Avoiding Common Big O Mistakes in Java Coding Interviews
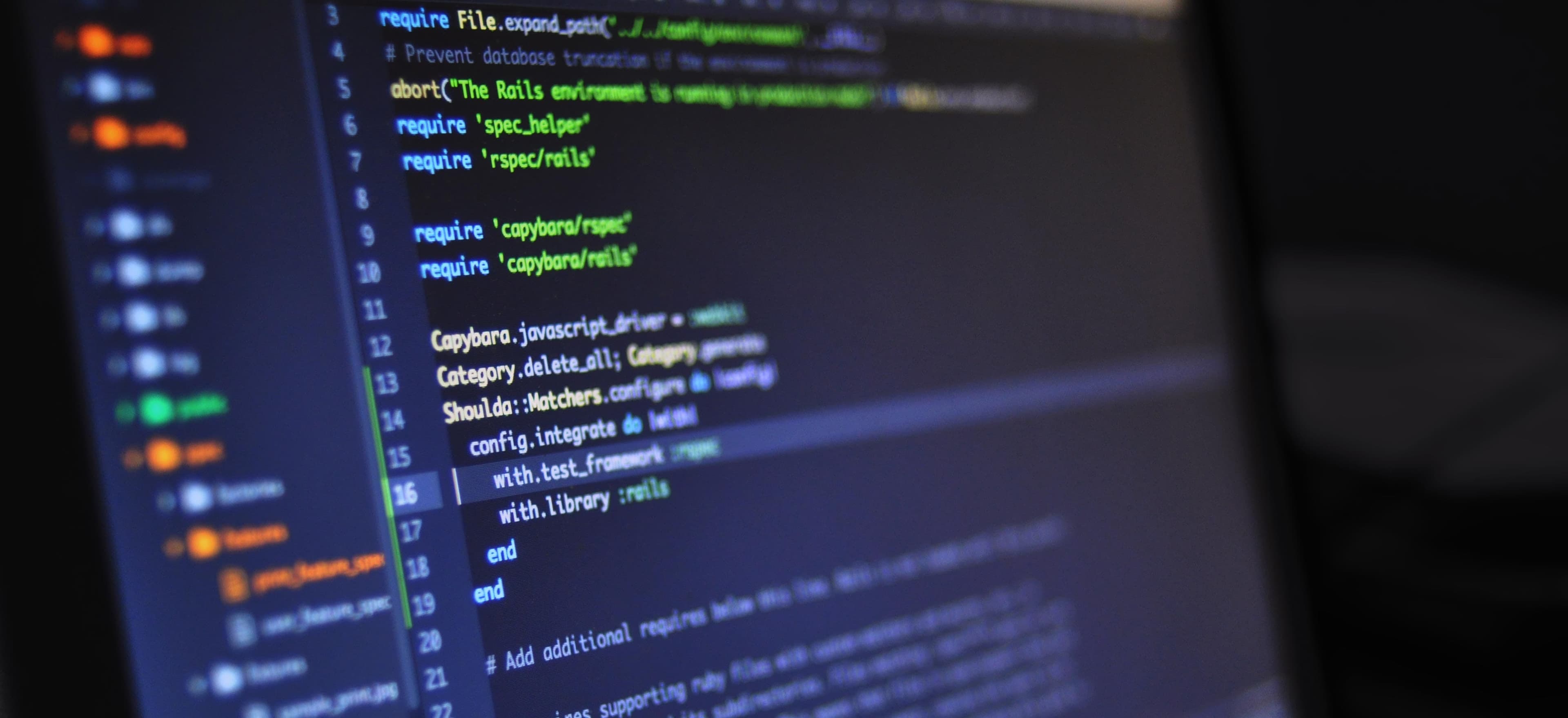
- Published on
Avoiding Common Big O Mistakes in Java Coding Interviews
In the competitive landscape of software engineering, Big O notation has become a fundamental concept for assessing algorithmic efficiency. It's a critical part of coding interviews, where interviewers often gauge your ability to solve problems efficiently. Many candidates, however, fall into common pitfalls when discussing or applying Big O notations. This blog post will delve into those errors, provide insights on mastering Big O concepts, and include Java code snippets to reinforce the learning process.
