Java NIO vs IO: Unraveling Performance Pitfalls
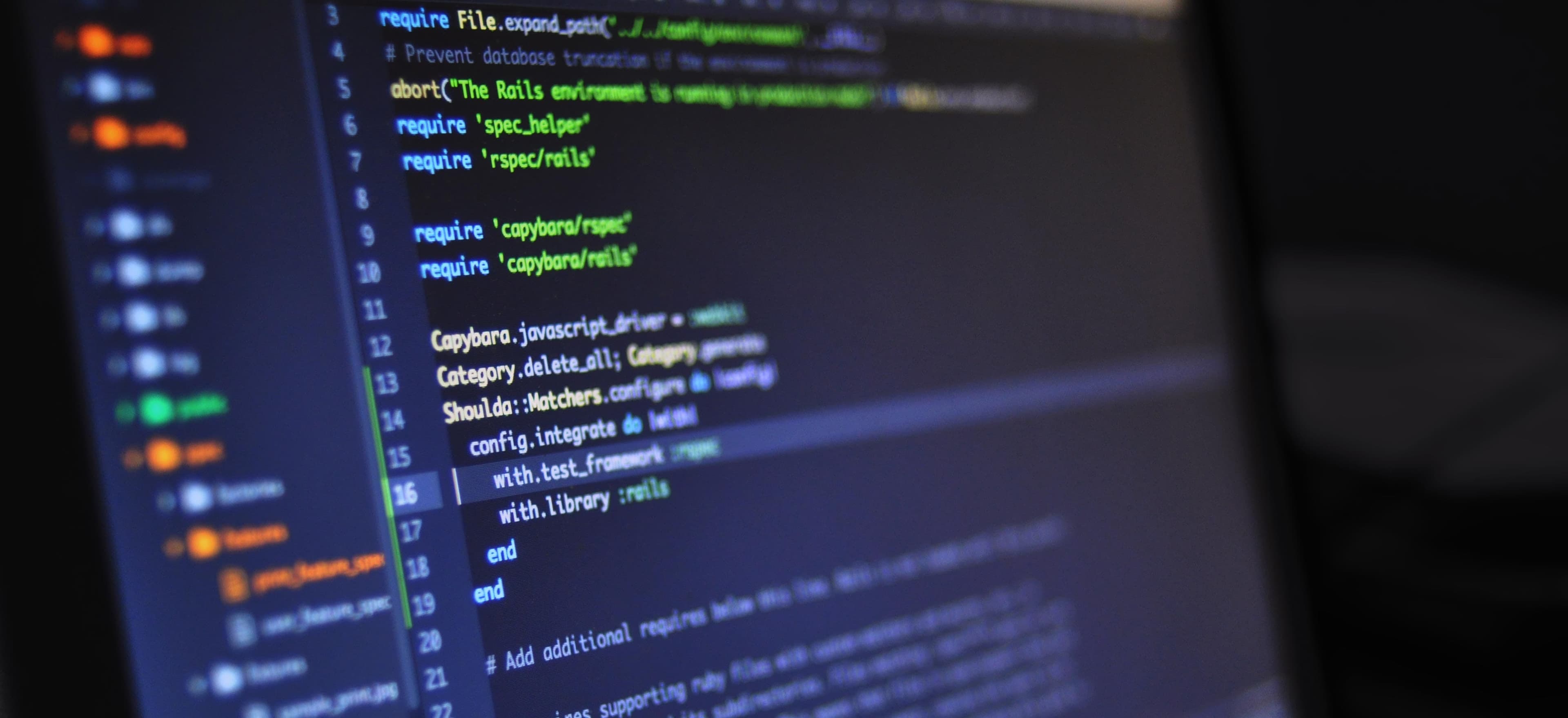
- Published on
Java NIO vs IO: Unraveling Performance Pitfalls
Java boasts a rich and robust set of APIs for handling input and output (I/O) operations. In the evolution of Java, two main I/O classes have gained attention: the traditional Java I/O (java.io) and the newer Java NIO (Java New Input/Output, java.nio). While both serve the primary purpose of data handling, they cater to different needs and use cases. In this article, we will explore the key differences between Java NIO and IO, their performance pitfalls, and which one might suit your needs.
