Understanding Leap Seconds in Java Time API
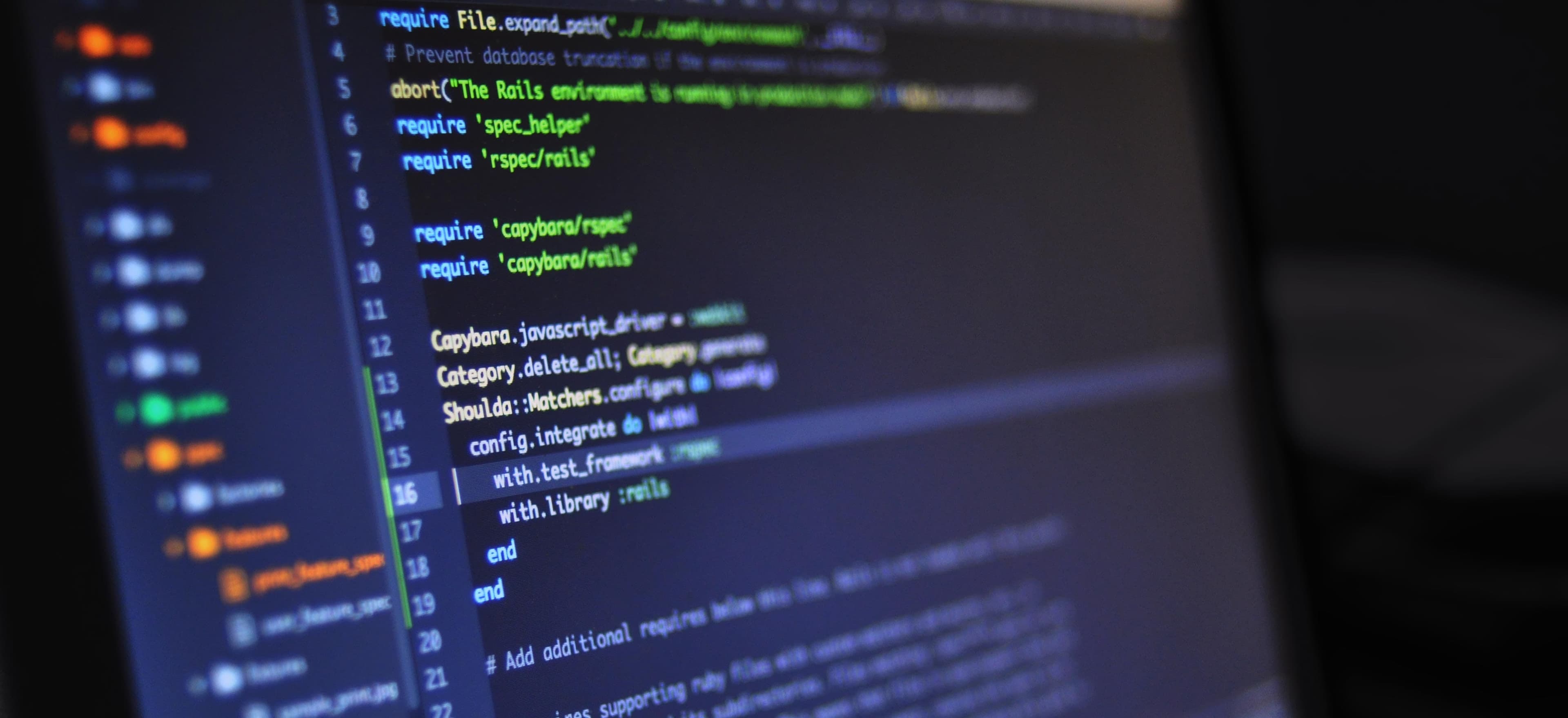
- Published on
Understanding Leap Seconds in Java Time API
Timekeeping is a fundamental aspect of human civilization, and it’s fascinating how our methods of tracking time have evolved. One of the more intricate components of timekeeping is the concept of leap seconds. In this post, we'll delve into what leap seconds are, discuss their implications in the Java Time API (introduced in Java 8), and explore how to work with them.
What are Leap Seconds?
At its core, a leap second is added to Coordinated Universal Time (UTC) to compensate for discrepancies between the Earth's rotation and atomic time. The Earth doesn’t rotate at a perfectly consistent speed; it experiences irregularities due to gravitational forces from the moon and sun, among other factors. Thus, the need for an occasional adjustment, known as a leap second.
Why Do We Need Leap Seconds?
