Reducing Overhead in Java Performance Logging
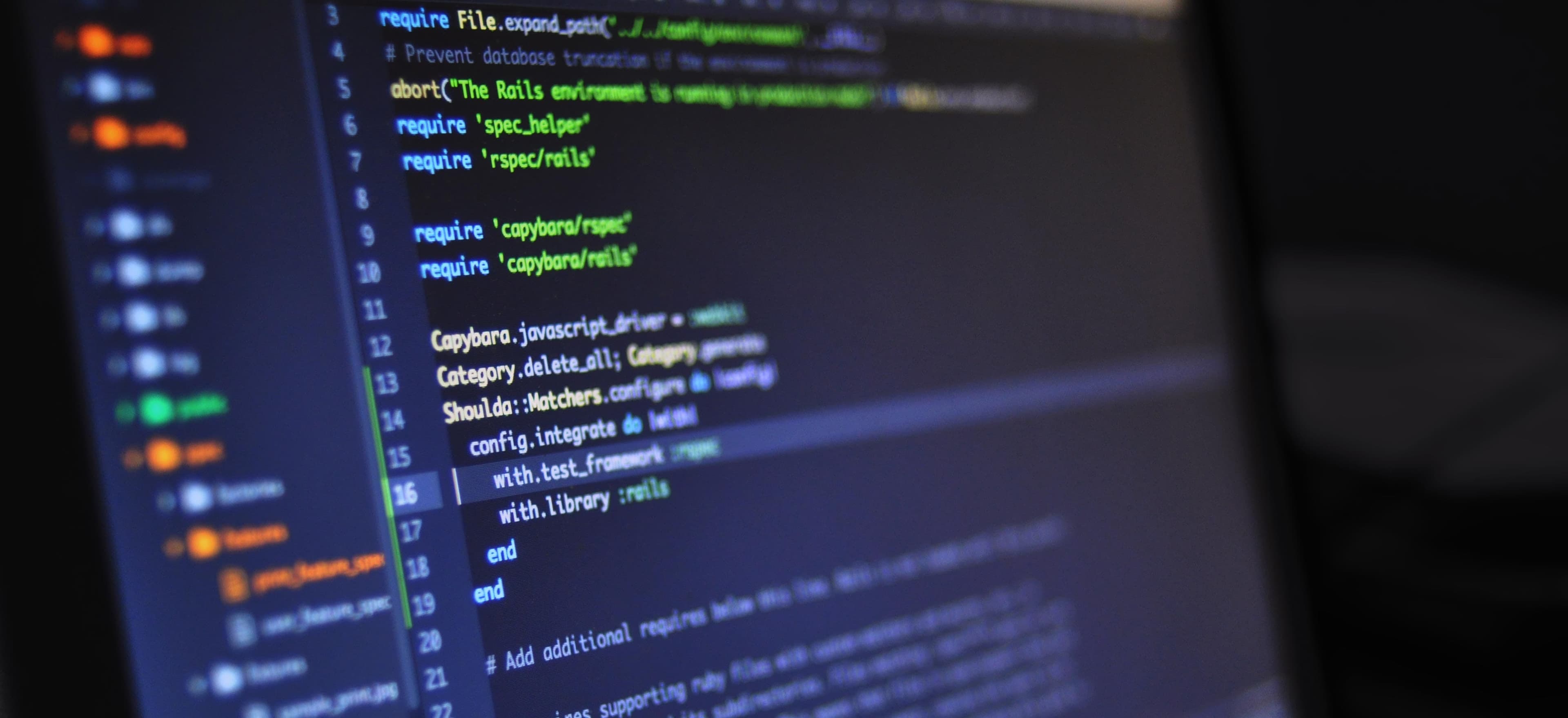
- Published on
Reducing Overhead in Java Performance Logging
Performance logging is a crucial aspect of managing applications, especially in Java, where performance bottlenecks can severely impact the user experience. However, logging can introduce significant overhead, especially if not managed properly. In this article, we will explore strategies to reduce overhead in Java performance logging, enhancing our application's efficiency without compromising the quality of information that logs provide.
Understanding Performance Logging
Before diving into optimization strategies, it’s essential to understand what performance logging entails. Performance logging typically includes:
- Execution Time: Measuring how long a function takes to execute.
- Resource Usage: Tracking memory and CPU usage.
- Error Rates: Monitoring error occurrences and frequency.
Why Optimize Performance Logging?
