Unlocking Java Mysteries: Crafting the Perfect Query
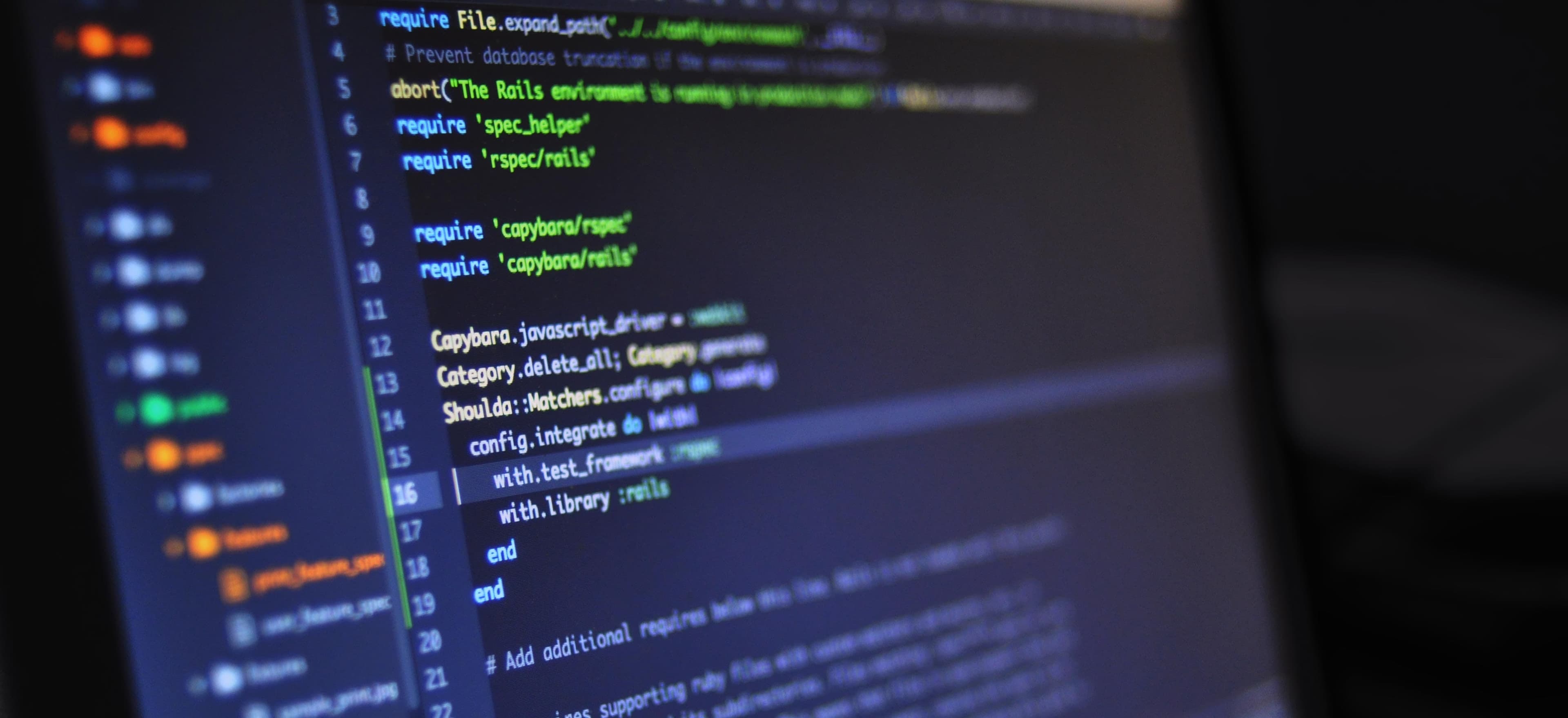
- Published on
Unlocking Java Mysteries: Crafting the Perfect Query
In the world of software development, the ability to ask the right questions—whether to colleagues, databases, or even yourself—is pivotal. Java, one of the most widely-used programming languages, allows developers to build applications that can pull and process data efficiently. In this blog post, we'll explore how to craft the perfect query in Java, paralleling this quest for clarity with concepts from the mystical world of tarot, particularly the insights provided in the article "Tarotkarten: So stellst du die perfekte Frage".
The Importance of Asking the Right Questions in Java
Before we delve into the technical aspects of crafting queries, let's take a moment to discuss the significance of formulating the right questions in software development. Just as in tarot reading—where asking the right question leads to a clearer understanding of the circumstances—the same applies when querying a database. A vague query might result in undesirable outcomes, while a precise one can yield exactly what you need.
