Challenges of Java Lambda Serialization: What You Need to Know
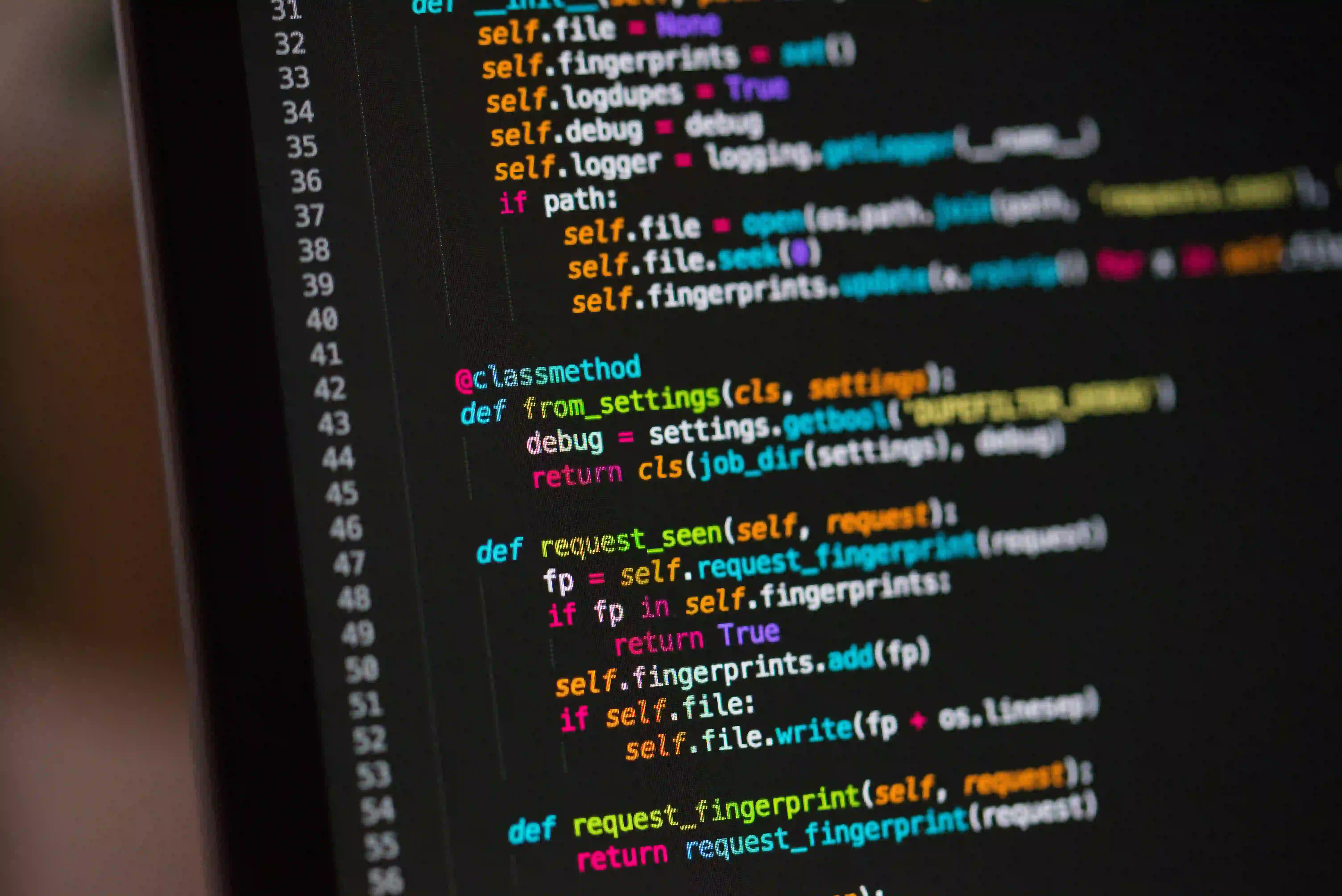
Challenges of Java Lambda Serialization: What You Need to Know
Java lambdas, introduced in Java 8, have transformed the landscape of Java programming by providing a clear and concise way to express instances of single-method interfaces (also known as functional interfaces). However, with the rise of lambda expressions comes unique challenges, especially concerning serialization. If you are a Java developer, understanding these challenges is crucial in ensuring the robustness and maintainability of your Java applications. In this post, we’ll dive deep into the intricacies of Java lambda serialization, highlighting important concepts and providing practical code examples along the way.
What is Serialization?
