Navigating the Java Diamond Problem in Inheritance
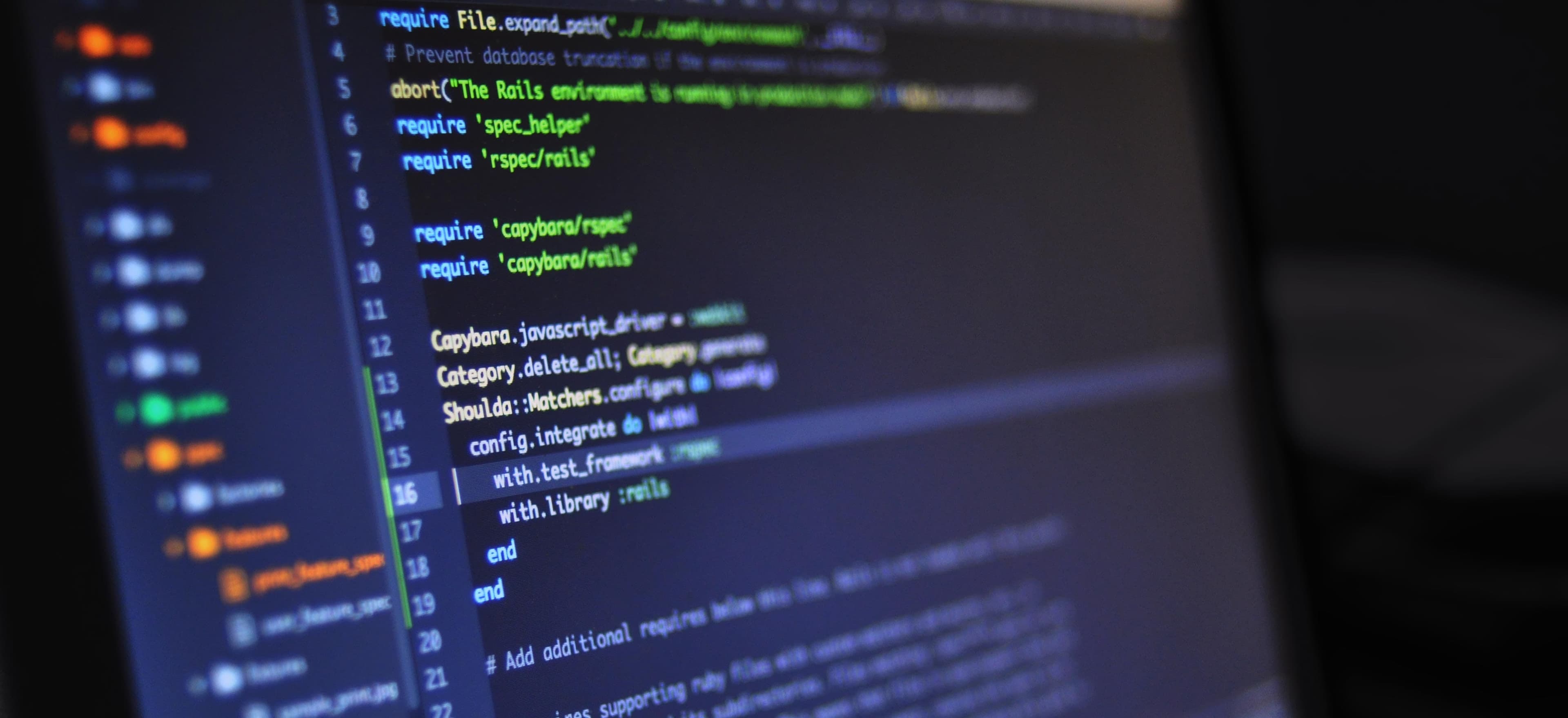
- Published on
Navigating the Java Diamond Problem in Inheritance
Diving Into the Subject
Java is a powerful, versatile programming language that has been a staple in the software development community for decades. Its object-oriented nature leads to hierarchical relationships between classes, which often promotes code reuse and modular design. However, this approach sometimes gives rise to complications, particularly with inheritance. One of the most notorious of these complications is the Diamond Problem. In this post, we will explore the concept of the Diamond Problem in Java inheritance, its implications, and how to resolve it.
