Mastering Java: Avoiding SQL Deadlocks in Your Applications
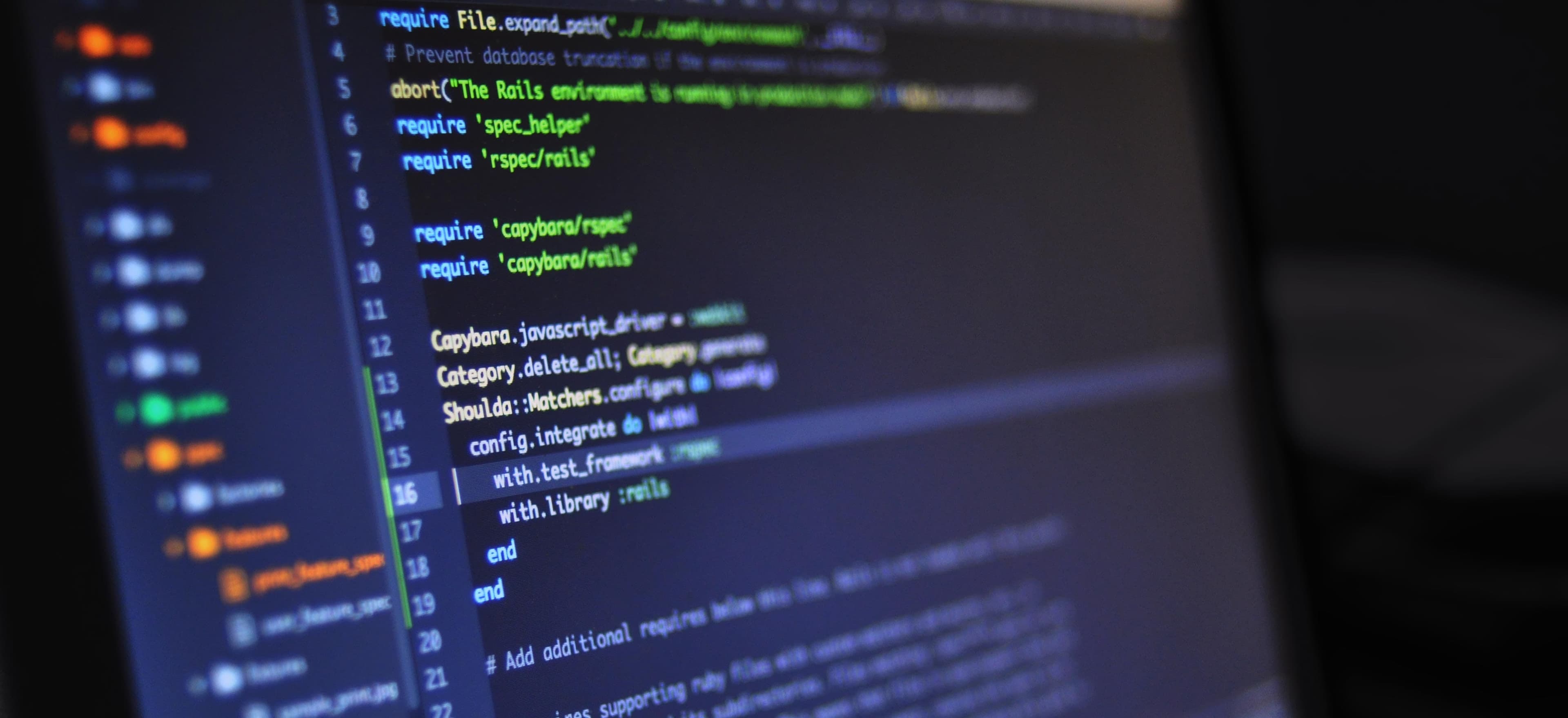
- Published on
Mastering Java: Avoiding SQL Deadlocks in Your Applications
When developing applications that interact with a relational database, you might occasionally encounter the dreaded SQL deadlock. Deadlocks can significantly hinder application performance and user experience, making it crucial for developers to understand how to avoid them. In this blog post, we will delve into the concept of SQL deadlocks, illustrate them with examples, and discuss strategies to prevent them in Java applications.
What is a Database Deadlock?
A deadlock occurs when two or more transactions are waiting for each other to release locks on resources, creating a situation where no transaction can proceed. Imagine a scenario where Transaction A holds a lock on Resource 1 and is waiting for a lock on Resource 2, while Transaction B holds a lock on Resource 2 and is waiting for the lock on Resource 1. Both transactions are in a stalemate, causing the database to lock indefinitely.
