Unlocking Java Serialization: Inner Class Challenges Explained
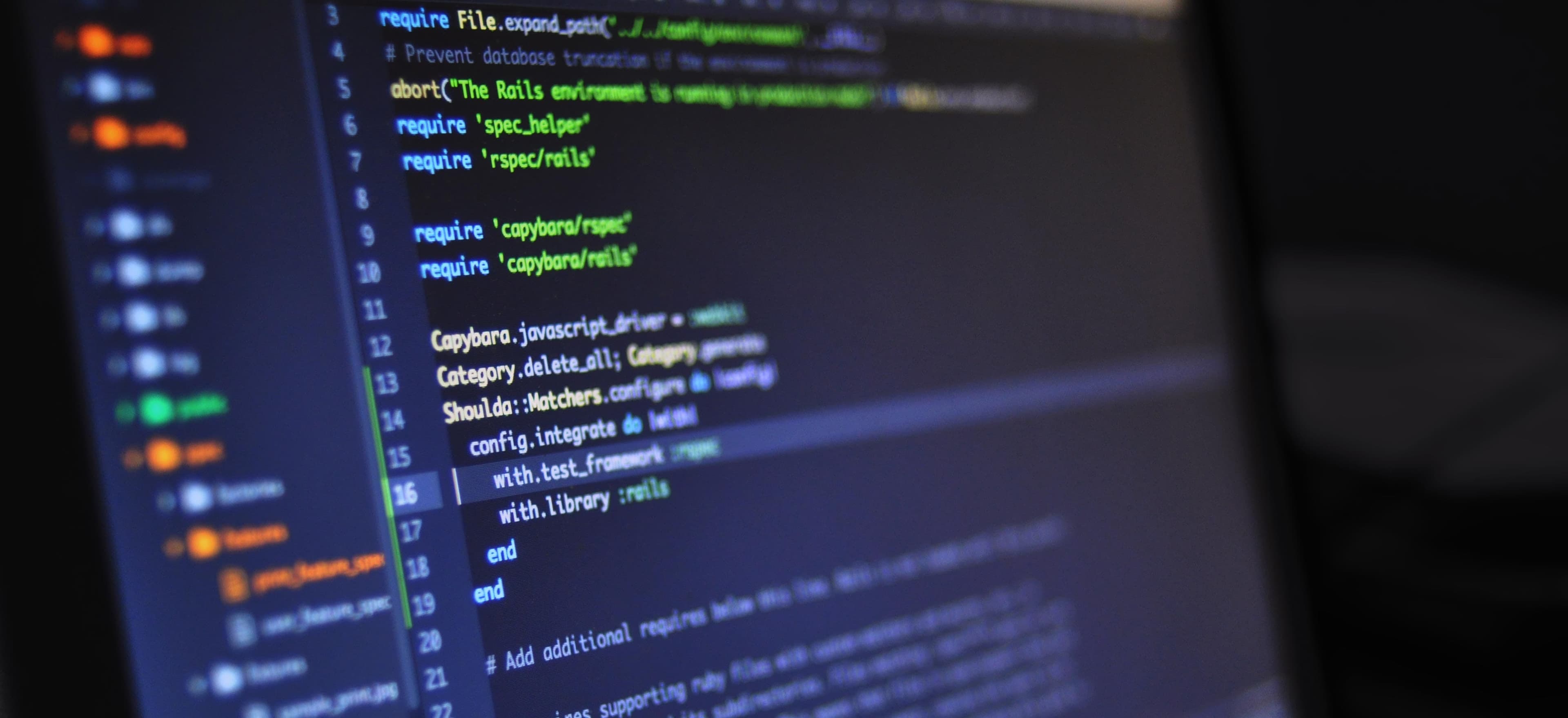
- Published on
Unlocking Java Serialization: Inner Class Challenges Explained
Java serialization is a vital concept in Java development, allowing developers to convert an object into a byte stream, which can be reverted back into a copy of the object later. This mechanism is particularly useful for saving the state of an object or transferring it over a network. However, when it comes to inner classes, Java serialization poses unique challenges that every developer should understand. In this post, we'll dig deep into these challenges, providing you with practical code examples and explanations.
Understanding Java Serialization
Before delving into the specifics of inner classes, it's crucial to have a foundational understanding of serialization in Java. The core of serialization revolves around two key interfaces: Serializable
and Externalizable
.
- Serializable: This is a marker interface, meaning it does not contain any methods. By implementing this interface, a class indicates that its objects can be serialized.