Understanding the Diamond Problem
The Diamond Problem occurs when a subclass inherits from two classes that have a common ancestor. This creates an ambiguity as the compiler struggles to ascertain which superclass method should be called. This is best illustrated with an example:
class A {
void display() {
System.out.println("Display from Class A");
}
}
class B extends A {
void display() {
System.out.println("Display from Class B");
}
}
class C extends A {
void display() {
System.out.println("Display from Class C");
}
}
// The problem arises here:
class D extends B, C {
// Which display() to inherit?
}
In the snippet above, Class D extends both Class B and Class C, which in turn extend Class A. When calling the display()
method from an instance of D
, it is unclear whether to execute B's version or C's.
Why is this an Issue?
This ambiguity becomes a significant problem, particularly in larger codebases where maintaining clarity and predictability is paramount. If the Java compiler cannot determine which superclass method should be utilized, it will result in a compilation error, making code difficult to manage and understand.
Java's Approach to the Diamond Problem
Java has a unique approach to avoid the Diamond Problem—it does not allow multiple inheritance for classes. Rather, Java allows a class to inherit from only one superclass. However, it allows a class to implement multiple interfaces, which leads us to another aspect of the Diamond Problem.
Example with Interfaces
In Java, you can implement multiple interfaces, which may introduce a different form of the Diamond Problem. Below is an illustrative code snippet:
interface A {
default void display() {
System.out.println("Display from Interface A");
}
}
interface B extends A {
default void display() {
System.out.println("Display from Interface B");
}
}
interface C extends A {
default void display() {
System.out.println("Display from Interface C");
}
}
// In this example, D faces the diamond problem
class D implements B, C {
@Override
public void display() {
// Need to explicitly call one of the super display methods
B.super.display(); // Calls display method from B
// C.super.display(); // Uncommenting this would call display from C
}
}
In this case, when D
implements both interfaces B and C, each of them provides a default implementation of the display()
method. To resolve this ambiguity, D
is required to override the display()
method and explicitly choose which superclass version to call.
Why Use Interfaces?
Opting for interfaces over classes provides underlying benefits, such as:
- Flexibility: Allows multiple inheritance of types while avoiding the complexities of state.
- Enforcement of Contracts: Ensures that derived classes adhere to a specified behavior.
- Default Methods: With Java 8 and above, interfaces can have default methods, allowing for a form of code reuse.
Handling the Diamond Problem in Java
When facing the Diamond Problem in Java, follow these strategies:
-
Single Inheritance for Classes: Stick to single inheritance for parent classes while leveraging interfaces for shared behavior.
-
Explicit Overriding: Always override methods when implementing multiple interfaces that may conflict. This ensures clarity about what behavior is being implemented.
-
Clear Documentation: Document the reasoning behind design choices, especially if multiple interfaces are being used. This helps future developers understand the structure quickly.
-
Code Reviews: Regularly conduct code reviews to ensure that potential diamond problems are identified during the design phase.
Bringing It All Together
Navigating the Diamond Problem in Java requires a solid understanding of inheritance principles. By leveraging Java's model of single inheritance while effectively utilizing interfaces, developers can design robust, modular, and clear object-oriented applications.
While this aspect of Java might initially seem complex, understanding it strengthens one's grasp of object-oriented programming principles and enhances your ability to write well-structured code.
For further reading, consider referring to Java's Official Documentation or exploring additional patterns such as Composition over Inheritance, which can also provide viable design alternatives in different contexts.
By implementing the strategies outlined above and staying at the forefront of best practices, you can confidently navigate the intricacies of the Java Diamond Problem and enhance your coding expertise. Happy coding!
Checkout our other articles