Fixing SSL Peer Unverified Exception in Java Applications
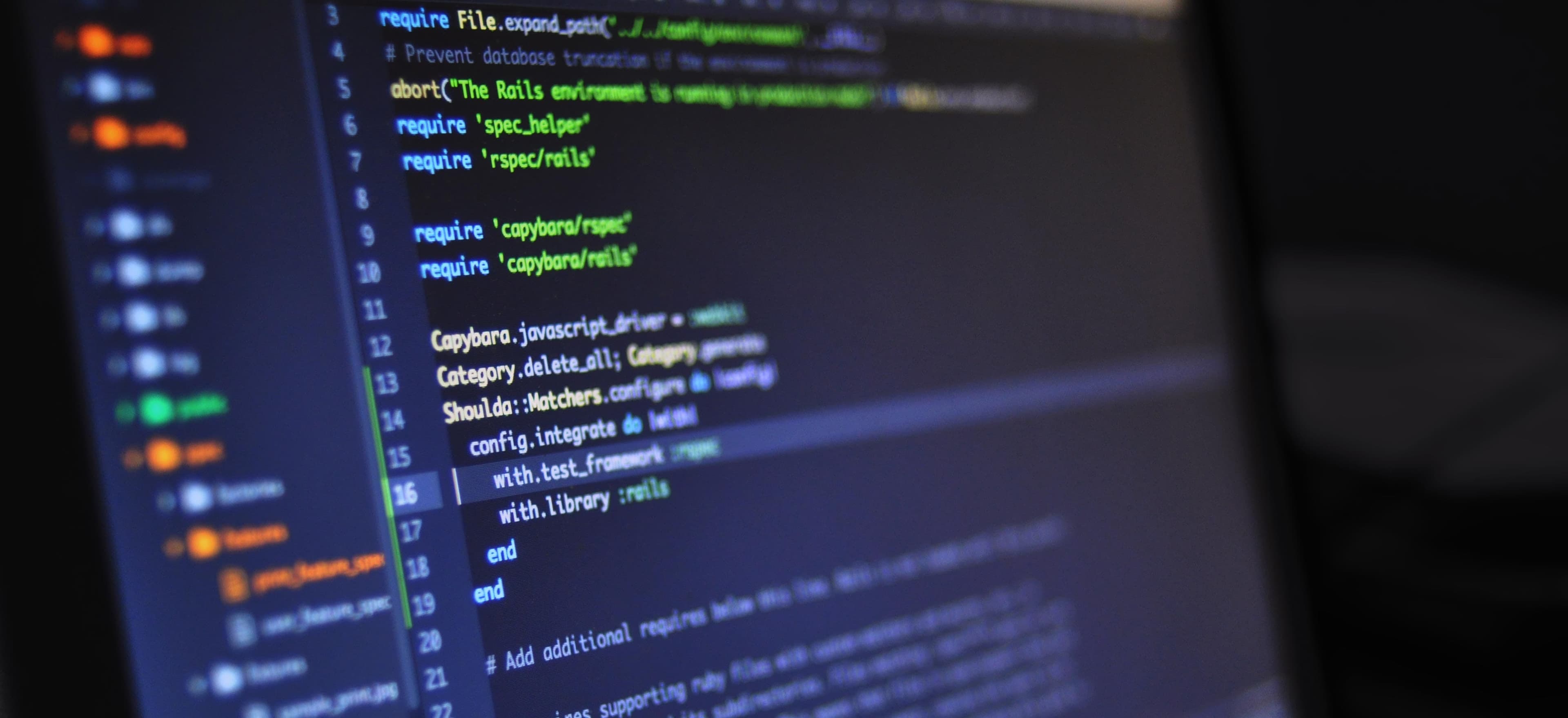
- Published on
Fixing SSL Peer Unverified Exception in Java Applications
In today's digital landscape, secure communication is paramount. When you're developing Java applications that interact with web services or APIs over HTTPS, you may encounter the infamous javax.net.ssl.SSLPeerUnverifiedException
. This error occurs when the SSL handshake fails, primarily owing to certificate validation issues. In this blog post, we'll explore the causes behind this exception and methods to fix it, ensuring that your Java applications communicate securely.
Understanding SSLPeerUnverifiedException
Before diving into solutions, it’s essential to understand what leads to this exception. An SSLPeerUnverifiedException
means that the connection could not be verified against the issuer's trusted certificates. Typically, this can happen due to a few reasons:
- The server's SSL certificate is self-signed (or not trusted).
- The client lacks the necessary Certificate Authorities (CAs).
- The server certificate has expired or is invalid.
Addressing these issues is crucial for maintaining secure communications in your applications.
