Mastering Delayed Tasks with Java ScheduledExecutorService
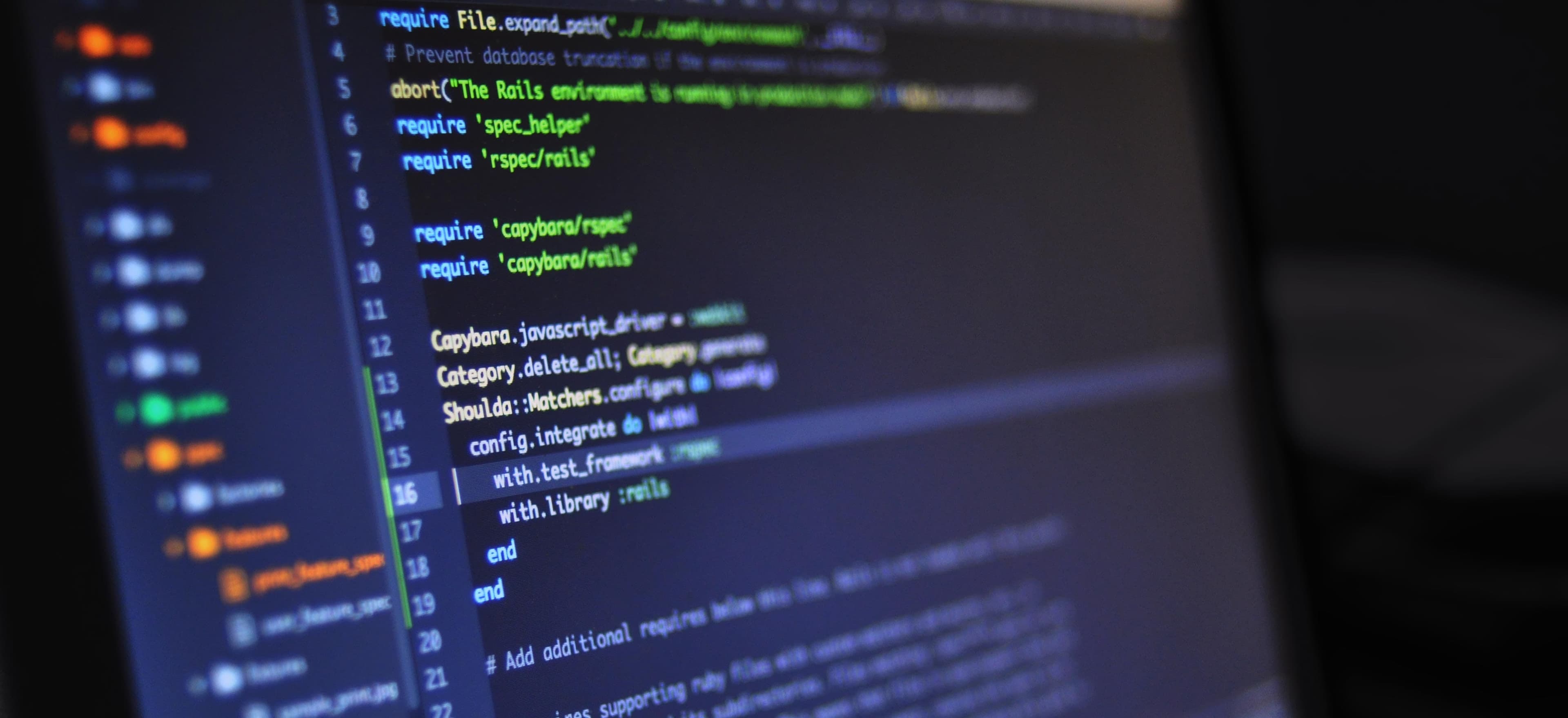
- Published on
Mastering Delayed Tasks with Java ScheduledExecutorService
In modern software development, managing tasks that need to run at scheduled times or with delays is a common requirement. Whether you're polling a server for data, scheduling reports, or simply planning periodic clean-ups of resources, having a robust mechanism to handle such tasks is crucial for your application's reliability and performance. In the Java ecosystem, the ScheduledExecutorService
offers a powerful way to handle scheduled and delayed tasks. This blog post will guide you through understanding and mastering ScheduledExecutorService
for smooth task management.
Beginning Insights to ScheduledExecutorService
ScheduledExecutorService is part of the Java Concurrency framework introduced in Java 5. It builds on the ExecutorService
interface, allowing you to schedule commands to run after a given delay or to execute periodically.
Key Features
- Flexible Scheduling: Unlike the
Timer
class, an instance ofScheduledExecutorService
can handle multiple scheduled tasks without risk of concurrency issues. - Task Management: You can easily manage the execution of delayed and periodic tasks.
