Mastering Exception Handling in Java Multithreading
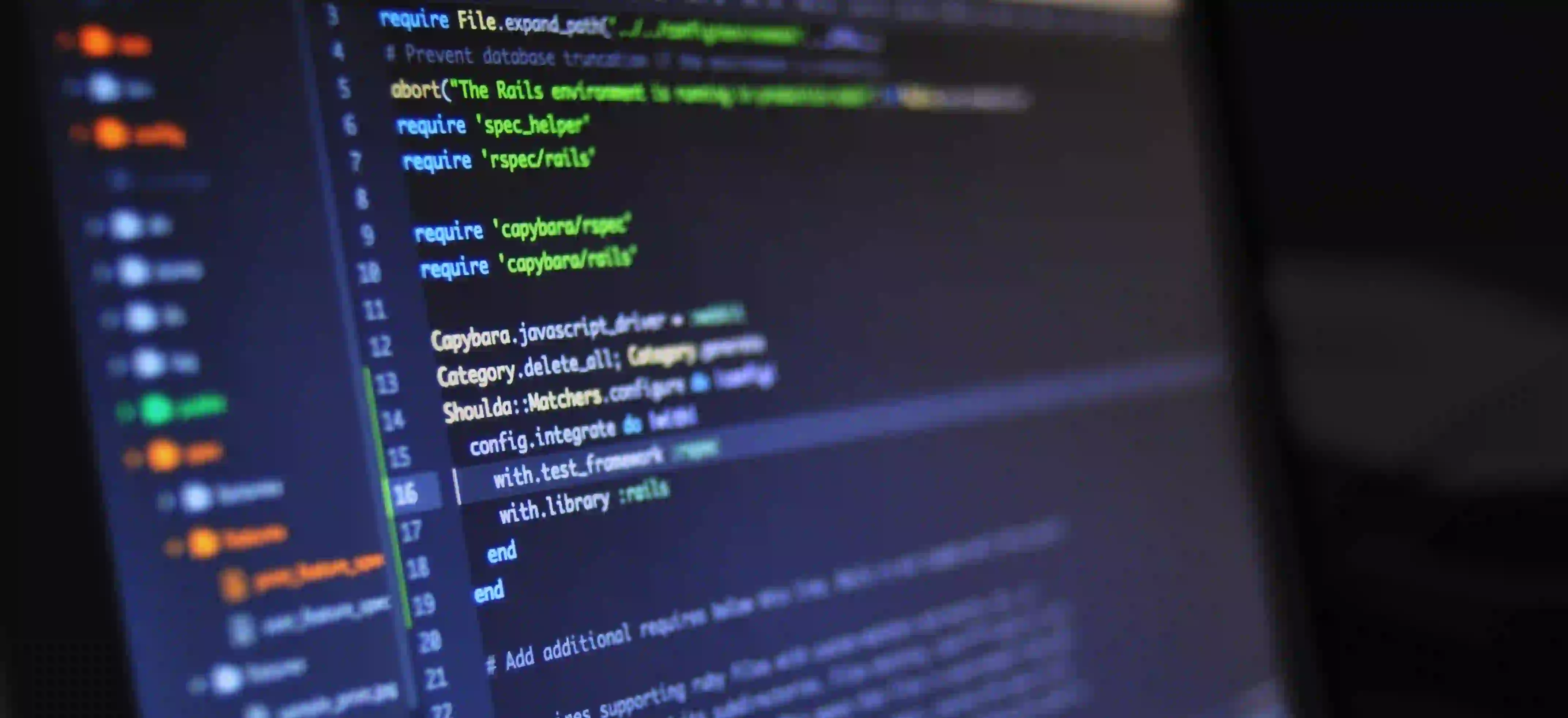
Mastering Exception Handling in Java Multithreading
In the realm of programming, exceptions are an inevitable part of the development process. In Java, managing exceptions in a multithreading context introduces a layer of complexity that demands careful consideration. In this post, we'll explore best practices for handling exceptions in Java threads, dive into relevant code snippets, and offer overarching guidelines that will take your understanding to new heights.
Understanding the Basics of Exception Handling
In Java, an exception is an event that disrupts the normal flow of a program’s execution. Exceptions in Java are classified into two main categories:
-
Checked Exceptions: These are exceptions that must be either caught or declared in the method where they might occur. Examples include
IOException
andSQLException
. -
Unchecked Exceptions: These are not required to be mentioned in a method's
throws
clause, and they usually signify programming errors. Examples includeNullPointerException
andArrayIndexOutOfBoundsException
.
It is important to understand these distinctions, as they guide how you will handle exceptions in a multithreaded environment.