The primary goal of adding leap seconds is to ensure that the time we measure using atomic clocks (which is incredibly precise) remains in sync with solar time (the time based on Earth's rotation). The average day is not precisely 24 hours; it's typically about 86,400 seconds but can vary. To maintain this synchronization, leap seconds are occasionally added—either June 30 or December 31.
Leap Seconds and the Java Time API
The Java Time API, introduced with Java 8 in the java.time
package, offers a comprehensive way to work with dates and times, including handling leap seconds more elegantly than the older java.util.Date
and java.util.Calendar
classes.
However, it’s important to note that while the Java Time API accommodates leap seconds conceptually, not all parts of the API use leap seconds in calculations. The Instant
class is the key player here.
Understanding Instant
An Instant
represents a moment on the timeline, expressed in UTC. An Instant
can be manipulated and queried, but how does it accommodate leap seconds? The truth is, leap seconds are not directly represented in the Instant
class. Instead, they are accounted for when you convert between different time zones or systems.
Example of Using Instant
Here's a short example to demonstrate how you might work with Instant
:
import java.time.Instant;
public class LeapSecondExample {
public static void main(String[] args) {
// Current Instant
Instant now = Instant.now();
System.out.println("Current Instant: " + now);
// Specific Instant for known leap second
Instant leapSecond = Instant.parse("2016-12-31T23:59:60Z");
System.out.println("Leap Second Instant: " + leapSecond);
// Comparing two Instants
System.out.println("Is the leap second the current instant? " + now.equals(leapSecond));
}
}
Code Commentary
- The first line retrieves the current moment in time and prints it.
- The second part creates an
Instant
at the known leap second time of December 31, 2016, where a second is added. - Finally, it checks whether the current instant is equal to the leap second instant (which, in most practical applications, it won't be).
Despite the leap second's theoretical significance, Java does not handle them as part of calculations, which is essentially why it is essential to monitor the synchronization between your application's timekeeping system and UTC.
Handling Time Zones
Leap seconds can affect the behavior of time zones, particularly when converting between UTC and local times. The Java Time API provides ZonedDateTime
for this purpose.
Example of Using ZonedDateTime
import java.time.ZonedDateTime;
import java.time.ZoneId;
public class ZoneLeapSecondExample {
public static void main(String[] args) {
// Current time in UTC
ZonedDateTime nowUTC = ZonedDateTime.now(ZoneId.of("UTC"));
System.out.println("Current time in UTC: " + nowUTC);
// Time in New York
ZonedDateTime newYorkTime = nowUTC.withZoneSameInstant(ZoneId.of("America/New_York"));
System.out.println("Current time in New York: " + newYorkTime);
// Adding a leap second
ZonedDateTime leapSecond = ZonedDateTime.parse("2016-12-31T23:59:60Z[UTC]");
System.out.println("Leap second in UTC: " + leapSecond);
// Convert leap second to New York time
ZonedDateTime leapSecondNy = leapSecond.withZoneSameInstant(ZoneId.of("America/New_York"));
System.out.println("Leap second in New York: " + leapSecondNy);
}
}
Code Commentary
- The code retrieves the current UTC time using
ZonedDateTime
. - By changing the time zone to "America/New_York", you convert the UTC time to Eastern Standard Time.
- The leap second created is then transformed to New York time.
Caveats and Considerations
While Java's Time API accommodates leap seconds in theory, many systems may ignore them. Here are a few important points to consider:
-
Not All Java Libraries Handle Leap Seconds: Libraries that rely heavily on
Instant
might not recognize leap seconds. -
External Systems: APIs or systems you may interact with could handle time without consideration for leap seconds.
-
Practical Synchronization: If your application depends on precise timing—such as satellite communication or space missions—it's crucial to manage timekeeping carefully and ensure your software is designed with leap seconds in mind.
Further Reading
For those keen on exploring these concepts beyond the scope of Java, consider checking out the International Earth Rotation and Reference Systems Service (IERS). They provide insightful details about leap seconds and timekeeping standards.
Additionally, for more in-depth understanding of the Java Time API and its components, the Java Documentation offers extensive resources.
The Bottom Line
Leap seconds are a fascinating yet complex aspect of global timekeeping. Understanding and accommodating the intricacies of leap seconds in programming—especially in Java—is vital for developers engaged in applications that require exact time measurements. The Java Time API provides an effective foundation for working with time, but always remember the significance of synchronization and the handling of possible leap seconds as part of your application's logic.
As the Earth continues to spin and orbit, the keeping of time will only become more vital, and our coding practices must evolve alongside it. Dive into your Java projects, keeping these principles in mind, and let leap seconds guide you toward more precise timekeeping!
Checkout our other articles