Handling Java Float NaN and Infinity: Common Pitfalls
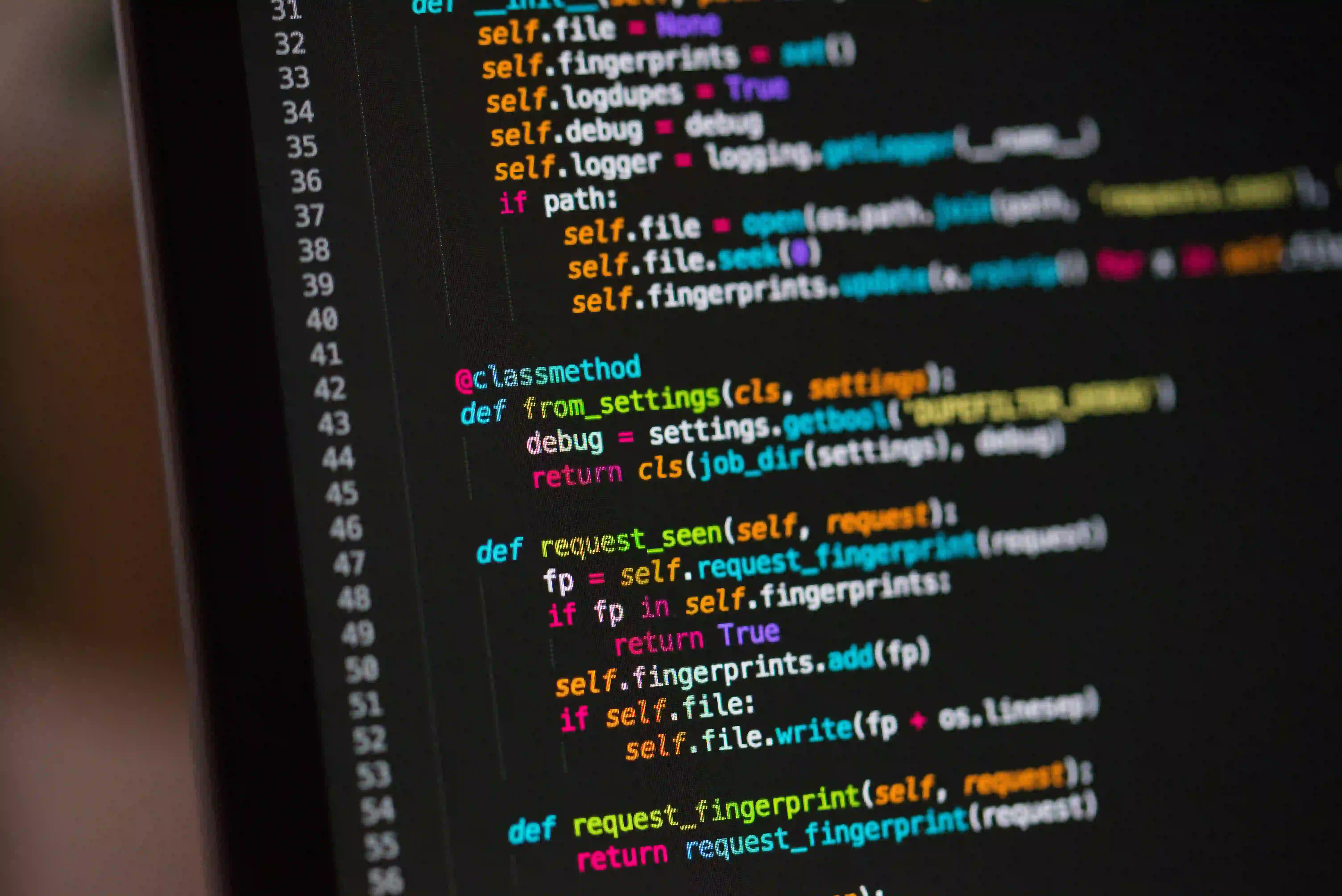
Handling Java Float NaN and Infinity: Common Pitfalls
In the vast world of programming, handling special values such as Not-a-Number (NaN) and Infinity in floating-point computations can be particularly tricky. Java, with its rich floating-point support, offers tools for dealing with these cases, but pitfalls can easily emerge if developers are not attentive. In this blog post, we will explore NaN and Infinity, their implications, and common pitfalls when handling them in Java.
Understanding NaN and Infinity
What is NaN?
NaN, or Not-a-Number, is a special floating-point value that signifies an undefined or unrepresentable value in mathematical computations. This can arise from operations like:
float result = 0.0f / 0.0f; // Division by zero
In this example, the result will be NaN because dividing zero by zero is mathematically ambiguous.
