Understanding Java Thread Priority: Boost Your App's Performance
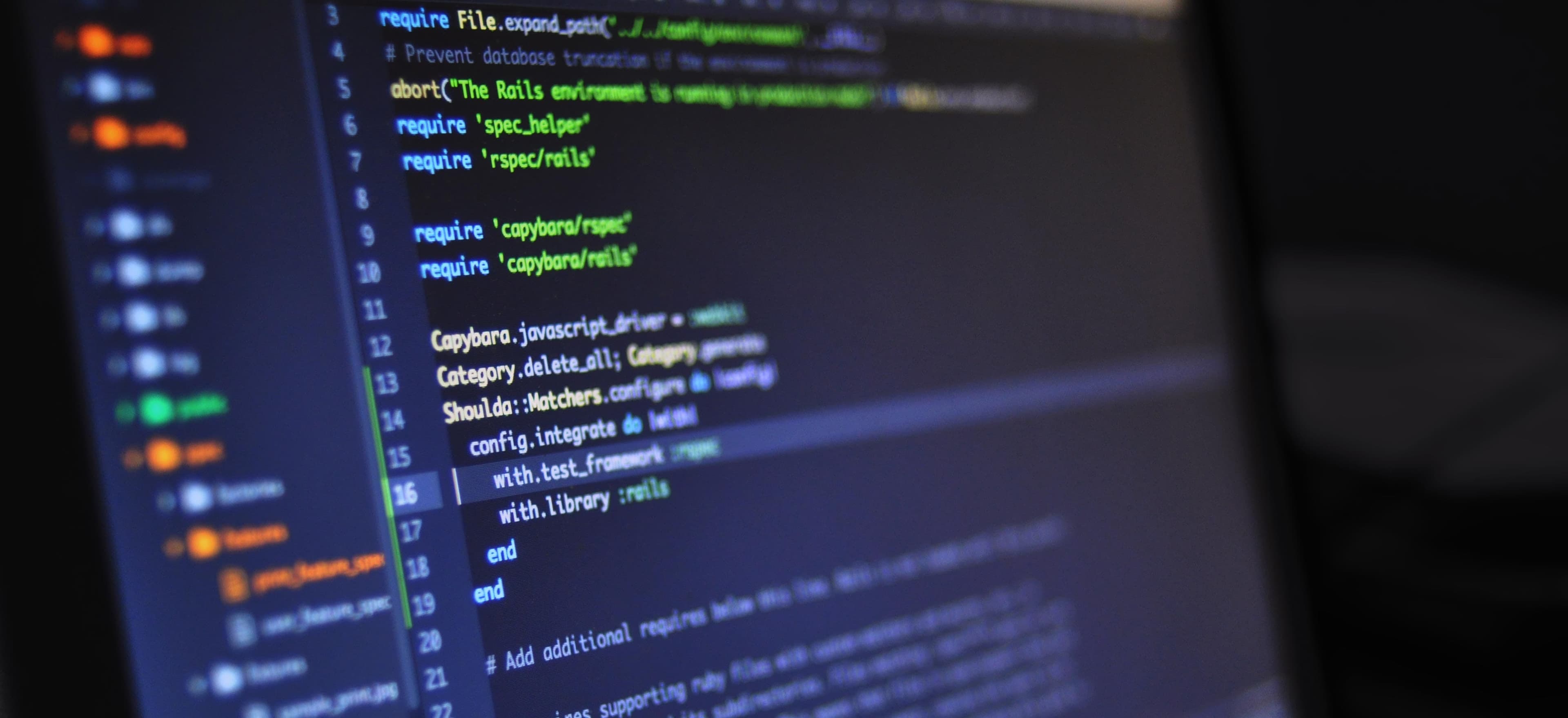
- Published on
Understanding Java Thread Priority: Boost Your App's Performance
In the world of Java programming, achieving optimal performance is often a critical goal. One vital element that can significantly impact the performance of multi-threaded applications is thread priority. But what exactly is thread priority in Java, and how can it be effectively utilized to enhance your application's performance? In this blog post, we'll take a deep dive into the topic, elucidate its mechanics, and share insightful code examples.
What is Thread Priority?
In Java, thread priority is an integer value that indicates the importance of a thread relative to others. The Java Virtual Machine (JVM) uses this value to determine how much CPU time the thread should receive when competing for resources.
Java provides a predefined set of thread priority levels:
Thread.MIN_PRIORITY (1)
Thread.NORM_PRIORITY (5)
Thread.MAX_PRIORITY (10)
When you create a thread, it inherits the priority of its parent thread. You can adjust a thread's priority using the setPriority(int newPriority)
method, which accepts values between Thread.MIN_PRIORITY
and Thread.MAX_PRIORITY
.
