Handling Memory Leaks with Phantom References in Java
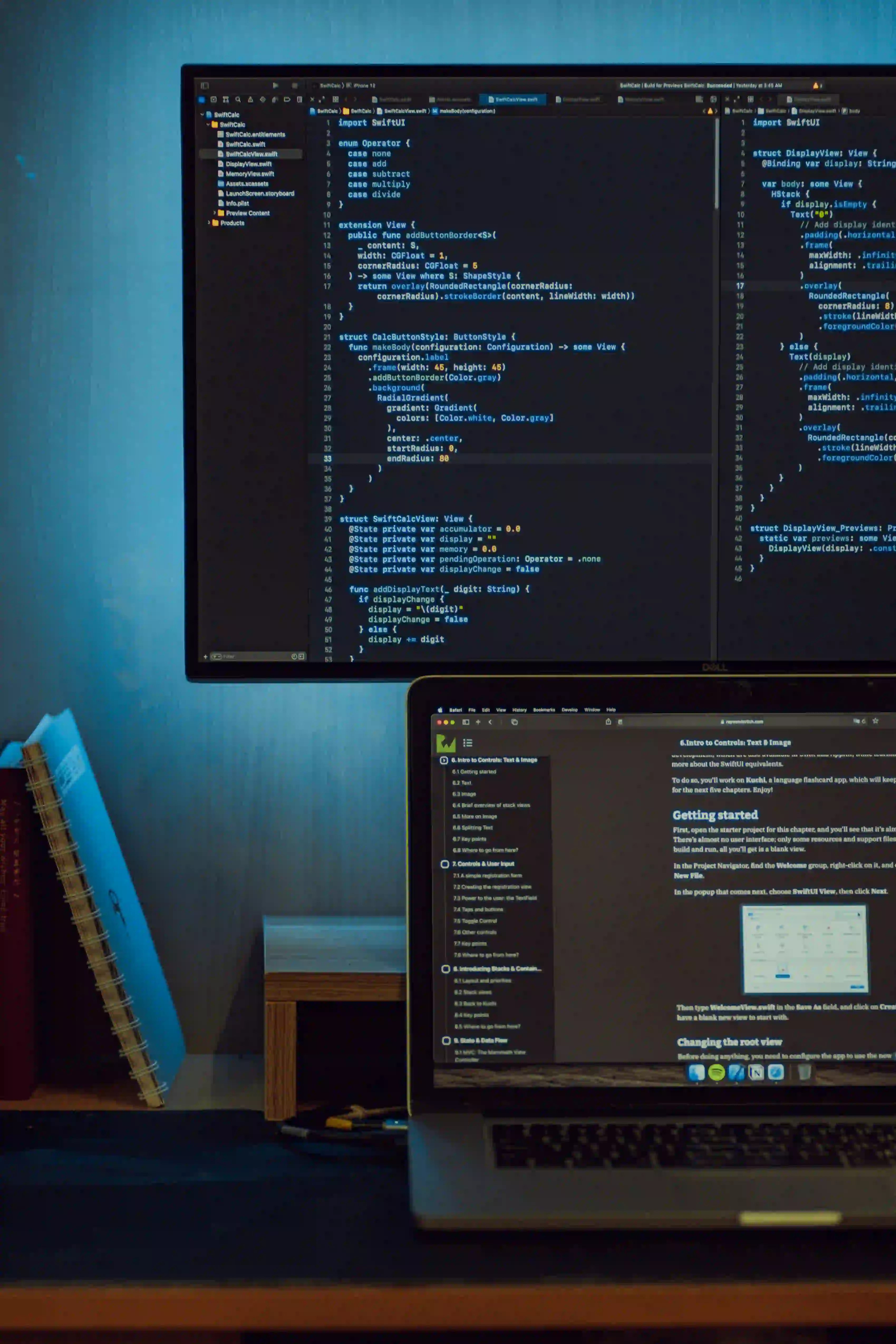
Handling Memory Leaks with Phantom References in Java
Memory management is a crucial aspect of programming in any language, and Java is no exception. Despite its automatic garbage collection, Java developers must stay vigilant against memory leaks—problems that can degrade performance over time. Understanding and applying the concept of phantom references can greatly aid in managing memory leaks effectively.
In this blog post, we will explore phantom references in Java, how they can be used to manage memory leaks, and when to implement them. We’ll also contrast them with other types of references, and provide code snippets for practical understanding.
Understanding Java References
Java categorizes references into four types:
-
Strong References: The default type of reference in Java. If an object has a strong reference, it is not eligible for garbage collection, even if there are no other references to it.