- Future: It provides a way to handle results and exceptions related to the tasks you execute.
Setting Up ScheduledExecutorService
Let's start with a practical example of how to set up and use ScheduledExecutorService
.
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class ScheduledTaskExample {
public static void main(String[] args) {
// Creating a ScheduledExecutorService instance with a thread pool of size 1
ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(1);
// Defining a task to be executed
Runnable task = () -> System.out.println("Task executed at: " + System.currentTimeMillis());
// Scheduling the task to run after a delay of 5 seconds
scheduler.schedule(task, 5, TimeUnit.SECONDS);
// Scheduling a task to run at fixed rate of 2 seconds, with an initial delay of 1 second
scheduler.scheduleAtFixedRate(task, 1, 2, TimeUnit.SECONDS);
// Shutting down the executor after a certain time to clean up resources
try {
Thread.sleep(10000); // Main thread sleeping for 10 seconds
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
scheduler.shutdown();
}
}
}
Explanation of the Code
-
Executor Creation: We create a scheduled executor service with a thread pool of size one. This means only one task will run at any time.
-
Task Definition: We define a simple
Runnable
that logs the current time when executed. -
Task Scheduling: First, we schedule the task to run after a delay of 5 seconds. Second, we use
scheduleAtFixedRate
to run the task at a fixed rate (every 2 seconds), starting after an initial delay of 1 second. -
Shutdown: It's important to manage resources effectively. The
shutdown
method is called to terminate the executor service gracefully after the main thread sleeps for 10 seconds.
How to Optimize Scheduled Tasks
While the ScheduledExecutorService
is powerful, make sure to consider the following optimizations:
-
Thread Pool Size: Determine the appropriate size of the thread pool based on your tasks' nature. The
newScheduledThreadPool(ThreadPoolSize)
method helps adapt the necessary resources. -
Error Handling: Use try-catch blocks inside your runnable tasks to handle exceptions properly. The
ScheduledExecutorService
will terminate the task if an exception is thrown, and it won't automatically retry. -
Maintainability: Keep your
Runnable
tasks simple. If you need complex logic, consider breaking it down into smaller methods or classes.
Proper Error Handling Example
Here’s how you can implement error handling in your scheduled task:
Runnable taskWithHandling = () -> {
try {
// Simulating a task that may fail
if (Math.random() < 0.5) {
throw new RuntimeException("Random failure occurred!");
}
System.out.println("Task executed successfully at: " + System.currentTimeMillis());
} catch (Exception e) {
System.err.println("Error executing task: " + e.getMessage());
// Optionally log the error or take other actions
}
};
In the example above, errors are caught and logged without halting the execution of the scheduler. This is a good practice to maintain ongoing task execution flexibility.
Periodic Execution and Their Caveats
When using scheduleAtFixedRate
, it’s important to understand its behavior regarding task execution time. If a task execution exceeds the scheduled period, the next execution will start immediately after the previous one finishes. If the task duration is unpredictable, consider using scheduleWithFixedDelay
, which waits for the specified delay after the task has completed.
Example of scheduleWithFixedDelay
scheduler.scheduleWithFixedDelay(() -> {
System.out.println("Task with fixed delay executed at: " + System.currentTimeMillis());
}, 1, 2, TimeUnit.SECONDS);
In this example, if your task takes longer than 2 seconds, the next invocation will be delayed, ensuring that each task completes before starting a new one.
Real-World Applications
Let’s explore some real-world applications where ScheduledExecutorService
can be beneficial:
1. Data Polling
For applications that need to poll data from external services, such as APIs or databases, you can easily create a scheduled task that fetches data at defined intervals.
2. Resource Cleanup
An application may allocate resources like memory or file handles. Scheduling regular cleanup tasks can enhance performance and prevent issues, such as memory leaks.
3. Notification Systems
Scheduled tasks can be used to send notifications, reminders, or alerts periodically, which is essential for communication applications or task management software.
Learn More
For additional insights into Java concurrency, refer to the official Java Documentation here.
If you're just starting with Java's concurrency framework or want to improve your multi-threading skills, check out Java Concurrency in Practice for deeper knowledge.
Lessons Learned
Mastering ScheduledExecutorService
provides you a clear path to managing delayed and periodic tasks in your Java applications effectively. Its flexibility and robustness are essential tools for developers looking to improve their application's performance and reliability.
By understanding and implementing scheduling principles, error handling, and optimization strategies, you can ensure that your tasks run smoothly, and your application remains responsive.
With this foundational knowledge, you are equipped to take full advantage of the captivating world of concurrency in Java. Happy coding!