Navigating Java's HashCode and Equality Traps
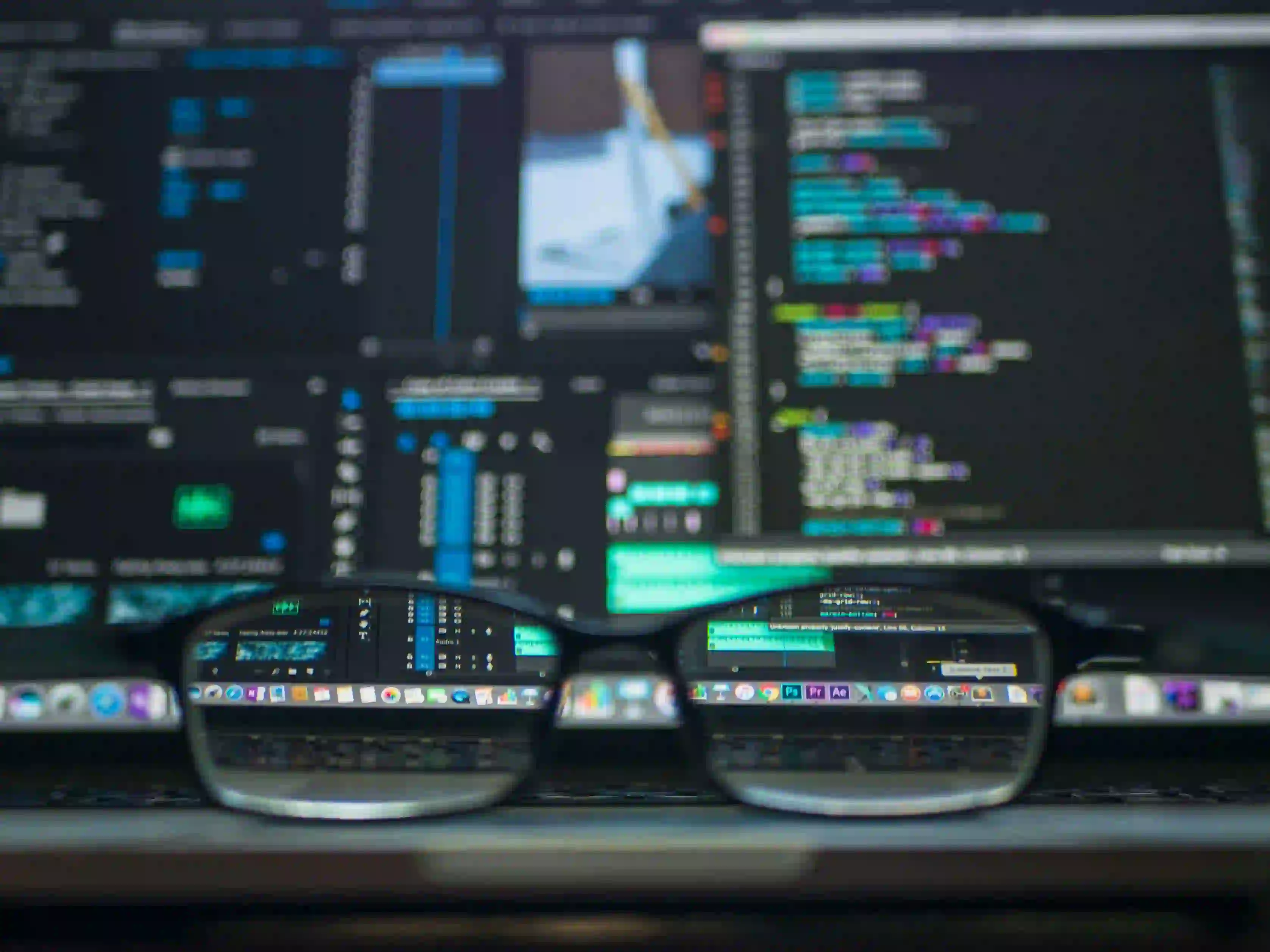
Navigating Java's HashCode and Equality Traps
Java developers often face challenges when dealing with objects, particularly regarding their equality and hash code functions. Understanding how to implement these methods appropriately is crucial for using collections like HashSet
, HashMap
, and other hash-based data structures. This blog post aims to clarify common pitfalls and best practices for overriding hashCode()
and equals()
, ensuring robust and efficient code.
