Why Java String Interning Can Waste Memory
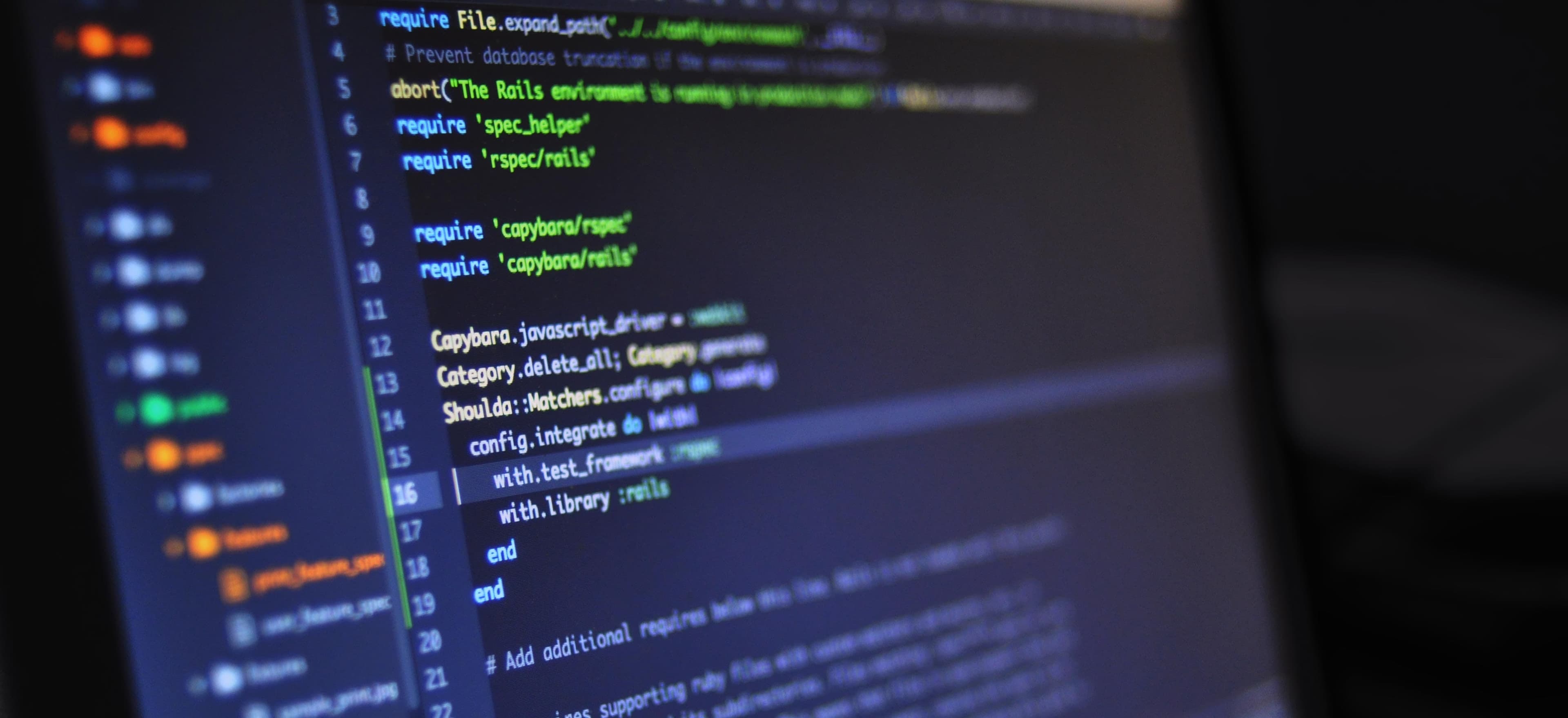
- Published on
Why Java String Interning Can Waste Memory
Java is well-known for its object-oriented structure and its robust handling of strings. In Java, strings are immutable, meaning once created, their values cannot be changed. However, for performance optimization, Java offers a feature known as string interning. This involves storing a single copy of each distinct string value in a special memory region called the "string pool." While this mechanism can save memory in some scenarios, it can also lead to unexpected memory consumption if not used judiciously.
Understanding String Interning
String interning is a method in Java that addresses the issue of duplicate string values being stored in memory. When strings are interned, they are stored in a special area of the heap called the string pool. This approach allows the JVM to not create new string instances for identical string literals; instead, they refer to the same instance.
Here's a simple example to illustrate the point:
String str1 = new String("Hello");
String str2 = new String("Hello");
String str3 = "Hello";
String str4 = "Hello";
System.out.println(str1 == str2); // false
System.out.println(str3 == str4); // true
In this example:
str1
andstr2
are separate instances, each occupying its own space in memory.str3
andstr4
point to the same interned string in the pool.
Why Use String Interning?
Interning can be beneficial in certain situations, particularly when dealing with a large number of strings that are duplicated. This can lead to significant memory savings.
- Memory Efficiency: If your application frequently uses the same string values, interning allows those strings to share a single instance.
- Performance: String comparisons using
==
can be faster when using interned strings because they compare references instead of creating new string objects.
When Can String Interning Waste Memory?
Despite its advantages, string interning can actually lead to increased memory consumption under certain conditions:
1. Excessive Number of Unique Strings
If your application produces a vast number of unique strings, one per runtime scenario, the string pool can grow very large. Consider the following code snippet:
List<String> uniqueStrings = new ArrayList<>();
for (int i = 0; i < 1000000; i++) {
uniqueStrings.add("String" + i.intern());
}
In the above example, you might expect to save memory by interning. However, due to the creation of such a large number of unique strings, you are actually consuming more space in the string pool than if you had allowed the garbage collector to clean up unneeded instances.
2. Long-Lived Applications
In long-lived applications, memory pressure from the string pool can accumulate over time. For instance, if you are performing operations on strings that lead to many interning calls, the memory usage may swell unnecessarily.
3. Switching Contexts Frequently
If your application frequently switches between different contexts, you may be interning strings that are only relevant for a brief period. This can lead to memory leakage, as those strings linger in the pool longer than necessary.
for (int i = 0; i < 100; i++) {
String tempStr = ("Temp" + i).intern();
// Do something with tempStr
}
// tempStr references linger despite not being used anymore
In this scenario, while tempStr
may take on values that are reused, they remain in memory, increasing the memory footprint of the application.
Effective Use of String Interning
To leverage string interning effectively, consider the following best practices:
1. Limit Interning to Constants
Use string interning primarily for constant values that are reused frequently throughout your application.
public static final String CONSTANT_STRING = "ConstantValue".intern();
2. Profile Memory Usage
Regularly monitor your application’s memory usage. Java profilers can help identify if string interning is contributing to increased memory consumption. Tools like VisualVM can help in diagnosing these situations.
3. Avoid Interning User Input
Never intern strings derived from user input. User-generated data is rarely duplicated and will only bloat the intern pool.
4. Remember Garbage Collection
Letting the garbage collector do its job is essential for managing memory. Overusing interning can lead to high memory pressure, impacting overall application performance.
A Final Look
While string interning in Java provides a mechanism for saving memory and improving performance in specific scenarios, using it indiscriminately can lead to increased memory consumption and other performance issues. Always profile your application and be mindful of when and how you use string interning. By following best practices, you can harness the benefits of string interning while mitigating its potential downsides.
For more about Java memory management and string handling, consider checking out the official Java documentation: Java SE Documentation.
Whether managing string literals or handling user input, understanding how string interning works helps developers make informed decisions that optimize their applications and maintain efficient memory usage. Happy coding!