Common Pitfalls in Testing Spring Components with Mockito
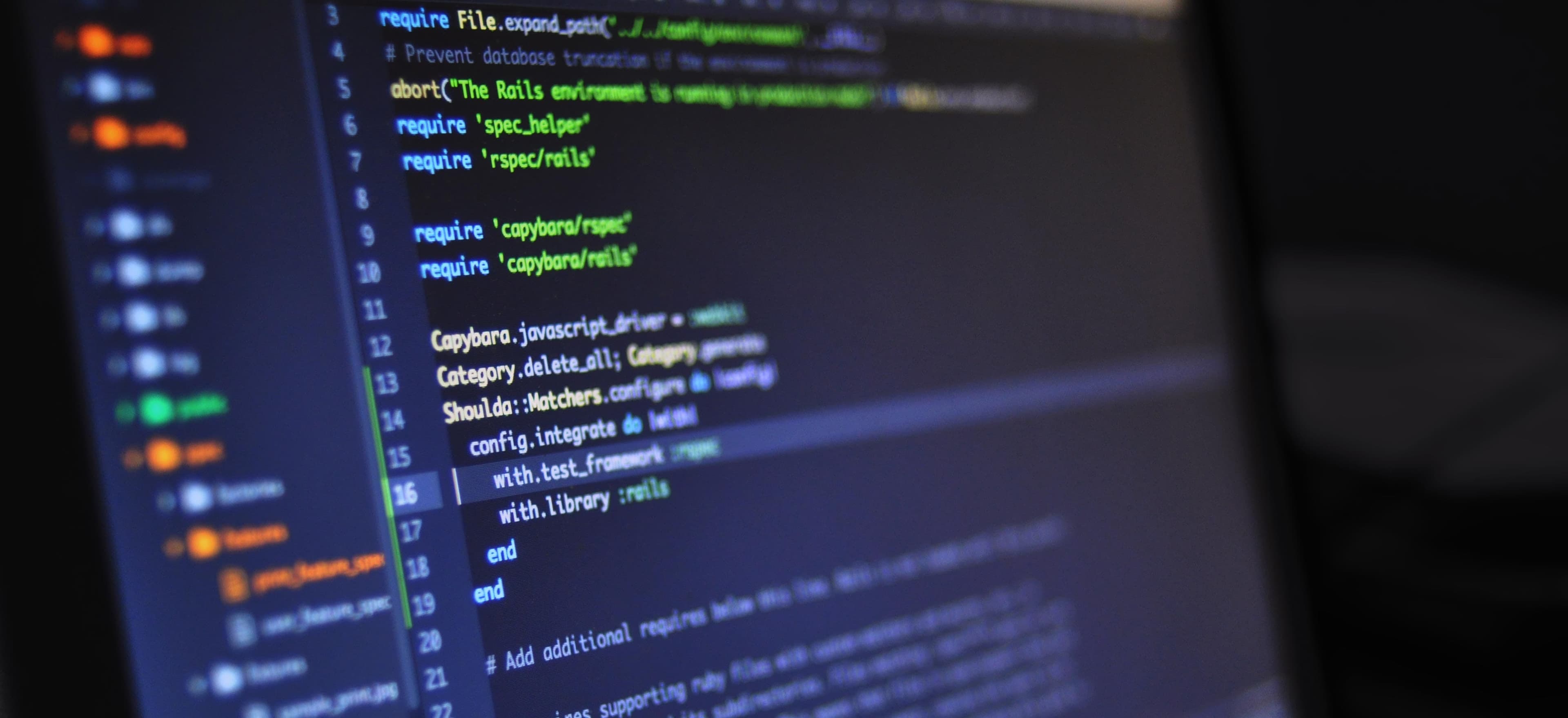
- Published on
Common Pitfalls in Testing Spring Components with Mockito
Testing is a critical aspect of software development, ensuring that applications work as intended and maintain their integrity through changes. When working with Spring applications, utilizing Mockito for testing components has become a widely accepted practice. However, there are common pitfalls developers encounter when writing tests. In this blog post, we will explore these pitfalls, provide best practices, and give examples that highlight effective testing strategies.
Overview of Mockito
Mockito is a popular mocking framework for Java, allowing you to create mock objects and facilitate unit testing. It helps in verifying interactions and stubbing responses in both simple and complex situations. A well-tested Spring application leverages Mockito to create isolated unit tests that accurately reflect business logic without being influenced by other components.