Understanding Java I/O
The traditional Java I/O package, java.io
, is straightforward, offering a set of stream-based classes that work seamlessly for reading and writing data.
Key Features of Java I/O:
- Stream-Oriented: Java I/O is stream-oriented, which means it handles data sequentially.
- Blocking Calls: Operations like reading and writing are blocking; the thread waits until the operation is complete.
- Simplicity: The simplicity of
java.io
makes it beginner-friendly.
Example of Java I/O
Here's a basic example of how Java I/O reads a file:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class SimpleFileReader {
public static void main(String[] args) {
String filePath = "example.txt";
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary: In the above code, we utilize BufferedReader
and FileReader
to read text files. This example illustrates the simplicity of Java I/O, which makes it easy for developers to perform straightforward file read operations. However, when it comes to performance and efficiency under heavy loads or multiple clients, its blocking nature can become a bottleneck.
The Emergence of Java NIO
Java NIO was introduced in Java 1.4 as an alternative to traditional I/O. It is designed for scalability and performance, particularly in network applications.
Key Features of Java NIO:
- Non-Blocking I/O: NIO allows threads to initiate an I/O operation without waiting for it to complete. This can lead to higher resource utilization.
- Selectors and Channels: NIO introduces a ‘selector’ that can monitor multiple channels for events. This feature is particularly useful in server applications.
- Buffer-Oriented: NIO uses buffers to handle data, resulting in better performance for bulk data handling.
Example of Java NIO
Let's look at how to read a file using Java NIO:
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
import java.util.List;
public class NioFileReader {
public static void main(String[] args) {
String filePath = "example.txt";
try {
List<String> lines = Files.readAllLines(Paths.get(filePath));
lines.forEach(System.out::println);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary: In the above example, we leverage Java NIO's Files
and Paths
classes for reading files. Unlike java.io
, NIO’s file handling methods are more flexible and can read files in one go, particularly useful for processing larger files efficiently.
Performance Comparison: Java I/O vs NIO
Understanding performance is crucial when selecting an I/O approach. Let’s take a closer look at performance characteristics.
Throughput and Latency
- Java I/O typically performs well in simple applications with less data and fewer threads. However, it becomes a performance bottleneck in high concurrency scenarios due to its blocking nature.
- Java NIO, on the other hand, shines in high-volume, concurrent tasks. Its non-blocking architecture allows it to handle numerous connections efficiently, boosting throughput.
Memory Consumption
Java NIO is generally more memory-efficient than Java I/O for large-scale applications. This efficiency stems from its buffer-centric approach, which can reduce the number of object allocations.
Code Complexity
While Java I/O is simpler and easier to understand, Java NIO requires a deeper understanding of buffers, selectors, and channels, which can temporarily increase code complexity. However, the payoff can be significant in high-performance applications.
Use Cases: When to Use Each
-
Use Java I/O when:
- You have simple, straightforward I/O tasks.
- You are working in a lightweight application where performance is not a critical concern.
-
Use Java NIO when:
- Building high-performance network applications.
- You need to handle a large number of operations concurrently.
- You require non-blocking I/O for improved scalability.
Lessons Learned
When choosing between Java I/O and NIO, it's essential to consider your application's specific needs and performance requirements. While Java I/O is user-friendly and sufficient for simple tasks, NIO offers significant advantages in performance and scalability for more demanding applications.
As we look to the future, the relevance of Java NIO in building robust, high-performing applications is only set to grow. Understanding these frameworks equips developers with the knowledge to optimize their applications strategically.
To dive deeper into the practical applications of Java NIO, you can check out the official documentation here.
For further reading on asynchronous programming patterns, consider exploring the concepts of Reactive Programming and its implementations in Java.
Happy coding!
Checkout our other articles