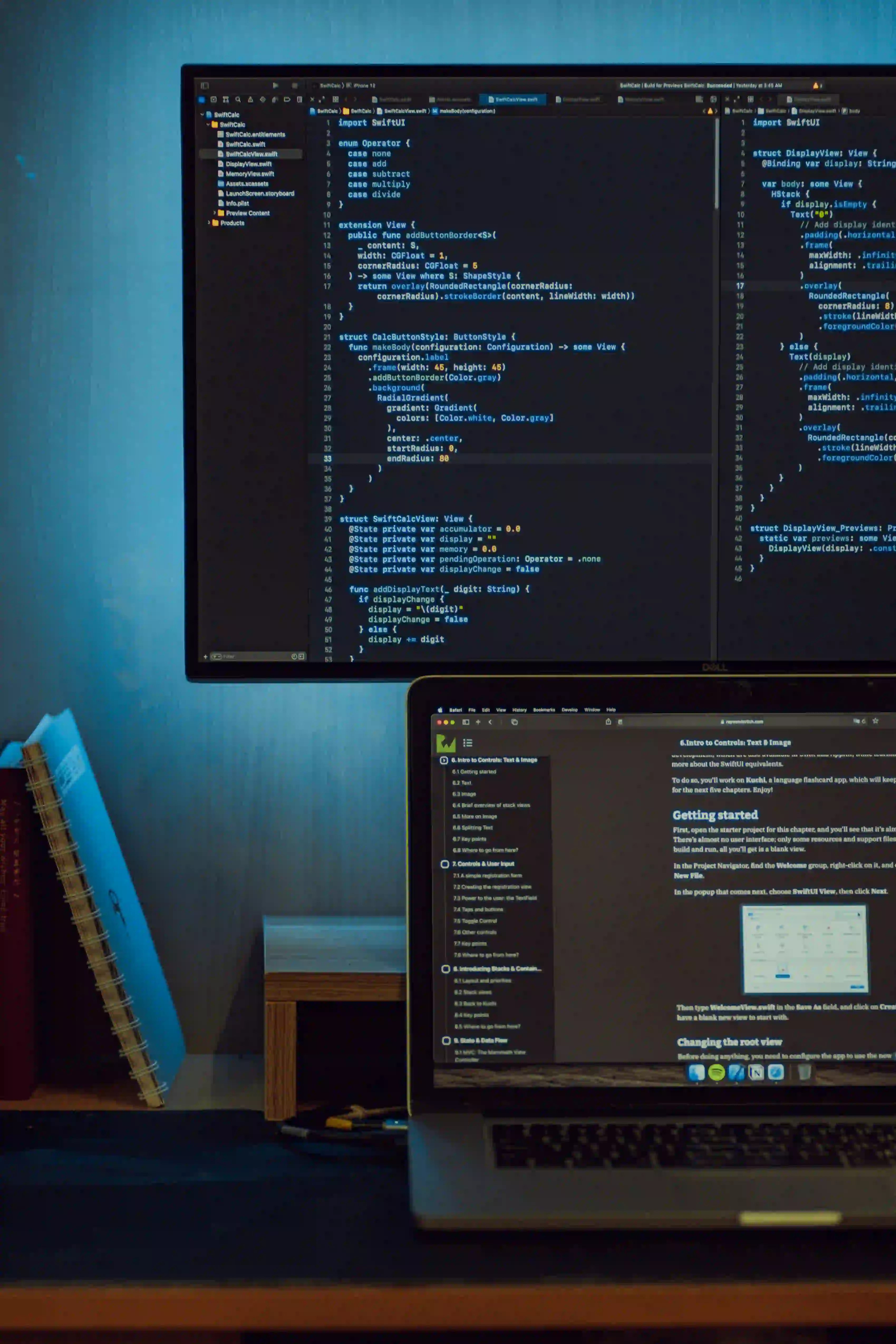
How Static Fields Cause Java Memory Leaks: A Deep Dive
Java developers enjoy a reputation for writing robust and reliable code, but even the best can encounter memory leaks, especially when using static fields. In this post, we will explore how static fields can lead to memory leaks in Java applications. We’ll draw on principles from Java memory management, look at problem scenarios, and provide code examples to help you understand how to prevent such leaks in your applications.
Understanding Memory Management in Java
Java handles memory through its garbage collection (GC) mechanism. This system automatically deletes objects that are no longer in use, freeing up memory for new allocations. However, static fields maintain a reference for the entire lifetime of the application. As a result, if a static field points to an object that is no longer needed, that object will not be garbage collected, resulting in a memory leak.