Common Pitfalls in Testing Spring Components with Mockito
1. Incorrectly Setting Up Mocks
One of the most common mistakes developers make is not properly setting up mock objects. This often leads to NullPointerExceptions
during tests, making debugging a cumbersome experience.
Example
@Mock
private MyRepository myRepository;
@InjectMocks
private MyService myService;
@BeforeEach
void setUp() {
MockitoAnnotations.openMocks(this);
}
In the above example, failing to call MockitoAnnotations.openMocks(this)
would prevent the mocks from being instantiated. Ensure that you correctly initialize your mocks; otherwise, they will remain null, leading to unexpected failures.
2. Not Verifying Interactions
Many developers forget to verify interactions between components. While stubbing responses is useful, it is equally important to ensure your code behaves as expected.
Example
@Test
void testSaveEntity() {
Entity entity = new Entity();
myService.save(entity);
verify(myRepository, times(1)).save(entity);
}
In this snippet, we assert that myRepository.save(entity)
was called exactly once. Failing to verify interactions can lead to false confidence in your tests, as they may pass even when the necessary interactions don't occur.
3. Over-Mocking
Over-mocking occurs when you create mock objects for dependencies that don’t need to be mocked. For simpler data classes or behavior that can be easily instantiated, mocks may add unnecessary complexity.
Example
@Mock
private MyService myService; // Over-mocking
@Test
void testWithoutMocking() {
Entity entity = new Entity("Sample");
assertEquals("Sample", entity.getName());
}
In this instance, mocking MyService
is unnecessary since the test is only validating the Entity
class's behavior. Use mocks wisely to maintain test readability and clarity.
4. Ignoring Exception Scenarios
Tests should not only focus on positive outcomes but also assess how your code reacts to exceptional situations. Neglecting to implement tests for exceptions can hide critical bugs.
Example
@Test
void testSaveEntity throws DataAccessException {
doThrow(new DataAccessException("DB Error")).when(myRepository).save(any(Entity.class));
assertThrows(DataAccessException.class, () -> myService.save(new Entity()));
}
Here, we simulate a failure from myRepository
and assert that the exception is thrown in the service layer. This verification is vital for understanding how your application will respond to real-world errors.
5. Using @Mock
Instead of @Spy
Sometimes, you may need to test partial behavior of a class without losing its original capabilities. Using @Mock
will limit you since mocks do not invoke the actual method implementations. Instead, consider using @Spy
.
Example
@Spy
private MyService myService;
@Test
void testPartialMock() {
myService.save(new Entity());
verify(myService).save(any(Entity.class));
// Ensure the real method is also invoked
assertEquals(1, myService.getSavedEntitiesCount());
}
In this example, @Spy
allows us to capture interactions while still using the actual method logic. This can be particularly useful for logging or collecting metrics during execution.
Learn more about Spys in Mockito here.
6. Lack of Test Coverage
Conducting thorough testing is essential; however, many developers erroneously skip edge cases that could lead to unreliable results. Strive to achieve comprehensive coverage across methods.
Code Quality Metric
Utilize tools like JaCoCo to assess code coverage within your Spring application. Aim for a balance between achieving satisfactory coverage levels and maintaining high-quality code.
<dependency>
<groupId>org.jacoco</groupId>
<artifactId>jacoco-maven-plugin</artifactId>
<version>0.8.5</version>
<scope>test</scope>
</dependency>
Example Coverage Assessment
After executing your tests, check the report generated by JaCoCo for methods or branches lacking test coverage. Address these areas to ensure robustness.
7. Dependency on External Systems
Unit tests should be isolated; relying on external systems such as databases, APIs, or message queues reduces your test's reliability and consistency.
Mocking External Calls
Ensure any call to external systems is mocked. For instance, when testing a service method that fetches data from an API, mock the API response instead of making an actual HTTP call.
when(myApiClient.getData()).thenReturn(mockedResponse);
By mocking the external call, you avoid complications related to network latency and availability, ensuring consistent test outcomes.
Closing the Chapter
Testing Spring components using Mockito can be straightforward, but several pitfalls threaten the reliability of your tests. By being aware of these common mistakes—incorrectly setting up mocks, failing to verify interactions, over-mocking, neglecting exception scenarios, and lack of coverage—you can craft better unit tests.
Mockito offers powerful features that, when used correctly, can lead to a robust testing framework. Always remember to design your tests around your business requirements, focusing on both positive and negative scenarios.
For more detailed examples and techniques, consider checking the official Mockito documentation.
By avoiding these common pitfalls, you can ensure that your Spring applications are not only well-tested but also maintainable and resilient to changes. Happy testing!
Checkout our other articles