Essential Concepts
To remedy the SSLPeerUnverifiedException
, we need to familiarize ourselves with a few concepts:
-
Keystore: This is a file that stores trusted certificates and private keys. When your application connects over SSL, it checks the server's certificate against the contents of the keystore.
-
Truststore: A specific keystore that contains trusted certificates used only for verifying the certificates presented by the remote server.
Let's explore step-by-step methods to solve the SSLPeerUnverifiedException.
Step 1: Validate the Cause
The first step is always to validate the cause of the issue. Check the server’s SSL certificate through a browser or using tools like openssl
command. Run the following command in your terminal:
openssl s_client -connect yourserver.com:443
This will provide detailed information about the certificate, including its expiration, issuer, and validity.
Step 2: Import the Server Certificate into the Truststore
If you determine that the server's certificate isn't trusted due to it being self-signed or not signed by a recognized authority, you'll need to import it into the Java truststore.
Export the certificate
You can export the server certificate using the openssl
command:
openssl s_client -connect yourserver.com:443 -showcerts
Copy the certificate data, including the -----BEGIN CERTIFICATE-----
and -----END CERTIFICATE-----
tags into a file named yourserver.crt
.
Import into Java Truststore
To import the certificate, run the following command:
keytool -import -alias yourserver -keystore cacerts -file yourserver.crt
The default location of cacerts
is typically in the JDK directory:
$JAVA_HOME/jre/lib/security/cacerts
. You'll be prompted for a password (the default is changeit
).
Step 3: Specify the Truststore in Your Application
Now that you've added the certificate, you need to ensure your application uses this truststore. You can achieve this by specifying properties in your application. Below is an example:
System.setProperty("javax.net.ssl.trustStore", "path/to/your/cacerts");
System.setProperty("javax.net.ssl.trustStorePassword", "changeit");
Code Example
This example demonstrates how to create an HTTPS connection after setting the truststore:
import javax.net.ssl.HttpsURLConnection;
import java.net.URL;
public class SSLExample {
public static void main(String[] args) {
try {
System.setProperty("javax.net.ssl.trustStore", "path/to/your/cacerts");
System.setProperty("javax.net.ssl.trustStorePassword", "changeit");
URL url = new URL("https://yourserver.com/api");
HttpsURLConnection connection = (HttpsURLConnection) url.openConnection();
int responseCode = connection.getResponseCode();
if (responseCode == HttpsURLConnection.HTTP_OK) {
System.out.println("Connection successful.");
} else {
System.out.println("Failed to connect. Response code: " + responseCode);
}
connection.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Explanation
- Setup SSL Properties: This code sets up the SSL context properties pointing to your truststore.
- Open Connection: It creates an
HttpsURLConnection
to your specified URL. - Check Response: The response code is checked to confirm the connection has succeeded.
Step 4: Using an Untrusted SSL Context (Not Recommended)
For debugging purposes only, you can use a custom TrustManager
that accepts all certificates. This approach is not recommended for production due to security vulnerabilities but can help diagnose your issue.
import javax.net.ssl.*;
import java.security.cert.X509Certificate;
public class SslTrustAll {
public static void main(String[] args) throws Exception {
TrustManager[] trustAllCerts = new TrustManager[]{new X509TrustManager() {
public X509Certificate[] getAcceptedIssuers() {
return null;
}
public void checkClientTrusted(X509Certificate[] certs, String authType) {}
public void checkServerTrusted(X509Certificate[] certs, String authType) {}
}};
SSLContext sc = SSLContext.getInstance("SSL");
sc.init(null, trustAllCerts, new java.security.SecureRandom());
HttpsURLConnection.setDefaultSSLSocketFactory(sc.getSocketFactory());
// Follow with your connection code here...
}
}
Caution: Use this only temporarily to expedite testing. Deploying such insecure code could leave your application vulnerable.
Step 5: Troubleshooting Common Issues
- Certificate Chain Issues: Ensure that the entire certificate chain is trusted.
- Firewall or Proxy Issues: Sometimes, corporate firewalls or proxies may affect SSL connections. Be sure your environment allows the required traffic.
- Java Version: Make sure you're using an up-to-date version of Java, as older versions may lack support for newer certificates.
The Last Word
In conclusion, the SSLPeerUnverifiedException
can be a significant hurdle when building Java applications that interact securely over HTTPS. By following the steps outlined, you can diagnose the issue, import necessary certificates into your truststore, and configure your application to trust the SSL connection. Remember, while quick fixes like trusting all certificates can help in development, these should be replaced with rigorous security practices in production environments.
For more information on Java security and SSL handling, check out the Oracle Java Documentation.
With this knowledge, you can enhance the security of your Java applications and contribute to creating a safer internet. Happy coding!