Understanding and Fixing Java's ConcurrentModificationException
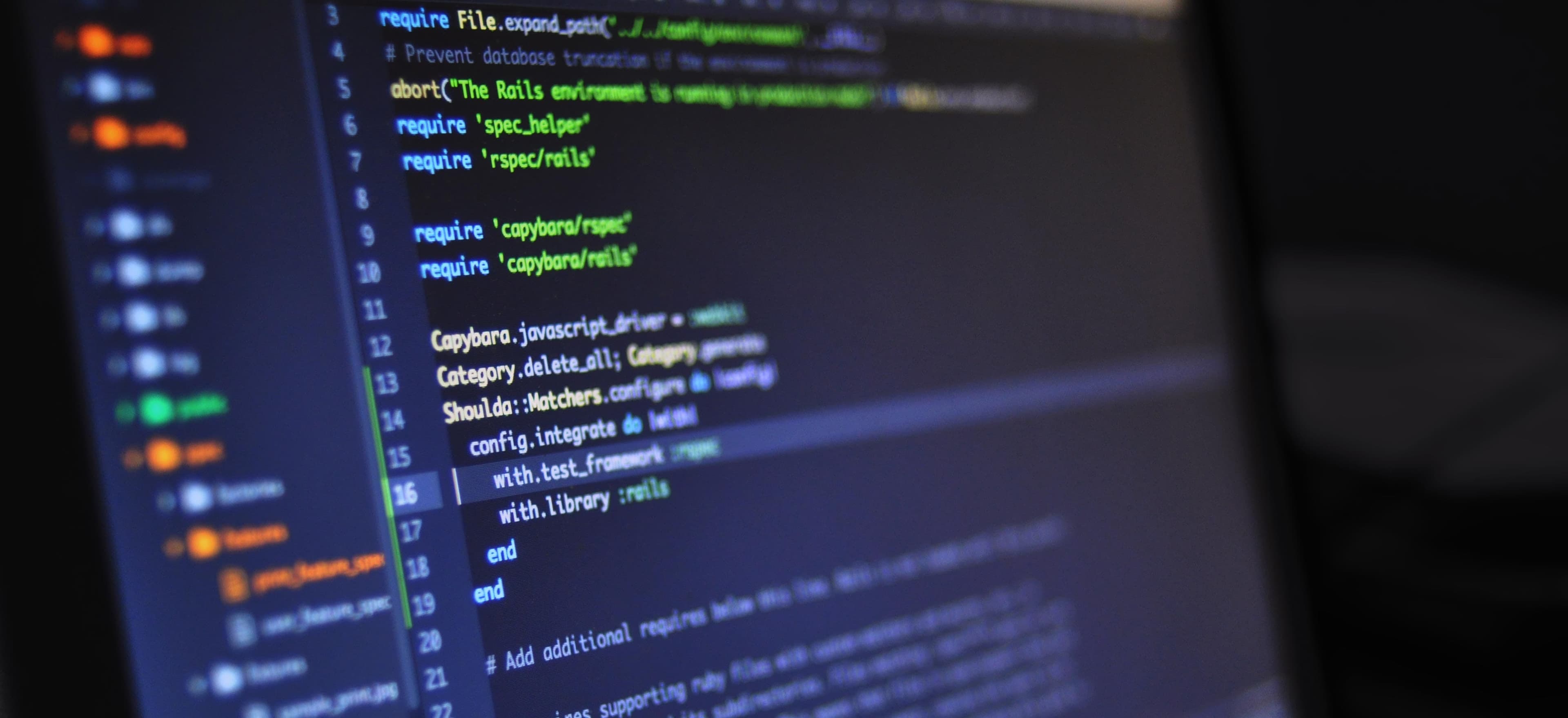
- Published on
Understanding and Fixing Java’s ConcurrentModificationException
Java is a powerful programming language that offers a rich set of data structures and algorithms. However, like all powerful tools, it comes with its challenges—one of which is the notorious ConcurrentModificationException
. In this blog post, we will delve into why this exception occurs, explore the scenarios that lead to it, and provide detailed solutions and best practices to avoid it.