In Java, effective querying involves understanding the underlying data structures and using the appropriate method to access information. Let’s consider some fundamental components that play a vital role in formulating queries.
1. Understanding Your Data Model
Before writing any query, understanding the structure of your data is crucial. Whether you are using relational databases, NoSQL databases, or even in-memory data structures, knowing how your data is organized will guide your querying process.
Example: Analyzing an SQL Database
Suppose we have a simple relational database with the following table structure:
CREATE TABLE Users (
id INT PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(100)
);
In Java, you might retrieve users from this database. Knowing that Users
contains an id
, name
, and email
helps us prepare an effective query to extract the needed data.
2. JDBC: Bridging Java and Databases
Java provides JDBC (Java Database Connectivity) for connecting and executing queries on databases. Here's a succinct example of how to use JDBC to connect and execute a simple query:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class DatabaseQueryExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "username";
String password = "password";
try {
Connection connection = DriverManager.getConnection(url, user, password);
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM Users");
while (resultSet.next()) {
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
String email = resultSet.getString("email");
System.out.println("ID: " + id + ", Name: " + name + ", Email: " + email);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary on the Code
This code snippet connects to a MySQL database and retrieves all records from the Users
table.
- The connection URL specifies the database to connect to.
- Using a
Statement
, we can execute standard SQL queries like select. - The
ResultSet
allows us to iterate through each record, making it easy to manipulate and display data.
By understanding how JDBC works, we can begin tailoring our queries to meet specific requirements, much like how tarot practitioners analyze the cards to gain clarity.
3. Crafting Precise Queries
When crafting queries in Java, it's akin to finding the right card in a tarot deck. The more specific you can be, the better your results will be. Let's illustrate this with a refined SQL query:
ResultSet resultSet = statement.executeQuery("SELECT * FROM Users WHERE email = 'john@example.com'");
In this case, we retrieve a specific user by email.
Why Use Prepared Statements?
When working with database queries, especially when user input is involved, it's crucial to utilize Prepared Statements to protect against SQL injection attacks. Here's how to rewrite the above example using a prepared statement:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class PreparedStatementExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "username";
String password = "password";
String emailToSearch = "john@example.com";
try {
Connection connection = DriverManager.getConnection(url, user, password);
String query = "SELECT * FROM Users WHERE email = ?";
PreparedStatement preparedStatement = connection.prepareStatement(query);
preparedStatement.setString(1, emailToSearch);
ResultSet resultSet = preparedStatement.executeQuery();
while (resultSet.next()) {
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
System.out.println("ID: " + id + ", Name: " + name);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
The 'Why' of Prepared Statements
In this code:
- The query is a template;
?
acts as a placeholder for binding parameters. - This prevents SQL injection as user input is treated as data, not executable code.
- Append future-proofing to your code, making it safer and more maintainable.
4. Optimizing Your Queries
Efficiency in queries is crucial. Large datasets can slow down performance. Consider indexing important columns in your database to speed up lookup times.
Example: Adding an Index in SQL
CREATE INDEX idx_email ON Users(email);
In Java, you might not directly deal with indexes, but understanding their implications when querying can help you optimize your data retrieval strategies.
5. Leveraging ORM Tools
For larger projects, consider using an Object-Relational Mapping (ORM) tool like Hibernate or JPA. These frameworks abstract much of the database interaction, allowing you to work with data as Java objects.
Example: Using Hibernate
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class HibernateExample {
public static void main(String[] args) {
SessionFactory factory = new Configuration().configure("hibernate.cfg.xml").addAnnotatedClass(User.class).buildSessionFactory();
Session session = factory.getCurrentSession();
try {
session.beginTransaction();
User user = session.get(User.class, 1);
System.out.println(user);
session.getTransaction().commit();
} finally {
factory.close();
}
}
}
Why Choose ORM?
- Simplicity: You interact with database records as Java objects.
- Maintainability: Business logic is separated from database interactions.
- Portability: Switching databases often requires minimal code changes.
The Closing Argument
Asking the right questions in Java, like in tarot, can unveil deeper insights when analyzing data. By understanding your data model, using JDBC or ORM tools, and constructing precise queries, you unlock the full potential of your applications.
In a world where data is abundant, the capacity to query it effectively is your greatest ally. As you delve into this discipline, remember the wisdom shared in the article "Tarotkarten: So stellst du die perfekte Frage". Just as that article teaches about formulating insightful questions in tarot, so too can we master the art of querying in Java, leading to clear, actionable results.
With this foundation, navigate your Java projects with confidence, ensuring each query is tailored to bring clarity and efficiency to your data retrieval endeavors. Happy coding!