Understanding Edge Cases in Java Float to Int Conversion
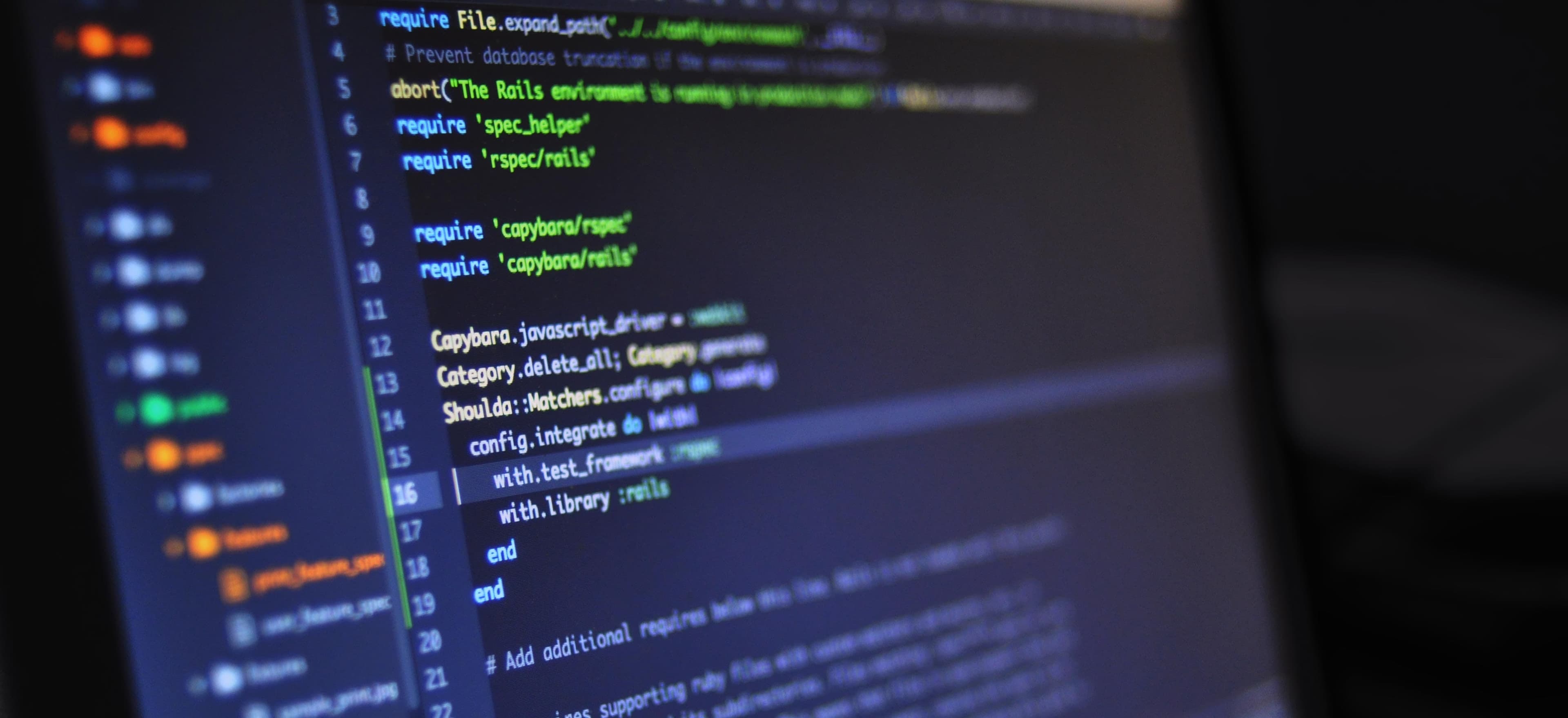
- Published on
Understanding Edge Cases in Java Float to Int Conversion
Java is an immensely popular programming language, primarily recognized for its portability, performance, and extensive libraries. However, like any programming language, it has its nuances and pitfalls—one of which is the conversion of floating-point values to integers. This blog post aims to elucidate what happens during the float to int conversion, focusing particularly on the edge cases that can lead to unexpected behavior.
The Basics of Float to Int Conversion
To begin, let’s consider the basic mechanism of converting a float
to an int
in Java. In programming, the term "conversion" refers to the process of changing a value from one data type to another. In Java, this typically involves narrowing conversions, where larger types must be converted to smaller types.
The float
type in Java is a 32-bit IEEE 754 floating-point. On the other hand, an int
is a 32-bit signed integer. When you convert a float
to an int
, Java will truncate the decimal part and only keep the integer portion, which may not always yield the desired results.
