Understanding Variable Capture Issues in Java Lambdas
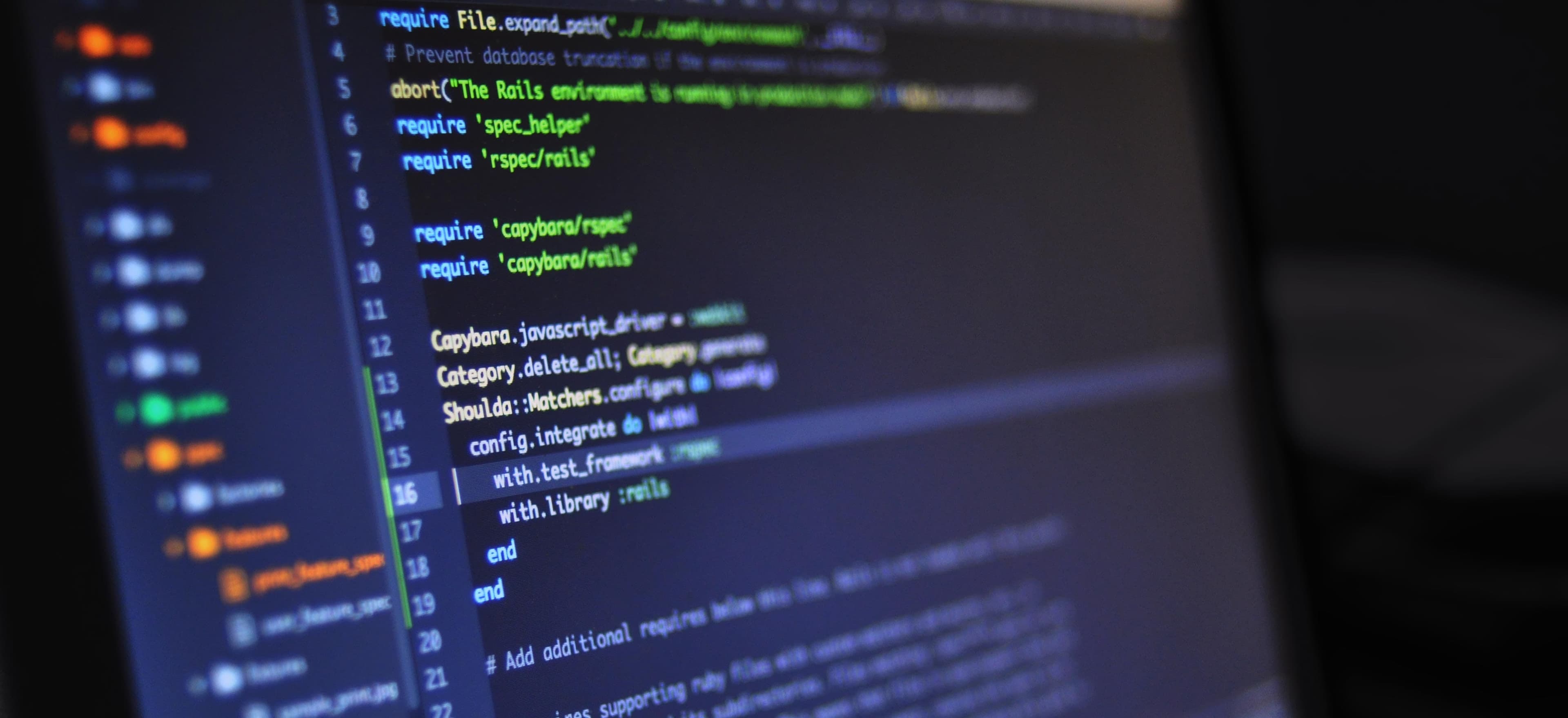
- Published on
Understanding Variable Capture Issues in Java Lambdas
Java introduced lambdas in Java 8, allowing developers to express instances of single-method interfaces (functional interfaces) more concisely. Along with the power of lambdas comes the intricacy of variable capture. Understanding how variable capture works—and the issues associated with it—is crucial for writing effective, efficient, and predictable code in Java. In this blog post, we will dive deep into variable capture in lambda expressions, exploring what it is, how it works, and potential pitfalls that developers might encounter.
