Common JNI Pitfalls: How to Avoid Memory Leaks
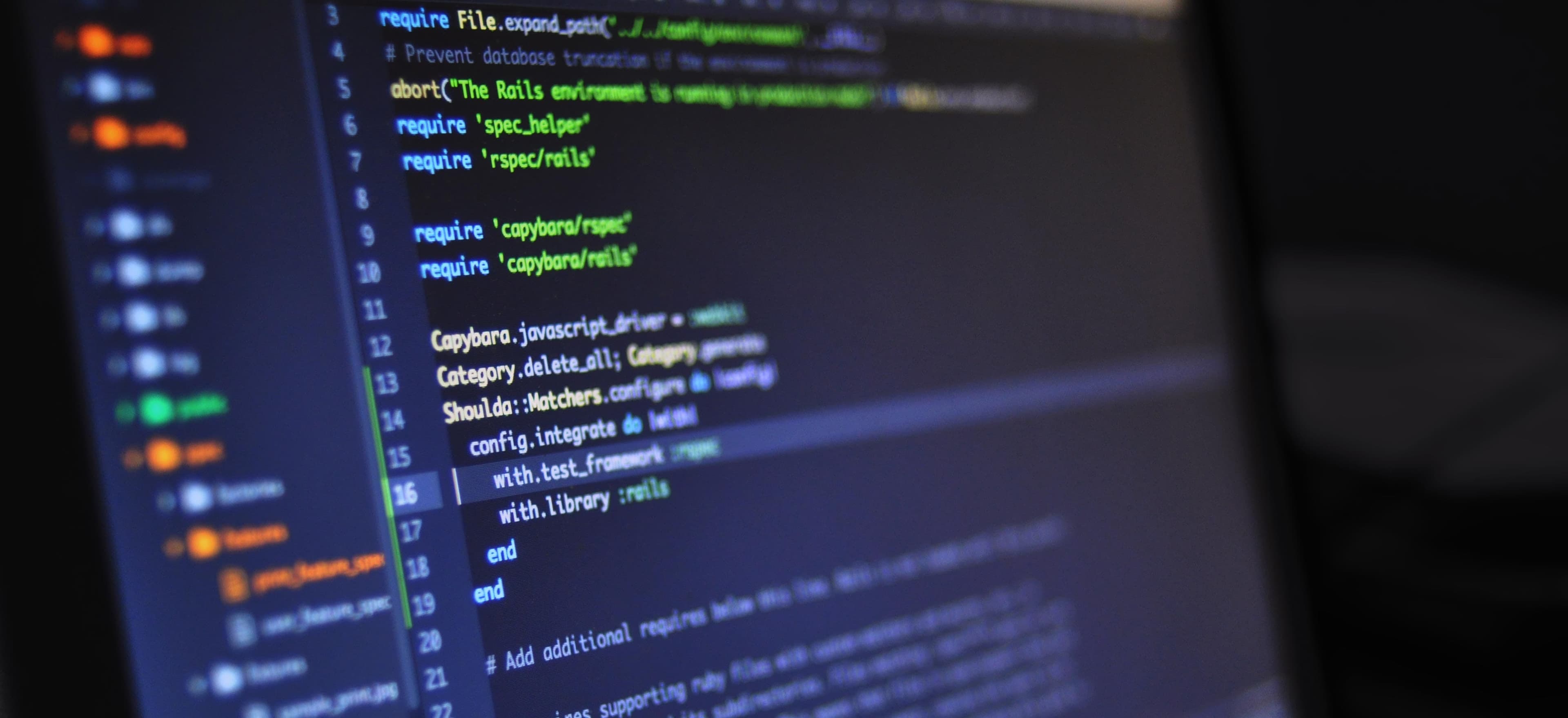
- Published on
Common JNI Pitfalls: How to Avoid Memory Leaks
Java Native Interface (JNI) provides Java applications the ability to call and be called by native applications and libraries, primarily written in C or C++. However, while JNI is a powerful feature, it comes with its own set of challenges, particularly regarding memory management. In this blog post, we will explore common JNI pitfalls that can lead to memory leaks and, most importantly, how to avoid them.
Understanding JNI and Memory Management
JNI serves as a bridge between Java and native languages. Java runs on a garbage-collected heap, while C and C++ manage their memory manually. This fundamental difference can lead to significant issues when Java objects are accessed by native code and vice versa. Failing to manage memory properly can result in memory leaks, leading to performance degradation and application crashes.
Key Concepts in JNI
- Local References: When a native method creates a new Java object, a local reference is generated. These references are automatically deleted when the native method returns.
- Global References: If you need a reference to a Java object beyond the lifespan of the local method, you need to create a global reference. However, this must be managed explicitly to avoid memory leaks.
- JNI Functions for Memory Management: JNI provides functions such as
NewGlobalRef()
,DeleteGlobalRef()
,NewLocalRef()
, andDeleteLocalRef()
that help manage these references.