Basic Conversion Example
Here’s a simple example of converting a float
to an int
:
public class FloatToIntConversion {
public static void main(String[] args) {
float myFloat = 10.75f;
// Conversion
int myInt = (int) myFloat;
System.out.println("Float: " + myFloat);
System.out.println("Converted Integer: " + myInt);
}
}
Output:
Float: 10.75
Converted Integer: 10
In this example, the decimal portion .75
is discarded, resulting in the integer 10
. Such behavior may seem predictable, but it's crucial to understand the implications of this conversion, particularly when dealing with edge cases.
Understanding Edge Cases
Edge cases are scenarios that occur at the extreme operating parameters of a program. They might not ordinarily present themselves during routine operations, but they can lead to ambiguous and sometimes incorrect results.
Let's examine a few edge cases that can arise when converting float
values to int
.
1. Negative Floats
When converting a negative float, it's important to note how truncation behaves.
public class NegativeFloatExample {
public static void main(String[] args) {
float negFloat = -10.75f;
// Conversion
int negInt = (int) negFloat;
System.out.println("Negative Float: " + negFloat);
System.out.println("Converted Integer: " + negInt);
}
}
Output:
Negative Float: -10.75
Converted Integer: -10
In this case, the value -10.75
is truncated to -10
. It’s worth noting that truncation towards zero can sometimes be counterintuitive, especially for those unfamiliar with how negative values are treated.
2. Using Math.floor()
When you want to round down your numbers, consider using Math.floor()
before converting. Here's how it works:
public class FloorConversionExample {
public static void main(String[] args) {
float posFloat = 10.75f;
// Use Math.floor
int flooredInt = (int) Math.floor(posFloat);
System.out.println("Float: " + posFloat);
System.out.println("Floored Integer: " + flooredInt);
}
}
Output:
Float: 10.75
Floored Integer: 10
Interestingly enough, the outcome is the same in this case, but Math.floor()
can be useful for negative values.
3. Maximum and Minimum Float Values
Java allows large float values, which can lead to overflow when converting them to integers.
public class MaxFloatExample {
public static void main(String[] args) {
float maxFloat = Float.MAX_VALUE;
// Attempt conversion
int maxInt = (int) maxFloat;
System.out.println("Max Float: " + maxFloat);
System.out.println("Converted Integer: " + maxInt);
}
}
Output:
Max Float: 3.4028235E38
Converted Integer: 2147483647
Here, the output indicates that Float.MAX_VALUE
converts to the maximum int
value (Integer.MAX_VALUE
), demonstrating the phenomenon of overflowing a smaller data type.
To prevent this overflow, always check for potential limits before converting:
if (myFloat > Integer.MAX_VALUE) {
// Handle overflow
} else if (myFloat < Integer.MIN_VALUE) {
// Handle underflow
}
4. NaN and Infinity
Java also provides special float values such as NaN
(Not a Number) and Infinity
. Attempting to convert these values yields interesting results:
public class NaNInfinityExample {
public static void main(String[] args) {
float nanFloat = Float.NaN;
float infinityFloat = Float.POSITIVE_INFINITY;
// Conversion
int nanInt = (int) nanFloat;
int infinityInt = (int) infinityFloat;
System.out.println("NaN Converted Integer: " + nanInt);
System.out.println("Infinity Converted Integer: " + infinityInt);
}
}
Output:
NaN Converted Integer: 0
Infinity Converted Integer: 2147483647
For NaN
, the integer conversion yields 0
, whereas, for Infinity
, it results in Integer.MAX_VALUE
, even though the original value is not a finite number.
Best Practices for Float to Int Conversion
With all these edge cases and peculiarities in mind, it's prudent to adopt certain best practices:
- Always Check Values: Before performing any conversion, validate the float value to avoid use cases where overflow or unwanted truncation can occur.
- Use Rounding Functions: Leverage built-in functions like
Math.floor()
,Math.ceil()
, orMath.round()
to achieve the desired rounding behavior. - Null and Non-Integer Values: Handle potential null references or values that aren't integers explicitly, to avoid runtime exceptions.
Closing Remarks
The conversion from float to int in Java might seem straightforward, but various edge cases can lead to confusion and mistakes. By carefully examining how Java handles negative values, maximum and minimum limits, along with special float values like NaN and Infinity, developers can better manage this process.
Next time you find yourself converting float values, remember to implement checks and consider the implications—your code will be cleaner, more robust, and maintainable. If you’d like to delve deeper into type conversions in Java, consider checking out the official Java documentation.
Feel free to share your own experiences or questions regarding float to int conversions in Java in the comments below! Let’s continue to learn together.
Checkout our other articles