How Thread Priority Works
The actual effect of thread priority is dependent on the operating system's thread scheduler. Some operating systems honor thread priorities more closely than others. In many cases, priorities may not result in substantial performance differences.
Thread Scheduling
Understanding how thread scheduling affects performance is crucial. The Java thread scheduler is responsible for allocating CPU time to various threads based on their priority. Ideally, threads with higher priority should be executed before lower-priority threads.
Here's a brief rundown of how the thread scheduler might work:
-
Time-Slicing: Many modern operating systems use time-slicing to allocate processor time. Each thread is given a small amount of time to execute before the operating system switches to another thread.
-
Preemption: Higher-priority threads might preempt lower-priority threads that are currently running if a higher-priority thread becomes runnable.
-
Fairness: While thread priority can, in theory, lead to better performance, thread schedulers must also ensure fairness among threads. This might result in lower-priority threads getting a chance to run.
Setting Thread Priority
Setting thread priority in Java is straightforward. Below is an example that illustrates how to create threads with different priorities.
public class ThreadPriorityDemo extends Thread {
@Override
public void run() {
System.out.println("Thread Name: " + Thread.currentThread().getName() +
", Priority: " + Thread.currentThread().getPriority());
}
public static void main(String[] args) {
ThreadPriorityDemo thread1 = new ThreadPriorityDemo();
ThreadPriorityDemo thread2 = new ThreadPriorityDemo();
ThreadPriorityDemo thread3 = new ThreadPriorityDemo();
// Set thread priorities
thread1.setPriority(Thread.MIN_PRIORITY);
thread2.setPriority(Thread.NORM_PRIORITY);
thread3.setPriority(Thread.MAX_PRIORITY);
// Start threads
thread1.start();
thread2.start();
thread3.start();
}
}
Explanation of the Code
-
Thread Creation: The
ThreadPriorityDemo
class extendsThread
. Inside therun()
method, we simply print the thread name and its priority. -
Setting Priorities: In the
main()
method, we create three thread instances. We assign them minimum, normal, and maximum priorities using thesetPriority(int newPriority)
method. -
Starting Threads: Finally, we invoke
start()
on each thread. The output will show how the thread priority affects which threads get executed first, though this is not guaranteed.
What You Should Know
It is essential to note that you cannot rely solely on thread priority for performance optimization. Other factors — such as competition for resources, I/O operations, and CPU-bound tasks — play significant roles.
To understand how these priorities behave in real-world applications, consider Java's Thread Scheduling or check out advanced techniques on Java Concurrency.
Performance Challenges with Thread Priority
Despite the powerful features that thread priorities might promise, they should be used judiciously. Here are some challenges you may face when using thread priorities:
-
Platform Dependency: As mentioned earlier, the effects of thread priority can vary based on the underlying operating system. For example, while a Unix-based system might provide strict priority handling, Windows could operate on a more unified scheduling model.
-
Neglected Lower-priority Threads: If your application doesn't manage thread priorities carefully, you might neglect lower-priority threads. In extreme cases, lower-priority threads could starve and never receive CPU time, leading to application hangs.
-
Not Always Effective: Simply increasing a thread's priority doesn't guarantee better performance. If too many threads are vying for a limited amount of CPU time, increased priority might not produce significant improvements.
When to Use Thread Priority
Understanding when to use thread priorities can be a game changer for some applications:
-
Real-time Applications: If you're developing real-time applications that require timely task execution, setting appropriate thread priorities can help meet stringent performance requirements.
-
Background Services: For processes that should run in the background without interrupting main application workflows, lower thread priorities can help maintain overall application responsiveness.
-
Resource-intensive Calculations: If a thread performs heavy calculations and is not time-sensitive, you might choose to run it at a lower priority while keeping UI-related tasks at higher priorities.
Bringing It All Together
Java thread priority is a powerful feature that, when used correctly, can enhance the performance of multi-threaded applications. Ultimately, achieving optimal performance requires a combination of proper thread priority settings and wise resource management strategies.
Remember, while increasing thread priority might sound like a good strategy, it's essential to consider how the thread scheduler behaves on your target platform. Always conduct performance testing and profiling to assess the actual impact of priority adjustments on your application.
For more details on threading in Java, explore the resources I've linked throughout this article, and don't hesitate to experiment with thread priorities in your projects. Happy coding!
By understanding and utilizing thread priorities correctly, you can give your Java applications a performance boost that can be particularly impactful in multi-threaded environments. For more in-depth discussions about concurrency in Java, stay tuned for upcoming posts, or check out the Java Concurrency in Practice book for a comprehensive overview.