Understanding Volatile: Memory Consistency Issues in Java
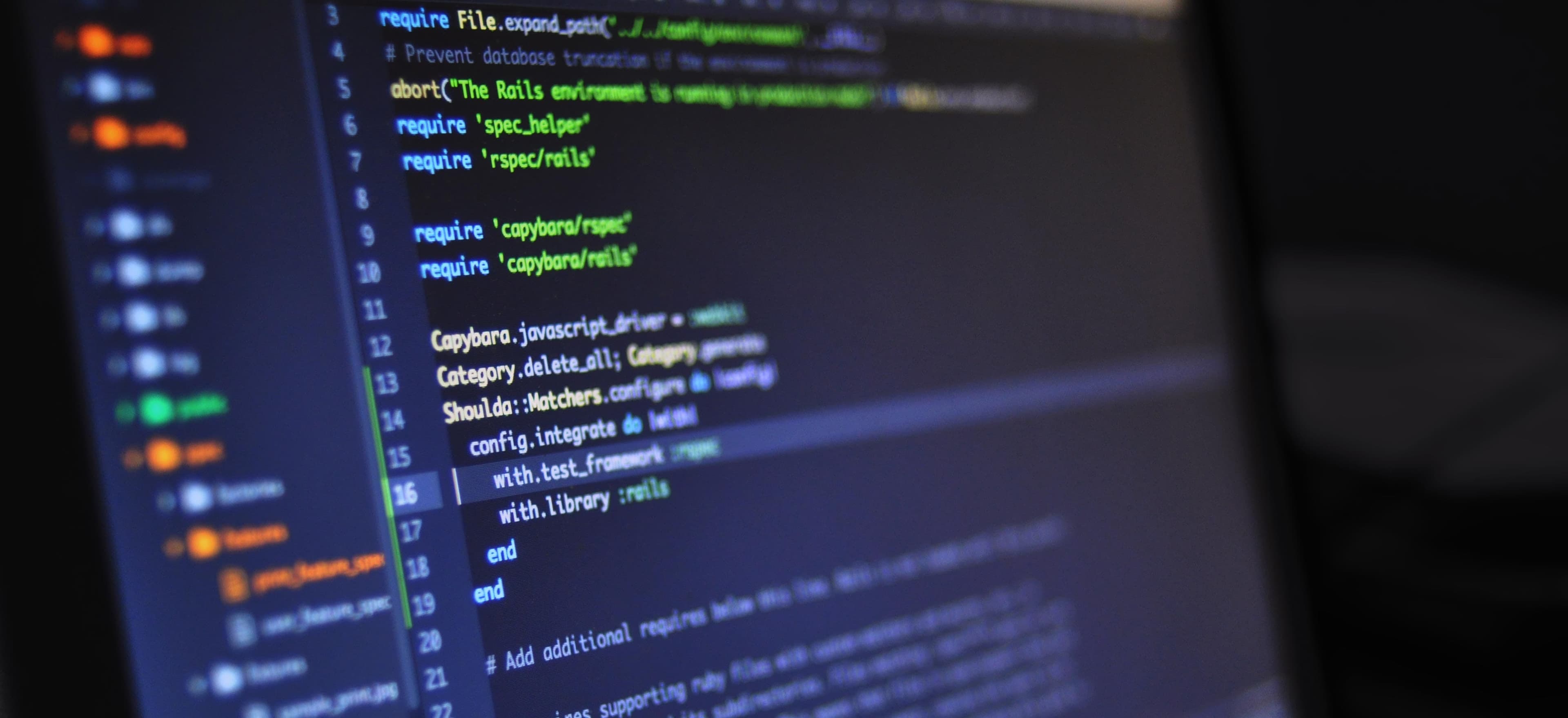
- Published on
Understanding Volatile: Memory Consistency Issues in Java
In the vast realm of Java programming, performance optimizations and memory management strategies are pivotal. One such strategy that often generates interest and questions is the volatile
keyword. This blog post delves deep into the concept of volatile
, what it means for memory consistency, and how it can affect the performance of your Java applications.
What is volatile
?
In Java, the volatile
keyword is a modifier that can be applied to instance variables to indicate that these variables can be accessed by multiple threads. Declaring a variable as volatile
ensures that any read or write to that variable occurs directly from the main memory rather than a thread-local cache. This is crucial for multi-threading environments where consistency is key.
Why Use volatile
?