Importance of Exception Handling in Multithreading
When multiple threads are in operation, the likelihood of encountering exceptions rises. Each thread operates independently and any uncaught exception in a thread can terminate that thread without affecting others. However, tracking these exceptions can become complex.
Why Handle Exceptions?
- Stability: An unhandled exception can lead to application crashes, undermining stability.
- Debugging: Well-logged exceptions can assist in easier debugging.
- User Experience: Proper exception handling allows for graceful degradation of service.
Best Practices for Exception Handling in Multithreading
1. Use Try-Catch Blocks within Threads
Each thread should handle its exceptions internally. By doing so, you can ensure that a single thread's failure does not derail the entire application.
class Task implements Runnable {
public void run() {
try {
// Your task logic goes here
System.out.println("Executing task...");
throw new RuntimeException("An error occurred.");
} catch (Exception e) {
System.err.println("Exception caught: " + e.getMessage());
// Log the error, perform cleanup, etc.
}
}
}
In the code snippet above, the Task
class implements Runnable
. The run
method contains a try-catch block that captures any exceptions occurring during execution. This approach ensures that exceptions are managed at the thread level.
2. Thread.UncaughtExceptionHandler
For uncaught exceptions in a thread, Java provides the UncaughtExceptionHandler
interface. This allows you to define what happens when a thread exits due to an uncaught exception.
class Task implements Runnable {
public void run() {
throw new RuntimeException("Unchecked Exception");
}
}
public class Main {
public static void main(String[] args) {
Thread thread = new Thread(new Task());
thread.setUncaughtExceptionHandler((t, e) -> {
System.err.println("Thread " + t.getName() + " threw exception: " + e.getMessage());
});
thread.start();
}
}
In the above example, the Task
class has a method that throws a RuntimeException
. By using the setUncaughtExceptionHandler
, we can specify a custom handler to deal with this exception, improving the clarity of error handling.
3. Propagate Exceptions with Future and Callable
When using the ExecutorService
, returning a Future
can be a valuable technique for propagating exceptions that occur within a thread.
import java.util.concurrent.*;
class CallableTask implements Callable<String> {
public String call() throws Exception {
throw new Exception("Simulated Exception");
}
}
public class Main {
public static void main(String[] args) {
ExecutorService executor = Executors.newSingleThreadExecutor();
Future<String> future = executor.submit(new CallableTask());
try {
System.out.println(future.get());
} catch (ExecutionException e) {
System.err.println("Caught exception: " + e.getCause().getMessage());
} catch (InterruptedException e) {
Thread.currentThread().interrupt(); // good practice to restore interrupt status
System.err.println("Thread was interrupted!");
} finally {
executor.shutdown();
}
}
}
In this example, the CallableTask
class implements Callable<String>
and throws an exception. By getting the result from the Future
object, we can handle the exception thrown during execution.
4. Log Exceptions
Logging exceptions is crucial for future analysis. Use logging frameworks like SLF4J or Log4j to effectively manage logging across your application.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
class Task implements Runnable {
private static final Logger logger = LoggerFactory.getLogger(Task.class);
public void run() {
try {
// Your task logic
throw new RuntimeException("An error during task execution");
} catch (Exception e) {
logger.error("Exception in Thread: ", e);
}
}
}
The above code leverages SLF4J to log exceptions in a structured and efficient manner. This practice can vastly improve the maintainability of your application.
5. Handling Thread Interruption
Be mindful of how your threads respond to interruptions; interruptions are a way to signal that a thread should stop what it is doing.
class InterruptibleTask implements Runnable {
public void run() {
try {
// Simulating work
for (int i = 0; i < 5; i++) {
Thread.sleep(1000);
System.out.println("Working...");
}
} catch (InterruptedException e) {
System.err.println("Task was interrupted");
// Optionally, restore the interrupt status
Thread.currentThread().interrupt();
}
}
}
In this example, the InterruptibleTask
class simulates a long-running task. We handle InterruptedException
by catching it and, if required, restoring the thread's interrupt status.
Lessons Learned
Mastering exception handling in Java multithreading is essential for building robust, maintainable applications. With well-structured code to handle exceptions, utilize logging, and communicate errors appropriately, you can provide a smooth user experience and maintain application stability.
By implementing these best practices, you’ll ensure that your multithreaded applications can gracefully handle disruptions while still delivering the intended functionality. Remember that thorough testing and logging will help you catch edge cases that you might not initially foresee.
For further reading, check out the official Java documentation on Exception Handling and the Java Concurrency Tutorials.
With a sound grasp of exception handling, your Java development skills will certainly flourish. Happy coding!