☕snippet.javaObject obj = new Object(); // Strong reference
-
Weak References: These allow the garbage collector to reclaim the object even if they are maintained. Weak references are useful for implementing cache behavior.
☕snippet.javaWeakHashMap<Key, Value> cache = new WeakHashMap<>();
-
Soft References: Useful for implementing memory-sensitive caches. The garbage collector might clear soft references in low memory conditions.
☕snippet.javaSoftReference<Object> softRef = new SoftReference<>(new Object());
-
Phantom References: These do not prevent the object from being garbage collected, but they provide a way to determine when an object has been collected. This makes phantom references particularly useful for cleanup actions.
☕snippet.javaPhantomReference<Object> phantomRef = new PhantomReference<>(new Object(), referentQueue);
What are Phantom References?
Phantom references are a unique kind of reference that allows developers to handle objects post-garbage collection. When an object is referenced by a phantom reference and is garbage collected, the reference itself is enqueued in a reference queue.
When to Use Phantom References
Phantom references are typically used in the following scenarios:
-
Managing Resources: They can help manage native resources not directly managed by the Java Virtual Machine (JVM), like file handles or database connections.
-
Cleanup Operations: Phantom references can ensure that resources are cleaned up only after the object is collected.
-
Avoiding Memory Leaks: They help avoid memory leaks in long-running applications, especially in combination with reference queues.
Implementing Phantom References to Avoid Memory Leaks
Let’s delve into a sample implementation of phantom references in Java which highlights their utility in preventing memory leaks.
import java.lang.ref.PhantomReference;
import java.lang.ref.ReferenceQueue;
public class PhantomReferenceExample {
private static class Resource {
private String name;
public Resource(String name) {
this.name = name;
System.out.println("Resource created: " + name);
}
@Override
protected void finalize() throws Throwable {
System.out.println("Resource finalized: " + name);
super.finalize();
}
}
public static void main(String[] args) {
ReferenceQueue<Resource> referenceQueue = new ReferenceQueue<>();
Resource resource = new Resource("MyResource");
// Create a phantom reference to the resource
PhantomReference<Resource> phantomRef = new PhantomReference<>(resource, referenceQueue);
// Now we can clear the strong reference to the resource
resource = null;
// At this point, the resource is eligible for garbage collection
System.gc(); // Request for garbage collection
// Process the reference queue to check for any phantom references
try {
PhantomReference<?> ref = (PhantomReference<?>) referenceQueue.remove();
System.out.println("Phantom reference cleared: " + ref);
// This is where clean-up logic could go.
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
Code Explanation
-
Resource Creation: We define a simple
Resource
class with a constructor and afinalize()
method to demonstrate when objects are garbage collected. -
ReferenceQueue: A
ReferenceQueue
is created to hold phantom references after they are collected. -
PhantomReference Initialization: The
Resource
object is wrapped in aPhantomReference
, which links it to the reference queue. -
Triggering Garbage Collection: After setting the strong reference to null, we call
System.gc()
, which suggests the JVM to perform garbage collection. -
Handling the Reference Queue: Finally, the program waits for the phantom reference to be enqueued and processes it, indicating that the resource is ready for cleanup.
Benefits of Using Phantom References
-
Memory Management: Phantom references allow you to perform cleanup operations once an object has been collected. This is crucial when working with objects that consume external resources.
-
Prevention of Memory Leaks: By ensuring extra references don’t keep objects alive, phantom references help keep your application memory-efficient.
-
Flexibility: You can implement custom clean-up logic, making phantom references versatile for different use cases.
Contrast with Other References
While weak and soft references are beneficial in caching data, they don’t allow post-garbage collection processing. Phantom references fill that gap, making them ideal when you need to know when a resource can be safely cleaned.
Phantom references do have a drawback—they do not directly give access to the object they reference. Because of this, phantom references must be used within the context of cleanup operations following a collection event.
When Not to Use Phantom References
Not every case requires the use of phantom references. If you are working with simple Java applications or if the objects are not managing significant external resources, it might be an overkill. Typical use cases include complex systems dealing with extensive resources or where finalization timing is critical.
Additional Resources
For more information on how memory management works in Java, check out the following links:
- Oracle’s Guide on Java Memory Management
- Understanding Java Garbage Collection
The Bottom Line
In summary, phantom references serve a unique purpose in Java’s memory management strategy. They enable developers to perform clean-up activities after objects are no longer referenced, helping to avoid memory leaks and manage resources better. By understanding the mechanics of phantom references, you can architect more robust Java applications that maintain performance over time.
Experimenting with this implementation offers an excellent opportunity to witness how phantom references can effectively manage memory. As with any tool, their utility will depend on the specific requirements of your application. Happy coding!