- Performance: Excessive logging can lead to degraded application performance.
- Storage: More logs consume more disk space and can lead to higher storage costs.
- Readability: Too many logs can obscure crucial information and make troubleshooting harder.
Strategies to Reduce Overhead in Java Performance Logging
1. Log Levels
Java's logging frameworks, such as Log4j and SLF4J, provide different logging levels: TRACE, DEBUG, INFO, WARN, ERROR, and FATAL. Strategically using these levels can help control the volume of logs.
Here’s an example of how to log using different levels in Java:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class PerformanceLogger {
private static final Logger logger = LoggerFactory.getLogger(PerformanceLogger.class);
public void performTask() {
long startTime = System.currentTimeMillis();
try {
// Task implementation
logger.info("Task started");
// Simulate work
Thread.sleep(500);
logger.info("Task completed");
} catch (Exception e) {
logger.error("Error during task execution", e);
} finally {
long duration = System.currentTimeMillis() - startTime;
logger.debug("Execution time: {} ms", duration); // Only logs if DEBUG level is enabled
}
}
}
Why this matters: By utilizing the correct log levels, you avoid logging detailed information in production unless necessary, thus minimizing overhead.
2. Conditional Logging
Sometimes, logging a significant amount of information can be costly. Conditional logging can help check whether a log level is enabled before constructing the log message:
if (logger.isDebugEnabled()) {
logger.debug("User data: {}", userData);
}
Why this matters: This approach prevents unnecessary string construction and reduces resource consumption if the log level is not set to DEBUG.
3. Asynchronous Logging
Synchronous logging blocks the execution thread, causing delays. Asynchronous logging, on the other hand, offloads the logging tasks to a separate thread, allowing your application to continue processing:
<AsyncLogger name="AsyncLogger" level="info">
<AppenderRef ref="Console" />
</AsyncLogger>
In Log4j2, configuring an AsyncLogger
is straightforward and can significantly improve performance.
Why this matters: By leveraging asynchronous logging, you reduce latency and improve application throughput.
4. Sampling Logs
Rather than logging every action, consider logging a sample of events. This can provide insights without overwhelming your logging system:
private int logCounter = 0;
public void performTask() {
logCounter++;
if (logCounter % 100 == 0) {
logger.info("Status update every 100 cycles");
}
}
Why this matters: Logging at a specific rate keeps the log manageable and still offers visibility into performance trends.
5. Log Aggregation and Analysis Tools
Utilizing tools such as ELK Stack (Elasticsearch, Logstash, and Kibana) or Prometheus can enhance your ability to analyze logs without needing to log everything in your application directly.
Why this matters: These tools can provide rich analytics without needing persistent high-volume logging in your application.
6. Use Efficient Data Formats
When logging performance data, consider using structured logging formats, such as JSON, which can be more efficient for parsing and analyzing logs:
import com.fasterxml.jackson.databind.ObjectMapper;
public void logPerformanceMetrics() {
Map<String, Object> metrics = new HashMap<>();
metrics.put("method", "performTask");
metrics.put("executionTime", 500);
ObjectMapper mapper = new ObjectMapper();
String json = mapper.writeValueAsString(metrics);
logger.info(json);
}
Why this matters: Structured logging allows for better searchability and a more efficient analysis of logs.
7. Limit Data Volume
Sometimes more isn’t better. Limiting the volume of logged data helps maintain performance. Rather than logging every detail, focus on key performance indicators (KPIs):
logger.info("Task execution time: {} ms", executionTime);
Why this matters: Focused logging means you’re capturing essential metrics without cluttering your logs.
The Closing Argument
Reducing overhead in Java performance logging is about finding the right balance between collecting important data and maintaining application performance. By implementing the strategies discussed—using log levels wisely, employing conditional logging, making logs asynchronous, and using efficient formats—you can create a robust logging system without compromising efficiency.
For further information on logging frameworks, consider checking Log4j or SLF4J. Implementing these practices can enhance your application's performance, giving you the insights you need while minimizing the impact on system resources.
Final Thoughts
Logging is a double-edged sword: while it’s essential for monitoring and troubleshooting, unoptimized logging can muddy the waters. Take the time to refine your logging strategy, and you’ll find a path that leads to both performance and transparency in your Java applications.
By leveraging best practices for performance logging and continuously assessing the logs produced by your applications, you can ensure that you're on a consistent path toward enhanced performance and reliability.
Checkout our other articles