Understanding Big O Notation
Big O notation is a mathematical representation used to describe the upper bound of an algorithm's running time or space utilization. It provides a framework for comparing the efficiency of different algorithms, especially as the size of the input data increases.
For example:
- O(1): Constant time. The operation does not depend on the size of the input.
- O(n): Linear time. The operation scales linearly with the size of the input.
- O(n^2): Quadratic time. The operation's growth is proportional to the square of the input size.
Code Example: Linear Search
Let's consider a straightforward example of a linear search algorithm that operates in O(n) time complexity.
public class LinearSearch {
public static int linearSearch(int[] arr, int target) {
for (int i = 0; i < arr.length; i++) {
if (arr[i] == target) {
return i; // Target found, returning index
}
}
return -1; // Target not found
}
public static void main(String[] args) {
int[] data = {2, 4, 6, 8, 10};
int target = 6;
int result = linearSearch(data, target);
System.out.println("Target found at index: " + result);
}
}
In this example, we perform a linear search through the array. The time complexity is O(n) because, in the worst-case scenario, we may need to inspect each element in the array.
Common Mistakes in Big O Analysis
While you may have a good grasp of the theoretical aspects of Big O, practical application often leads to common mistakes. Here are a few to watch out for:
1. Ignoring Constants and Lower Order Terms
One frequent error is disregarding constants and lower-order terms when analyzing time complexity. For instance, O(2n) would be simplified to O(n). While constants are indeed disregarded in the worst-case analysis, it can matter in practical applications.
Example Reflection
Consider an algorithm with two nested loops, as shown below:
public class NestedLoops {
public static void printPairs(int[] arr) {
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr.length; j++) {
System.out.print(arr[i] + ", " + arr[j] + "; ");
}
}
}
public static void main(String[] args) {
int[] data = {1, 2, 3};
printPairs(data);
}
}
The time complexity is O(n^2) because we have two nested loops. Learning to recognize such patterns is crucial for avoiding oversimplifications.
2. Misjudging Space Complexity
Many candidates focus strictly on time complexity and neglect space complexity. This oversight can mislead you during interviews, especially when your solution is memory-bound.
Code Example: Recursive Fibonacci
public class Fibonacci {
public static int fibonacci(int n) {
if (n <= 1) {
return n;
}
return fibonacci(n - 1) + fibonacci(n - 2);
}
public static void main(String[] args) {
int result = fibonacci(5);
System.out.println("Fibonacci of 5: " + result);
}
}
The time complexity for the above Fibonacci function is O(2^n) due to the branching factor of 2 for each recursive call. The space complexity, however, is O(n) due to the call stack created by the recursion. Understanding both complexities is essential for effective problem-solving.
3. Failing to Consider Input Size and Type
Input size and type can significantly influence algorithm efficiency. For example, if you're asked to sort a data structure, the chosen sorting algorithm's efficiency will dramatically change based on the data's nature.
Example with Sorting
import java.util.Arrays;
public class QuickSortExample {
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
public static int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
// swap arr[i] and arr[j]
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
// swap arr[i + 1] and arr[high] (or pivot)
int temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
return i + 1;
}
public static void main(String[] args) {
int[] data = {10, 7, 8, 9, 1, 5};
quickSort(data, 0, data.length - 1);
System.out.println("Sorted array: " + Arrays.toString(data));
}
}
QuickSort operates on average in O(n log n) time complexity, but in the worst case, it degrades to O(n^2). Grasping such nuances can elevate your understanding and preparation for interviews.
Resources for Further Learning
Cross-referencing other resources can enhance your knowledge and give you a wider perspective on algorithm efficiency. One useful article is "Mastering Big O Notation: Common Pitfalls in Interviews," which details some of the most common mistakes interview candidates make. You can find it here.
Lessons Learned
Mastering Big O notation is essential for successful coding interviews and efficient algorithm design. By avoiding common pitfalls—like neglecting constants, overlooking space complexity, and not considering input types—you can ensure more accurate analyses of your algorithms.
As you practice, continually challenge yourself to analyze both time and space complexities from different angles. This will not only make you a better coder but also prepare you to ace your next coding interview. Remember, effective preparation bridges the gap between theoretical knowledge and practical application.
Continue building your skills in Java and beyond! Happy coding!