What is ConcurrentModificationException?
The ConcurrentModificationException
is an unchecked exception that indicates that a thread has modified a collection while another thread is iterating over it. It's part of the Java Collections Framework and is thrown to prevent unexpected behaviors. This ensures that the integrity of the data structure is maintained during concurrent access.
When Does It Occur?
This exception typically occurs in the following scenarios:
- Fail-Fast Iterators: Java collections like
ArrayList
,HashMap
, and others, come equipped with fail-fast iterators. If a collection is modified while iterating over it (except through the iterator's ownremove
method), aConcurrentModificationException
is thrown.
List<String> list = new ArrayList<>();
list.add("One");
list.add("Two");
for (String item : list) {
if (item.equals("One")) {
list.remove(item); // ConcurrentModificationException
}
}
In this example, modifying the list while iterating through it leads to the exception.
- Multi-threaded Scenarios: When multiple threads access and modify a collection without proper synchronization, this exception can also occur.
List<Integer> list = new ArrayList<>();
list.add(1);
list.add(2);
Runnable task1 = () -> {
for (Integer num : list) {
System.out.println(num);
list.add(3); // ConcurrentModificationException
}
};
new Thread(task1).start();
Here, two threads may be trying to modify the collection at the same time.
How to Fix ConcurrentModificationException
1. Use Iterator's remove() Method
The simplest way to avoid this exception is to utilize the remove()
method provided by the iterator.
List<String> list = new ArrayList<>();
list.add("One");
list.add("Two");
list.add("Three");
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String item = iterator.next();
if ("Two".equals(item)) {
iterator.remove(); // Safe removal
}
}
System.out.println(list); // Output: [One, Three]
2. Copy the Collection
Another approach is to copy the contents of your collection into a new one before iterating through the original. This way, you can avoid concurrent modification by only operating on the copy.
List<String> list = new ArrayList<>();
list.add("One");
list.add("Two");
for (String item : new ArrayList<>(list)) {
if ("One".equals(item)) {
list.remove(item); // No Exception
}
}
System.out.println(list); // Output: [Two]
3. Use Concurrent Collections
If you are working in a multi-threaded environment, consider using collections designed for concurrent access. Java’s java.util.concurrent
package provides several thread-safe collections, such as ConcurrentHashMap
and CopyOnWriteArrayList
.
List<String> list = new CopyOnWriteArrayList<>();
list.add("One");
list.add("Two");
for (String item : list) {
if ("One".equals(item)) {
list.remove(item); // No Exception
}
}
System.out.println(list); // Output: [Two]
4. Synchronized Blocks
Another option is to provide synchronization around the collection. Ensure that only one thread accesses the collection at a time.
List<String> list = Collections.synchronizedList(new ArrayList<>());
list.add("One");
list.add("Two");
synchronized (list) {
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String item = iterator.next();
if ("One".equals(item)) {
iterator.remove(); // Safe removal
}
}
}
System.out.println(list); // Output: [Two]
Best Practices to Avoid ConcurrentModificationException
-
Understand When Your Collection May Change: Always examine scenarios where collections might be modified, especially in loops or multi-threaded environments.
-
Use the Right Collection: Choose collections based on your needs. For sequential access with frequent modifications, prefer collections from the
java.util.concurrent
package. -
Prefer Immutability: If your collection doesn't need to change, consider using immutable collections. This prevents modifications that could lead to exceptions.
-
Unit Testing: Write unit tests that simulate concurrent access to your collections. This practice can help catch potential issues early.
-
Review Code Regularly: Code reviews and pair programming can help identify areas prone to
ConcurrentModificationException
. Getting a second pair of eyes on the code could reveal conflicts.
In Conclusion, Here is What Matters
The ConcurrentModificationException
is a clear example of Java’s philosophy: safety first. Understanding when and why this exception occurs is key to effective programming. By adhering to the practices discussed, you can avoid these pitfalls and write cleaner, safer Java code.
For further reading, you might enjoy the official Java Tutorials or delve deeper into concurrency in Java in Effective Java by Joshua Bloch.
Happy coding!