What is Infinity?
Infinity in Java represents a value that exceeds the largest representable floating-point number. This can occur with operations such as:
float result = 1.0f / 0.0f; // Division by zero
Here, the result will be positive Infinity. Conversely, dividing a negative number by zero will yield negative Infinity:
float negativeResult = -1.0f / 0.0f; // Negative Infinity
Why Handle NaN and Infinity?
Handling NaN and Infinity correctly is crucial to ensure the integrity of calculations, especially in scientific, financial, and data processing applications. Not addressing these values can lead to unexpected behavior, incorrect results, or even crashes.
Common Pitfalls
Pitfall 1: Comparing NaN Values
A frequent mistake when dealing with NaN is to use equality comparisons. In Java, NaN is not equal to itself:
float nanValue = Float.NaN;
if (nanValue == Float.NaN) {
// This block will never execute
System.out.println("NaN is equal to NaN");
}
The Right Approach
To properly check if a value is NaN, use the Float.isNaN
method:
if (Float.isNaN(nanValue)) {
System.out.println("This value is NaN");
}
This approach correctly identifies NaN values, preventing misleading logic errors in your code.
Pitfall 2: Using NaN in Calculations
Another common pitfall is carrying NaN through calculations without proper checks. Any operation involving NaN will result in NaN:
float a = Float.NaN;
float b = 5.0f;
float result = a + b; // result will be NaN
The Recommended Practice
Always validate your inputs before performing calculations:
if (Float.isNaN(a) || Float.isNaN(b)) {
System.out.println("Valid input required for calculations");
} else {
float result = a + b;
}
By implementing such validations, you'll avoid propagating NaN through equations inadvertently.
Pitfall 3: Comparing Infinity Values
Like NaN, Infinity comparisons can also be misleading. For instance:
float positiveInfinity = Float.POSITIVE_INFINITY;
float negativeInfinity = Float.NEGATIVE_INFINITY;
if (positiveInfinity == negativeInfinity) {
// This will also not execute
System.out.println("Infinity is equal to -Infinity");
}
Correct Comparison Solution
Use the predefined constants for comparisons:
if (positiveInfinity > 0f) {
System.out.println("This is positive Infinity");
}
if (negativeInfinity < 0f) {
System.out.println("This is negative Infinity");
}
Comparing against 0, or even using Float.isInfinite
, increases clarity and correctness.
Pitfall 4: Not Handling Infinite Values Appropriately
Another trap developers can fall into is treating Infinity as a viable number. Operations with Infinity can yield unexpected results, and using it without caution can distort calculations.
float a = Float.POSITIVE_INFINITY;
float b = 100.0f;
float result = a + b; // result will still be Infinity
Handling Infinite Values
When Infinity is a possibility, it helps to define how your application should behave:
if (Float.isInfinite(a)) {
System.out.println("Value is infinite, handle accordingly");
} else {
float result = a + b;
}
In applications requiring precise calculations, handling Infinity should be prioritized to maintain accuracy.
Pitfall 5: Misunderstanding the Behavior of Operations Resulting in NaN or Infinity
Operations such as subtraction, multiplication, or even square roots can yield NaN or Infinity without explicit reasons. For instance:
float result = Math.sqrt(-1); // result will be NaN
Recognizing the Need for Valid Input
To avoid surprises, consistently check conditions before performing operations that can yield NaN:
float value = -1.0f;
if (value < 0) {
System.out.println("Square root cannot be calculated for negative numbers");
} else {
float result = Math.sqrt(value);
}
Validating input prior to executing operations will help maintain the expected flow of data.
Best Practices for Managing NaN and Infinity
-
Use Helper Methods: Create utility methods to check for NaN and Infinity consistently.
-
Input Validation: Always validate inputs to ensure they fall within expected ranges.
-
Use Logging: Log when NaN or Infinity is encountered, which can aid in debugging.
-
Iterative Testing: Regularly run unit tests to handle edge cases involving NaN and Infinity.
-
Documentation: Ensure your code is well-documented so that future developers understand how to handle special floating-point values effectively.
Wrapping Up
Handling NaN and Infinity in Java requires a deliberate and cautious approach. By avoiding common pitfalls and employing best practices, you can ensure that your applications behave predictably and robustly in the presence of these special floating-point values. As always, keep testing and refining your code to handle edge cases effectively.
For further reading on handling floating-point values in Java, you can explore the Java Documentation and consider checking IEEE Floating Point Standard for a deeper understanding of floating-point representations.
Happy coding!