1. The Importance of hashCode() and equals()
Before diving into the complexities, let's establish why hashCode()
and equals()
are essential. Java uses the Object
class's hashCode()
and equals()
methods when comparing objects.
- Equals: Defines a logical equality relation. Two objects that are logically equal must return
true
when calling theirequals()
method. - HashCode: Provides a hash value for an object, enabling its placement in hash-based collections such as hash tables.
The Java contract for these two methods states that if two objects are equal according to equals()
, then they must return the same hash code. If two objects are not equal, they should ideally return different hash codes. However, violating this principle can lead to unexpected behaviors and bugs.
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
MyObject that = (MyObject) obj;
return this.id != null ? this.id.equals(that.id) : that.id == null;
}
@Override
public int hashCode() {
return (id == null) ? 0 : id.hashCode();
}
Commentary
- The
equals()
method checks for reference equality first, which is fast and efficient. - It then checks for null and class type to ensure safe casting.
- The
hashCode()
method utilizes the object's ID to generate a unique code. This approach ensures that if two objects are equal, they generate the same hash code.
2. Common Pitfalls
2.1 Forgetting to Override Both Methods
One of the most common mistakes developers make is overriding equals()
but forgetting to implement hashCode()
. This oversight can lead to issues, especially when using collections. For example:
If A.equals(B)
returns true
, but A.hashCode() != B.hashCode()
, then this property is violated. The offending objects may behave unpredictably when stored in a HashMap
or HashSet
.
2.2 Using Non-Deterministic Properties
Another mistake is including non-deterministic properties in either hashCode()
or equals()
. For instance, if the equals()
method involves a mutable field, the result may change over the object’s lifetime, which can lead to inconsistencies in collections.
Example Code
public class User {
private String username;
private transient String password; // Transient field
// Constructor, getters, and setters...
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
User user = (User) obj;
return username.equals(user.username);
}
@Override
public int hashCode() {
return username.hashCode();
}
}
Commentary
- The
password
field is marked astransient
, meaning it won't be part of the serialization process or its hash code. - The
equals()
method relies solely onusername
, which is immutable once assigned, preventing issues related to mutability.
3. Best Practices for Overriding hashCode() and equals()
3.1 Use Consistent Fields
When implementing these methods, choose fields that represent the object's identity consistently. The chosen fields should ideally be:
- Immutable (like numeric IDs or strings).
- Have unique values to minimize hash collisions.
3.2 Keep HashCode() Fast
Optimizing the hashCode()
for performance is essential since hash codes are called frequently. Ideally, keep your computations simple:
@Override
public int hashCode() {
int result = 17; // Starting point
result = 31 * result + (field1 != null ? field1.hashCode() : 0);
result = 31 * result + (field2 != null ? field2.hashCode() : 0);
return result;
}
Commentary
- The magic number
31
is used because it is an odd prime. Multiplying by prime numbers helps to create a more evenly distributed hash table. - Using
17
as a starting point is a common practice in generating hash codes.
3.3 Use Java's Built-In Functions
For complex objects, consider using the java.util.Objects
class, specifically the Objects.equals()
and Objects.hash()
methods, to simplify your implementation:
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (!(obj instanceof MyObject)) return false;
MyObject that = (MyObject) obj;
return Objects.equals(this.field1, that.field1) &&
Objects.equals(this.field2, that.field2);
}
@Override
public int hashCode() {
return Objects.hash(field1, field2);
}
Commentary
Using these built-in methods makes your code more readable and less error-prone. Not only do they handle null
checks, but they also promote consistency in equals and hashCode operations.
4. Testing Your Implementation
Once you’ve implemented equals()
and hashCode()
, test your implementation thoroughly. Here’s a simple JUnit test example:
@Test
public void testEqualsAndHashCode() {
User user1 = new User("username1", "password");
User user2 = new User("username1", "anotherPassword");
assertTrue(user1.equals(user2));
assertEquals(user1.hashCode(), user2.hashCode());
}
Commentary
- Testing helps confirm that both methods function as expected.
- Consider edge cases, such as null values and different object types.
The Bottom Line
Navigating Java's hashCode()
and equals()
traps is essential for any Java developer. By understanding how these methods interact with collections, you can prevent common pitfalls and ensure your objects behave as expected. Remember to maintain consistency, use immutable fields, and keep your code clean and efficient.
For deeper insights into Java collections, consider reviewing the Java Documentation on Collections, or explore practical examples on Baeldung.
By following these best practices, you'll ensure that your Java applications are both performant and reliable.