Serialization is the process of converting an object into a byte stream, enabling easy storage (on disk or in memory) or transmission (across networks). In Java, you can serialize an object by implementing the Serializable
interface. The desirable outcome? A serialized object can be deserialized back into the original object state.
import java.io.*;
public class SerializationExample implements Serializable {
private static final long serialVersionUID = 1L;
private String name;
public SerializationExample(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
// Serializing the object
SerializationExample example = new SerializationExample("Sample");
try (ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("example.ser"))) {
oos.writeObject(example);
}
// Deserializing the object
try (ObjectInputStream ois = new ObjectInputStream(new FileInputStream("example.ser"))) {
SerializationExample deserializedExample = (SerializationExample) ois.readObject();
System.out.println("Deserialized Name: " + deserializedExample.getName());
}
As demonstrated, serialization deconstructs an object into a stream of bytes. Similarly, deserialization reconstructs the object from this byte stream. Simple enough, right? However, complications arise when we bring lambda expressions into the conversation.
The Serialization of Lambda Expressions
Lambda expressions are often used for creating quick, functional interfaces without the boilerplate of anonymous inner classes. However, they are not straightforward to serialize. When a lambda is captured, its serialization does not inherently include information about the enclosing class or instance in which it was defined. This can lead to runtime exceptions if the environment changes or if the class is modified in a way that affects the lambda’s dependencies.
Challenge 1: Capturing Context
When a lambda expression captures certain variables from its context, it creates a closure. For serialization, this is a critical area of concern.
import java.io.Serializable;
public class LambdaSerialization {
public static void main(String[] args) {
final String greeting = "Hello";
// Lambda captures the context
SerializableFunction function = () -> System.out.println(greeting);
function.execute();
}
}
@FunctionalInterface
interface SerializableFunction extends Serializable {
void execute();
}
In this example, the lambda captures the variable greeting
. When serialized, it becomes challenging to reconstruct the environment surrounding that lambda. If greeting
was not serializable or if it changed after serialization, the deserialization would lead to issues.
Challenge 2: Non-Serializable Enclosures
If the lambda references non-serializable enclosing instances, it can also lead to runtime exceptions. For instance, consider the following adjustment to our earlier example:
class NonSerializable {
private String message = "Non-Serializable Context";
}
public class LambdaContextIssue {
public static void main(String[] args) {
NonSerializable nonSerializable = new NonSerializable();
// This will cause serialization issues
SerializableFunction function = () -> System.out.println(nonSerializable.message);
function.execute();
}
}
Here, nonSerializable
instance will trigger problems when attempting to serialize the lambda. The lambda cannot handle a non-serializable object, resulting in a java.io.NotSerializableException
.
Challenge 3: Inheriting Serialization Behavior
In Java, lambdas are compiled into a specific class that implements the functional interface. As such, if you ever modify or extend a class that contains lambdas, you may inadvertently change how that lambda functions, especially in terms of what it captures.
interface MyFunction extends Serializable {
void apply();
}
class MyClass {
private String name = "Default";
public MyFunction getFunction() {
return () -> System.out.println("Name: " + name);
}
public void setName(String name) {
this.name = name;
}
}
If later on you add properties or methods that change the nature of your MyClass
, the lambda may reference outdated or incorrect data. This leads to subtle bugs that are hard to track down and correct.
Solutions to Lambda Serialization Challenges
To navigate these serialization challenges, several strategies can be applied.
1. Use Static Methods for Lambdas
If lambdas don’t need to capture any local contextual variables, consider using static methods. Static methods do not depend on any instance context and remain serializable.
public class LambdaStaticExample {
public static void main(String[] args) {
MyFunction function = LambdaStaticExample::staticMethod;
function.apply();
}
public static void staticMethod() {
System.out.println("Static Method Called");
}
}
In this case, the method itself can be serialized without holding any instance variables, preventing context issues.
2. Ensure Captured Variables are Serializable
If you must capture variables from your context, ensure that they are serializable. This prevents NotSerializableException
during serialization.
class SerializableContext implements Serializable {
private String message = "Serializable Context";
}
class LambdaWithSerializableContext {
public static void main(String[] args) {
SerializableContext context = new SerializableContext();
SerializableFunction function = () -> System.out.println(context.message);
function.execute();
}
}
3. Externalize Context
Instead of allowing lambdas to capture local scope directly, you can pass required parameters through the method calls instead. This way, the lambda remains independent of the surrounding state.
public class LambdaParameterExample {
public static void main(String[] args) {
String message = "Passing Context Explicitly";
SerializableFunction function = () -> useMessage(message);
function.execute();
}
private static void useMessage(String msg) {
System.out.println(msg);
}
}
My Closing Thoughts on the Matter
Lambdas are a powerful addition to the Java toolbox, offering conciseness and functional programming capabilities. However, developers must be vigilant when it comes to serialization. The challenges associated with lambda serialization often lead to runtime errors and make code maintenance a headache.
By understanding the nuances of capturing context, ensuring serializability, and designing with serialization in mind, you can mitigate the risks associated with lambda serialization in your Java applications.
Further Reading:
Embrace the flexibility of lambdas while maintaining the integrity of your code. Happy coding!