The primary reason to use the volatile
keyword is to maintain visibility and ordering guarantees across threads. In multi-threaded applications, threads may cache variables in their local memory. Without proper synchronization, modifications made by one thread may not be visible to others, leading to unpredictable behavior.
When a variable is declared as volatile
:
- Visibility: Any changes made to a
volatile
variable by one thread are visible to all other threads. It prevents caching of the variable. - Ordering: It establishes a happens-before relationship, ensuring that all operations prior to writing to the
volatile
variable will be visible after a read of that variable.
The Memory Consistency Errors
Memory consistency errors occur when threads have inconsistent views of what should be shared variables. Consider the following simple example:
class SharedResource {
private boolean flag = false;
public void writer() {
flag = true; // Write
}
public boolean reader() {
return flag; // Read
}
}
In the above code, a thread could potentially read flag
as false
even after writer
has been executed in another thread because of caching and optimization done by the JVM. This is a classic case of memory consistency issues.
How volatile
Resolves the Issue
Introducing volatile
can change the scenario significantly. Here’s how the code would look:
class SharedResource {
private volatile boolean flag = false;
public void writer() {
flag = true; // Write
}
public boolean reader() {
return flag; // Read
}
}
With the use of the volatile
keyword:
- The
flag
variable is guaranteed to reflect the most recent write by any thread. - The Java Memory Model ensures a stronger guarantee that changes to
flag
made by thewriter
are visible to thereader
.
Limitations of volatile
However, using volatile
comes with its own set of limitations. It's important to recognize when volatile
is appropriate, and when a synchronized approach may be more beneficial.
-
Atomicity: While
volatile
provides visibility guarantees, it does NOT guarantee atomicity. For example, the following increment operation is NOT atomic:public void increment() { count++; // Not atomic if 'count' is a regular variable }
Instead, consider using
AtomicInteger
, for atomic operations without the need for synchronization:import java.util.concurrent.atomic.AtomicInteger; class SharedNumber { private AtomicInteger count = new AtomicInteger(0); public void increment() { count.incrementAndGet(); // Atomic } }
For more on atomic variables, refer to Java's Atomic Classes.
-
Mutable Objects: Using
volatile
on mutable objects (like lists, maps, etc.) does not provide any guarantee regarding the mutability of the objects themselves. For example:private volatile List<String> list = new ArrayList<>(); public void addItem(String item) { list.add(item); // Not synchronized, potential inconsistency }
In such cases, consider using
Collections.synchronizedList
to ensure full thread safety when modifying the contents of the list. -
No Locking Mechanism:
volatile
does not provide any locking, meaning that if multiple threads are writing or reading at the same time, you might still encounter issues. It's essential to choose between locks andvolatile
based on the use case.
When to Use volatile
vs. Synchronized
The choice between volatile
and synchronized
can be clarified by examining their differences:
-
Use
volatile
when:- A variable is being shared between threads and you only need visibility guarantees without the need for atomic operations.
- Example: Flags, completion signals.
-
Use
synchronized
when:- You are working with compound actions (like check-then-act, increment operations, etc.) where a single atomic operation is needed.
- Example: Complex critical sections that require consistent state updates.
Best Practices
Here are some best practices for using volatile
effectively in Java:
-
Use for simple flags and state indicators: If your use case involves flags or state indicators that are simply set and read by multiple threads,
volatile
fits perfectly. -
Do not replace
synchronized
entirely: Whilevolatile
can prevent some memory consistency issues, it is not a substitution forsynchronized
. -
Make reading and writing simple: Avoid using
volatile
with complex structures. Use it with simple types or references that don’t require compound actions. -
Document your decision: When using
volatile
, it’s a good idea to document the reasoning behind your choice for team members and future maintainers.
The Closing Argument
Understanding how the volatile
keyword works and when to use it can significantly improve your multi-threaded Java applications. It provides a lightweight mechanism for ensuring visibility without the overhead of thread locks in scenarios that require very simple thread coordination.
However, it's essential to remember its limitations. By employing volatile
appropriately and in the right context, you can enhance the reliability and performance of multi-threaded applications.
For more complex threading solutions and patterns in Java, consider checking out Java Concurrency in Practice, a key resource for mastering concurrency in Java.
Additional Resources
- Java Memory Model Specification
- Understanding the Java Memory Model
By designing with volatile
, you'll not only improve your application’s thread safety but also lay a solid foundation for fine-tuned performance. Happy coding!