Common JNI Pitfalls
1. Failing to Delete Local References
Local references are automatically cleaned up when a native method exits, but if you create many local references within a loop, you risk exceeding the JNI limit on local references and causing a memory leak.
JNIEXPORT void JNICALL Java_YourClass_yourMethod(JNIEnv *env, jobject obj) {
for (int i = 0; i < 1000; i++) {
jstring localStr = (*env)->NewStringUTF(env, "This is a local reference");
// Use localStr
} // The loop completes but localStr references are still on the stack.
}
Why Avoid This?
The above code will create 1000 local references without deleting them, potentially leading to memory issues. To properly manage memory, delete local references as shown below.
JNIEXPORT void JNICALL Java_YourClass_yourMethod(JNIEnv *env, jobject obj) {
for (int i = 0; i < 1000; i++) {
jstring localStr = (*env)->NewStringUTF(env, "This is a local reference");
// Use localStr
(*env)->DeleteLocalRef(env, localStr); // Clean up
}
}
2. Creating Global References Without Deletion
Global references last as long as the process runs, meaning that they can easily lead to leaks if not deleted. Every created global reference consumes memory, and failing to delete them can lead to exhausting the heap.
JNIEXPORT void JNICALL Java_YourClass_createGlobalRef(JNIEnv *env, jobject obj) {
jobject globalObj = (*env)->NewGlobalRef(env, obj); // Long-lasting reference
// Use globalObj
// No subsequent DeleteGlobalRef, which will lead to leaks
}
Why Avoid This?
If the method that creates these global references is called frequently, it can lead to high memory usage. You should always pair NewGlobalRef()
with DeleteGlobalRef()
.
JNIEXPORT void JNICALL Java_YourClass_createGlobalRef(JNIEnv *env, jobject obj) {
jobject globalObj = (*env)->NewGlobalRef(env, obj); // Create a global reference
// Use globalObj
(*env)->DeleteGlobalRef(env, globalObj); // Clean up
}
3. Memory Allocation in Native Code
When using native methods, memory management shifts from Java’s garbage collection to manual management. C and C++ use malloc()
or new
to allocate memory. If you forget to free this memory, it will lead to leaks.
JNIEXPORT void *allocateMemory() {
return malloc(100); // Memory allocated but not freed
}
Why Avoid This?
The above approach will leave the allocated memory unusable after your native calls are done. You must ensure that every allocation is paired with a corresponding free.
JNIEXPORT void *allocateMemory() {
void *ptr = malloc(100);
// Use ptr
free(ptr); // Clean up the allocated memory
}
4. Mismanagement of JNI Environment
The JNI environment pointer is not thread-safe and cannot be shared across multiple threads unless properly managed. Each thread should have its own JNI environment pointer obtained from AttachCurrentThread
.
JNIEnv *env;
// Assuming Multiple Threads
// You need to attach and detach them properly to avoid leaks.
Why Avoid This?
If multiple threads are involved, not managing the JNI environment correctly can lead to undefined behavior, including crashes or memory leaks. Ensure to attach and detach threads properly.
JNIEXPORT void JNICALL Java_YourClass_threadSafeMethod(JNIEnv *env, jobject obj) {
JNIEnv *threadEnv;
JavaVM *jvm; // Assume this is initialized somewhere
(*jvm)->AttachCurrentThread(jvm, (void**)&threadEnv, NULL);
// Do work with threadEnv...
(*jvm)->DetachCurrentThread(jvm); // Always detach the thread
}
5. Ignoring Exception Handling
When a JNI operation fails, an exception may be thrown, which can be ignored if you're not careful. This oversight could leave native-side resources unmanaged.
JNIEXPORT void JNICALL Java_YourClass_handleException(JNIEnv *env, jobject obj) {
jclass cls = (*env)->FindClass(env, "SomeClass");
if (cls == NULL) {
// Possible exceptions but not handled
}
}
Why Avoid This?
Ignoring exceptions can leave environments in an inconsistent state. Handling exceptions ensures your application remains robust.
JNIEXPORT void JNICALL Java_YourClass_handleException(JNIEnv *env, jobject obj) {
jclass cls = (*env)->FindClass(env, "SomeClass");
if (cls == NULL) {
(*env)->ExceptionCheck(env); // Check for exceptions
(*env)->ExceptionDescribe(env); // Print exception details
return; // Exit to avoid leaks
}
}
The Last Word
JNI is a powerful tool that, when used correctly, can enhance the performance and capabilities of Java applications. However, it requires meticulous memory management to avoid common pitfalls resulting in memory leaks. Following the best practices outlined in this post will go a long way in helping you use JNI effectively while maintaining optimal memory use.
For further reading on JNI and memory management, consider checking Oracle's JNI Documentation for expansive information. With the right knowledge and care, you can harness the full potential of JNI without succumbing to its challenges.
By focusing on proper memory management in JNI, you can avoid the debilitating effects of memory leaks and ensure the stability and efficiency of your applications. Happy coding!
Checkout our other articles