Static Fields and Their Lifespan
Static fields belong to the class rather than to any instance of the class. This means they can be accessed without creating an object of the class, and they are allocated in the method area of the Java heap memory. Here’s a simple example:
public class StaticExample {
static String staticField = "I am a static field.";
public static void printStaticField() {
System.out.println(staticField);
}
}
In this code, staticField
is tied to StaticExample
and will persist as long as the application runs.
The Danger of Keeping References
Keeping references via static fields can inadvertently prevent the garbage collector from reclaiming memory. This is particularly dangerous when the static field refers to a large object or a collection of objects that, under normal circumstances, should not be held indefinitely.
Example of a Potential Memory Leak
Consider the following example, where a static field retains a reference to a large collection:
import java.util.HashMap;
import java.util.Map;
public class MemoryLeakExample {
private static Map<String, String> cache = new HashMap<>();
public static void addToCache(String key, String value) {
cache.put(key, value);
}
public static void clearCache() {
cache.clear(); // Not clearing the static reference itself.
}
}
In this scenario, cache
is a static field that grows as new entries are added through addToCache()
. While the clearCache()
method clears the map's contents, it doesn’t release the static reference itself. If a large number of entries are added, they will continue to occupy memory even if the application no longer needs them.
Why This Leads to a Leak
The above example reveals a subtle problem: even when there might be no references to the keys or values stored in cache
, the static field cache
itself remains alive within memory. Therefore, all previously cached data is never released. This situation is a classic case of a memory leak.
Real-World Case: User Sessions
A common scenario where static fields can lead to memory leaks is user session management. Let’s say we store user sessions using static fields; this can lead to issues, particularly in web applications:
import java.util.HashMap;
import java.util.Map;
public class UserSessionManager {
private static Map<String, UserSession> sessions = new HashMap<>();
public static void addSession(String userId, UserSession session) {
sessions.put(userId, session);
}
public static void removeSession(String userId) {
sessions.remove(userId); // This does not help unless explicitly called.
}
}
In this example, UserSessionManager
keeps track of user sessions in a static map. If users log in and out frequently, the application could end up with stale session objects bouncing around in memory unless removeSession
is called every time a user logs out. If it is frequently omitted due to bugs or neglect, this can lead to significant memory resource drain.
Monitoring for Memory Leaks
Java provides a native option to monitor memory usage through tools like Java VisualVM, Eclipse Memory Analyzer (MAT), or profiling tools like JProfiler. These can help developers visualize heap memory, track down leaks, and assess object retention.
To illustrate this with VisualVM:
- Run your application.
- Launch VisualVM and connect it to your Java process.
- Take a heap dump, which allows you to analyze all objects in memory.
This process helps determine whether static fields are the cause of a leak by shedding light on how much memory is allocated by these fields.
Preventing Memory Leaks from Static Fields
It is crucial to design your application carefully to avoid leaks when using static fields. Here are a few strategies to consider:
-
Nullify Static References: When an object is no longer needed, set static fields to
null
.☕snippet.javapublic static void removeSession(String userId) { sessions.remove(userId); if (sessions.isEmpty()) { sessions = null; // This helps to free up resources if applicable. } }
-
Weak References: Use
WeakHashMap
for cache-like structures to automatically enable garbage collection on entries that are only referenced weakly.☕snippet.javaimport java.util.Map; import java.util.WeakHashMap; public class WeakCacheExample { private static Map<String, String> cache = new WeakHashMap<>(); }
-
Avoid Storing Large Objects: Whenever possible, consider the size and lifespan of the data you intend to store. Avoid using static collections for objects that can be managed elsewhere.
-
Use Dependency Injection: Design your application to use instance variables instead of static fields wherever feasible, which can help mitigate potential leaks.
To Wrap Things Up
Static fields in Java can simplify code structure and improve convenience, but they come with significant risks concerning memory management. By maintaining a clear understanding of how static fields work and implementing best practices in your application design, you can prevent unwanted memory leaks.
Whether you are an experienced Java developer or a newcomer, understanding the implications of static fields and their impact on memory is essential for building efficient applications. Remember: while garbage collection helps, the responsibility lies in your hands to manage object lifetimes—static fields included.
For more information on Java memory management techniques, consider reviewing the Java documentation or exploring articles on memory optimization in Java.
Happy coding!