What is Variable Capture?
Variable capture refers to the way lambda expressions in Java can access variables from the surrounding (enclosing) scope. This enables lambda expressions to work with variables defined outside of their own body. When you create a lambda expression, the Java compiler captures the state of these variables for use within the lambda.
For example, consider the following code snippet:
public class CaptureExample {
public static void main(String[] args) {
int number = 10;
Runnable runnable = () -> {
System.out.println("Number: " + number);
};
runnable.run(); // Output will be: Number: 10
}
}
Commentary on the Example
In this example, the number
variable is defined outside the lambda expression but is accessed within it. The lambda expression captures the value of number
, allowing it to print "Number: 10" when runnable.run()
is invoked. However, it's important to note that the captured variable must be effectively final. This means that once its value is assigned, it must not change for the duration of its capture.
The Concept of Effective Finality
From Java 8 onwards, Java introduced the concept of effective finality to simplify the usage of local variables in lambdas. A variable is considered effectively final if it is not modified after its initial assignment.
Here’s an example to observe how effective finality works:
public class EffectiveFinalExample {
public static void main(String[] args) {
int number = 10;
Runnable runnable = () -> {
// The following line would cause a compile-time error.
// number = 15; // This line is commented out to maintain effective finality
System.out.println("Effective Final Number: " + number);
};
runnable.run(); // Output will be: Effective Final Number: 10
}
}
Why Effective Finality Matters
Understanding effective finality matters because it ensures clarity and predictability in your code. If a variable is modified after its definition, it could lead to confusing results. The lambda would reference the last assigned value of the variable, not the value at the time the lambda was created. This could lead to bugs that are hard to trace.
Common Pitfalls with Variable Capture
1. Modifying Captured Variables
As already mentioned, you cannot modify captured local variables, which can lead to confusion during code refactoring. Here is an example:
public class CapturePitfallExample {
public static void main(String[] args) {
int count = 0; // This will throw an error if attempted to modify
Runnable runnable = () -> {
// count++; // Uncommenting this line will cause a compile error
System.out.println("Count: " + count);
};
runnable.run(); // Output will be: Count: 0
}
}
2. Capturing Mutable Objects
When capturing mutable objects, unexpected behavior can occur. Consider this example where we capture a mutable list:
import java.util.ArrayList;
import java.util.List;
public class MutableCaptureExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
Runnable runnable = () -> {
list.add("Hello");
};
runnable.run();
System.out.println(list); // Output will be: [Hello]
}
}
Commentary on Object Mutability
In this case, while list
is mutable and can be modified inside the lambda, if the list was reassigned within the lambda itself, it would cause an error. This behavior contrasts with primitive types, which become immutable once captured as references.
Handling Variable Capture in Real-World Applications
In real-world applications, managing variable capture effectively is crucial. Here are some best practices:
-
Use Effectively Final Variables: Always strive to use effectively final variables whenever possible to maintain clarity.
-
Avoid Capturing State: When possible, avoid capturing mutable state in lambdas. Instead, consider using immutable types or encapsulating the logic to reduce side effects.
-
Leverage Local Classes: When you need to encapsulate behaviors alongside state, consider using local classes instead of lambdas. Local classes allow you to encapsulate both state and behavior more succinctly.
-
Use Streams Carefully: When manipulating collections with streams, leverage method references and avoid capturing external state unnecessarily.
Stream Example
Here’s how you might handle a collection without unnecessary variable capture:
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Using forEach with a method reference to avoid capturing the local variable
names.forEach(System.out::println); // Output will be: Alice Bob Charlie
}
}
Final Considerations
Variable capture in Java lambdas is a powerful feature that allows you to create concise code that interacts with variables from an enclosing scope. However, with this power comes the need to understand effective finality and the potential pitfalls associated with capturing mutable state.
By adhering to best practices such as avoiding captured mutable state and leveraging effectively final variables, you can write robust and maintainable Java code that utilizes lambda expressions effectively. Keep these considerations in mind during your next coding session, and you'll be well on your way to harnessing the full potential of lambdas in Java.
For further reading on functional programming concepts in Java, you can explore Oracle's official documentation on Java 8 features and Java's lambda expressions.
Happy coding!