Example of a Deadlock
Let's consider an example using two simple SQL transactions:
-- Transaction A
BEGIN;
UPDATE Account SET balance = balance - 100 WHERE account_id = 1; -- Lock on Resource 1
UPDATE Account SET balance = balance + 100 WHERE account_id = 2; -- Wait for Resource 2
COMMIT;
-- Transaction B
BEGIN;
UPDATE Account SET balance = balance - 50 WHERE account_id = 2; -- Lock on Resource 2
UPDATE Account SET balance = balance + 50 WHERE account_id = 1; -- Wait for Resource 1
COMMIT;
In this example, if both transactions execute concurrently, they will wait on each other, resulting in a deadlock.
Detecting Deadlocks
Most modern relational database management systems (RDBMS) have an internal mechanism to detect deadlocks. When a deadlock is detected, the system typically rolls back one of the involved transactions, allowing the other transaction to continue.
In Java, you can handle these detection events gracefully using exception handling. Here's how to do it:
Example of Handling Deadlock in Java
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class BankTransaction {
public void transferMoney(Connection connection, int fromAccountId, int toAccountId, double amount) {
String query = "UPDATE Account SET balance = balance - ? WHERE account_id = ?";
String query2 = "UPDATE Account SET balance = balance + ? WHERE account_id = ?";
try {
connection.setAutoCommit(false);
try (PreparedStatement withdrawStatement = connection.prepareStatement(query);
PreparedStatement depositStatement = connection.prepareStatement(query2)) {
withdrawStatement.setDouble(1, amount);
withdrawStatement.setInt(2, fromAccountId);
withdrawStatement.executeUpdate();
// Simulate delay to increase chances of a deadlock
Thread.sleep(100);
depositStatement.setDouble(1, amount);
depositStatement.setInt(2, toAccountId);
depositStatement.executeUpdate();
connection.commit();
}
} catch (SQLException e) {
if (e.getSQLState().equals("40P01")) { // PostgreSQL deadlock
System.out.println("Deadlock occurred. Applying retry logic.");
// Implement a retry mechanism here
} else {
System.out.println("SQLException occurred: " + e.getMessage());
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
Why is Exception Handling Important?
Using exception handling allows your application to deal with unforeseen circumstances without crashing. In our example, if a deadlock is detected, the user is informed, and appropriate recovery steps can be taken, such as retrying the transaction.
Strategies for Avoiding Deadlocks
-
Consistent Lock Ordering: Always acquire locks in a consistent order. This reduces the chances of deadlocks by ensuring that if two transactions are trying to access multiple resources, they do so in the same sequence. For example, always lock Account 1 before Account 2.
-
Reduce Transaction Scope: Minimize the time length of transactions. By keeping transactions as short as possible, you reduce the window for deadlocks to occur.
-
Use Row-Level Locks: Instead of locking entire tables, utilize row-level locks when possible. This can significantly reduce contention between transactions, lowering the chances of deadlocks.
-
Retry Logic: Implement a retry mechanism when a deadlock is detected. By retrying the transaction after a brief wait, you can often succeed on subsequent tries.
-
Lock Timeout: Set a timeout for locks. If a transaction cannot acquire a lock within a specific timeframe, it will automatically be rolled back, reducing deadlock scenarios.
Example: Setting Lock Timeout in Java
Setting a lock timeout can be easily achieved in Java with JDBC. Here is an exemplary code snippet:
import java.sql.Connection;
import java.sql.SQLException;
import java.sql.Statement;
public class ConnectionUtil {
public static void configureConnection(Connection connection) throws SQLException {
// Set the lock timeout to 5 seconds
connection.createStatement().execute("SET lock_timeout = '5s'");
}
}
Wrapping Up
Deadlocks can be a critical issue in database-driven applications, leading to degraded performance and poor user experience. By understanding the nature of deadlocks and adopting preventive strategies, you can significantly reduce their occurrence in your Java applications.
As you build and maintain your applications, always be mindful of the ways in which your code can interact with the database and how to manage those interactions effectively. Implementing best practices, such as consistent lock ordering and robust exception handling, lays the groundwork for a smoothly running application.
For further reading, consider exploring SQL Server Deadlock and Advanced Java Database Concepts for deeper insights into managing database connections and error handling.
By mastering these techniques, you can effectively reduce the likelihood of encountering deadlocks and ensure your applications run smoothly. Happy coding!