- Externalizable: This interface extends Serializable and requires explicit definition of how an object’s state is written and read.
Basic Example of Serialization
Here is a simple example of a class that implements Serializable
:
import java.io.*;
class Person implements Serializable {
private String name;
private transient int age; // transient variable will not be serialized
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Person{name='" + name + "', age=" + age + '}';
}
}
public class SerializationDemo {
public static void main(String[] args) {
Person person = new Person("John", 30);
// Serialize the object
try (ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("person.ser"))) {
oos.writeObject(person);
} catch (IOException e) {
e.printStackTrace();
}
// Deserialize the object
try (ObjectInputStream ois = new ObjectInputStream(new FileInputStream("person.ser"))) {
Person savedPerson = (Person) ois.readObject();
System.out.println(savedPerson);
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
Why Use Serialization?
Serialization plays a crucial role in situations where you need to:
- Persist application states.
- Send data over a network.
- Clone objects in a straightforward manner.
It ensures that the object's state is preserved across sessions, making it one of the essential features in Java.
The Inner Class Enigma
When dealing with inner classes in Java, serialization introduces several intricacies. An inner class inherently carries a reference to its outer class. This connection can lead to complications during serialization.
Challenges with Inner Classes
-
Implicit References: Inner classes hold an implicit reference to their enclosing class. When you serialize an inner class instance, you inadvertently serialize its outer class instance as well. If the outer class is not serializable, a
NotSerializableException
will be thrown. -
Static Inner Classes: Conversely, a static inner class does not hold a reference to its enclosing class. This means it can be serialized independently.
Example of Inner Class Serialization
To illustrate these challenges, let’s look at a common scenario:
import java.io.*;
class OuterClass implements Serializable {
private String outerField = "Outer Field";
class InnerClass implements Serializable {
private String innerField = "Inner Field";
@Override
public String toString() {
return "InnerClass{" + "innerField='" + innerField + '\'' + ", outerField='" + outerField + '\'' + '}';
}
}
}
public class InnerClassSerialization {
public static void main(String[] args) {
OuterClass outer = new OuterClass();
OuterClass.InnerClass inner = outer.new InnerClass();
try (ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("inner.ser"))) {
oos.writeObject(inner);
} catch (IOException e) {
e.printStackTrace();
}
try (ObjectInputStream ois = new ObjectInputStream(new FileInputStream("inner.ser"))) {
OuterClass.InnerClass savedInner = (OuterClass.InnerClass) ois.readObject();
System.out.println(savedInner);
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
Why This Matters
In the above example, the inner class InnerClass
can be serialized because it implements Serializable
and its enclosing class OuterClass
is also serializable. If you were to modify OuterClass
to not implement Serializable
, the serialization will fail.
Static Inner Classes: A Different Approach
Static inner classes can sidestep some of the serialization issues faced by non-static inner classes. Because static inner classes do not hold a reference to the instance of the containing class, they can be serialized independently.
Here’s how you can leverage static inner classes:
class OuterClass {
static class StaticInnerClass implements Serializable {
private String field = "Static Inner Field";
@Override
public String toString() {
return "StaticInnerClass{" + "field='" + field + '\'' + '}';
}
}
}
public class StaticInnerClassSerialization {
public static void main(String[] args) {
OuterClass.StaticInnerClass staticInner = new OuterClass.StaticInnerClass();
try (ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("staticInner.ser"))) {
oos.writeObject(staticInner);
} catch (IOException e) {
e.printStackTrace();
}
try (ObjectInputStream ois = new ObjectInputStream(new FileInputStream("staticInner.ser"))) {
OuterClass.StaticInnerClass savedStaticInner = (OuterClass.StaticInnerClass) ois.readObject();
System.out.println(savedStaticInner);
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
Rationale Behind Static Inner Classes
A static inner class's independence from its enclosing class makes it a versatile choice. It provides the encapsulation of the inner class while eliminating potential serialization pitfalls, as it doesn't carry context about the containing class.
Bringing It All Together
Understanding serialization in Java, especially concerning inner classes, is essential for Java developers. The complexities of inner class serialization can lead to unexpected errors if not properly managed. Always evaluate whether an inner class is a suitable design choice when serialization is involved.
For further reading on Java serialization, you can refer to Oracle's official guide on Java Serialization and Baeldung's tutorial on Serialization.
By mastering these concepts, you will enhance your Java programming skills significantly and avoid common pitfalls when implementing serialization, especially in the context of inner classes. Happy coding!